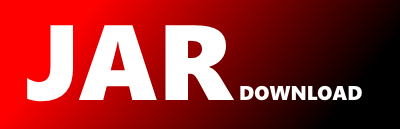
org.lwjgl.opengl.ARBDrawBuffers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwjgl-opengl Show documentation
Show all versions of lwjgl-opengl Show documentation
The most widely adopted 2D and 3D graphics API in the industry, bringing thousands of applications to a wide variety of computer platforms.
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.opengl;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* Native bindings to the ARB_draw_buffers extension.
*
* This extension extends {@link ARBFragmentProgram ARB_fragment_program} and {@link ARBFragmentShader ARB_fragment_shader} to allow multiple output colors, and provides a mechanism for
* directing those outputs to multiple color buffers.
*
* Requires {@link GL13 OpenGL 1.3}. Promoted to core in {@link GL20 OpenGL 2.0}.
*/
public class ARBDrawBuffers {
/** Accepted by the {@code pname} parameters of GetIntegerv, GetFloatv, and GetDoublev. */
public static final int
GL_MAX_DRAW_BUFFERS_ARB = 0x8824,
GL_DRAW_BUFFER0_ARB = 0x8825,
GL_DRAW_BUFFER1_ARB = 0x8826,
GL_DRAW_BUFFER2_ARB = 0x8827,
GL_DRAW_BUFFER3_ARB = 0x8828,
GL_DRAW_BUFFER4_ARB = 0x8829,
GL_DRAW_BUFFER5_ARB = 0x882A,
GL_DRAW_BUFFER6_ARB = 0x882B,
GL_DRAW_BUFFER7_ARB = 0x882C,
GL_DRAW_BUFFER8_ARB = 0x882D,
GL_DRAW_BUFFER9_ARB = 0x882E,
GL_DRAW_BUFFER10_ARB = 0x882F,
GL_DRAW_BUFFER11_ARB = 0x8830,
GL_DRAW_BUFFER12_ARB = 0x8831,
GL_DRAW_BUFFER13_ARB = 0x8832,
GL_DRAW_BUFFER14_ARB = 0x8833,
GL_DRAW_BUFFER15_ARB = 0x8834;
static { GL.initialize(); }
protected ARBDrawBuffers() {
throw new UnsupportedOperationException();
}
static boolean isAvailable(GLCapabilities caps) {
return checkFunctions(
caps.glDrawBuffersARB
);
}
// --- [ glDrawBuffersARB ] ---
/**
* Unsafe version of: {@link #glDrawBuffersARB DrawBuffersARB}
*
* @param n the number of buffers in {@code bufs}
*/
public static native void nglDrawBuffersARB(int n, long bufs);
/**
* Defines the draw buffers to which all output colors are written.
*
* @param bufs a buffer of symbolic constants specifying the buffer to which each output color is written. One of:
{@link GL11#GL_NONE NONE} {@link GL11#GL_FRONT_LEFT FRONT_LEFT} {@link GL11#GL_FRONT_RIGHT FRONT_RIGHT} {@link GL11#GL_BACK_LEFT BACK_LEFT} {@link GL11#GL_BACK_RIGHT BACK_RIGHT} {@link GL11#GL_AUX0 AUX0} {@link GL11#GL_AUX1 AUX1} {@link GL11#GL_AUX2 AUX2} {@link GL11#GL_AUX3 AUX3} {@link GL30#GL_COLOR_ATTACHMENT0 COLOR_ATTACHMENT0} GL30.GL_COLOR_ATTACHMENT[1-15]
*/
public static void glDrawBuffersARB(@NativeType("const GLenum *") IntBuffer bufs) {
nglDrawBuffersARB(bufs.remaining(), memAddress(bufs));
}
/** Array version of: {@link #glDrawBuffersARB DrawBuffersARB} */
public static void glDrawBuffersARB(@NativeType("const GLenum *") int[] bufs) {
long __functionAddress = GL.getICD().glDrawBuffersARB;
if (CHECKS) {
check(__functionAddress);
}
callPV(__functionAddress, bufs.length, bufs);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy