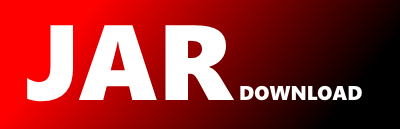
leap.lang.jdbc.SimpleWhereBuilder Maven / Gradle / Ivy
The newest version!
/*
*
* * Copyright 2019 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
package leap.lang.jdbc;
import leap.lang.Arrays2;
import leap.lang.Collections2;
import leap.lang.Strings;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
public class SimpleWhereBuilder implements WhereBuilder {
private final StringBuilder where;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy