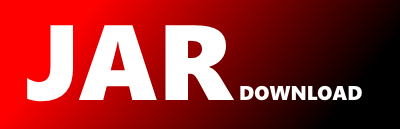
org.linuxprobe.spring.boot.start.MybatisProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-universal-crud-spring-boot-starter Show documentation
Show all versions of mybatis-universal-crud-spring-boot-starter Show documentation
mybatis-universal-crud-spring-boot-starter
package org.linuxprobe.spring.boot.start;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Properties;
import org.apache.ibatis.session.ExecutorType;
import org.linuxprobe.crud.mybatis.session.UniversalCrudConfiguration;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.context.properties.NestedConfigurationProperty;
import org.springframework.core.io.Resource;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import org.springframework.core.io.support.ResourcePatternResolver;
@ConfigurationProperties(prefix = "mybatis")
public class MybatisProperties {
/** 扫描包路径,如果需要扫描多个,中间使用半角的逗号隔开 */
private String[] mapperLocations;
/** mybatis配置文件路径 */
private String configLocation;
/** universalCrudScan扫描路径,如果需要扫描多个,中间使用半角的逗号隔开 */
private String universalCrudScan;
/**
* Packages to search type aliases. (Package delimiters are ",; \t\n")
*/
private String typeAliasesPackage;
/**
* Packages to search for type handlers. (Package delimiters are ",; \t\n")
*/
private String typeHandlersPackage;
/**
* Indicates whether perform presence check of the MyBatis xml config file.
*/
private boolean checkConfigLocation = false;
/**
* Execution mode for {@link org.mybatis.spring.SqlSessionTemplate}.
*/
private ExecutorType executorType;
/**
* Externalized properties for MyBatis configuration.
*/
private Properties configurationProperties;
/**
* A Configuration object for customize default settings. If
* {@link #configLocation} is specified, this property is not used.
*/
@NestedConfigurationProperty
private UniversalCrudConfiguration configuration;
public String[] getMapperLocations() {
return mapperLocations;
}
public void setMapperLocations(String[] mapperLocations) {
this.mapperLocations = mapperLocations;
}
public String getConfigLocation() {
return configLocation;
}
public void setConfigLocation(String configLocation) {
this.configLocation = configLocation;
}
public String getUniversalCrudScan() {
return universalCrudScan;
}
public void setUniversalCrudScan(String universalCrudScan) {
this.universalCrudScan = universalCrudScan;
}
public String getTypeAliasesPackage() {
return typeAliasesPackage;
}
public void setTypeAliasesPackage(String typeAliasesPackage) {
this.typeAliasesPackage = typeAliasesPackage;
}
public String getTypeHandlersPackage() {
return typeHandlersPackage;
}
public void setTypeHandlersPackage(String typeHandlersPackage) {
this.typeHandlersPackage = typeHandlersPackage;
}
public boolean isCheckConfigLocation() {
return checkConfigLocation;
}
public void setCheckConfigLocation(boolean checkConfigLocation) {
this.checkConfigLocation = checkConfigLocation;
}
public ExecutorType getExecutorType() {
return executorType;
}
public void setExecutorType(ExecutorType executorType) {
this.executorType = executorType;
}
public Properties getConfigurationProperties() {
return configurationProperties;
}
public void setConfigurationProperties(Properties configurationProperties) {
this.configurationProperties = configurationProperties;
}
public UniversalCrudConfiguration getConfiguration() {
return configuration;
}
public void setConfiguration(UniversalCrudConfiguration configuration) {
this.configuration = configuration;
}
public Resource[] resolveMapperLocations() {
ResourcePatternResolver resourceResolver = new PathMatchingResourcePatternResolver();
List resources = new ArrayList();
if (this.mapperLocations != null) {
for (String mapperLocation : this.mapperLocations) {
try {
Resource[] mappers = resourceResolver.getResources(mapperLocation);
resources.addAll(Arrays.asList(mappers));
} catch (IOException e) {
// ignore
}
}
}
return resources.toArray(new Resource[resources.size()]);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy