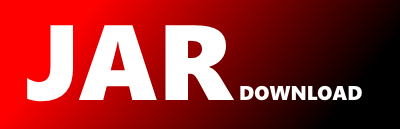
leap.lang.yaml.YamlCollection Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package leap.lang.yaml;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import leap.lang.Emptiable;
import leap.lang.collection.UnmodifiableIteratorBase;
import leap.lang.convert.Converts;
@SuppressWarnings({"unchecked","rawtypes"})
public class YamlCollection implements Iterable,Emptiable,YamlValue {
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy