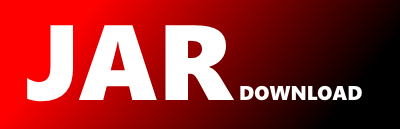
org.loadui.testfx.Assertions Maven / Gradle / Ivy
The newest version!
package org.loadui.testfx;
import com.google.common.base.Predicate;
import javafx.scene.Node;
import org.hamcrest.Matcher;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.loadui.testfx.GuiTest.find;
import static org.loadui.testfx.utils.FXTestUtils.releaseButtons;
public class Assertions
{
@SuppressWarnings( "unchecked" )
public static void assertNodeExists( Matcher> matcher )
{
find((Matcher
© 2015 - 2025 Weber Informatics LLC | Privacy Policy