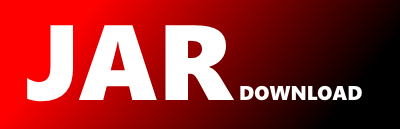
org.lwjgl.openvr.PropertyWrite Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwjgl-openvr Show documentation
Show all versions of lwjgl-openvr Show documentation
An API and runtime that allows access to VR hardware from multiple vendors without requiring that applications have specific knowledge of the hardware they are targeting.
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openvr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.MemoryUtil.*;
import static org.lwjgl.system.MemoryStack.*;
/**
* Layout
*
*
* struct PropertyWrite_t {
* ETrackedDeviceProperty {@link #prop};
* EPropertyWriteType {@link #writeType};
* ETrackedPropertyError {@link #eSetError};
* void * pvBuffer;
* uint32_t unBufferSize;
* PropertyTypeTag_t unTag;
* ETrackedPropertyError {@link #eError};
* }
*/
@NativeType("struct PropertyWrite_t")
public class PropertyWrite extends Struct implements NativeResource {
/** The struct size in bytes. */
public static final int SIZEOF;
/** The struct alignment in bytes. */
public static final int ALIGNOF;
/** The struct member offsets. */
public static final int
PROP,
WRITETYPE,
ESETERROR,
PVBUFFER,
UNBUFFERSIZE,
UNTAG,
EERROR;
static {
Layout layout = __struct(
__member(4),
__member(4),
__member(4),
__member(POINTER_SIZE),
__member(4),
__member(4),
__member(4)
);
SIZEOF = layout.getSize();
ALIGNOF = layout.getAlignment();
PROP = layout.offsetof(0);
WRITETYPE = layout.offsetof(1);
ESETERROR = layout.offsetof(2);
PVBUFFER = layout.offsetof(3);
UNBUFFERSIZE = layout.offsetof(4);
UNTAG = layout.offsetof(5);
EERROR = layout.offsetof(6);
}
protected PropertyWrite(long address, @Nullable ByteBuffer container) {
super(address, container);
}
@Override
protected PropertyWrite create(long address, @Nullable ByteBuffer container) {
return new PropertyWrite(address, container);
}
/**
* Creates a {@code PropertyWrite} instance at the current position of the specified {@link ByteBuffer} container. Changes to the buffer's content will be
* visible to the struct instance and vice versa.
*
* The created instance holds a strong reference to the container object.
*/
public PropertyWrite(ByteBuffer container) {
super(memAddress(container), __checkContainer(container, SIZEOF));
}
@Override
public int sizeof() { return SIZEOF; }
/** one of:
{@link VR#ETrackedDeviceProperty_Prop_Invalid} {@link VR#ETrackedDeviceProperty_Prop_TrackingSystemName_String} {@link VR#ETrackedDeviceProperty_Prop_ModelNumber_String} {@link VR#ETrackedDeviceProperty_Prop_SerialNumber_String} {@link VR#ETrackedDeviceProperty_Prop_RenderModelName_String} {@link VR#ETrackedDeviceProperty_Prop_WillDriftInYaw_Bool} {@link VR#ETrackedDeviceProperty_Prop_ManufacturerName_String} {@link VR#ETrackedDeviceProperty_Prop_TrackingFirmwareVersion_String} {@link VR#ETrackedDeviceProperty_Prop_HardwareRevision_String} {@link VR#ETrackedDeviceProperty_Prop_AllWirelessDongleDescriptions_String} {@link VR#ETrackedDeviceProperty_Prop_ConnectedWirelessDongle_String} {@link VR#ETrackedDeviceProperty_Prop_DeviceIsWireless_Bool} {@link VR#ETrackedDeviceProperty_Prop_DeviceIsCharging_Bool} {@link VR#ETrackedDeviceProperty_Prop_DeviceBatteryPercentage_Float} {@link VR#ETrackedDeviceProperty_Prop_StatusDisplayTransform_Matrix34} {@link VR#ETrackedDeviceProperty_Prop_Firmware_UpdateAvailable_Bool} {@link VR#ETrackedDeviceProperty_Prop_Firmware_ManualUpdate_Bool} {@link VR#ETrackedDeviceProperty_Prop_Firmware_ManualUpdateURL_String} {@link VR#ETrackedDeviceProperty_Prop_HardwareRevision_Uint64} {@link VR#ETrackedDeviceProperty_Prop_FirmwareVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_FPGAVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_VRCVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_RadioVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_DongleVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_BlockServerShutdown_Bool} {@link VR#ETrackedDeviceProperty_Prop_CanUnifyCoordinateSystemWithHmd_Bool} {@link VR#ETrackedDeviceProperty_Prop_ContainsProximitySensor_Bool} {@link VR#ETrackedDeviceProperty_Prop_DeviceProvidesBatteryStatus_Bool} {@link VR#ETrackedDeviceProperty_Prop_DeviceCanPowerOff_Bool} {@link VR#ETrackedDeviceProperty_Prop_Firmware_ProgrammingTarget_String} {@link VR#ETrackedDeviceProperty_Prop_DeviceClass_Int32} {@link VR#ETrackedDeviceProperty_Prop_HasCamera_Bool} {@link VR#ETrackedDeviceProperty_Prop_DriverVersion_String} {@link VR#ETrackedDeviceProperty_Prop_Firmware_ForceUpdateRequired_Bool} {@link VR#ETrackedDeviceProperty_Prop_ViveSystemButtonFixRequired_Bool} {@link VR#ETrackedDeviceProperty_Prop_ParentDriver_Uint64} {@link VR#ETrackedDeviceProperty_Prop_ResourceRoot_String} {@link VR#ETrackedDeviceProperty_Prop_RegisteredDeviceType_String} {@link VR#ETrackedDeviceProperty_Prop_InputProfilePath_String} {@link VR#ETrackedDeviceProperty_Prop_NeverTracked_Bool} {@link VR#ETrackedDeviceProperty_Prop_NumCameras_Int32} {@link VR#ETrackedDeviceProperty_Prop_CameraFrameLayout_Int32} {@link VR#ETrackedDeviceProperty_Prop_CameraStreamFormat_Int32} {@link VR#ETrackedDeviceProperty_Prop_AdditionalDeviceSettingsPath_String} {@link VR#ETrackedDeviceProperty_Prop_Identifiable_Bool} {@link VR#ETrackedDeviceProperty_Prop_BootloaderVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_AdditionalSystemReportData_String} {@link VR#ETrackedDeviceProperty_Prop_CompositeFirmwareVersion_String} {@link VR#ETrackedDeviceProperty_Prop_Firmware_RemindUpdate_Bool} {@link VR#ETrackedDeviceProperty_Prop_PeripheralApplicationVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_ManufacturerSerialNumber_String} {@link VR#ETrackedDeviceProperty_Prop_ComputedSerialNumber_String} {@link VR#ETrackedDeviceProperty_Prop_EstimatedDeviceFirstUseTime_Int32} {@link VR#ETrackedDeviceProperty_Prop_DevicePowerUsage_Float} {@link VR#ETrackedDeviceProperty_Prop_IgnoreMotionForStandby_Bool} {@link VR#ETrackedDeviceProperty_Prop_ActualTrackingSystemName_String} {@link VR#ETrackedDeviceProperty_Prop_ReportsTimeSinceVSync_Bool} {@link VR#ETrackedDeviceProperty_Prop_SecondsFromVsyncToPhotons_Float} {@link VR#ETrackedDeviceProperty_Prop_DisplayFrequency_Float} {@link VR#ETrackedDeviceProperty_Prop_UserIpdMeters_Float} {@link VR#ETrackedDeviceProperty_Prop_CurrentUniverseId_Uint64} {@link VR#ETrackedDeviceProperty_Prop_PreviousUniverseId_Uint64} {@link VR#ETrackedDeviceProperty_Prop_DisplayFirmwareVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_IsOnDesktop_Bool} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCType_Int32} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCOffset_Float} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCScale_Float} {@link VR#ETrackedDeviceProperty_Prop_EdidVendorID_Int32} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCImageLeft_String} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCImageRight_String} {@link VR#ETrackedDeviceProperty_Prop_DisplayGCBlackClamp_Float} {@link VR#ETrackedDeviceProperty_Prop_EdidProductID_Int32} {@link VR#ETrackedDeviceProperty_Prop_CameraToHeadTransform_Matrix34} {@link VR#ETrackedDeviceProperty_Prop_DisplayGCType_Int32} {@link VR#ETrackedDeviceProperty_Prop_DisplayGCOffset_Float} {@link VR#ETrackedDeviceProperty_Prop_DisplayGCScale_Float} {@link VR#ETrackedDeviceProperty_Prop_DisplayGCPrescale_Float} {@link VR#ETrackedDeviceProperty_Prop_DisplayGCImage_String} {@link VR#ETrackedDeviceProperty_Prop_LensCenterLeftU_Float} {@link VR#ETrackedDeviceProperty_Prop_LensCenterLeftV_Float} {@link VR#ETrackedDeviceProperty_Prop_LensCenterRightU_Float} {@link VR#ETrackedDeviceProperty_Prop_LensCenterRightV_Float} {@link VR#ETrackedDeviceProperty_Prop_UserHeadToEyeDepthMeters_Float} {@link VR#ETrackedDeviceProperty_Prop_CameraFirmwareVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_CameraFirmwareDescription_String} {@link VR#ETrackedDeviceProperty_Prop_DisplayFPGAVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_DisplayBootloaderVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_DisplayHardwareVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_AudioFirmwareVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_CameraCompatibilityMode_Int32} {@link VR#ETrackedDeviceProperty_Prop_ScreenshotHorizontalFieldOfViewDegrees_Float} {@link VR#ETrackedDeviceProperty_Prop_ScreenshotVerticalFieldOfViewDegrees_Float} {@link VR#ETrackedDeviceProperty_Prop_DisplaySuppressed_Bool} {@link VR#ETrackedDeviceProperty_Prop_DisplayAllowNightMode_Bool} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCImageWidth_Int32} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCImageHeight_Int32} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCImageNumChannels_Int32} {@link VR#ETrackedDeviceProperty_Prop_DisplayMCImageData_Binary} {@link VR#ETrackedDeviceProperty_Prop_SecondsFromPhotonsToVblank_Float} {@link VR#ETrackedDeviceProperty_Prop_DriverDirectModeSendsVsyncEvents_Bool} {@link VR#ETrackedDeviceProperty_Prop_DisplayDebugMode_Bool} {@link VR#ETrackedDeviceProperty_Prop_GraphicsAdapterLuid_Uint64} {@link VR#ETrackedDeviceProperty_Prop_DriverProvidedChaperonePath_String} {@link VR#ETrackedDeviceProperty_Prop_ExpectedTrackingReferenceCount_Int32} {@link VR#ETrackedDeviceProperty_Prop_ExpectedControllerCount_Int32} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathControllerLeftDeviceOff_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathControllerRightDeviceOff_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathTrackingReferenceDeviceOff_String} {@link VR#ETrackedDeviceProperty_Prop_DoNotApplyPrediction_Bool} {@link VR#ETrackedDeviceProperty_Prop_CameraToHeadTransforms_Matrix34_Array} {@link VR#ETrackedDeviceProperty_Prop_DistortionMeshResolution_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverIsDrawingControllers_Bool} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestsApplicationPause_Bool} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestsReducedRendering_Bool} {@link VR#ETrackedDeviceProperty_Prop_MinimumIpdStepMeters_Float} {@link VR#ETrackedDeviceProperty_Prop_AudioBridgeFirmwareVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_ImageBridgeFirmwareVersion_Uint64} {@link VR#ETrackedDeviceProperty_Prop_ImuToHeadTransform_Matrix34} {@link VR#ETrackedDeviceProperty_Prop_ImuFactoryGyroBias_Vector3} {@link VR#ETrackedDeviceProperty_Prop_ImuFactoryGyroScale_Vector3} {@link VR#ETrackedDeviceProperty_Prop_ImuFactoryAccelerometerBias_Vector3} {@link VR#ETrackedDeviceProperty_Prop_ImuFactoryAccelerometerScale_Vector3} {@link VR#ETrackedDeviceProperty_Prop_ConfigurationIncludesLighthouse20Features_Bool} {@link VR#ETrackedDeviceProperty_Prop_AdditionalRadioFeatures_Uint64} {@link VR#ETrackedDeviceProperty_Prop_CameraWhiteBalance_Vector4_Array} {@link VR#ETrackedDeviceProperty_Prop_CameraDistortionFunction_Int32_Array} {@link VR#ETrackedDeviceProperty_Prop_CameraDistortionCoefficients_Float_Array} {@link VR#ETrackedDeviceProperty_Prop_ExpectedControllerType_String} {@link VR#ETrackedDeviceProperty_Prop_HmdTrackingStyle_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverProvidedChaperoneVisibility_Bool} {@link VR#ETrackedDeviceProperty_Prop_HmdColumnCorrectionSettingPrefix_String} {@link VR#ETrackedDeviceProperty_Prop_CameraSupportsCompatibilityModes_Bool} {@link VR#ETrackedDeviceProperty_Prop_SupportsRoomViewDepthProjection_Bool} {@link VR#ETrackedDeviceProperty_Prop_DisplayAvailableFrameRates_Float_Array} {@link VR#ETrackedDeviceProperty_Prop_DisplaySupportsMultipleFramerates_Bool} {@link VR#ETrackedDeviceProperty_Prop_DisplayColorMultLeft_Vector3} {@link VR#ETrackedDeviceProperty_Prop_DisplayColorMultRight_Vector3} {@link VR#ETrackedDeviceProperty_Prop_DisplaySupportsRuntimeFramerateChange_Bool} {@link VR#ETrackedDeviceProperty_Prop_DisplaySupportsAnalogGain_Bool} {@link VR#ETrackedDeviceProperty_Prop_DisplayMinAnalogGain_Float} {@link VR#ETrackedDeviceProperty_Prop_DisplayMaxAnalogGain_Float} {@link