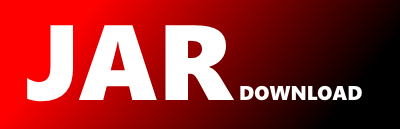
org.ligoj.app.plugin.prov.Workload Maven / Gradle / Ivy
The newest version!
/*
* Licensed under MIT (https://github.com/ligoj/ligoj/blob/master/LICENSE)
*/
package org.ligoj.app.plugin.prov;
import java.util.List;
import java.util.stream.Stream;
import org.apache.commons.lang3.StringUtils;
import lombok.AllArgsConstructor;
import lombok.Getter;
/**
* Efficient baseline with detailed CPU workload.
*/
@Getter
public class Workload {
private final double baseline;
private final List periods;
/**
* Default baseline value.
*/
private static final double DEFAULT_BASELINE = 5d;
/**
* Default workload profile.
*/
private static final Workload DEFAULT_WORKLOAD = new Workload(DEFAULT_BASELINE,
new String[] { null, "100@" + DEFAULT_BASELINE });
/**
* Full workload profile.
*/
private static final Workload FULL_WORKLOAD = new Workload(100, new String[] { null, "100@100" });
private Workload(double baseline, String[] parts) {
this.baseline = baseline;
if (parts.length == 1) {
this.periods = List.of(new WorkloadPeriod(100, baseline));
} else {
this.periods = Stream.of(parts).skip(1).map(p -> {
final var periodParts = StringUtils.split(p, '@');
return new WorkloadPeriod(Double.parseDouble(periodParts[0]), Double.parseDouble(periodParts[1]));
}).toList();
}
}
@AllArgsConstructor
static class WorkloadPeriod {
double duration;
double value;
}
/**
* This string follows this pattern: $baseline(,$duration@$cpu)*
. Sample value:
* 80,20@55,10@23,65@10
. Constraints:
*
* - Weighted average of values corresponds to the baseline
* - When there is no details (only the baseline), no computation has to be done. Min is
0
and max is
* 100
.
* - Sum of durations should be
100
. When different than 100
a pro rata is applied to
* each value according to their weight.
* - Min value's duration is
0
* - Max value's duration should be
100
, however adjustment is applied to be aligned to
* 100
* - Min value's COU is
0
* - Max value's CPU is
100
*
*
* @param rawData The raw data containing baseline and optional details.
* @return The Workload entity built from this raw value.
*/
public static Workload from(final String rawData) {
if (rawData == null) {
return DEFAULT_WORKLOAD;
}
final var parts = StringUtils.split(rawData, ',');
final var baseline = Double.parseDouble(parts[0]);
if (baseline == 100d) {
return FULL_WORKLOAD;
}
return new Workload(baseline, parts);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy