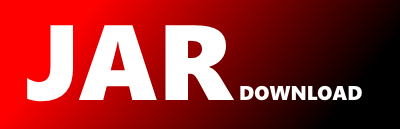
liquibase.util.StringUtils Maven / Gradle / Ivy
package liquibase.util;
import liquibase.database.Database;
import java.util.*;
import java.util.regex.Pattern;
/**
* Various utility methods for working with strings.
*/
public class StringUtils {
private static final Pattern commentPattern = Pattern.compile("/\\*.*?\\*/", Pattern.DOTALL);
private static final Pattern upperCasePattern = Pattern.compile(".*[A-Z].*");
private static final Pattern lowerCasePattern = Pattern.compile(".*[a-z].*");
/**
* This pattern is used to recognize end-of-line ANSI style comments at the end of lines of SQL. -- like this
* and strip them out. We might need to watch the case of new space--like this
* and the case of having a space -- like this.
*/
private static final Pattern dashPattern = Pattern.compile("\\-\\-.*$", Pattern.MULTILINE);
public static String trimToEmpty(String string) {
if (string == null) {
return "";
}
return string.trim();
}
public static String trimToNull(String string) {
if (string == null) {
return null;
}
String returnString = string.trim();
if (returnString.length() == 0) {
return null;
} else {
return returnString;
}
}
/**
* Removes any comments from multiple line SQL using {@link #stripComments(String)}
* and then extracts each individual statement using {@link #splitSQL(String, String)}.
*
* @param multiLineSQL A String containing all the SQL statements
* @param stripComments If true then comments will be stripped, if false then they will be left in the code
*/
public static String[] processMutliLineSQL(String multiLineSQL,boolean stripComments, boolean splitStatements, String endDelimiter) {
String stripped = stripComments ? stripComments(multiLineSQL) : multiLineSQL;
if (splitStatements) {
return splitSQL(stripped, endDelimiter);
} else {
return new String[]{stripped};
}
}
/**
* Splits a (possible) multi-line SQL statement along ;'s and "go"'s.
*/
public static String[] splitSQL(String multiLineSQL, String endDelimiter) {
if (endDelimiter == null) {
endDelimiter = ";\\s*\\n|;$|\\n[gG][oO]\\s*\\n|\\n[Gg][oO]\\s*$";
} else {
if (endDelimiter.equalsIgnoreCase("go")) {
endDelimiter = "\\n[gG][oO]\\s*\\n|\\n[Gg][oO]\\s*$";
}
}
String[] initialSplit = multiLineSQL.split(endDelimiter);
List strings = new ArrayList();
for (String anInitialSplit : initialSplit) {
String singleLineSQL = anInitialSplit.trim();
if (singleLineSQL.length() > 0) {
strings.add(singleLineSQL);
}
}
return strings.toArray(new String[strings.size()]);
}
/**
* Searches through a String which contains SQL code and strips out
* any comments that are between \/**\/ or anything that matches
* SP--SP\n (to support the ANSI standard commenting of --
* at the end of a line).
*
* @return The String without the comments in
*/
public static String stripComments(String multiLineSQL) {
String strippedSingleLines = Pattern.compile("\\s*\\-\\-.*\\n").matcher(multiLineSQL).replaceAll("\n");
strippedSingleLines = Pattern.compile("\\s*\\-\\-.*$").matcher(strippedSingleLines).replaceAll("\n");
return Pattern.compile("/\\*.*?\\*/", Pattern.DOTALL).matcher(strippedSingleLines).replaceAll("").trim();
}
public static String join(Object[] array, String delimiter, StringUtilsFormatter formatter) {
if (array == null) {
return null;
}
return join(Arrays.asList(array), delimiter, formatter);
}
public static String join(String[] array, String delimiter) {
return join(Arrays.asList(array), delimiter);
}
public static String join(Collection collection, String delimiter) {
return join(collection, delimiter, new ToStringFormatter());
}
public static String join(Collection collection, String delimiter, StringUtilsFormatter formatter) {
if (collection == null) {
return null;
}
if (collection.size() == 0) {
return "";
}
StringBuffer buffer = new StringBuffer();
for (Object val : collection) {
buffer.append(formatter.toString(val)).append(delimiter);
}
String returnString = buffer.toString();
return returnString.substring(0, returnString.length()-delimiter.length());
}
public static String join(Collection collection, String delimiter, StringUtilsFormatter formatter, boolean sorted) {
if (sorted) {
TreeSet sortedSet = new TreeSet();
for (Object obj : collection) {
sortedSet.add(formatter.toString(obj));
}
return join(sortedSet, delimiter);
}
return join(collection, delimiter, formatter);
}
public static String join(Collection collection, String delimiter, boolean sorted) {
if (sorted) {
return join(new TreeSet(collection), delimiter);
} else {
return join(collection, delimiter);
}
}
public static String join(Map map, String delimiter) {
return join(map, delimiter, new ToStringFormatter());
}
public static String join(Map map, String delimiter, StringUtilsFormatter formatter) {
List list = new ArrayList();
for (Map.Entry entry : (Set) map.entrySet()) {
list.add(entry.getKey().toString()+"="+formatter.toString(entry.getValue()));
}
return join(list, delimiter);
}
public static List splitAndTrim(String s, String regex) {
if (s == null) {
return null;
}
List returnList = new ArrayList();
for (String string : s.split(regex)) {
returnList.add(string.trim());
}
return returnList;
}
public static String repeat(String string, int times) {
String returnString = "";
for (int i=0; i 0x7F) {
out.append("");
out.append(Integer.toString(c, 10));
out.append(';');
} else {
out.append(c);
}
}
return out.toString();
}
public static String pad(String value, int length) {
value = StringUtils.trimToEmpty(value);
if (value.length() >= length) {
return value;
}
return value + StringUtils.repeat(" ", length - value.length());
}
public static interface StringUtilsFormatter {
public String toString(Type obj);
}
public static class ToStringFormatter implements StringUtilsFormatter {
@Override
public String toString(Object obj) {
if (obj == null) {
return null;
}
return obj.toString();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy