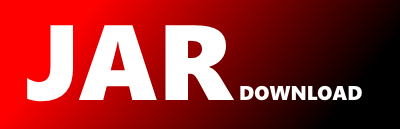
org.liquibase.maven.plugins.AbstractLiquibaseMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of liquibase-maven-plugin Show documentation
Show all versions of liquibase-maven-plugin Show documentation
A Maven plugin wraps up some of the functionality of Liquibase
package org.liquibase.maven.plugins;
import liquibase.Liquibase;
import liquibase.configuration.LiquibaseConfiguration;
import liquibase.configuration.GlobalConfiguration;
import liquibase.database.Database;
import liquibase.exception.DatabaseException;
import liquibase.exception.LiquibaseException;
import liquibase.exception.UnexpectedLiquibaseException;
import liquibase.integration.commandline.CommandLineUtils;
import liquibase.logging.LogFactory;
import liquibase.resource.CompositeResourceAccessor;
import liquibase.resource.FileSystemResourceAccessor;
import liquibase.resource.ResourceAccessor;
import liquibase.util.StreamUtil;
import liquibase.util.ui.UIFactory;
import org.apache.maven.artifact.manager.WagonManager;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import org.apache.maven.wagon.authentication.AuthenticationInfo;
import java.io.*;
import java.lang.reflect.Field;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.*;
/**
* A base class for providing Liquibase {@link liquibase.Liquibase} functionality.
*
* @author Peter Murray
* @author Florent Biville
*
* Test dependency is used because when you run a goal outside the build phases you want to have the same dependencies
* that it would had if it was ran inside test phase
* @requiresDependencyResolution test
*/
public abstract class AbstractLiquibaseMojo extends AbstractMojo {
/**
* Suffix for fields that are representing a default value for a another field.
*/
private static final String DEFAULT_FIELD_SUFFIX = "Default";
/**
* The fully qualified name of the driver class to use to connect to the database.
*
* @parameter expression="${liquibase.driver}"
*/
protected String driver;
/**
* The Database URL to connect to for executing Liquibase.
*
* @parameter expression="${liquibase.url}"
*/
protected String url;
/**
The Maven Wagon manager to use when obtaining server authentication details.
@component role="org.apache.maven.artifact.manager.WagonManager"
@required
@readonly
*/
protected WagonManager wagonManager;
/**
* The server id in settings.xml to use when authenticating with.
*
* @parameter expression="${liquibase.server}"
*/
private String server;
/**
* The database username to use to connect to the specified database.
*
* @parameter expression="${liquibase.username}"
*/
protected String username;
/**
* The database password to use to connect to the specified database.
*
* @parameter expression="${liquibase.password}"
*/
protected String password;
/**
* Use an empty string as the password for the database connection. This should not be
* used along side the {@link #password} setting.
*
* @parameter expression="${liquibase.emptyPassword}" default-value="false"
* @deprecated Use an empty or null value for the password instead.
*/
protected boolean emptyPassword;
/**
* Whether to ignore the schema name.
*
* @parameter expression="${liquibase.outputDefaultSchema}"
*/
protected boolean outputDefaultSchema;
/**
* Whether to ignore the catalog/database name.
*
* @parameter expression="${liquibase.outputDefaultCatalog}"
*/
protected boolean outputDefaultCatalog;
/**
* The default catalog name to use the for database connection.
*
* @parameter expression="${liquibase.defaultCatalogName}"
*/
protected String defaultCatalogName;
/**
* The default schema name to use the for database connection.
*
* @parameter expression="${liquibase.defaultSchemaName}"
*/
protected String defaultSchemaName;
/**
* The class to use as the database object.
*
* @parameter expression="${liquibase.databaseClass}"
*/
protected String databaseClass;
/**
* The class to use as the property provider (must be a java.util.Properties implementation).
*
* @parameter expression="${liquibase.propertyProviderClass}"
*/
protected String propertyProviderClass;
/**
* Controls the prompting of users as to whether or not they really want to run the
* changes on a database that is not local to the machine that the user is current
* executing the plugin on.
*
* @parameter expression="${liquibase.promptOnNonLocalDatabase}" default-value="true"
*/
protected boolean promptOnNonLocalDatabase;
/**
* Allows for the maven project artifact to be included in the class loader for
* obtaining the Liquibase property and DatabaseChangeLog files.
*
* @parameter expression="${liquibase.includeArtifact}" default-value="true"
*/
protected boolean includeArtifact;
/**
* Allows for the maven test output directory to be included in the class loader for
* obtaining the Liquibase property and DatabaseChangeLog files.
*
* @parameter expression="${liquibase.includeTestOutputDirectory}" default-value="true"
*/
protected boolean includeTestOutputDirectory;
/**
* Controls the verbosity of the output from invoking the plugin.
*
* @parameter expression="${liquibase.verbose}" default-value="false"
* @description Controls the verbosity of the plugin when executing
*/
protected boolean verbose;
/**
* Controls the level of logging from Liquibase when executing. The value can be
* "debug", "info", "warning", "severe", or "off". The value is
* case insensitive.
*
* @parameter expression="${liquibase.logging}" default-value="INFO"
* @description Controls the verbosity of the plugin when executing
*/
protected String logging;
/**
* The Liquibase properties file used to configure the Liquibase {@link
* liquibase.Liquibase}.
*
* @parameter expression="${liquibase.propertyFile}"
*/
protected String propertyFile;
/**
* Flag allowing for the Liquibase properties file to override any settings provided in
* the Maven plugin configuration. By default if a property is explicity specified it is
* not overridden if it also appears in the properties file.
*
* @parameter expression="${liquibase.propertyFileWillOverride}" default-value="false"
*/
protected boolean propertyFileWillOverride;
/**
* Flag for forcing the checksums to be cleared from teh DatabaseChangeLog table.
*
* @parameter expression="${liquibase.clearCheckSums}" default-value="false"
*/
protected boolean clearCheckSums;
/**
* List of system properties to pass to the database.
*
* @parameter
*/
protected Properties systemProperties;
/**
* The Maven project that plugin is running under.
*
* @parameter expression="${project}"
* @required
* @readonly
*/
protected MavenProject project;
/**
* The {@link Liquibase} object used modify the database.
*/
private Liquibase liquibase;
/**
* Array to put a expression variable to maven plugin.
*
* @parameter
*/
private Properties expressionVars;
/**
* Set this to 'false' to skip running liquibase. Its use is NOT RECOMMENDED, but quite
* convenient on occasion.
*
* @parameter expression="${liquibase.skip}"
*/
protected boolean skip = false;
/**
* Array to put a expression variable to maven plugin.
*
* @parameter
*/
private Map expressionVariables;
/**
* Flag to set the character encoding of the output file produced by Liquibase during the updateSQL phase.
*
* @parameter expression="${liquibase.outputFileEncoding}"
*/
protected String outputFileEncoding;
/**
* Schema against which Liquibase changelog tables will be created.
*
* @parameter expression="${liquibase.changelogCatalogName}"
*/
protected String changelogCatalogName;
/**
* Schema against which Liquibase changelog tables will be created.
*
* @parameter expression="${liquibase.changelogSchemaName}"
*/
protected String changelogSchemaName;
/**
* Location of a properties file containing JDBC connection properties for use by the driver.
*
* @parameter
*/
private File driverPropertiesFile;
/**
* Table name to use for the databasechangelog.
*
* @parameter expression="${liquibase.databaseChangeLogTableName}"
*/
protected String databaseChangeLogTableName;
/**
* Table name to use for the databasechangelog.
*
* @parameter expression="${liquibase.databaseChangeLogLockTableName}"
*/
protected String databaseChangeLogLockTableName;
protected Writer getOutputWriter(final File outputFile) throws IOException {
if (outputFileEncoding==null) {
getLog().info("Char encoding not set! The created file will be system dependent!");
return new FileWriter(outputFile);
}
getLog().debug("Writing output file with [" + outputFileEncoding + "] file encoding.");
return new BufferedWriter(new OutputStreamWriter( new FileOutputStream(outputFile), outputFileEncoding));
}
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
getLog().info(MavenUtils.LOG_SEPARATOR);
if (server != null) {
AuthenticationInfo info = wagonManager.getAuthenticationInfo(server);
if (info != null) {
username = info.getUserName();
password = info.getPassword();
}
}
processSystemProperties();
LiquibaseConfiguration liquibaseConfiguration = LiquibaseConfiguration.getInstance();
if (!liquibaseConfiguration.getConfiguration(GlobalConfiguration.class).getShouldRun()) {
getLog().info("Liquibase did not run because " + liquibaseConfiguration.describeValueLookupLogic(GlobalConfiguration.class, GlobalConfiguration.SHOULD_RUN) + " was set to false");
return;
}
if (skip) {
getLog().warn("Liquibase skipped due to maven configuration");
return;
}
ClassLoader artifactClassLoader = getMavenArtifactClassLoader();
ResourceAccessor fileOpener = getFileOpener(artifactClassLoader);
configureFieldsAndValues(fileOpener);
LogFactory.getInstance().setDefaultLoggingLevel(logging);
// Displays the settings for the Mojo depending of verbosity mode.
displayMojoSettings();
// Check that all the parameters that must be specified have been by the user.
checkRequiredParametersAreSpecified();
Database database = null;
try {
String dbPassword = emptyPassword || password == null ? "" : password;
String driverPropsFile = (driverPropertiesFile == null) ? null : driverPropertiesFile.getAbsolutePath();
database = CommandLineUtils.createDatabaseObject(artifactClassLoader,
url,
username,
dbPassword,
driver,
defaultCatalogName,
defaultSchemaName,
outputDefaultCatalog,
outputDefaultSchema,
databaseClass,
driverPropsFile,
propertyProviderClass,
changelogCatalogName,
changelogSchemaName,
databaseChangeLogTableName,
databaseChangeLogLockTableName);
liquibase = createLiquibase(fileOpener, database);
getLog().debug("expressionVars = " + String.valueOf(expressionVars));
if (expressionVars != null) {
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy