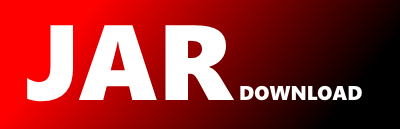
org.lwjgl.opengl.EXTDepthBoundsTest Maven / Gradle / Ivy
Show all versions of lwjgl-opengl Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.opengl;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
/**
* Native bindings to the EXT_depth_bounds_test extension.
*
* This extension adds a new per-fragment test that is, logically, after the scissor test and before the alpha test. The depth bounds test compares the
* depth value stored at the location given by the incoming fragment's (xw,yw) coordinates to a user-defined minimum and maximum depth value. If the stored
* depth value is outside the user-defined range (exclusive), the incoming fragment is discarded.
*
* Unlike the depth test, the depth bounds test has NO dependency on the fragment's window-space depth value.
*
* This functionality is useful in the context of attenuated stenciled shadow volume rendering. To motivate the functionality's utility in this context, we
* first describe how conventional scissor testing can be used to optimize shadow volume rendering.
*
* If an attenuated light source's illumination can be bounded to a rectangle in XY window-space, the conventional scissor test can be used to discard
* shadow volume fragments that are guaranteed to be outside the light source's window-space XY rectangle. The stencil increments and decrements that would
* otherwise be generated by these scissored fragments are inconsequential because the light source's illumination can pre-determined to be fully
* attenuated outside the scissored region. In other words, the scissor test can be used to discard shadow volume fragments rendered outside the scissor,
* thereby improving performance, without affecting the ultimate illumination of these pixels with respect to the attenuated light source.
*
* This scissoring optimization can be used both when rendering the stenciled shadow volumes to update stencil (incrementing and decrementing the stencil
* buffer) AND when adding the illumination contribution of attenuated light source's.
*
* In a similar fashion, we can compute the attenuated light source's window-space Z bounds (zmin,zmax) of consequential illumination. Unless a depth value
* (in the depth buffer) at a pixel is within the range [zmin,zmax], the light source's illumination can be pre-determined to be inconsequential for the
* pixel. Said another way, the pixel being illuminated is either far enough in front of or behind the attenuated light source so that the light source's
* illumination for the pixel is fully attenuated. The depth bounds test can perform this test.
*/
public class EXTDepthBoundsTest {
/**
* Accepted by the {@code cap} parameter of Enable, Disable, and IsEnabled, and by the {@code pname} parameter of GetBooleanv, GetIntegerv, GetFloatv, and
* GetDoublev.
*/
public static final int GL_DEPTH_BOUNDS_TEST_EXT = 0x8890;
/** Accepted by the {@code pname} parameter of GetBooleanv, GetIntegerv, GetFloatv, and GetDoublev. */
public static final int GL_DEPTH_BOUNDS_EXT = 0x8891;
static { GL.initialize(); }
protected EXTDepthBoundsTest() {
throw new UnsupportedOperationException();
}
static boolean isAvailable(GLCapabilities caps) {
return checkFunctions(
caps.glDepthBoundsEXT
);
}
// --- [ glDepthBoundsEXT ] ---
public static native void glDepthBoundsEXT(double zmin, double zmax);
}