VR#ETrackedDeviceProperty_Prop_CameraExposureTime_Float} {@link VR#ETrackedDeviceProperty_Prop_CameraGlobalGain_Float} {@link VR#ETrackedDeviceProperty_Prop_DashboardScale_Float} {@link VR#ETrackedDeviceProperty_Prop_PeerButtonInfo_String} {@link VR#ETrackedDeviceProperty_Prop_Hmd_SupportsHDR10_Bool} {@link VR#ETrackedDeviceProperty_Prop_Hmd_EnableParallelRenderCameras_Bool} {@link VR#ETrackedDeviceProperty_Prop_DriverProvidedChaperoneJson_String} {@link VR#ETrackedDeviceProperty_Prop_ForceSystemLayerUseAppPoses_Bool} {@link VR#ETrackedDeviceProperty_Prop_IpdUIRangeMinMeters_Float} {@link VR#ETrackedDeviceProperty_Prop_IpdUIRangeMaxMeters_Float} {@link VR#ETrackedDeviceProperty_Prop_Hmd_SupportsHDCP14LegacyCompat_Bool} {@link VR#ETrackedDeviceProperty_Prop_Hmd_SupportsMicMonitoring_Bool} {@link VR#ETrackedDeviceProperty_Prop_Hmd_SupportsDisplayPortTrainingMode_Bool} {@link VR#ETrackedDeviceProperty_Prop_Hmd_SupportsRoomViewDirect_Bool} {@link VR#ETrackedDeviceProperty_Prop_Hmd_SupportsAppThrottling_Bool} {@link VR#ETrackedDeviceProperty_Prop_Hmd_SupportsGpuBusMonitoring_Bool} {@link VR#ETrackedDeviceProperty_Prop_DriverDisplaysIPDChanges_Bool} {@link VR#ETrackedDeviceProperty_Prop_Driver_Reserved_01} {@link VR#ETrackedDeviceProperty_Prop_DSCVersion_Int32} {@link VR#ETrackedDeviceProperty_Prop_DSCSliceCount_Int32} {@link VR#ETrackedDeviceProperty_Prop_DSCBPPx16_Int32} {@link VR#ETrackedDeviceProperty_Prop_Hmd_MaxDistortedTextureWidth_Int32} {@link VR#ETrackedDeviceProperty_Prop_Hmd_MaxDistortedTextureHeight_Int32} {@link VR#ETrackedDeviceProperty_Prop_Hmd_AllowSupersampleFiltering_Bool} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraCorrectionMode_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraFeather_InnerLeft_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraFeather_InnerRight_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraFeather_InnerTop_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraFeather_InnerBottom_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraFeather_OuterLeft_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraFeather_OuterRight_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraFeather_OuterTop_Int32} {@link VR#ETrackedDeviceProperty_Prop_DriverRequestedMuraFeather_OuterBottom_Int32} {@link VR#ETrackedDeviceProperty_Prop_Audio_DefaultPlaybackDeviceId_String} {@link VR#ETrackedDeviceProperty_Prop_Audio_DefaultRecordingDeviceId_String} {@link VR#ETrackedDeviceProperty_Prop_Audio_DefaultPlaybackDeviceVolume_Float} {@link VR#ETrackedDeviceProperty_Prop_Audio_SupportsDualSpeakerAndJackOutput_Bool} {@link VR#ETrackedDeviceProperty_Prop_Audio_DriverManagesPlaybackVolumeControl_Bool} {@link VR#ETrackedDeviceProperty_Prop_Audio_DriverPlaybackVolume_Float} {@link VR#ETrackedDeviceProperty_Prop_Audio_DriverPlaybackMute_Bool} {@link VR#ETrackedDeviceProperty_Prop_Audio_DriverManagesRecordingVolumeControl_Bool} {@link VR#ETrackedDeviceProperty_Prop_Audio_DriverRecordingVolume_Float} {@link VR#ETrackedDeviceProperty_Prop_Audio_DriverRecordingMute_Bool} {@link VR#ETrackedDeviceProperty_Prop_AttachedDeviceId_String} {@link VR#ETrackedDeviceProperty_Prop_SupportedButtons_Uint64} {@link VR#ETrackedDeviceProperty_Prop_Axis0Type_Int32} {@link VR#ETrackedDeviceProperty_Prop_Axis1Type_Int32} {@link VR#ETrackedDeviceProperty_Prop_Axis2Type_Int32} {@link VR#ETrackedDeviceProperty_Prop_Axis3Type_Int32} {@link VR#ETrackedDeviceProperty_Prop_Axis4Type_Int32} {@link VR#ETrackedDeviceProperty_Prop_ControllerRoleHint_Int32} {@link VR#ETrackedDeviceProperty_Prop_FieldOfViewLeftDegrees_Float} {@link VR#ETrackedDeviceProperty_Prop_FieldOfViewRightDegrees_Float} {@link VR#ETrackedDeviceProperty_Prop_FieldOfViewTopDegrees_Float} {@link VR#ETrackedDeviceProperty_Prop_FieldOfViewBottomDegrees_Float} {@link VR#ETrackedDeviceProperty_Prop_TrackingRangeMinimumMeters_Float} {@link VR#ETrackedDeviceProperty_Prop_TrackingRangeMaximumMeters_Float} {@link VR#ETrackedDeviceProperty_Prop_ModeLabel_String} {@link VR#ETrackedDeviceProperty_Prop_CanWirelessIdentify_Bool} {@link VR#ETrackedDeviceProperty_Prop_Nonce_Int32} {@link VR#ETrackedDeviceProperty_Prop_IconPathName_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceOff_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceSearching_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceSearchingAlert_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceReady_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceReadyAlert_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceNotReady_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceStandby_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceAlertLow_String} {@link VR#ETrackedDeviceProperty_Prop_NamedIconPathDeviceStandbyAlert_String} {@link VR#ETrackedDeviceProperty_Prop_DisplayHiddenArea_Binary_Start} {@link VR#ETrackedDeviceProperty_Prop_DisplayHiddenArea_Binary_End} {@link VR#ETrackedDeviceProperty_Prop_ParentContainer} {@link VR#ETrackedDeviceProperty_Prop_OverrideContainer_Uint64} {@link VR#ETrackedDeviceProperty_Prop_UserConfigPath_String} {@link VR#ETrackedDeviceProperty_Prop_InstallPath_String} {@link VR#ETrackedDeviceProperty_Prop_HasDisplayComponent_Bool} {@link VR#ETrackedDeviceProperty_Prop_HasControllerComponent_Bool} {@link VR#ETrackedDeviceProperty_Prop_HasCameraComponent_Bool} {@link VR#ETrackedDeviceProperty_Prop_HasDriverDirectModeComponent_Bool} {@link VR#ETrackedDeviceProperty_Prop_HasVirtualDisplayComponent_Bool} {@link VR#ETrackedDeviceProperty_Prop_HasSpatialAnchorsSupport_Bool} {@link VR#ETrackedDeviceProperty_Prop_SupportsXrTextureSets_Bool} {@link VR#ETrackedDeviceProperty_Prop_ControllerType_String} {@link VR#ETrackedDeviceProperty_Prop_ControllerHandSelectionPriority_Int32} {@link VR#ETrackedDeviceProperty_Prop_VendorSpecific_Reserved_Start} {@link VR#ETrackedDeviceProperty_Prop_VendorSpecific_Reserved_End} {@link VR#ETrackedDeviceProperty_Prop_TrackedDeviceProperty_Max}
*/
@NativeType("ETrackedDeviceProperty")
public int prop() { return nprop(address()); }
/** one of:
{@link VR#EPropertyWriteType_PropertyWrite_Set} {@link VR#EPropertyWriteType_PropertyWrite_Erase} {@link VR#EPropertyWriteType_PropertyWrite_SetError}
*/
@NativeType("EPropertyWriteType")
public int writeType() { return nwriteType(address()); }
/** one of:
{@link VR#ETrackedPropertyError_TrackedProp_Success} {@link VR#ETrackedPropertyError_TrackedProp_WrongDataType} {@link VR#ETrackedPropertyError_TrackedProp_WrongDeviceClass} {@link VR#ETrackedPropertyError_TrackedProp_BufferTooSmall} {@link VR#ETrackedPropertyError_TrackedProp_UnknownProperty} {@link VR#ETrackedPropertyError_TrackedProp_InvalidDevice} {@link VR#ETrackedPropertyError_TrackedProp_CouldNotContactServer} {@link VR#ETrackedPropertyError_TrackedProp_ValueNotProvidedByDevice} {@link VR#ETrackedPropertyError_TrackedProp_StringExceedsMaximumLength} {@link VR#ETrackedPropertyError_TrackedProp_NotYetAvailable} {@link VR#ETrackedPropertyError_TrackedProp_PermissionDenied} {@link VR#ETrackedPropertyError_TrackedProp_InvalidOperation} {@link VR#ETrackedPropertyError_TrackedProp_CannotWriteToWildcards} {@link VR#ETrackedPropertyError_TrackedProp_IPCReadFailure} {@link VR#ETrackedPropertyError_TrackedProp_OutOfMemory} {@link VR#ETrackedPropertyError_TrackedProp_InvalidContainer}
*/
@NativeType("ETrackedPropertyError")
public int eSetError() { return neSetError(address()); }
/** @return a {@link ByteBuffer} view of the data pointed to by the {@code pvBuffer} field. */
@NativeType("void *")
public ByteBuffer pvBuffer() { return npvBuffer(address()); }
/** @return the value of the {@code unBufferSize} field. */
@NativeType("uint32_t")
public int unBufferSize() { return nunBufferSize(address()); }
/** @return the value of the {@code unTag} field. */
@NativeType("PropertyTypeTag_t")
public int unTag() { return nunTag(address()); }
/** one of:
{@link VR#ETrackedPropertyError_TrackedProp_Success} {@link VR#ETrackedPropertyError_TrackedProp_WrongDataType} {@link VR#ETrackedPropertyError_TrackedProp_WrongDeviceClass} {@link VR#ETrackedPropertyError_TrackedProp_BufferTooSmall} {@link VR#ETrackedPropertyError_TrackedProp_UnknownProperty} {@link VR#ETrackedPropertyError_TrackedProp_InvalidDevice} {@link VR#ETrackedPropertyError_TrackedProp_CouldNotContactServer} {@link VR#ETrackedPropertyError_TrackedProp_ValueNotProvidedByDevice} {@link VR#ETrackedPropertyError_TrackedProp_StringExceedsMaximumLength} {@link VR#ETrackedPropertyError_TrackedProp_NotYetAvailable} {@link VR#ETrackedPropertyError_TrackedProp_PermissionDenied} {@link VR#ETrackedPropertyError_TrackedProp_InvalidOperation} {@link VR#ETrackedPropertyError_TrackedProp_CannotWriteToWildcards} {@link VR#ETrackedPropertyError_TrackedProp_IPCReadFailure} {@link VR#ETrackedPropertyError_TrackedProp_OutOfMemory} {@link VR#ETrackedPropertyError_TrackedProp_InvalidContainer}
*/
@NativeType("ETrackedPropertyError")
public int eError() { return neError(address()); }
/** Sets the specified value to the {@link #prop} field. */
public PropertyWrite prop(@NativeType("ETrackedDeviceProperty") int value) { nprop(address(), value); return this; }
/** Sets the specified value to the {@link #writeType} field. */
public PropertyWrite writeType(@NativeType("EPropertyWriteType") int value) { nwriteType(address(), value); return this; }
/** Sets the specified value to the {@link #eSetError} field. */
public PropertyWrite eSetError(@NativeType("ETrackedPropertyError") int value) { neSetError(address(), value); return this; }
/** Sets the address of the specified {@link ByteBuffer} to the {@code pvBuffer} field. */
public PropertyWrite pvBuffer(@NativeType("void *") ByteBuffer value) { npvBuffer(address(), value); return this; }
/** Sets the specified value to the {@code unTag} field. */
public PropertyWrite unTag(@NativeType("PropertyTypeTag_t") int value) { nunTag(address(), value); return this; }
/** Sets the specified value to the {@link #eError} field. */
public PropertyWrite eError(@NativeType("ETrackedPropertyError") int value) { neError(address(), value); return this; }
/** Initializes this struct with the specified values. */
public PropertyWrite set(
int prop,
int writeType,
int eSetError,
ByteBuffer pvBuffer,
int unTag,
int eError
) {
prop(prop);
writeType(writeType);
eSetError(eSetError);
pvBuffer(pvBuffer);
unTag(unTag);
eError(eError);
return this;
}
/**
* Copies the specified struct data to this struct.
*
* @param src the source struct
*
* @return this struct
*/
public PropertyWrite set(PropertyWrite src) {
memCopy(src.address(), address(), SIZEOF);
return this;
}
// -----------------------------------
/** Returns a new {@code PropertyWrite} instance allocated with {@link MemoryUtil#memAlloc memAlloc}. The instance must be explicitly freed. */
public static PropertyWrite malloc() {
return new PropertyWrite(nmemAllocChecked(SIZEOF), null);
}
/** Returns a new {@code PropertyWrite} instance allocated with {@link MemoryUtil#memCalloc memCalloc}. The instance must be explicitly freed. */
public static PropertyWrite calloc() {
return new PropertyWrite(nmemCallocChecked(1, SIZEOF), null);
}
/** Returns a new {@code PropertyWrite} instance allocated with {@link BufferUtils}. */
public static PropertyWrite create() {
ByteBuffer container = BufferUtils.createByteBuffer(SIZEOF);
return new PropertyWrite(memAddress(container), container);
}
/** Returns a new {@code PropertyWrite} instance for the specified memory address. */
public static PropertyWrite create(long address) {
return new PropertyWrite(address, null);
}
/** Like {@link #create(long) create}, but returns {@code null} if {@code address} is {@code NULL}. */
public static @Nullable PropertyWrite createSafe(long address) {
return address == NULL ? null : new PropertyWrite(address, null);
}
/**
* Returns a new {@link PropertyWrite.Buffer} instance allocated with {@link MemoryUtil#memAlloc memAlloc}. The instance must be explicitly freed.
*
* @param capacity the buffer capacity
*/
public static PropertyWrite.Buffer malloc(int capacity) {
return new Buffer(nmemAllocChecked(__checkMalloc(capacity, SIZEOF)), capacity);
}
/**
* Returns a new {@link PropertyWrite.Buffer} instance allocated with {@link MemoryUtil#memCalloc memCalloc}. The instance must be explicitly freed.
*
* @param capacity the buffer capacity
*/
public static PropertyWrite.Buffer calloc(int capacity) {
return new Buffer(nmemCallocChecked(capacity, SIZEOF), capacity);
}
/**
* Returns a new {@link PropertyWrite.Buffer} instance allocated with {@link BufferUtils}.
*
* @param capacity the buffer capacity
*/
public static PropertyWrite.Buffer create(int capacity) {
ByteBuffer container = __create(capacity, SIZEOF);
return new Buffer(memAddress(container), container, -1, 0, capacity, capacity);
}
/**
* Create a {@link PropertyWrite.Buffer} instance at the specified memory.
*
* @param address the memory address
* @param capacity the buffer capacity
*/
public static PropertyWrite.Buffer create(long address, int capacity) {
return new Buffer(address, capacity);
}
/** Like {@link #create(long, int) create}, but returns {@code null} if {@code address} is {@code NULL}. */
public static PropertyWrite.@Nullable Buffer createSafe(long address, int capacity) {
return address == NULL ? null : new Buffer(address, capacity);
}
/**
* Returns a new {@code PropertyWrite} instance allocated on the specified {@link MemoryStack}.
*
* @param stack the stack from which to allocate
*/
public static PropertyWrite malloc(MemoryStack stack) {
return new PropertyWrite(stack.nmalloc(ALIGNOF, SIZEOF), null);
}
/**
* Returns a new {@code PropertyWrite} instance allocated on the specified {@link MemoryStack} and initializes all its bits to zero.
*
* @param stack the stack from which to allocate
*/
public static PropertyWrite calloc(MemoryStack stack) {
return new PropertyWrite(stack.ncalloc(ALIGNOF, 1, SIZEOF), null);
}
/**
* Returns a new {@link PropertyWrite.Buffer} instance allocated on the specified {@link MemoryStack}.
*
* @param stack the stack from which to allocate
* @param capacity the buffer capacity
*/
public static PropertyWrite.Buffer malloc(int capacity, MemoryStack stack) {
return new Buffer(stack.nmalloc(ALIGNOF, capacity * SIZEOF), capacity);
}
/**
* Returns a new {@link PropertyWrite.Buffer} instance allocated on the specified {@link MemoryStack} and initializes all its bits to zero.
*
* @param stack the stack from which to allocate
* @param capacity the buffer capacity
*/
public static PropertyWrite.Buffer calloc(int capacity, MemoryStack stack) {
return new Buffer(stack.ncalloc(ALIGNOF, capacity, SIZEOF), capacity);
}
// -----------------------------------
/** Unsafe version of {@link #prop}. */
public static int nprop(long struct) { return memGetInt(struct + PropertyWrite.PROP); }
/** Unsafe version of {@link #writeType}. */
public static int nwriteType(long struct) { return memGetInt(struct + PropertyWrite.WRITETYPE); }
/** Unsafe version of {@link #eSetError}. */
public static int neSetError(long struct) { return memGetInt(struct + PropertyWrite.ESETERROR); }
/** Unsafe version of {@link #pvBuffer() pvBuffer}. */
public static ByteBuffer npvBuffer(long struct) { return memByteBuffer(memGetAddress(struct + PropertyWrite.PVBUFFER), nunBufferSize(struct)); }
/** Unsafe version of {@link #unBufferSize}. */
public static int nunBufferSize(long struct) { return memGetInt(struct + PropertyWrite.UNBUFFERSIZE); }
/** Unsafe version of {@link #unTag}. */
public static int nunTag(long struct) { return memGetInt(struct + PropertyWrite.UNTAG); }
/** Unsafe version of {@link #eError}. */
public static int neError(long struct) { return memGetInt(struct + PropertyWrite.EERROR); }
/** Unsafe version of {@link #prop(int) prop}. */
public static void nprop(long struct, int value) { memPutInt(struct + PropertyWrite.PROP, value); }
/** Unsafe version of {@link #writeType(int) writeType}. */
public static void nwriteType(long struct, int value) { memPutInt(struct + PropertyWrite.WRITETYPE, value); }
/** Unsafe version of {@link #eSetError(int) eSetError}. */
public static void neSetError(long struct, int value) { memPutInt(struct + PropertyWrite.ESETERROR, value); }
/** Unsafe version of {@link #pvBuffer(ByteBuffer) pvBuffer}. */
public static void npvBuffer(long struct, ByteBuffer value) { memPutAddress(struct + PropertyWrite.PVBUFFER, memAddress(value)); nunBufferSize(struct, value.remaining()); }
/** Sets the specified value to the {@code unBufferSize} field of the specified {@code struct}. */
public static void nunBufferSize(long struct, int value) { memPutInt(struct + PropertyWrite.UNBUFFERSIZE, value); }
/** Unsafe version of {@link #unTag(int) unTag}. */
public static void nunTag(long struct, int value) { memPutInt(struct + PropertyWrite.UNTAG, value); }
/** Unsafe version of {@link #eError(int) eError}. */
public static void neError(long struct, int value) { memPutInt(struct + PropertyWrite.EERROR, value); }
/**
* Validates pointer members that should not be {@code NULL}.
