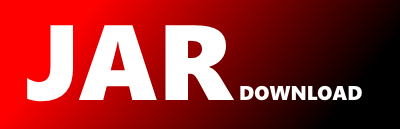
liquibase.statement.core.AddColumnStatement Maven / Gradle / Ivy
package liquibase.statement.core;
import liquibase.statement.*;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
public class AddColumnStatement implements SqlStatement {
private String schemaName;
private String tableName;
private String columnName;
private String columnType;
private Object defaultValue;
private Set constraints = new HashSet();
public AddColumnStatement(String schemaName, String tableName, String columnName, String columnType, Object defaultValue, ColumnConstraint... constraints) {
this.schemaName = schemaName;
this.tableName = tableName;
this.columnName = columnName;
this.columnType = columnType;
this.defaultValue = defaultValue;
if (constraints != null) {
this.constraints.addAll(Arrays.asList(constraints));
}
}
public String getSchemaName() {
return schemaName;
}
public String getTableName() {
return tableName;
}
public String getColumnName() {
return columnName;
}
public String getColumnType() {
return columnType;
}
public Set getConstraints() {
return constraints;
}
public boolean isAutoIncrement() {
for (ColumnConstraint constraint : getConstraints()) {
if (constraint instanceof AutoIncrementConstraint) {
return true;
}
}
return false;
}
public boolean isPrimaryKey() {
for (ColumnConstraint constraint : getConstraints()) {
if (constraint instanceof PrimaryKeyConstraint) {
return true;
}
}
return false;
}
public boolean isNullable() {
if (isPrimaryKey()) {
return false;
}
for (ColumnConstraint constraint : getConstraints()) {
if (constraint instanceof NotNullConstraint) {
return false;
}
}
return true;
}
public boolean isUnique() {
for (ColumnConstraint constraint : getConstraints()) {
if (constraint instanceof UniqueConstraint) {
return true;
}
}
return false;
}
public Object getDefaultValue() {
return defaultValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy