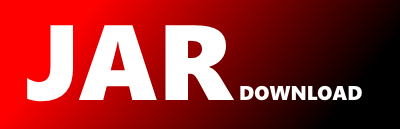
leap.oauth2.server.OAuth2AuthzServerConfig Maven / Gradle / Ivy
/*
* Copyright 2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package leap.oauth2.server;
import java.security.PrivateKey;
import java.security.PublicKey;
import leap.core.AppConfig;
import leap.core.security.token.jwt.JwtVerifier;
import leap.oauth2.server.client.AuthzClientStore;
import leap.oauth2.server.code.AuthzCodeStore;
import leap.oauth2.server.sso.AuthzSSOStore;
import leap.oauth2.server.token.AuthzTokenStore;
/**
* The configuration of oauth2 authorization server.
*/
public interface OAuth2AuthzServerConfig {
/**
* Returns true
if oauth2 authorization server is enabled.
*
*
* Default is false
.
*/
boolean isEnabled();
/**
* Returns true
if enables to cleanup expired tokens, authorization codes, etc.
*
*
* Default is true
.
*/
boolean isCleanupEnabled();
/**
* Returns the interval in seconds for cleanup expired tokens, authorization codes, etc.
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_CLEANUP_INTERVAL}.
*/
int getCleanupInterval();
/**
* Returns true
if the auth server accepts https request only.
*
*
* Default is true
.
*/
boolean isHttpsOnly();
/**
* Returns
if single login enabled.
*
*
* Default is true
.
*/
boolean isSingleLoginEnabled();
/**
* Returns true
if single logout enabled.
*
*
* Default is true
.
*/
boolean isSingleLogoutEnabled();
/**
* Returns true
if user info (endpoint) enabled.
*
*
* Default is true
.
*/
boolean isUserInfoEnabled();
/**
* Returns true
if client credentials grant type is allowed.
*
*
* Default is true
.
*/
boolean isClientCredentialsEnabled();
/**
* Returns true
if token client grant type is allowed.
*
*
* Default is true
.
*/
boolean isTokenClientEnabled();
/**
* Returns true
if password credentials grant type is allowed.
*
*
* Default is true
.
*/
boolean isPasswordCredentialsEnabled();
boolean isRequestLevelScopeEnabled();
/**
* Returns true
if authorization code flow enabled.
*
*
* Default is true
.
*/
boolean isAuthorizationCodeEnabled();
/**
* todo: doc
*/
boolean isSessionRefreshEnabled();
/**
* Returns true
if implicit flow enabled.
*
*
* Default is true
*/
boolean isImplicitGrantEnabled();
/**
* Returns the path of authorization endpoint.
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_AUTHZ_ENDPOINT_PATH}.
*/
String getAuthzEndpointPath();
/**
* Returns the path of token endpoint.
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_TOKEN_ENDPOINT_PATH}.
*/
String getTokenEndpointPath();
/**
* Returns the path of tokeninfo endpoint.
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_TOKENINFO_ENDPOINT_PATH}.
*/
String getTokenInfoEndpointPath();
/**
* todo: doc
*/
String getSessionRefreshEndpointPath();
/**
* Returns the path of logout endopint.
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_LOGOUT_ENDPOINT_PATH}.
*/
String getLogoutEndpointPath();
/**
* Returns the path of userinfo endpoint.
*
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_USERINFO_ENDPOINT_PATH}.
*/
String getUserInfoEndpointPath();
/**
* todo : doc
*/
String getPublicKeyEndpointPath();
/**
* Retruns the error view for rendering oauth2 request error.
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_ERROR_VIEW}.
*/
String getErrorView();
/**
* Returns the login view or null
if use default login flow.
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_LOGIN_VIEW}.
*/
String getLoginView();
/**
* Returns the logout view or null
use default.
*
*
* Default is {@link OAuth2AuthzServerConfigurator#DEFAULT_LOGOUT_VIEW}.
*/
String getLogoutView();
/**
* Returns the default expires in (seconds) of access token.
*/
int getDefaultAccessTokenExpires();
/**
* Returns the default expires in (seconds) of refresh token.
*/
int getDefaultRefreshTokenExpires();
/**
* Returns the default expires in (seconds) of authorization code.
*/
int getDefaultAuthorizationCodeExpires();
/**
* Returns the default expires in (seconds) of login token.
*/
int getDefaultLoginTokenExpires();
/**
* Returns the default expires in (seconds) of id token for Open ID Connect.
*/
int getDefaultIdTokenExpires();
/**
* Returns the default expires in (seconds) of a sso login session.
*/
int getDefaultLoginSessionExpires();
/**
* Returns the datasource name if use jdbc store.
*/
String getJdbcDataSourceName();
/**
* Returns the global private key of authz server.
*
*
* Returns null
if the private key not configured.
*/
PrivateKey getPrivateKey();
/**
* Returns the global public key of authz server.
*
*
* Returns null
if the public key not configured.
*/
PublicKey getPublicKey();
/**
* Returns the jwt verifier
*/
JwtVerifier getJwtVerifier();
/**
* Returns the global private key of authz server.
*
*
* Returns the private key from {@link AppConfig#ensureGetPrivateKey()} if not configured.
*/
PrivateKey ensureGetPrivateKey();
/**
* Returns the {@link AuthzClientStore}.
*/
AuthzClientStore getClientStore();
/**
* Returns the {@link AuthzCodeStore}.
*/
AuthzCodeStore getCodeStore();
/**
* Returns the {@link AuthzTokenStore}.
*/
AuthzTokenStore getTokenStore();
/**
* Returns the {@link AuthzSSOStore}
*/
AuthzSSOStore getSSOStore();
}