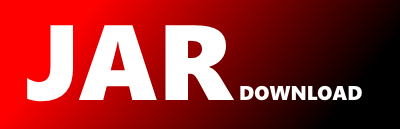
org.ligoj.app.plugin.prov.model.ProvQuote Maven / Gradle / Ivy
The newest version!
/*
* Licensed under MIT (https://github.com/ligoj/ligoj/blob/master/LICENSE)
*/
package org.ligoj.app.plugin.prov.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import lombok.Getter;
import lombok.Setter;
import org.hibernate.annotations.ColumnDefault;
import org.ligoj.app.model.PluginConfiguration;
import org.ligoj.app.model.Subscription;
import org.ligoj.app.plugin.prov.ProvisioningService;
import org.ligoj.bootstrap.core.model.AbstractDescribedAuditedEntity;
import jakarta.persistence.*;
import jakarta.validation.constraints.Max;
import jakarta.validation.constraints.Min;
import jakarta.validation.constraints.NotNull;
import jakarta.validation.constraints.PositiveOrZero;
import java.util.ArrayList;
import java.util.List;
/**
* A saved quote.
*/
@Getter
@Setter
@Entity
@Table(name = "LIGOJ_PROV_QUOTE")
public class ProvQuote extends AbstractDescribedAuditedEntity
implements PluginConfiguration, Costed, ResourceScope {
/**
* SID
*/
private static final long serialVersionUID = 1L;
/**
* Minimal monthly cost, computed during the creation and kept synchronized with the updates. Includes support cost.
*/
@NotNull
@PositiveOrZero
private double cost = 0d;
/**
* Maximal determined monthly cost, computed during the creation and kept synchronized with the updates. Includes
* support cost. When there are unbound maximal quantities (unboundCostCounter > 0
), the
* {@link #unboundCostCounter} is incremented. Otherwise, this value is equals to {@link #cost}
*/
@NotNull
@PositiveOrZero
private double maxCost = 0d;
/**
* Minimal initial cost. Does not include support cost.
*/
@PositiveOrZero
private double initialCost = 0d;
/**
* Maximal initial cost. Does not include support cost.
*
* @see #maxCost
*/
@PositiveOrZero
private double maxInitialCost = 0d;
/**
* Minimal monthly support cost, computed during the creation and kept synchronized with the updates.
*/
@PositiveOrZero
@NotNull
private Double costSupport = 0d;
/**
* Maximal monthly support cost, computed during the creation and kept synchronized with the updates.
*/
@PositiveOrZero
@NotNull
private Double maxCostSupport = 0d;
/**
* Minimal monthly cost without cost, computed during the creation and kept synchronized with the updates.
*/
@PositiveOrZero
@NotNull
private Double costNoSupport = 0d;
/**
* Maximal monthly cost without cost, computed during the creation and kept synchronized with the updates.
*/
@PositiveOrZero
@NotNull
private Double maxCostNoSupport = 0d;
/**
* The amount unbound maximal quantities. Would be used to track the unbound monthly bills on different scenarios.
*/
@NotNull
@PositiveOrZero
private Integer unboundCostCounter = 0;
/**
* The related subscription.
*/
@NotNull
@ManyToOne
@JsonIgnore
private Subscription subscription;
/**
* Quoted instance.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List instances;
/**
* Quoted databases.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List databases;
/**
* Quoted containers.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List containers;
/**
* Quoted functions.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List functions;
/**
* Usages associated to this quote.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List usages = new ArrayList<>();
/**
* Budgets associated to this quote.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List budgets = new ArrayList<>();
/**
* Optimizers associated to this quote.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List optimizers = new ArrayList<>();
/**
* Quoted storages.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List storages;
/**
* Quoted supports.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List supports = new ArrayList<>();
/**
* Attached tags.
*/
@OneToMany(mappedBy = "configuration", cascade = CascadeType.REMOVE)
@JsonIgnore
private List tags;
/**
* UI settings. Properties are:
*
* - Attached tags colors mapping as a JSON map. Key is the tag name. Value is the color code. Color name is not
* accepted. Sample:
#e4560f
or rgb(255, 0, 0)
, hsl(0, 100%, 50%)
.
*
*/
private String uiSettings;
/**
* Default location constraint.
*/
@ManyToOne
@NotNull
private ProvLocation location;
/**
* Optional default usage. When null
, full time.
*/
@ManyToOne
private ProvUsage usage;
/**
* Optional default budget. When null
, full time.
*/
@ManyToOne
private ProvBudget budget;
/**
* Optional default optimizer. When null
, full time.
*/
@ManyToOne
private ProvOptimizer optimizer;
/**
* Optional license model. null
value corresponds to {@value ProvQuoteInstance#LICENSE_INCLUDED}.
*/
private String license;
/**
* Rate applied to required RAM to find the suiting instance type. This rate is divided by 100
, then
* multiplied to the required RAM of each memory before calling the lookup. Value lesser than 100
* allows the lookup to elect an instance having less RAM than the requested one. Value greater than
* 100
makes the lookup to request instance types providing more RAM than the requested one.
*/
@Min(50)
@Max(150)
private Integer ramAdjustedRate = 100;
/**
* Optional reservation mode. By default is {@link org.ligoj.app.plugin.prov.model.ReservationMode#RESERVED}
*/
private ReservationMode reservationMode = ReservationMode.RESERVED;
/**
* Optional currency. When null
, the currency is USD
with 1
rate.
*/
@ManyToOne
private ProvCurrency currency;
/**
* Optional processor. May be null
.
*/
private String processor;
/**
* Optional physical host requirement. May be null
. When true
, this instance type is
* physical, not virtual.
*/
private Boolean physical;
@Override
@JsonIgnore
public boolean isUnboundCost() {
return unboundCostCounter > 0;
}
@Override
@JsonIgnore
public ProvQuote getConfiguration() {
return this;
}
/**
* Resolved service instance. May be null
while unresolved.
*/
@Transient
@JsonIgnore
private transient ProvisioningService service;
/**
* Lock object for lean operation.
*/
@Transient
@JsonIgnore
private final Object leanLock = new Object();
/**
* When true
, the lean process is executed after each change. This option implies more computations
* when there are budgets with associated initial cost.
*/
private Boolean leanOnChange = false;
/**
* Minimal monthly CO2 consumption, computed during the creation and kept synchronized with the updates.
*/
@NotNull
@PositiveOrZero
@ColumnDefault("0")
private double co2 = 0d;
/**
* Maximal determined monthly CO2 consumption, computed during the creation and kept synchronized with the updates.
* When there are unbound maximal quantities (unboundCostCounter > 0
), the
* {@link #unboundCostCounter} is incremented. Otherwise, this value is equals to {@link #co2}
*/
@NotNull
@PositiveOrZero
@ColumnDefault("0")
private double maxCo2 = 0d;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy