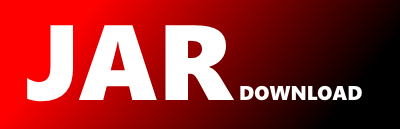
org.ligoj.app.plugin.prov.AbstractProvQuoteInstanceOsResource Maven / Gradle / Ivy
/*
* Licensed under MIT (https://github.com/ligoj/ligoj/blob/master/LICENSE)
*/
package org.ligoj.app.plugin.prov;
import java.util.List;
import org.apache.commons.lang3.ObjectUtils;
import org.apache.commons.lang3.StringUtils;
import org.ligoj.app.plugin.prov.dao.BaseProvTermPriceOsRepository;
import org.ligoj.app.plugin.prov.model.AbstractInstanceType;
import org.ligoj.app.plugin.prov.model.AbstractQuoteVm;
import org.ligoj.app.plugin.prov.model.AbstractQuoteVmOs;
import org.ligoj.app.plugin.prov.model.AbstractTermPriceVmOs;
import org.ligoj.app.plugin.prov.model.ProvInstancePrice;
import org.ligoj.app.plugin.prov.model.QuoteVmOs;
import org.ligoj.app.plugin.prov.model.VmOs;
import org.ligoj.bootstrap.core.validation.ValidationJsonException;
import lombok.extern.slf4j.Slf4j;
/**
* The resource part of the provisioning.
*
* @param The instance resource type.
* @param Quoted resource price type.
* @param Quoted resource type.
* @param Quoted resource edition VO type.
* @param Quoted resource lookup result type.
* @param Quoted resource details type.
*/
@Slf4j
public abstract class AbstractProvQuoteInstanceOsResource, C extends AbstractQuoteVmOs, E extends AbstractQuoteInstanceOsEditionVo, L extends AbstractLookup
, Q extends QuoteVmOs>
extends AbstractProvQuoteVmResource {
@Override
public abstract BaseProvTermPriceOsRepository getIpRepository();
/**
* Check the requested OS is compliant with the one of associated {@link ProvInstancePrice}
*
* @param entity The instance to check.
*/
protected void checkOs(final AbstractQuoteVmOs entity) {
final var service = getService(entity.getConfiguration());
if (service.getCatalogOs(entity.getOs()) != entity.getPrice().getOs()) {
// Incompatible, hack attempt?
log.warn("Attempt to create an instance with an incompatible OS {} with catalog OS {}", entity.getOs(),
entity.getPrice().getOs());
throw new ValidationJsonException("os", "incompatible-os", entity.getPrice().getOs());
}
}
/**
* Return true
if the current OS accept BYOL.
*
* @param os The OS to evaluate.
* @return true
if the current OS accept BYOL.
*/
protected boolean canByol(final VmOs os) {
return os == VmOs.WINDOWS;
}
@Override
protected void saveOrUpdateSpec(final C entity, final E vo) {
entity.setOs(ObjectUtils.defaultIfNull(vo.getOs(), entity.getPrice().getOs()));
entity.setEphemeral(vo.isEphemeral());
entity.setMaxVariableCost(vo.getMaxVariableCost());
entity.setInternet(vo.getInternet());
checkOs(entity);
}
/**
* Return the available instance licenses for a subscription.
*
* @param subscription The subscription identifier, will be used to filter the instances from the associated
* provider.
* @param os The filtered OS.
* @return The available licenses for the given subscription.
*/
public List findLicenses(final int subscription, final VmOs os) {
final var result = getIpRepository()
.findAllLicenses(subscriptionResource.checkVisible(subscription).getNode().getTool().getId(), os);
result.replaceAll(l -> StringUtils.defaultIfBlank(l, AbstractQuoteVm.LICENSE_INCLUDED));
return result;
}
/**
* Return the available instance OS names for a subscription.
*
* @param subscription The subscription identifier, will be used to filter the instances from the associated
* provider.
* @return The available OS names for the given subscription.
*/
public List findOs(final int subscription) {
return getIpRepository().findAllOs(subscriptionResource.checkVisible(subscription).getNode().getTool().getId());
}
}