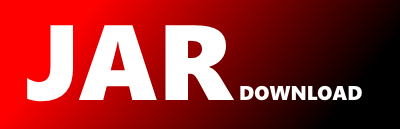
org.lwapp.commons.utils.MapUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwapp-commons Show documentation
Show all versions of lwapp-commons Show documentation
Lwapp commons is a utility jar file with most common features needed in daily java programming.
The newest version!
package org.lwapp.commons.utils;
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
public class MapUtil {
private static final String DELIMITER = ";";
public static String mapToString(final Map map) {
if (map == null) {
return StringUtils.EMPTY;
}
final StringBuilder stringBuilder = new StringBuilder();
for (final String key : map.keySet()) {
if (stringBuilder.length() > 0) {
stringBuilder.append(DELIMITER);
}
final String value = map.get(key);
try {
stringBuilder.append(key != null ? URLEncoder.encode(key, "UTF-8") : "");
stringBuilder.append("=");
stringBuilder.append(value != null ? URLEncoder.encode(value, "UTF-8") : "");
} catch (final UnsupportedEncodingException e) {
throw new RuntimeException("This method requires UTF-8 encoding support", e);
}
}
return stringBuilder.toString();
}
public static Map stringToMap(final String input) {
if (StringUtils.isBlank(input)) {
return Collections.emptyMap();
}
final Map map = new HashMap();
final String[] nameValuePairs = input.split(DELIMITER);
for (final String nameValuePair : nameValuePairs) {
final String[] nameValue = nameValuePair.split("=");
try {
map.put(URLDecoder.decode(nameValue[0], "UTF-8"), nameValue.length > 1 ? URLDecoder.decode(nameValue[1], "UTF-8") : "");
} catch (final UnsupportedEncodingException e) {
throw new RuntimeException("This method requires UTF-8 encoding support", e);
}
}
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy