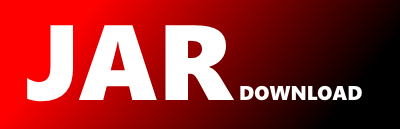
org.lwjgl.openvr.VREvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwjgl-openvr Show documentation
Show all versions of lwjgl-openvr Show documentation
An API and runtime that allows access to VR hardware from multiple vendors without requiring that applications have specific knowledge of the hardware they are targeting.
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openvr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.MemoryUtil.*;
import static org.lwjgl.system.MemoryStack.*;
/**
* An event posted by the server to all running applications.
*
* Layout
*
*
* struct VREvent_t {
* uint32_t {@link #eventType};
* TrackedDeviceIndex_t {@link #trackedDeviceIndex};
* float {@link #eventAgeSeconds};
* {@link VREventData VREvent_Data_t} {@link #data};
* }
*/
@NativeType("struct VREvent_t")
public class VREvent extends Struct implements NativeResource {
/** The struct size in bytes. */
public static final int SIZEOF;
/** The struct alignment in bytes. */
public static final int ALIGNOF;
/** The struct member offsets. */
public static final int
EVENTTYPE,
TRACKEDDEVICEINDEX,
EVENTAGESECONDS,
DATA;
static {
Layout layout = __struct(
Platform.get() == Platform.LINUX || Platform.get() == Platform.MACOSX ? 4 : DEFAULT_PACK_ALIGNMENT, DEFAULT_ALIGN_AS,
__member(4),
__member(4),
__member(4),
__member(VREventData.SIZEOF, VREventData.ALIGNOF)
);
SIZEOF = layout.getSize();
ALIGNOF = layout.getAlignment();
EVENTTYPE = layout.offsetof(0);
TRACKEDDEVICEINDEX = layout.offsetof(1);
EVENTAGESECONDS = layout.offsetof(2);
DATA = layout.offsetof(3);
}
protected VREvent(long address, @Nullable ByteBuffer container) {
super(address, container);
}
@Override
protected VREvent create(long address, @Nullable ByteBuffer container) {
return new VREvent(address, container);
}
/**
* Creates a {@code VREvent} instance at the current position of the specified {@link ByteBuffer} container. Changes to the buffer's content will be
* visible to the struct instance and vice versa.
*
* The created instance holds a strong reference to the container object.
*/
public VREvent(ByteBuffer container) {
super(memAddress(container), __checkContainer(container, SIZEOF));
}
@Override
public int sizeof() { return SIZEOF; }
/** the type of the event. One of:
{@link VR#EVREventType_VREvent_None} {@link VR#EVREventType_VREvent_TrackedDeviceActivated} {@link VR#EVREventType_VREvent_TrackedDeviceDeactivated} {@link VR#EVREventType_VREvent_TrackedDeviceUpdated} {@link VR#EVREventType_VREvent_TrackedDeviceUserInteractionStarted} {@link VR#EVREventType_VREvent_TrackedDeviceUserInteractionEnded} {@link VR#EVREventType_VREvent_IpdChanged} {@link VR#EVREventType_VREvent_EnterStandbyMode} {@link VR#EVREventType_VREvent_LeaveStandbyMode} {@link VR#EVREventType_VREvent_TrackedDeviceRoleChanged} {@link VR#EVREventType_VREvent_WatchdogWakeUpRequested} {@link VR#EVREventType_VREvent_LensDistortionChanged} {@link VR#EVREventType_VREvent_PropertyChanged} {@link VR#EVREventType_VREvent_WirelessDisconnect} {@link VR#EVREventType_VREvent_WirelessReconnect} {@link VR#EVREventType_VREvent_Reserved_01} {@link VR#EVREventType_VREvent_Reserved_02} {@link VR#EVREventType_VREvent_ButtonPress} {@link VR#EVREventType_VREvent_ButtonUnpress} {@link VR#EVREventType_VREvent_ButtonTouch} {@link VR#EVREventType_VREvent_ButtonUntouch} {@link VR#EVREventType_VREvent_Modal_Cancel} {@link VR#EVREventType_VREvent_MouseMove} {@link VR#EVREventType_VREvent_MouseButtonDown} {@link VR#EVREventType_VREvent_MouseButtonUp} {@link VR#EVREventType_VREvent_FocusEnter} {@link VR#EVREventType_VREvent_FocusLeave} {@link VR#EVREventType_VREvent_ScrollDiscrete} {@link VR#EVREventType_VREvent_TouchPadMove} {@link VR#EVREventType_VREvent_OverlayFocusChanged} {@link VR#EVREventType_VREvent_ReloadOverlays} {@link VR#EVREventType_VREvent_ScrollSmooth} {@link VR#EVREventType_VREvent_LockMousePosition} {@link VR#EVREventType_VREvent_UnlockMousePosition} {@link VR#EVREventType_VREvent_InputFocusCaptured} {@link VR#EVREventType_VREvent_InputFocusReleased} {@link VR#EVREventType_VREvent_SceneApplicationChanged} {@link VR#EVREventType_VREvent_InputFocusChanged} {@link VR#EVREventType_VREvent_SceneApplicationUsingWrongGraphicsAdapter} {@link VR#EVREventType_VREvent_ActionBindingReloaded} {@link VR#EVREventType_VREvent_HideRenderModels} {@link VR#EVREventType_VREvent_ShowRenderModels} {@link VR#EVREventType_VREvent_SceneApplicationStateChanged} {@link VR#EVREventType_VREvent_SceneAppPipeDisconnected} {@link VR#EVREventType_VREvent_ConsoleOpened} {@link VR#EVREventType_VREvent_ConsoleClosed} {@link VR#EVREventType_VREvent_OverlayShown} {@link VR#EVREventType_VREvent_OverlayHidden} {@link VR#EVREventType_VREvent_DashboardActivated} {@link VR#EVREventType_VREvent_DashboardDeactivated} {@link VR#EVREventType_VREvent_DashboardRequested} {@link VR#EVREventType_VREvent_ResetDashboard} {@link VR#EVREventType_VREvent_ImageLoaded} {@link VR#EVREventType_VREvent_ShowKeyboard} {@link VR#EVREventType_VREvent_HideKeyboard} {@link VR#EVREventType_VREvent_OverlayGamepadFocusGained} {@link VR#EVREventType_VREvent_OverlayGamepadFocusLost} {@link VR#EVREventType_VREvent_OverlaySharedTextureChanged} {@link VR#EVREventType_VREvent_ScreenshotTriggered} {@link VR#EVREventType_VREvent_ImageFailed} {@link VR#EVREventType_VREvent_DashboardOverlayCreated} {@link VR#EVREventType_VREvent_SwitchGamepadFocus} {@link VR#EVREventType_VREvent_RequestScreenshot} {@link VR#EVREventType_VREvent_ScreenshotTaken} {@link VR#EVREventType_VREvent_ScreenshotFailed} {@link VR#EVREventType_VREvent_SubmitScreenshotToDashboard} {@link VR#EVREventType_VREvent_ScreenshotProgressToDashboard} {@link VR#EVREventType_VREvent_PrimaryDashboardDeviceChanged} {@link VR#EVREventType_VREvent_RoomViewShown} {@link VR#EVREventType_VREvent_RoomViewHidden} {@link VR#EVREventType_VREvent_ShowUI} {@link VR#EVREventType_VREvent_ShowDevTools} {@link VR#EVREventType_VREvent_DesktopViewUpdating} {@link VR#EVREventType_VREvent_DesktopViewReady} {@link VR#EVREventType_VREvent_StartDashboard} {@link VR#EVREventType_VREvent_ElevatePrism} {@link VR#EVREventType_VREvent_OverlayClosed} {@link VR#EVREventType_VREvent_DashboardThumbChanged} {@link VR#EVREventType_VREvent_DesktopMightBeVisible} {@link VR#EVREventType_VREvent_DesktopMightBeHidden} {@link VR#EVREventType_VREvent_MutualSteamCapabilitiesChanged} {@link VR#EVREventType_VREvent_OverlayCreated} {@link VR#EVREventType_VREvent_OverlayDestroyed} {@link VR#EVREventType_VREvent_Notification_Shown} {@link VR#EVREventType_VREvent_Notification_Hidden} {@link VR#EVREventType_VREvent_Notification_BeginInteraction} {@link VR#EVREventType_VREvent_Notification_Destroyed} {@link VR#EVREventType_VREvent_Quit} {@link VR#EVREventType_VREvent_ProcessQuit} {@link VR#EVREventType_VREvent_QuitAcknowledged} {@link VR#EVREventType_VREvent_DriverRequestedQuit} {@link VR#EVREventType_VREvent_RestartRequested} {@link VR#EVREventType_VREvent_InvalidateSwapTextureSets} {@link VR#EVREventType_VREvent_ChaperoneDataHasChanged} {@link VR#EVREventType_VREvent_ChaperoneUniverseHasChanged} {@link VR#EVREventType_VREvent_ChaperoneTempDataHasChanged} {@link VR#EVREventType_VREvent_ChaperoneSettingsHaveChanged} {@link VR#EVREventType_VREvent_SeatedZeroPoseReset} {@link VR#EVREventType_VREvent_ChaperoneFlushCache} {@link VR#EVREventType_VREvent_ChaperoneRoomSetupStarting} {@link VR#EVREventType_VREvent_ChaperoneRoomSetupFinished} {@link VR#EVREventType_VREvent_StandingZeroPoseReset} {@link VR#EVREventType_VREvent_AudioSettingsHaveChanged} {@link VR#EVREventType_VREvent_BackgroundSettingHasChanged} {@link VR#EVREventType_VREvent_CameraSettingsHaveChanged} {@link VR#EVREventType_VREvent_ReprojectionSettingHasChanged} {@link VR#EVREventType_VREvent_ModelSkinSettingsHaveChanged} {@link VR#EVREventType_VREvent_EnvironmentSettingsHaveChanged} {@link VR#EVREventType_VREvent_PowerSettingsHaveChanged} {@link VR#EVREventType_VREvent_EnableHomeAppSettingsHaveChanged} {@link VR#EVREventType_VREvent_SteamVRSectionSettingChanged} {@link VR#EVREventType_VREvent_LighthouseSectionSettingChanged} {@link VR#EVREventType_VREvent_NullSectionSettingChanged} {@link VR#EVREventType_VREvent_UserInterfaceSectionSettingChanged} {@link VR#EVREventType_VREvent_NotificationsSectionSettingChanged} {@link VR#EVREventType_VREvent_KeyboardSectionSettingChanged} {@link VR#EVREventType_VREvent_PerfSectionSettingChanged} {@link VR#EVREventType_VREvent_DashboardSectionSettingChanged} {@link VR#EVREventType_VREvent_WebInterfaceSectionSettingChanged} {@link VR#EVREventType_VREvent_TrackersSectionSettingChanged} {@link VR#EVREventType_VREvent_LastKnownSectionSettingChanged} {@link VR#EVREventType_VREvent_DismissedWarningsSectionSettingChanged} {@link VR#EVREventType_VREvent_GpuSpeedSectionSettingChanged} {@link VR#EVREventType_VREvent_WindowsMRSectionSettingChanged} {@link VR#EVREventType_VREvent_OtherSectionSettingChanged} {@link VR#EVREventType_VREvent_AnyDriverSettingsChanged} {@link VR#EVREventType_VREvent_StatusUpdate} {@link VR#EVREventType_VREvent_WebInterface_InstallDriverCompleted} {@link VR#EVREventType_VREvent_MCImageUpdated} {@link VR#EVREventType_VREvent_FirmwareUpdateStarted} {@link VR#EVREventType_VREvent_FirmwareUpdateFinished} {@link VR#EVREventType_VREvent_KeyboardClosed} {@link VR#EVREventType_VREvent_KeyboardCharInput} {@link VR#EVREventType_VREvent_KeyboardDone} {@link VR#EVREventType_VREvent_KeyboardOpened_Global} {@link VR#EVREventType_VREvent_KeyboardClosed_Global} {@link VR#EVREventType_VREvent_ApplicationListUpdated} {@link VR#EVREventType_VREvent_ApplicationMimeTypeLoad} {@link VR#EVREventType_VREvent_ProcessConnected} {@link VR#EVREventType_VREvent_ProcessDisconnected} {@link VR#EVREventType_VREvent_Compositor_ChaperoneBoundsShown} {@link VR#EVREventType_VREvent_Compositor_ChaperoneBoundsHidden} {@link VR#EVREventType_VREvent_Compositor_DisplayDisconnected} {@link VR#EVREventType_VREvent_Compositor_DisplayReconnected} {@link VR#EVREventType_VREvent_Compositor_HDCPError} {@link VR#EVREventType_VREvent_Compositor_ApplicationNotResponding} {@link VR#EVREventType_VREvent_Compositor_ApplicationResumed} {@link VR#EVREventType_VREvent_Compositor_OutOfVideoMemory} {@link VR#EVREventType_VREvent_Compositor_DisplayModeNotSupported} {@link VR#EVREventType_VREvent_Compositor_StageOverrideReady} {@link VR#EVREventType_VREvent_Compositor_RequestDisconnectReconnect} {@link VR#EVREventType_VREvent_TrackedCamera_StartVideoStream} {@link VR#EVREventType_VREvent_TrackedCamera_StopVideoStream} {@link VR#EVREventType_VREvent_TrackedCamera_PauseVideoStream} {@link VR#EVREventType_VREvent_TrackedCamera_ResumeVideoStream} {@link VR#EVREventType_VREvent_TrackedCamera_EditingSurface} {@link VR#EVREventType_VREvent_PerformanceTest_EnableCapture} {@link VR#EVREventType_VREvent_PerformanceTest_DisableCapture} {@link VR#EVREventType_VREvent_PerformanceTest_FidelityLevel} {@link VR#EVREventType_VREvent_MessageOverlay_Closed} {@link VR#EVREventType_VREvent_MessageOverlayCloseRequested} {@link VR#EVREventType_VREvent_Input_HapticVibration} {@link VR#EVREventType_VREvent_Input_BindingLoadFailed} {@link VR#EVREventType_VREvent_Input_BindingLoadSuccessful} {@link VR#EVREventType_VREvent_Input_ActionManifestReloaded} {@link VR#EVREventType_VREvent_Input_ActionManifestLoadFailed} {@link VR#EVREventType_VREvent_Input_ProgressUpdate} {@link VR#EVREventType_VREvent_Input_TrackerActivated} {@link VR#EVREventType_VREvent_Input_BindingsUpdated} {@link VR#EVREventType_VREvent_Input_BindingSubscriptionChanged} {@link VR#EVREventType_VREvent_SpatialAnchors_PoseUpdated} {@link VR#EVREventType_VREvent_SpatialAnchors_DescriptorUpdated} {@link VR#EVREventType_VREvent_SpatialAnchors_RequestPoseUpdate} {@link VR#EVREventType_VREvent_SpatialAnchors_RequestDescriptorUpdate} {@link VR#EVREventType_VREvent_SystemReport_Started} {@link VR#EVREventType_VREvent_Monitor_ShowHeadsetView} {@link VR#EVREventType_VREvent_Monitor_HideHeadsetView} {@link VR#EVREventType_VREvent_Audio_SetSpeakersVolume} {@link VR#EVREventType_VREvent_Audio_SetSpeakersMute} {@link VR#EVREventType_VREvent_Audio_SetMicrophoneVolume} {@link VR#EVREventType_VREvent_Audio_SetMicrophoneMute} {@link VR#EVREventType_VREvent_VendorSpecific_Reserved_Start} {@link VR#EVREventType_VREvent_VendorSpecific_Reserved_End}
*/
@NativeType("uint32_t")
public int eventType() { return neventType(address()); }
/** the tracked device index of the event. For events that aren't connected to a tracked device this is {@link VR#k_unTrackedDeviceIndexInvalid}. */
@NativeType("TrackedDeviceIndex_t")
public int trackedDeviceIndex() { return ntrackedDeviceIndex(address()); }
/** the age of the event in seconds */
public float eventAgeSeconds() { return neventAgeSeconds(address()); }
/**
* more information about the event. This is a union of several structs. See the event type enum for information about which union member to look at for
* each event.
*/
@NativeType("VREvent_Data_t")
public VREventData data() { return ndata(address()); }
// -----------------------------------
/** Returns a new {@code VREvent} instance allocated with {@link MemoryUtil#memAlloc memAlloc}. The instance must be explicitly freed. */
public static VREvent malloc() {
return new VREvent(nmemAllocChecked(SIZEOF), null);
}
/** Returns a new {@code VREvent} instance allocated with {@link MemoryUtil#memCalloc memCalloc}. The instance must be explicitly freed. */
public static VREvent calloc() {
return new VREvent(nmemCallocChecked(1, SIZEOF), null);
}
/** Returns a new {@code VREvent} instance allocated with {@link BufferUtils}. */
public static VREvent create() {
ByteBuffer container = BufferUtils.createByteBuffer(SIZEOF);
return new VREvent(memAddress(container), container);
}
/** Returns a new {@code VREvent} instance for the specified memory address. */
public static VREvent create(long address) {
return new VREvent(address, null);
}
/** Like {@link #create(long) create}, but returns {@code null} if {@code address} is {@code NULL}. */
public static @Nullable VREvent createSafe(long address) {
return address == NULL ? null : new VREvent(address, null);
}
/**
* Returns a new {@link VREvent.Buffer} instance allocated with {@link MemoryUtil#memAlloc memAlloc}. The instance must be explicitly freed.
*
* @param capacity the buffer capacity
*/
public static VREvent.Buffer malloc(int capacity) {
return new Buffer(nmemAllocChecked(__checkMalloc(capacity, SIZEOF)), capacity);
}
/**
* Returns a new {@link VREvent.Buffer} instance allocated with {@link MemoryUtil#memCalloc memCalloc}. The instance must be explicitly freed.
*
* @param capacity the buffer capacity
*/
public static VREvent.Buffer calloc(int capacity) {
return new Buffer(nmemCallocChecked(capacity, SIZEOF), capacity);
}
/**
* Returns a new {@link VREvent.Buffer} instance allocated with {@link BufferUtils}.
*
* @param capacity the buffer capacity
*/
public static VREvent.Buffer create(int capacity) {
ByteBuffer container = __create(capacity, SIZEOF);
return new Buffer(memAddress(container), container, -1, 0, capacity, capacity);
}
/**
* Create a {@link VREvent.Buffer} instance at the specified memory.
*
* @param address the memory address
* @param capacity the buffer capacity
*/
public static VREvent.Buffer create(long address, int capacity) {
return new Buffer(address, capacity);
}
/** Like {@link #create(long, int) create}, but returns {@code null} if {@code address} is {@code NULL}. */
public static VREvent.@Nullable Buffer createSafe(long address, int capacity) {
return address == NULL ? null : new Buffer(address, capacity);
}
// -----------------------------------
/** Deprecated for removal in 3.4.0. Use {@link #malloc(MemoryStack)} instead. */
@Deprecated public static VREvent mallocStack() { return malloc(stackGet()); }
/** Deprecated for removal in 3.4.0. Use {@link #calloc(MemoryStack)} instead. */
@Deprecated public static VREvent callocStack() { return calloc(stackGet()); }
/** Deprecated for removal in 3.4.0. Use {@link #malloc(MemoryStack)} instead. */
@Deprecated public static VREvent mallocStack(MemoryStack stack) { return malloc(stack); }
/** Deprecated for removal in 3.4.0. Use {@link #calloc(MemoryStack)} instead. */
@Deprecated public static VREvent callocStack(MemoryStack stack) { return calloc(stack); }
/** Deprecated for removal in 3.4.0. Use {@link #malloc(int, MemoryStack)} instead. */
@Deprecated public static VREvent.Buffer mallocStack(int capacity) { return malloc(capacity, stackGet()); }
/** Deprecated for removal in 3.4.0. Use {@link #calloc(int, MemoryStack)} instead. */
@Deprecated public static VREvent.Buffer callocStack(int capacity) { return calloc(capacity, stackGet()); }
/** Deprecated for removal in 3.4.0. Use {@link #malloc(int, MemoryStack)} instead. */
@Deprecated public static VREvent.Buffer mallocStack(int capacity, MemoryStack stack) { return malloc(capacity, stack); }
/** Deprecated for removal in 3.4.0. Use {@link #calloc(int, MemoryStack)} instead. */
@Deprecated public static VREvent.Buffer callocStack(int capacity, MemoryStack stack) { return calloc(capacity, stack); }
/**
* Returns a new {@code VREvent} instance allocated on the specified {@link MemoryStack}.
*
* @param stack the stack from which to allocate
*/
public static VREvent malloc(MemoryStack stack) {
return new VREvent(stack.nmalloc(ALIGNOF, SIZEOF), null);
}
/**
* Returns a new {@code VREvent} instance allocated on the specified {@link MemoryStack} and initializes all its bits to zero.
*
* @param stack the stack from which to allocate
*/
public static VREvent calloc(MemoryStack stack) {
return new VREvent(stack.ncalloc(ALIGNOF, 1, SIZEOF), null);
}
/**
* Returns a new {@link VREvent.Buffer} instance allocated on the specified {@link MemoryStack}.
*
* @param stack the stack from which to allocate
* @param capacity the buffer capacity
*/
public static VREvent.Buffer malloc(int capacity, MemoryStack stack) {
return new Buffer(stack.nmalloc(ALIGNOF, capacity * SIZEOF), capacity);
}
/**
* Returns a new {@link VREvent.Buffer} instance allocated on the specified {@link MemoryStack} and initializes all its bits to zero.
*
* @param stack the stack from which to allocate
* @param capacity the buffer capacity
*/
public static VREvent.Buffer calloc(int capacity, MemoryStack stack) {
return new Buffer(stack.ncalloc(ALIGNOF, capacity, SIZEOF), capacity);
}
// -----------------------------------
/** Unsafe version of {@link #eventType}. */
public static int neventType(long struct) { return memGetInt(struct + VREvent.EVENTTYPE); }
/** Unsafe version of {@link #trackedDeviceIndex}. */
public static int ntrackedDeviceIndex(long struct) { return memGetInt(struct + VREvent.TRACKEDDEVICEINDEX); }
/** Unsafe version of {@link #eventAgeSeconds}. */
public static float neventAgeSeconds(long struct) { return memGetFloat(struct + VREvent.EVENTAGESECONDS); }
/** Unsafe version of {@link #data}. */
public static VREventData ndata(long struct) { return VREventData.create(struct + VREvent.DATA); }
// -----------------------------------
/** An array of {@link VREvent} structs. */
public static class Buffer extends StructBuffer implements NativeResource {
private static final VREvent ELEMENT_FACTORY = VREvent.create(-1L);
/**
* Creates a new {@code VREvent.Buffer} instance backed by the specified container.
*
* Changes to the container's content will be visible to the struct buffer instance and vice versa. The two buffers' position, limit, and mark values
* will be independent. The new buffer's position will be zero, its capacity and its limit will be the number of bytes remaining in this buffer divided
* by {@link VREvent#SIZEOF}, and its mark will be undefined.
*
* The created buffer instance holds a strong reference to the container object.
*/
public Buffer(ByteBuffer container) {
super(container, container.remaining() / SIZEOF);
}
public Buffer(long address, int cap) {
super(address, null, -1, 0, cap, cap);
}
Buffer(long address, @Nullable ByteBuffer container, int mark, int pos, int lim, int cap) {
super(address, container, mark, pos, lim, cap);
}
@Override
protected Buffer self() {
return this;
}
@Override
protected Buffer create(long address, @Nullable ByteBuffer container, int mark, int position, int limit, int capacity) {
return new Buffer(address, container, mark, position, limit, capacity);
}
@Override
protected VREvent getElementFactory() {
return ELEMENT_FACTORY;
}
/** @return the value of the {@link VREvent#eventType} field. */
@NativeType("uint32_t")
public int eventType() { return VREvent.neventType(address()); }
/** @return the value of the {@link VREvent#trackedDeviceIndex} field. */
@NativeType("TrackedDeviceIndex_t")
public int trackedDeviceIndex() { return VREvent.ntrackedDeviceIndex(address()); }
/** @return the value of the {@link VREvent#eventAgeSeconds} field. */
public float eventAgeSeconds() { return VREvent.neventAgeSeconds(address()); }
/** @return a {@link VREventData} view of the {@link VREvent#data} field. */
@NativeType("VREvent_Data_t")
public VREventData data() { return VREvent.ndata(address()); }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy