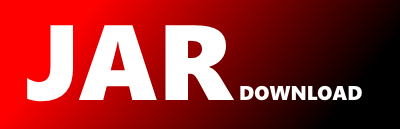
leap.lang.convert.MapConverter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package leap.lang.convert;
import java.lang.reflect.Type;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Map.Entry;
import leap.lang.NamedWithSetter;
import leap.lang.Out;
import leap.lang.Strings;
import leap.lang.Types;
import leap.lang.reflect.Reflection;
@SuppressWarnings("rawtypes")
public class MapConverter extends AbstractConverter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy