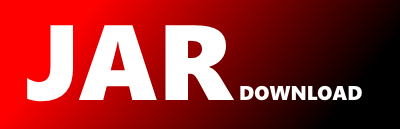
org.lwjgl.opengl.GLXSGIXSwapBarrier Maven / Gradle / Ivy
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.opengl;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* Native bindings to the GLX_SGIX_swap_barrier extension.
*
* This extension provides the capability to sychronize the buffer swaps of different swap groups.
*
* Requires {@link GLXSGIXSwapGroup GLX_SGIX_swap_group}.
*/
public class GLXSGIXSwapBarrier {
protected GLXSGIXSwapBarrier() {
throw new UnsupportedOperationException();
}
static boolean isAvailable(GLXCapabilities caps) {
return checkFunctions(
caps.glXBindSwapBarrierSGIX, caps.glXQueryMaxSwapBarriersSGIX
);
}
// --- [ glXBindSwapBarrierSGIX ] ---
/**
* Binds the swap group that contains {@code drawable} to {@code barrier}. Subsequent buffer swaps for that group will be subject to this binding, until
* the group is unbound from {@code barrier}. If {@code barrier} is zero, the group is unbound from its current barrier, if any.
*
* @param display the connection to the X server
* @param drawable the swap group GLXDrawable
* @param barrier the swap barrier
*/
public static void glXBindSwapBarrierSGIX(@NativeType("Display *") long display, @NativeType("GLXDrawable") long drawable, int barrier) {
long __functionAddress = GL.getCapabilitiesGLXClient().glXBindSwapBarrierSGIX;
if (CHECKS) {
check(__functionAddress);
check(display);
check(drawable);
}
callPPV(__functionAddress, display, drawable, barrier);
}
// --- [ glXQueryMaxSwapBarriersSGIX ] ---
/** Unsafe version of: {@link #glXQueryMaxSwapBarriersSGIX QueryMaxSwapBarriersSGIX} */
public static int nglXQueryMaxSwapBarriersSGIX(long display, int screen, long max) {
long __functionAddress = GL.getCapabilitiesGLXClient().glXQueryMaxSwapBarriersSGIX;
if (CHECKS) {
check(__functionAddress);
check(display);
}
return callPPI(__functionAddress, display, screen, max);
}
/**
* Returns in {@code max} the maximum number of barriers supported by an implementation on {@code screen}.
*
* @param display the connection to the X server
* @param screen the screen
* @param max returns the maximum number of barriers
*/
@NativeType("Bool")
public static boolean glXQueryMaxSwapBarriersSGIX(@NativeType("Display *") long display, int screen, @NativeType("int *") IntBuffer max) {
if (CHECKS) {
check(max, 1);
}
return nglXQueryMaxSwapBarriersSGIX(display, screen, memAddress(max)) != 0;
}
/** Array version of: {@link #glXQueryMaxSwapBarriersSGIX QueryMaxSwapBarriersSGIX} */
@NativeType("Bool")
public static boolean glXQueryMaxSwapBarriersSGIX(@NativeType("Display *") long display, int screen, @NativeType("int *") int[] max) {
long __functionAddress = GL.getCapabilitiesGLXClient().glXQueryMaxSwapBarriersSGIX;
if (CHECKS) {
check(__functionAddress);
check(display);
check(max, 1);
}
return callPPI(__functionAddress, display, screen, max) != 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy