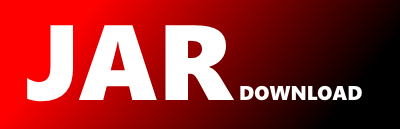
leap.oauth2.server.userinfo.AuthzUserInfo Maven / Gradle / Ivy
/*
*
* * Copyright 2013 the original author or authors.
* *
* * Licensed under the Apache License, Version 2.0 (the "License");
* * you may not use this file except in compliance with the License.
* * You may obtain a copy of the License at
* *
* * http://www.apache.org/licenses/LICENSE-2.0
* *
* * Unless required by applicable law or agreed to in writing, software
* * distributed under the License is distributed on an "AS IS" BASIS,
* * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* * See the License for the specific language governing permissions and
* * limitations under the License.
*
*/
package leap.oauth2.server.userinfo;
import java.util.Map;
/**
* Standard End-User claim
*
* @see OpenId Connect
*
* @author kael.
*/
public interface AuthzUserInfo {
String SUBJECT = "sub";
String NAME = "name";
String GIVEN_NAME = "given_name";
String FAMILY_NAME = "family_name";
String MIDDLE_NAME = "middle_name";
String NICKNAME = "nickname";
String PREFERRED_USERNAME = "preferred_username";
String PROFILE = "profile";
String PICTURE = "picture";
String WEBSITE = "website";
String EMAIL = "email";
String EMAIL_VERIFIED = "email_verified";
String GENDER = "gender";
String BIRTHDATE = "birthdate";
String ZONEINFO = "zoneinfo";
String LOCALE = "locale";
String PHONE_NUMBER = "phone_number";
String PHONE_NUMBER_VERIFIED = "phone_number_verified";
String ADDRESS = "address";
String UPDATED_AT = "updated_at";
/**
* Returns sub or null
*/
String getSubject();
/**
* Returns name or null
*/
String getFullName();
/**
* Returns given_name or null
*/
String getGivenName();
/**
* Returns family_name or null
*/
String getFamilyName();
/**
* Returns middle_name or null
*/
String getMiddleName();
/**
* Returns nickname or null
*/
String getNickname();
/**
* Returns preferred_username or null
*/
String getPreferredUsername();
/**
* Returns profile or null
*
* url format
*/
String getProfile();
/**
* Returns picture or null
*
* url format
*
*/
String getPicture();
/**
* Returns website or null
*/
String getWebsite();
/**
* Returns email or null
*/
String getEmail();
/**
* Returns trur
if this email has been verified.
*/
boolean isEmailVerified();
/**
* Returns gender or null
*/
String getGender();
/**
* Returns birthdate or null
*
* YYYY-MM-DD
format or YYYY
format
*/
String getBirthdate();
/**
* Returns zoneinfo or null
*/
String getZoneinfo();
/**
* Returns locale or null
*/
String getLocale();
/**
* Returns phone number or null
*/
String getPhoneNumber();
/**
* Returns true
if this phone number has been verified.
*/
boolean isPhoneNumberVerified();
/**
* Returns address or null
*/
AuthzAddress getAddress();
/**
* Returns updated_at or null
*/
long getUpdatedAt();
/**
* Convert this object to a map
*/
Map toMap();
/**
* Returns an unmodifiable extend properties map
*/
Map getExtProperties();
/**
* put an extend property
*/
void putExtProperty(String name, Object value);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy