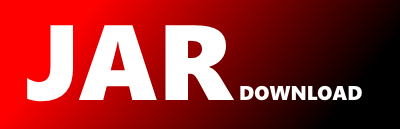
leap.lang.jdbc.StatementProxy Maven / Gradle / Ivy
The newest version!
/*
*
* * Copyright 2016 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
package leap.lang.jdbc;
import leap.lang.logging.Log;
import leap.lang.logging.LogFactory;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class StatementProxy extends StatementWrapper {
private static final Log log = LogFactory.get(StatementProxy.class);
protected final T conn;
protected final Exception stackTraceException;
protected long lastExecutingTime;
protected long lastExecutingDurationMs;
protected String lastExecutingSql;
protected boolean closed;
public StatementProxy(T connection, Statement stmt) {
this(connection, stmt, false);
}
public StatementProxy(T connection, Statement stmt, boolean stackTrace) {
super(stmt);
this.conn = connection;
this.stackTraceException = stackTrace ? new Exception("") : null;
}
public final Statement wrapped() {
return stmt;
}
public final String getLastExecutingSql() {
return lastExecutingSql;
}
public final long getLastExecutingTime() {
return lastExecutingTime;
}
public final long getLastExecutingDurationMs() {
return lastExecutingDurationMs;
}
public StackTraceElement[] getStackTraceOnOpen() {
return conn.getStackTrace(stackTraceException);
}
@Override
public final Connection getConnection() throws SQLException {
return conn;
}
@Override
public final ResultSet getResultSet() throws SQLException {
return proxyOfResultSet(super.getResultSet());
}
@Override
public final ResultSet getGeneratedKeys() throws SQLException {
return proxyOfResultSet(super.getGeneratedKeys());
}
@Override
public final ResultSet executeQuery(String sql) throws SQLException {
try {
beginExecute(sql);
return proxyOfResultSet(stmt.executeQuery(sql));
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final int executeUpdate(String sql) throws SQLException {
try {
beginExecute(sql);
return stmt.executeUpdate(sql);
}catch(SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final boolean execute(String sql) throws SQLException {
try {
beginExecute(sql);
return stmt.execute(sql);
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final void addBatch(String sql) throws SQLException {
this.lastExecutingSql = sql;
try {
super.addBatch(sql);
}catch (SQLException e) {
throw handleSQLException(e);
}
}
@Override
public final int[] executeBatch() throws SQLException {
try {
beginExecute(null);
return stmt.executeBatch();
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final int executeUpdate(String sql, int autoGeneratedKeys) throws SQLException {
try {
beginExecute(sql);
return stmt.executeUpdate(sql, autoGeneratedKeys);
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final int executeUpdate(String sql, int[] columnIndexes) throws SQLException {
try {
beginExecute(sql);
return stmt.executeUpdate(sql, columnIndexes);
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final int executeUpdate(String sql, String[] columnNames) throws SQLException {
try {
beginExecute(sql);
return stmt.executeUpdate(sql, columnNames);
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final boolean execute(String sql, int autoGeneratedKeys) throws SQLException {
try {
beginExecute(sql);
return stmt.execute(sql, autoGeneratedKeys);
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final boolean execute(String sql, int[] columnIndexes) throws SQLException {
try {
beginExecute(sql);
return stmt.execute(sql, columnIndexes);
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final boolean execute(String sql, String[] columnNames) throws SQLException {
try {
beginExecute(sql);
return stmt.execute(sql, columnNames);
}catch (SQLException e) {
throw handleSQLException(e);
}finally{
endExecute();
}
}
@Override
public final boolean isClosed() throws SQLException {
return closed;
}
@Override
public final void close() throws SQLException {
if(closed) {
log.warn("Invalid state, the proxy statement already closed", new Exception("Statement already closed"));
return;
}
this.closed = true;
conn.closeStatement(this);
}
protected void beginExecute(String sql) {
lastExecutingTime = System.currentTimeMillis();
lastExecutingDurationMs = -1;
if(null != sql) {
lastExecutingSql = sql;
}
}
protected void endExecute() {
lastExecutingDurationMs = System.currentTimeMillis() - lastExecutingTime;
lastExecutingTime = -1;
conn.endExecuteStatement(this);
}
protected SQLException handleSQLException(SQLException e) {
return e;
}
protected ResultSet proxyOfResultSet(ResultSet rs) {
return new ResultSetProxy(this, rs);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy