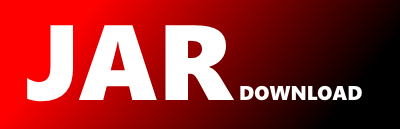
org.lwapp.notification.config.ApplicationServerConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwapp-notification Show documentation
Show all versions of lwapp-notification Show documentation
Lwapp notification is a utility jar file with most common notification features(email, sms, twitter) needed in daily java programming.
The newest version!
package org.lwapp.notification.config;
import javax.inject.Inject;
import javax.inject.Singleton;
import org.lwapp.configclient.client.ConfigurationServiceClient;
import org.lwapp.jms.common.JmsDestination;
import com.google.common.base.Preconditions;
@Singleton
public class ApplicationServerConfig {
@Inject
private ConfigurationServiceClient configurationServiceClient;
public int getValueAsInt(final LwappConfigurations config) {
return Preconditions.checkNotNull(configurationServiceClient.getInt(config), "Please provide mandatory config property: " + config);
}
public String getValue(final LwappConfigurations config) {
return configurationServiceClient.getString(config);
}
public String getSystemEmailAddress() {
return getValue(LwappConfigurations.SYSTEM_EMAIL_ADDRESS);
}
public int getMaxRetryEmailAttempts() {
return configurationServiceClient.getInt(LwappConfigurations.MAX_EMAIL_ATTEMPTS);
}
public int getMaxRetrySMSAttempts() {
return configurationServiceClient.getInt(LwappConfigurations.MAX_SMS_ATTEMPTS);
}
public JmsDestination getEmailIncomingJmsQueue() {
final String incomingQueue = getValue(LwappConfigurations.EMAIL_INCOMING_QUEUE);
return new JmsDestination(getJmsUrl(), incomingQueue, incomingQueue);
}
public JmsDestination getEmailErrorJmsQueue() {
final String errorQueue = getValue(LwappConfigurations.EMAIL_ERROR_QUEUE);
return new JmsDestination(getJmsUrl(), errorQueue, errorQueue);
}
public JmsDestination getSmsIncomingJmsQueue() {
final String incomingQueue = getValue(LwappConfigurations.SMS_INCOMING_QUEUE);
return new JmsDestination(getJmsUrl(), incomingQueue, incomingQueue);
}
public JmsDestination getSmsErrorJmsQueue() {
final String errorQueue = getValue(LwappConfigurations.SMS_ERROR_QUEUE);
return new JmsDestination(getJmsUrl(), errorQueue, errorQueue);
}
public JmsDestination getTwitterIncomingJmsQueue() {
final String incomingQueue = getValue(LwappConfigurations.TWITTER_INCOMING_QUEUE);
return new JmsDestination(getJmsUrl(), incomingQueue, incomingQueue);
}
public JmsDestination getTwitterErrorJmsQueue() {
final String errorQueue = getValue(LwappConfigurations.TWITTER_ERROR_QUEUE);
return new JmsDestination(getJmsUrl(), errorQueue, errorQueue);
}
public String getJmsUrl() {
return configurationServiceClient.getString(org.lwapp.jms.config.LwappConfigurations.JMS_URL);
}
public String getTechinalSupportEmailAddress() {
return getValue(LwappConfigurations.STSTEM_TECHNICAL_SUPPORT_EMAIL_ADDRESS);
}
public boolean isNotificationsEnabled() {
return configurationServiceClient.getBoolean(LwappConfigurations.ENABLE_NOTIFICATIONS);
}
public boolean isSmsNotificationsEnabled() {
return configurationServiceClient.getBoolean(LwappConfigurations.ENABLE_SMS_NOTIFICATIONS);
}
public boolean isEmailNotificationsEnabled() {
return configurationServiceClient.getBoolean(LwappConfigurations.ENABLE_EMAIL_NOTIFICATIONS);
}
public boolean isTwitterNotificationsEnabled() {
return configurationServiceClient.getBoolean(LwappConfigurations.ENABLE_TWITTER_NOTIFICATIONS);
}
public String getSmtpPropertiesFilePath() {
return getValue(LwappConfigurations.STSTEM_SMTP_PROPERTIES_FILE_PATH);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy