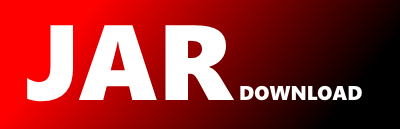
leap.lang.json.JsonObject Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package leap.lang.json;
import leap.lang.Strings;
import leap.lang.convert.BeanConverter;
import leap.lang.convert.Converts;
import leap.lang.exception.ObjectExistsException;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
@SuppressWarnings({"rawtypes","unchecked"})
public class JsonObject implements JsonValue {
private static final BeanConverter BEAN_CONVERTER = new BeanConverter();
public static JsonObject of(Map map) {
return new JsonObject(map);
}
private final Map map;
public JsonObject(Map map){
this.map = map;
}
@Override
public Object raw() {
return map;
}
@Override
public Map asMap() {
return map;
}
@Override
public JsonObject asJsonObject() {
return this;
}
/**
* Returns the property keys of json object.
*/
public Set keys() {
return map.keySet();
}
/**
* Returns the property values of json object.
*/
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy