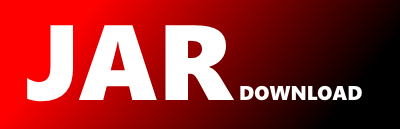
org.lwjgl.openxr.ALMALENCEDigitalLensControl Maven / Gradle / Ivy
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The XR_ALMALENCE_digital_lens_control extension.
*
* Digital Lens for VR (DLVR) is a computational lens aberration correction technology enabling high resolution, visual clarity and fidelity in VR head mounted displays. The Digital Lens allows to overcome two fundamental factors limiting VR picture quality, size constraints and presence of a moving optical element — the eye pupil.
*
* Features:
*
*
* - Complete removal of lateral chromatic aberrations, across the entire FoV, at all gaze directions.
* - Correction of longitudinal chromatic aberrations, lens blur and higher order aberrations.
* - Increase of visible resolution.
* - Enhancement of edge contrast (otherwise degraded due to lens smear).
* - Enables high quality at wide FoV.
*
*
* For OpenXR runtimes DLVR is implemented as implicit API Layer distributed by Almalence Inc. as installable package. DLVR utilize eye tracking data (eye pupil coordinates and gaze direction) to produce corrections of render frames. As long as current core OpenXR API does not expose an eye tracking data, DLVR API Layer relies on 3rd-party eye tracking runtimes.
*
* List of supported eye tracking devices:
*
*
* - Tobii_VR4_CARBON_P1 (HP Reverb G2 Omnicept Edition)
* - Tobii_VR4_U2_P2 (HTC Vive Pro Eye)
*
*
* This extension enables the handling of the Digital Lens for VR API Layer by calling {@link #xrSetDigitalLensControlALMALENCE SetDigitalLensControlALMALENCE}.
*/
public class ALMALENCEDigitalLensControl {
/** The extension specification version. */
public static final int XR_ALMALENCE_digital_lens_control_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_ALMALENCE_DIGITAL_LENS_CONTROL_EXTENSION_NAME = "XR_ALMALENCE_digital_lens_control";
/** Extends {@code XrStructureType}. */
public static final int XR_TYPE_DIGITAL_LENS_CONTROL_ALMALENCE = 1000196000;
/**
* XrDigitalLensControlFlagBitsALMALENCE - XrDigitalLensControlFlagBitsALMALENCE
*
* Flag Descriptions
*
*
* - {@link #XR_DIGITAL_LENS_CONTROL_PROCESSING_DISABLE_BIT_ALMALENCE DIGITAL_LENS_CONTROL_PROCESSING_DISABLE_BIT_ALMALENCE} — disables Digital Lens processing of render textures
*
*/
public static final int XR_DIGITAL_LENS_CONTROL_PROCESSING_DISABLE_BIT_ALMALENCE = 0x1;
protected ALMALENCEDigitalLensControl() {
throw new UnsupportedOperationException();
}
// --- [ xrSetDigitalLensControlALMALENCE ] ---
/** Unsafe version of: {@link #xrSetDigitalLensControlALMALENCE SetDigitalLensControlALMALENCE} */
public static int nxrSetDigitalLensControlALMALENCE(XrSession session, long digitalLensControl) {
long __functionAddress = session.getCapabilities().xrSetDigitalLensControlALMALENCE;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(session.address(), digitalLensControl, __functionAddress);
}
/**
* Sets DLVR status.
*
* C Specification
*
* The {@link #xrSetDigitalLensControlALMALENCE SetDigitalLensControlALMALENCE} function is defined as:
*
*
* XrResult xrSetDigitalLensControlALMALENCE(
* XrSession session,
* const XrDigitalLensControlALMALENCE* digitalLensControl);
*
* Description
*
* {@link #xrSetDigitalLensControlALMALENCE SetDigitalLensControlALMALENCE} handles state of Digital Lens API Layer
*
* Valid Usage (Implicit)
*
*
* - The {@link ALMALENCEDigitalLensControl XR_ALMALENCE_digital_lens_control} extension must be enabled prior to calling {@link #xrSetDigitalLensControlALMALENCE SetDigitalLensControlALMALENCE}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code digitalLensControl} must be a pointer to a valid {@link XrDigitalLensControlALMALENCE} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link XrDigitalLensControlALMALENCE}
*
* @param session a handle to a running {@code XrSession}.
* @param digitalLensControl the {@link XrDigitalLensControlALMALENCE} that contains desired characteristics for the Digital Lens
*/
@NativeType("XrResult")
public static int xrSetDigitalLensControlALMALENCE(XrSession session, @NativeType("XrDigitalLensControlALMALENCE const *") XrDigitalLensControlALMALENCE digitalLensControl) {
return nxrSetDigitalLensControlALMALENCE(session, digitalLensControl.address());
}
}