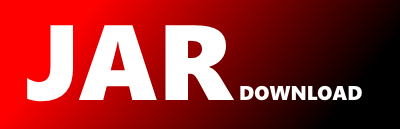
org.lwjgl.openxr.EXTConformanceAutomation Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import org.lwjgl.system.libffi.*;
import static org.lwjgl.system.APIUtil.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.libffi.LibFFI.*;
import static org.lwjgl.system.MemoryStack.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_EXT_conformance_automation extension.
*
* The XR_EXT_conformance_automation allows conformance test and runtime developers to provide hints to the underlying runtime as to what input the test is expecting. This enables runtime authors to automate the testing of their runtime conformance. This is useful for achieving rapidly iterative runtime development whilst maintaining conformance for runtime releases.
*
* This extension provides the following capabilities:
*
*
* - The ability to toggle the active state of an input device.
* - The ability to set the state of an input device button or other input component.
* - The ability to set the location of the input device.
*
*
* Applications may call these functions at any time. The runtime must do its best to honor the request of applications calling these functions, however it does not guarantee that any state change will be reflected immediately, at all, or with the exact value that was requested. Applications are thus advised to wait for the state change to be observable and to not assume that the value they requested will be the value observed. If any of the functions of this extension are called, control over input must be removed from the physical hardware of the system.
*
* Warning
*
* This extension is not intended for use by non-conformance-test applications. A runtime may require a runtime-specified configuration such as a "developer mode" to be enabled before reporting support for this extension or providing a non-stub implementation of it.
*
* Do not use this functionality in a non-conformance-test application!
*/
public class EXTConformanceAutomation {
/** The extension specification version. */
public static final int XR_EXT_conformance_automation_SPEC_VERSION = 3;
/** The extension name. */
public static final String XR_EXT_CONFORMANCE_AUTOMATION_EXTENSION_NAME = "XR_EXT_conformance_automation";
protected EXTConformanceAutomation() {
throw new UnsupportedOperationException();
}
// --- [ xrSetInputDeviceActiveEXT ] ---
/**
* Sets the active state of the input device.
*
* C Specification
*
*
* XrResult xrSetInputDeviceActiveEXT(
* XrSession session,
* XrPath interactionProfile,
* XrPath topLevelPath,
* XrBool32 isActive);
*
* Valid Usage
*
*
* - {@code session} must be a valid session handle.
* - {@code topLevelPath} must be a valid top level path.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTConformanceAutomation XR_EXT_conformance_automation} extension must be enabled prior to calling {@link #xrSetInputDeviceActiveEXT SetInputDeviceActiveEXT}
* - {@code session} must be a valid {@code XrSession} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
*
*
*
* @param session the {@code XrSession} to set the input device state in.
* @param interactionProfile the path representing the interaction profile of the input device (e.g. pathname:/interaction_profiles/khr/simple_controller).
* @param topLevelPath the path representing the input device (e.g. pathname:/user/hand/left).
* @param isActive the requested activation state of the input device.
*/
@NativeType("XrResult")
public static int xrSetInputDeviceActiveEXT(XrSession session, @NativeType("XrPath") long interactionProfile, @NativeType("XrPath") long topLevelPath, @NativeType("XrBool32") boolean isActive) {
long __functionAddress = session.getCapabilities().xrSetInputDeviceActiveEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPJJI(session.address(), interactionProfile, topLevelPath, isActive ? 1 : 0, __functionAddress);
}
// --- [ xrSetInputDeviceStateBoolEXT ] ---
/**
* Sets the state of a boolean input source on the input device.
*
* C Specification
*
*
* XrResult xrSetInputDeviceStateBoolEXT(
* XrSession session,
* XrPath topLevelPath,
* XrPath inputSourcePath,
* XrBool32 state);
*
* Valid Usage
*
*
* - {@code session} must be a valid session handle.
* - {@code topLevelPath} must be a valid top level path.
* - {@code inputSourcePath} must be a valid input source path.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTConformanceAutomation XR_EXT_conformance_automation} extension must be enabled prior to calling {@link #xrSetInputDeviceStateBoolEXT SetInputDeviceStateBoolEXT}
* - {@code session} must be a valid {@code XrSession} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
*
*
*
* @param session the {@code XrSession} to set the input device state in.
* @param topLevelPath the path representing the input device (e.g. pathname:/user/hand/left).
* @param inputSourcePath the full path of the input component for which we wish to set the state for (e.g. pathname:/user/hand/left/input/select/click).
* @param state the requested boolean state of the input device.
*/
@NativeType("XrResult")
public static int xrSetInputDeviceStateBoolEXT(XrSession session, @NativeType("XrPath") long topLevelPath, @NativeType("XrPath") long inputSourcePath, @NativeType("XrBool32") boolean state) {
long __functionAddress = session.getCapabilities().xrSetInputDeviceStateBoolEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPJJI(session.address(), topLevelPath, inputSourcePath, state ? 1 : 0, __functionAddress);
}
// --- [ xrSetInputDeviceStateFloatEXT ] ---
/**
* Sets the state of a float input source on the input device.
*
* C Specification
*
*
* XrResult xrSetInputDeviceStateFloatEXT(
* XrSession session,
* XrPath topLevelPath,
* XrPath inputSourcePath,
* float state);
*
* Valid Usage
*
*
* - {@code session} must be a valid session handle.
* - {@code topLevelPath} must be a valid top level path.
* - {@code inputSourcePath} must be a valid input source path.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTConformanceAutomation XR_EXT_conformance_automation} extension must be enabled prior to calling {@link #xrSetInputDeviceStateFloatEXT SetInputDeviceStateFloatEXT}
* - {@code session} must be a valid {@code XrSession} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
*
*
*
* @param session the {@code XrSession} to set the input device state in.
* @param topLevelPath the path representing the input device (e.g. pathname:/user/hand/left).
* @param inputSourcePath the full path of the input component for which we wish to set the state for (e.g. pathname:/user/hand/left/input/trigger/value).
* @param state the requested float state of the input device.
*/
@NativeType("XrResult")
public static int xrSetInputDeviceStateFloatEXT(XrSession session, @NativeType("XrPath") long topLevelPath, @NativeType("XrPath") long inputSourcePath, float state) {
long __functionAddress = session.getCapabilities().xrSetInputDeviceStateFloatEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPJJI(session.address(), topLevelPath, inputSourcePath, state, __functionAddress);
}
// --- [ xrSetInputDeviceStateVector2fEXT ] ---
private static final FFICIF xrSetInputDeviceStateVector2fEXTCIF = apiCreateCIF(
apiStdcall(), ffi_type_uint32,
ffi_type_pointer, ffi_type_uint64, ffi_type_uint64, apiCreateStruct(ffi_type_float, ffi_type_float)
);
/** Unsafe version of: {@link #xrSetInputDeviceStateVector2fEXT SetInputDeviceStateVector2fEXT} */
public static int nxrSetInputDeviceStateVector2fEXT(XrSession session, long topLevelPath, long inputSourcePath, long state) {
long __functionAddress = session.getCapabilities().xrSetInputDeviceStateVector2fEXT;
if (CHECKS) {
check(__functionAddress);
}
MemoryStack stack = stackGet(); int stackPointer = stack.getPointer();
try {
long __result = stack.nint(0);
long arguments = stack.nmalloc(POINTER_SIZE, POINTER_SIZE * 4);
memPutAddress(arguments, stack.npointer(session));
memPutAddress(arguments + POINTER_SIZE, stack.nlong(topLevelPath));
memPutAddress(arguments + 2 * POINTER_SIZE, stack.nlong(inputSourcePath));
memPutAddress(arguments + 3 * POINTER_SIZE, state);
nffi_call(xrSetInputDeviceStateVector2fEXTCIF.address(), __functionAddress, __result, arguments);
return memGetInt(__result);
} finally {
stack.setPointer(stackPointer);
}
}
/**
* Sets the state of a 2D vector input source on the input device.
*
* C Specification
*
*
* XrResult xrSetInputDeviceStateVector2fEXT(
* XrSession session,
* XrPath topLevelPath,
* XrPath inputSourcePath,
* XrVector2f state);
*
* Valid Usage
*
*
* - {@code session} must be a valid session handle.
* - {@code topLevelPath} must be a valid top level path.
* - {@code inputSourcePath} must be a valid input source path.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTConformanceAutomation XR_EXT_conformance_automation} extension must be enabled prior to calling {@link #xrSetInputDeviceStateVector2fEXT SetInputDeviceStateVector2fEXT}
* - {@code session} must be a valid {@code XrSession} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
*
*
*
* See Also
*
* {@link XrVector2f}
*
* @param session the {@code XrSession} to set the input device state in.
* @param topLevelPath the path representing the input device (e.g. pathname:/user/hand/left).
* @param inputSourcePath the full path of the input component for which we wish to set the state for (e.g. pathname:/user/hand/left/input/thumbstick).
* @param state the requested two-dimensional state of the input device.
*/
@NativeType("XrResult")
public static int xrSetInputDeviceStateVector2fEXT(XrSession session, @NativeType("XrPath") long topLevelPath, @NativeType("XrPath") long inputSourcePath, XrVector2f state) {
return nxrSetInputDeviceStateVector2fEXT(session, topLevelPath, inputSourcePath, state.address());
}
// --- [ xrSetInputDeviceLocationEXT ] ---
private static final FFICIF xrSetInputDeviceLocationEXTCIF = apiCreateCIF(
apiStdcall(), ffi_type_uint32,
ffi_type_pointer, ffi_type_uint64, ffi_type_uint64, ffi_type_pointer, apiCreateStruct(apiCreateStruct(ffi_type_float, ffi_type_float, ffi_type_float, ffi_type_float), apiCreateStruct(ffi_type_float, ffi_type_float, ffi_type_float))
);
/** Unsafe version of: {@link #xrSetInputDeviceLocationEXT SetInputDeviceLocationEXT} */
public static int nxrSetInputDeviceLocationEXT(XrSession session, long topLevelPath, long inputSourcePath, XrSpace space, long pose) {
long __functionAddress = session.getCapabilities().xrSetInputDeviceLocationEXT;
if (CHECKS) {
check(__functionAddress);
}
MemoryStack stack = stackGet(); int stackPointer = stack.getPointer();
try {
long __result = stack.nint(0);
long arguments = stack.nmalloc(POINTER_SIZE, POINTER_SIZE * 5);
memPutAddress(arguments, stack.npointer(session));
memPutAddress(arguments + POINTER_SIZE, stack.nlong(topLevelPath));
memPutAddress(arguments + 2 * POINTER_SIZE, stack.nlong(inputSourcePath));
memPutAddress(arguments + 3 * POINTER_SIZE, stack.npointer(space));
memPutAddress(arguments + 4 * POINTER_SIZE, pose);
nffi_call(xrSetInputDeviceLocationEXTCIF.address(), __functionAddress, __result, arguments);
return memGetInt(__result);
} finally {
stack.setPointer(stackPointer);
}
}
/**
* Sets the effective location of a pose input source on the input device.
*
* C Specification
*
*
* XrResult xrSetInputDeviceLocationEXT(
* XrSession session,
* XrPath topLevelPath,
* XrPath inputSourcePath,
* XrSpace space,
* XrPosef pose);
*
* Valid Usage
*
*
* - {@code session} must be a valid session handle.
* - {@code topLevelPath} must be a valid top level path.
* - {@code inputSourcePath} must be a valid input source path.
* - {@code space} must be a valid {@code XrSpace}.
* - {@code pose} must be a valid {@link XrPosef}.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTConformanceAutomation XR_EXT_conformance_automation} extension must be enabled prior to calling {@link #xrSetInputDeviceLocationEXT SetInputDeviceLocationEXT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code space} must be a valid {@code XrSpace} handle
* - {@code space} must have been created, allocated, or retrieved from {@code session}
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
*
*
*
* See Also
*
* {@link XrPosef}
*
* @param session the {@code XrSession} to set the input device state in.
* @param topLevelPath the path representing the input device (e.g. pathname:/user/hand/left).
* @param inputSourcePath the full path of the input component for which we wish to set the pose for (e.g. pathname:/user/hand/left/input/grip/pose).
* @param pose the requested pose state of the input device.
*/
@NativeType("XrResult")
public static int xrSetInputDeviceLocationEXT(XrSession session, @NativeType("XrPath") long topLevelPath, @NativeType("XrPath") long inputSourcePath, XrSpace space, XrPosef pose) {
return nxrSetInputDeviceLocationEXT(session, topLevelPath, inputSourcePath, space, pose.address());
}
}