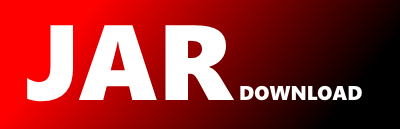
org.linguafranca.pwdb.kdbx.jaxb.binding.JaxbGroupBinding Maven / Gradle / Ivy
Show all versions of KeePassJava2-jaxb Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.8-b130911.1802
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2023.05.17 at 11:23:19 AM BST
//
package org.linguafranca.pwdb.kdbx.jaxb.binding;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.linguafranca.pwdb.kdbx.jaxb.base.AbstractJaxbParentedBinding;
/**
* Java class for Group element declaration.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <element name="Group">
* <complexType>
* <complexContent>
* <extension base="{}Parented">
* <sequence>
* <element name="UUID" type="{}uuid"/>
* <element name="Name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Notes" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="IconID" type="{}iconId"/>
* <element name="CustomIconUUID" type="{}customIconUuidRef" minOccurs="0"/>
* <element ref="{}Times"/>
* <element name="IsExpanded" type="{}keepassBoolean"/>
* <element name="DefaultAutoTypeSequence" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="EnableAutoType" type="{}keepassBoolean"/>
* <element name="EnableSearching" type="{}keepassBoolean"/>
* <element name="LastTopVisibleEntry" type="{}uuidRef"/>
* <element ref="{}Entry" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{}Group" maxOccurs="unbounded" minOccurs="0"/>
* <element name="CustomData" type="{}customData" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
* </element>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"uuid",
"name",
"notes",
"iconID",
"customIconUUID",
"times",
"isExpanded",
"defaultAutoTypeSequence",
"enableAutoType",
"enableSearching",
"lastTopVisibleEntry",
"entry",
"group",
"customData"
})
@XmlRootElement(name = "Group")
public class JaxbGroupBinding
extends AbstractJaxbParentedBinding
{
@XmlElement(name = "UUID", required = true, type = String.class)
@XmlJavaTypeAdapter(Adapter3 .class)
@XmlSchemaType(name = "base64Binary")
protected UUID uuid;
@XmlElement(name = "Name", required = true)
protected String name;
@XmlElement(name = "Notes", required = true)
protected String notes;
@XmlElement(name = "IconID")
protected int iconID;
@XmlElement(name = "CustomIconUUID", type = String.class)
@XmlJavaTypeAdapter(Adapter3 .class)
@XmlSchemaType(name = "base64Binary")
protected UUID customIconUUID;
@XmlElement(name = "Times", required = true)
protected Times times;
@XmlElement(name = "IsExpanded", required = true, type = String.class)
@XmlJavaTypeAdapter(Adapter2 .class)
protected Boolean isExpanded;
@XmlElement(name = "DefaultAutoTypeSequence", required = true)
protected String defaultAutoTypeSequence;
@XmlElement(name = "EnableAutoType", required = true, type = String.class)
@XmlJavaTypeAdapter(Adapter2 .class)
protected Boolean enableAutoType;
@XmlElement(name = "EnableSearching", required = true, type = String.class)
@XmlJavaTypeAdapter(Adapter2 .class)
protected Boolean enableSearching;
@XmlElement(name = "LastTopVisibleEntry", required = true, type = String.class)
@XmlJavaTypeAdapter(Adapter3 .class)
@XmlSchemaType(name = "base64Binary")
protected UUID lastTopVisibleEntry;
@XmlElement(name = "Entry")
protected List entry;
@XmlElement(name = "Group")
protected List group;
@XmlElement(name = "CustomData")
protected CustomData customData;
/**
* Gets the value of the uuid property.
*
* @return
* possible object is
* {@link String }
*
*/
public UUID getUUID() {
return uuid;
}
/**
* Sets the value of the uuid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUUID(UUID value) {
this.uuid = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the notes property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNotes() {
return notes;
}
/**
* Sets the value of the notes property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNotes(String value) {
this.notes = value;
}
/**
* Gets the value of the iconID property.
*
*/
public int getIconID() {
return iconID;
}
/**
* Sets the value of the iconID property.
*
*/
public void setIconID(int value) {
this.iconID = value;
}
/**
* Gets the value of the customIconUUID property.
*
* @return
* possible object is
* {@link String }
*
*/
public UUID getCustomIconUUID() {
return customIconUUID;
}
/**
* Sets the value of the customIconUUID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCustomIconUUID(UUID value) {
this.customIconUUID = value;
}
/**
* Gets the value of the times property.
*
* @return
* possible object is
* {@link Times }
*
*/
public Times getTimes() {
return times;
}
/**
* Sets the value of the times property.
*
* @param value
* allowed object is
* {@link Times }
*
*/
public void setTimes(Times value) {
this.times = value;
}
/**
* Gets the value of the isExpanded property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean getIsExpanded() {
return isExpanded;
}
/**
* Sets the value of the isExpanded property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIsExpanded(Boolean value) {
this.isExpanded = value;
}
/**
* Gets the value of the defaultAutoTypeSequence property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDefaultAutoTypeSequence() {
return defaultAutoTypeSequence;
}
/**
* Sets the value of the defaultAutoTypeSequence property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDefaultAutoTypeSequence(String value) {
this.defaultAutoTypeSequence = value;
}
/**
* Gets the value of the enableAutoType property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean getEnableAutoType() {
return enableAutoType;
}
/**
* Sets the value of the enableAutoType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEnableAutoType(Boolean value) {
this.enableAutoType = value;
}
/**
* Gets the value of the enableSearching property.
*
* @return
* possible object is
* {@link String }
*
*/
public Boolean getEnableSearching() {
return enableSearching;
}
/**
* Sets the value of the enableSearching property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEnableSearching(Boolean value) {
this.enableSearching = value;
}
/**
* Gets the value of the lastTopVisibleEntry property.
*
* @return
* possible object is
* {@link String }
*
*/
public UUID getLastTopVisibleEntry() {
return lastTopVisibleEntry;
}
/**
* Sets the value of the lastTopVisibleEntry property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLastTopVisibleEntry(UUID value) {
this.lastTopVisibleEntry = value;
}
/**
* Gets the value of the entry property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the entry property.
*
*
* For example, to add a new item, do as follows:
*
* getEntry().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link JaxbEntryBinding }
*
*
*/
public List getEntry() {
if (entry == null) {
entry = new ArrayList();
}
return this.entry;
}
/**
* Gets the value of the group property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the group property.
*
*
* For example, to add a new item, do as follows:
*
* getGroup().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link JaxbGroupBinding }
*
*
*/
public List getGroup() {
if (group == null) {
group = new ArrayList();
}
return this.group;
}
/**
* Gets the value of the customData property.
*
* @return
* possible object is
* {@link CustomData }
*
*/
public CustomData getCustomData() {
return customData;
}
/**
* Sets the value of the customData property.
*
* @param value
* allowed object is
* {@link CustomData }
*
*/
public void setCustomData(CustomData value) {
this.customData = value;
}
}