*
* @param struct the struct to validate
*/
public static void validate(long struct) {
check(memGetAddress(struct + PropertyWrite.PVBUFFER));
}
// -----------------------------------
/** An array of {@link PropertyWrite} structs. */
public static class Buffer extends StructBuffer implements NativeResource {
private static final PropertyWrite ELEMENT_FACTORY = PropertyWrite.create(-1L);
/**
* Creates a new {@code PropertyWrite.Buffer} instance backed by the specified container.
*
* Changes to the container's content will be visible to the struct buffer instance and vice versa. The two buffers' position, limit, and mark values
* will be independent. The new buffer's position will be zero, its capacity and its limit will be the number of bytes remaining in this buffer divided
* by {@link PropertyWrite#SIZEOF}, and its mark will be undefined.
*
* The created buffer instance holds a strong reference to the container object.
*/
public Buffer(ByteBuffer container) {
super(container, container.remaining() / SIZEOF);
}
public Buffer(long address, int cap) {
super(address, null, -1, 0, cap, cap);
}
Buffer(long address, @Nullable ByteBuffer container, int mark, int pos, int lim, int cap) {
super(address, container, mark, pos, lim, cap);
}
@Override
protected Buffer self() {
return this;
}
@Override
protected Buffer create(long address, @Nullable ByteBuffer container, int mark, int position, int limit, int capacity) {
return new Buffer(address, container, mark, position, limit, capacity);
}
@Override
protected PropertyWrite getElementFactory() {
return ELEMENT_FACTORY;
}
/** @return the value of the {@link PropertyWrite#prop} field. */
@NativeType("ETrackedDeviceProperty")
public int prop() { return PropertyWrite.nprop(address()); }
/** @return the value of the {@link PropertyWrite#writeType} field. */
@NativeType("EPropertyWriteType")
public int writeType() { return PropertyWrite.nwriteType(address()); }
/** @return the value of the {@link PropertyWrite#eSetError} field. */
@NativeType("ETrackedPropertyError")
public int eSetError() { return PropertyWrite.neSetError(address()); }
/** @return a {@link ByteBuffer} view of the data pointed to by the {@code pvBuffer} field. */
@NativeType("void *")
public ByteBuffer pvBuffer() { return PropertyWrite.npvBuffer(address()); }
/** @return the value of the {@code unBufferSize} field. */
@NativeType("uint32_t")
public int unBufferSize() { return PropertyWrite.nunBufferSize(address()); }
/** @return the value of the {@code unTag} field. */
@NativeType("PropertyTypeTag_t")
public int unTag() { return PropertyWrite.nunTag(address()); }
/** @return the value of the {@link PropertyWrite#eError} field. */
@NativeType("ETrackedPropertyError")
public int eError() { return PropertyWrite.neError(address()); }
/** Sets the specified value to the {@link PropertyWrite#prop} field. */
public PropertyWrite.Buffer prop(@NativeType("ETrackedDeviceProperty") int value) { PropertyWrite.nprop(address(), value); return this; }
/** Sets the specified value to the {@link PropertyWrite#writeType} field. */
public PropertyWrite.Buffer writeType(@NativeType("EPropertyWriteType") int value) { PropertyWrite.nwriteType(address(), value); return this; }
/** Sets the specified value to the {@link PropertyWrite#eSetError} field. */
public PropertyWrite.Buffer eSetError(@NativeType("ETrackedPropertyError") int value) { PropertyWrite.neSetError(address(), value); return this; }
/** Sets the address of the specified {@link ByteBuffer} to the {@code pvBuffer} field. */
public PropertyWrite.Buffer pvBuffer(@NativeType("void *") ByteBuffer value) { PropertyWrite.npvBuffer(address(), value); return this; }
/** Sets the specified value to the {@code unTag} field. */
public PropertyWrite.Buffer unTag(@NativeType("PropertyTypeTag_t") int value) { PropertyWrite.nunTag(address(), value); return this; }
/** Sets the specified value to the {@link PropertyWrite#eError} field. */
public PropertyWrite.Buffer eError(@NativeType("ETrackedPropertyError") int value) { PropertyWrite.neError(address(), value); return this; }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy