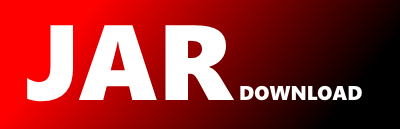
leap.lang.csv.CSV Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package leap.lang.csv;
import leap.lang.Args;
import leap.lang.Exceptions;
import leap.lang.enums.CaseType;
import leap.lang.exception.NestedIOException;
import leap.lang.io.IO;
import leap.lang.resource.Resource;
import leap.lang.resource.Resources;
import java.io.*;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
//Most codes in this package from apache commons-csv
public class CSV {
private static final CSVFormat DEFAULT_FORMAT = CSVFormat.DEFAULT.withIgnoreSurroundingSpaces(true);
private static final CSVFormat DEFAULT_FORMAT_SKIP_HEADER = DEFAULT_FORMAT.withSkipHeaderRecord(true);
public static List read(Reader reader) throws NestedIOException{
return readList(reader, DEFAULT_FORMAT);
}
public static List readSkipHeader(Reader reader) {
return readList(reader, DEFAULT_FORMAT_SKIP_HEADER);
}
protected static List readList(Reader reader,CSVFormat format) throws NestedIOException {
Args.notNull(reader,"reader");
CSVParser parser = null;
try{
parser = new CSVParser(reader, format);
return parser.getRecords1();
}catch(IOException e){
throw Exceptions.wrap(e);
}finally{
IO.close(reader);
IO.close(parser);
}
}
public static void read(Reader reader,CsvProcessor processor) throws NestedIOException {
CSVParser parser = null;
try{
parser = new CSVParser(reader, DEFAULT_FORMAT);
String[] row;
int rownum = 0;
while((row = parser.nextRecord1()) != null){
rownum++;
processor.process(rownum,row);
}
}catch(IOException e){
throw Exceptions.wrap(e);
}catch(Exception e){
throw Exceptions.uncheck(e);
}finally{
IO.close(reader);
IO.close(parser);
}
}
public static void write(Writer writer,List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy