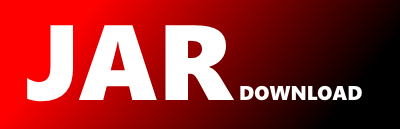
org.lwjgl.system.libc.LibCErrno Maven / Gradle / Ivy
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.system.libc;
import org.lwjgl.system.*;
/** Native bindings to errno.h. */
public class LibCErrno {
static { Library.initialize(); }
/**
* Standard error codes.
*
* Enum values:
*
*
* - {@link #EPERM EPERM} - Operation not permitted
* - {@link #ENOENT ENOENT} - No such file or directory
* - {@link #ESRCH ESRCH} - No such process
* - {@link #EINTR EINTR} - Interrupted function
* - {@link #EIO EIO} - I/O error
* - {@link #ENXIO ENXIO} - No such device or address
* - {@link #E2BIG E2BIG} - Argument list too long
* - {@link #ENOEXEC ENOEXEC} - Exec format error
* - {@link #EBADF EBADF} - Bad file number
* - {@link #ECHILD ECHILD} - No spawned processes
* - {@link #EAGAIN EAGAIN} - No more processes or not enough memory or maximum nesting level reached
* - {@link #ENOMEM ENOMEM} - Not enough memory
* - {@link #EACCES EACCES} - Permission denied
* - {@link #EFAULT EFAULT} - Bad address
* - {@link #EBUSY EBUSY} - Device or resource busy
* - {@link #EEXIST EEXIST} - File exists
* - {@link #EXDEV EXDEV} - Cross-device link
* - {@link #ENODEV ENODEV} - No such device
* - {@link #ENOTDIR ENOTDIR} - Not a directory
* - {@link #EISDIR EISDIR} - Is a directory
* - {@link #EINVAL EINVAL} - Invalid argument
* - {@link #ENFILE ENFILE} - Too many files open in system
* - {@link #EMFILE EMFILE} - Too many open files
* - {@link #ENOTTY ENOTTY} - Inappropriate I/O control operation
* - {@link #EFBIG EFBIG} - File too large
* - {@link #ENOSPC ENOSPC} - No space left on device
* - {@link #ESPIPE ESPIPE} - Invalid seek
* - {@link #EROFS EROFS} - Read-only file system
* - {@link #EMLINK EMLINK} - Too many links
* - {@link #EPIPE EPIPE} - Broken pipe
* - {@link #EDOM EDOM} - Math argument
* - {@link #ERANGE ERANGE} - Result too large
* - {@link #EDEADLK EDEADLK} - Resource deadlock would occur
* - {@link #EDEADLOCK EDEADLOCK} - Same as EDEADLK for compatibility with older Microsoft C versions
* - {@link #ENAMETOOLONG ENAMETOOLONG} - Filename too long
* - {@link #ENOLCK ENOLCK} - No locks available
* - {@link #ENOSYS ENOSYS} - Function not supported
* - {@link #ENOTEMPTY ENOTEMPTY} - Directory not empty
* - {@link #EILSEQ EILSEQ} - Illegal byte sequence
* - {@link #STRUNCATE STRUNCATE} - String was truncated
*
*/
public static final int
EPERM = 0x1,
ENOENT = 0x2,
ESRCH = 0x3,
EINTR = 0x4,
EIO = 0x5,
ENXIO = 0x6,
E2BIG = 0x7,
ENOEXEC = 0x8,
EBADF = 0x9,
ECHILD = 0xA,
EAGAIN = 0xB,
ENOMEM = 0xC,
EACCES = 0xD,
EFAULT = 0xE,
EBUSY = 0x10,
EEXIST = 0x11,
EXDEV = 0x12,
ENODEV = 0x13,
ENOTDIR = 0x14,
EISDIR = 0x15,
EINVAL = 0x16,
ENFILE = 0x17,
EMFILE = 0x18,
ENOTTY = 0x19,
EFBIG = 0x1B,
ENOSPC = 0x1C,
ESPIPE = 0x1D,
EROFS = 0x1E,
EMLINK = 0x1F,
EPIPE = 0x20,
EDOM = 0x21,
ERANGE = 0x22,
EDEADLK = 0x24,
EDEADLOCK = 0x24,
ENAMETOOLONG = 0x26,
ENOLCK = 0x27,
ENOSYS = 0x28,
ENOTEMPTY = 0x29,
EILSEQ = 0x2A,
STRUNCATE = 0x50;
protected LibCErrno() {
throw new UnsupportedOperationException();
}
// --- [ errno ] ---
/**
* Returns the integer variable {@code errno}, which is set by system calls and some library functions in the event of an error to indicate what went
* wrong. Its value is significant only when the return value of the call indicated an error (i.e., -1 from most system calls; -1 or {@code NULL} from most
* library functions); a function that succeeds is allowed to change errno.
*
* LWJGL note: This function cannot be used after another JNI call to a function, because the last error resets before that call returns. For this
* reason, LWJGL stores the last error in thread-local storage, you can use {@link #getErrno} to access it.
*/
public static native int errno();
// --- [ getErrno ] ---
/**
* Returns the integer variable {@code errno}, which is set by system calls and some library functions in the event of an error to indicate what went
* wrong. Its value is significant only when the return value of the call indicated an error (i.e., -1 from most system calls; -1 or {@code NULL} from most
* library functions); a function that succeeds is allowed to change errno.
*
* LWJGL note: This method has a meaningful value only after another LWJGL JNI call. It does not return {@code errno} from errno.h, but the
* thread-local error code stored by a previous JNI call.
*/
public static native int getErrno();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy