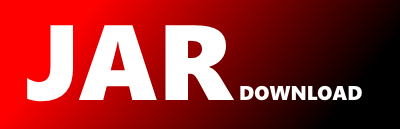
org.lwapp.commons.file.ManifestFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwapp-commons Show documentation
Show all versions of lwapp-commons Show documentation
Lwapp commons is a utility jar file with most common features needed in daily java programming.
The newest version!
package org.lwapp.commons.file;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.Serializable;
import java.util.Collections;
import java.util.Date;
import java.util.InvalidPropertiesFormatException;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import org.apache.commons.lang3.time.DateFormatUtils;
import org.lwapp.commons.config.DateFormats;
public final class ManifestFile {
public final Properties properties = new Properties();
public void addProperty(final Manifest mf, final Serializable value) {
if (mf == null) {
throw new NullPointerException("Manifest is mandatory.");
}
if (value != null) {
properties.setProperty(mf.property, value.toString());
}
}
public String getProperty(final Manifest mf) {
return properties.getProperty(mf.property);
}
public void load(final InputStream in) throws InvalidPropertiesFormatException, IOException {
properties.load(in);
}
@Override
public String toString() {
return properties.toString();
}
public static ManifestFile getInstance() {
return new ManifestFile();
}
public ManifestFile setSha512(final String sha512Hash) {
addProperty(Manifest.SHA512, sha512Hash);
return this;
}
public ManifestFile setManifestVersion(final String version) {
addProperty(Manifest.MANIFEST_VERSION, version);
return this;
}
public ManifestFile setDataFileName(final File dataFile) {
addProperty(Manifest.DATA_FILENAME, dataFile.getName());
return this;
}
public ManifestFile setCompressed(final boolean compressed) {
addProperty(Manifest.IS_COMPRESSED, compressed);
return this;
}
public ManifestFile setEncrypted(final boolean encrypted) {
addProperty(Manifest.IS_ENCRYPTED, encrypted);
return this;
}
public ManifestFile setTotalBytes(final int length) {
addProperty(Manifest.TOTAL_BYTES, length);
return this;
}
public ManifestFile setApplicationSystemIdentity(final String applicationId) {
addProperty(Manifest.APPLICATION_IDENTITY, applicationId);
return this;
}
public ManifestFile setTotalBytesWritten(final int length) {
addProperty(Manifest.TOTAL_BYTESWRITTEN, length);
return this;
}
public ManifestFile setCompressedBZIP2FileName(final File compressedBZIP2File) {
addProperty(Manifest.COMPRESSED_BZIP2_FILENAME, compressedBZIP2File.getName());
return this;
}
public ManifestFile setChecksum(final long checksum) {
addProperty(Manifest.CHECKSUM, checksum);
return this;
}
public ManifestFile setManifestFileName(final File mfFile) {
addProperty(Manifest.MANIFEST_FILENAME, mfFile.getName());
return this;
}
public ManifestFile setInboxFolder(final File folder) {
addProperty(Manifest.FOLDER_NAME, folder.getAbsolutePath());
return this;
}
public ManifestFile setCreatedDate(final Date date) {
addProperty(Manifest.CREATED_DATE, DateFormatUtils.format(date, DateFormats.YYYY_MM_DD_HMS));
return this;
}
public ManifestFile setProperites(Map propertyMap) {
if (propertyMap == null) {
propertyMap = Collections.emptyMap();
}
for (final Entry entry : propertyMap.entrySet()) {
if (properties.containsKey(entry.getKey())) {
throw new RuntimeException("Property already set" + entry.getValue());
}
}
properties.putAll(propertyMap);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy