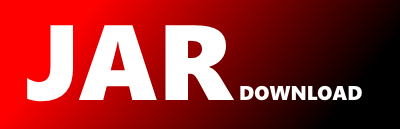
org.lwjgl.openxr.EXTDebugUtils Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_EXT_debug_utils extension.
*
* Due to the nature of the OpenXR interface, there is very little error information available to the developer and application. By using the {@link EXTDebugUtils XR_EXT_debug_utils} extension, developers can obtain more information. When combined with validation layers, even more detailed feedback on the application’s use of OpenXR will be provided.
*
* This extension provides the following capabilities:
*
*
* - The ability to create a debug messenger which will pass along debug messages to an application supplied callback.
* - The ability to identify specific OpenXR handles using a name to improve tracking.
*
*/
public class EXTDebugUtils {
/** The extension specification version. */
public static final int XR_EXT_debug_utils_SPEC_VERSION = 5;
/** The extension name. */
public static final String XR_EXT_DEBUG_UTILS_EXTENSION_NAME = "XR_EXT_debug_utils";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_DEBUG_UTILS_OBJECT_NAME_INFO_EXT TYPE_DEBUG_UTILS_OBJECT_NAME_INFO_EXT}
* - {@link #XR_TYPE_DEBUG_UTILS_MESSENGER_CALLBACK_DATA_EXT TYPE_DEBUG_UTILS_MESSENGER_CALLBACK_DATA_EXT}
* - {@link #XR_TYPE_DEBUG_UTILS_MESSENGER_CREATE_INFO_EXT TYPE_DEBUG_UTILS_MESSENGER_CREATE_INFO_EXT}
* - {@link #XR_TYPE_DEBUG_UTILS_LABEL_EXT TYPE_DEBUG_UTILS_LABEL_EXT}
*
*/
public static final int
XR_TYPE_DEBUG_UTILS_OBJECT_NAME_INFO_EXT = 1000019000,
XR_TYPE_DEBUG_UTILS_MESSENGER_CALLBACK_DATA_EXT = 1000019001,
XR_TYPE_DEBUG_UTILS_MESSENGER_CREATE_INFO_EXT = 1000019002,
XR_TYPE_DEBUG_UTILS_LABEL_EXT = 1000019003;
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_DEBUG_UTILS_MESSENGER_EXT = 1000019000;
/**
* XrDebugUtilsMessageSeverityFlagBitsEXT - XrDebugUtilsMessageSeverityFlagBitsEXT
*
* Enum values:
*
*
* - {@link #XR_DEBUG_UTILS_MESSAGE_SEVERITY_VERBOSE_BIT_EXT DEBUG_UTILS_MESSAGE_SEVERITY_VERBOSE_BIT_EXT}
* - {@link #XR_DEBUG_UTILS_MESSAGE_SEVERITY_INFO_BIT_EXT DEBUG_UTILS_MESSAGE_SEVERITY_INFO_BIT_EXT}
* - {@link #XR_DEBUG_UTILS_MESSAGE_SEVERITY_WARNING_BIT_EXT DEBUG_UTILS_MESSAGE_SEVERITY_WARNING_BIT_EXT}
* - {@link #XR_DEBUG_UTILS_MESSAGE_SEVERITY_ERROR_BIT_EXT DEBUG_UTILS_MESSAGE_SEVERITY_ERROR_BIT_EXT}
*
*/
public static final int
XR_DEBUG_UTILS_MESSAGE_SEVERITY_VERBOSE_BIT_EXT = 0x1,
XR_DEBUG_UTILS_MESSAGE_SEVERITY_INFO_BIT_EXT = 0x10,
XR_DEBUG_UTILS_MESSAGE_SEVERITY_WARNING_BIT_EXT = 0x100,
XR_DEBUG_UTILS_MESSAGE_SEVERITY_ERROR_BIT_EXT = 0x1000;
/**
* XrDebugUtilsMessageTypeFlagBitsEXT - XrDebugUtilsMessageTypeFlagBitsEXT
*
* Enum values:
*
*
* - {@link #XR_DEBUG_UTILS_MESSAGE_TYPE_GENERAL_BIT_EXT DEBUG_UTILS_MESSAGE_TYPE_GENERAL_BIT_EXT}
* - {@link #XR_DEBUG_UTILS_MESSAGE_TYPE_VALIDATION_BIT_EXT DEBUG_UTILS_MESSAGE_TYPE_VALIDATION_BIT_EXT}
* - {@link #XR_DEBUG_UTILS_MESSAGE_TYPE_PERFORMANCE_BIT_EXT DEBUG_UTILS_MESSAGE_TYPE_PERFORMANCE_BIT_EXT}
* - {@link #XR_DEBUG_UTILS_MESSAGE_TYPE_CONFORMANCE_BIT_EXT DEBUG_UTILS_MESSAGE_TYPE_CONFORMANCE_BIT_EXT}
*
*/
public static final int
XR_DEBUG_UTILS_MESSAGE_TYPE_GENERAL_BIT_EXT = 0x1,
XR_DEBUG_UTILS_MESSAGE_TYPE_VALIDATION_BIT_EXT = 0x2,
XR_DEBUG_UTILS_MESSAGE_TYPE_PERFORMANCE_BIT_EXT = 0x4,
XR_DEBUG_UTILS_MESSAGE_TYPE_CONFORMANCE_BIT_EXT = 0x8;
protected EXTDebugUtils() {
throw new UnsupportedOperationException();
}
// --- [ xrSetDebugUtilsObjectNameEXT ] ---
/** Unsafe version of: {@link #xrSetDebugUtilsObjectNameEXT SetDebugUtilsObjectNameEXT} */
public static int nxrSetDebugUtilsObjectNameEXT(XrInstance instance, long nameInfo) {
long __functionAddress = instance.getCapabilities().xrSetDebugUtilsObjectNameEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(instance.address(), nameInfo, __functionAddress);
}
/**
* Sets debug utils object name.
*
* C Specification
*
*
* XrResult xrSetDebugUtilsObjectNameEXT(
* XrInstance instance,
* const XrDebugUtilsObjectNameInfoEXT* nameInfo);
*
* Valid Usage
*
*
* - In the structure pointed to by {@code nameInfo}, {@link XrDebugUtilsObjectNameInfoEXT}{@code ::objectType} must not be {@link XR10#XR_OBJECT_TYPE_UNKNOWN OBJECT_TYPE_UNKNOWN}
* - In the structure pointed to by {@code nameInfo}, {@link XrDebugUtilsObjectNameInfoEXT}{@code ::objectHandle} must not be {@link XR10#XR_NULL_HANDLE NULL_HANDLE}
*
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTDebugUtils XR_EXT_debug_utils} extension must be enabled prior to calling {@link #xrSetDebugUtilsObjectNameEXT SetDebugUtilsObjectNameEXT}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code nameInfo} must be a pointer to a valid {@link XrDebugUtilsObjectNameInfoEXT} structure
*
*
* Thread Safety
*
*
* - Access to the {@code objectHandle} member of the {@code nameInfo} parameter must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
*
*
*
* Applications may change the name associated with an object simply by calling {@link #xrSetDebugUtilsObjectNameEXT SetDebugUtilsObjectNameEXT} again with a new string. If {@link XrDebugUtilsObjectNameInfoEXT}{@code ::objectName} is an empty string, then any previously set name is removed.
*
* See Also
*
* {@link XrDebugUtilsObjectNameInfoEXT}
*
* @param instance the {@code XrInstance} that the object was created under.
* @param nameInfo a pointer to an instance of the {@link XrDebugUtilsObjectNameInfoEXT} structure specifying the parameters of the name to set on the object.
*/
@NativeType("XrResult")
public static int xrSetDebugUtilsObjectNameEXT(XrInstance instance, @NativeType("XrDebugUtilsObjectNameInfoEXT const *") XrDebugUtilsObjectNameInfoEXT nameInfo) {
return nxrSetDebugUtilsObjectNameEXT(instance, nameInfo.address());
}
// --- [ xrCreateDebugUtilsMessengerEXT ] ---
/** Unsafe version of: {@link #xrCreateDebugUtilsMessengerEXT CreateDebugUtilsMessengerEXT} */
public static int nxrCreateDebugUtilsMessengerEXT(XrInstance instance, long createInfo, long messenger) {
long __functionAddress = instance.getCapabilities().xrCreateDebugUtilsMessengerEXT;
if (CHECKS) {
check(__functionAddress);
XrDebugUtilsMessengerCreateInfoEXT.validate(createInfo);
}
return callPPPI(instance.address(), createInfo, messenger, __functionAddress);
}
/**
* Creates a debug messenger.
*
* C Specification
*
*
* XrResult xrCreateDebugUtilsMessengerEXT(
* XrInstance instance,
* const XrDebugUtilsMessengerCreateInfoEXT* createInfo,
* XrDebugUtilsMessengerEXT* messenger);
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTDebugUtils XR_EXT_debug_utils} extension must be enabled prior to calling {@link #xrCreateDebugUtilsMessengerEXT CreateDebugUtilsMessengerEXT}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code createInfo} must be a pointer to a valid {@link XrDebugUtilsMessengerCreateInfoEXT} structure
* - {@code messenger} must be a pointer to an {@code XrDebugUtilsMessengerEXT} handle
*
*
* Thread Safety
*
*
* - Access to {@code instance}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* The application must ensure that {@link #xrCreateDebugUtilsMessengerEXT CreateDebugUtilsMessengerEXT} is not executed in parallel with any OpenXR function that is also called with {@code instance} or child of {@code instance}.
*
* When an event of interest occurs a debug messenger calls its {@link XrDebugUtilsMessengerCreateInfoEXT}{@code ::userCallback} with a debug message from the producer of the event. Additionally, the debug messenger must filter out any debug messages that the application’s callback is not interested in based on {@link XrDebugUtilsMessengerCreateInfoEXT} flags, as described below.
*
* See Also
*
* {@link XrDebugUtilsMessengerCreateInfoEXT}, {@link #xrDestroyDebugUtilsMessengerEXT DestroyDebugUtilsMessengerEXT}
*
* @param instance the instance the messenger will be used with.
* @param createInfo points to an {@link XrDebugUtilsMessengerCreateInfoEXT} structure, which contains the callback pointer as well as defines the conditions under which this messenger will trigger the callback.
* @param messenger a pointer to which the created {@code XrDebugUtilsMessengerEXT} object is returned.
*/
@NativeType("XrResult")
public static int xrCreateDebugUtilsMessengerEXT(XrInstance instance, @NativeType("XrDebugUtilsMessengerCreateInfoEXT const *") XrDebugUtilsMessengerCreateInfoEXT createInfo, @NativeType("XrDebugUtilsMessengerEXT *") PointerBuffer messenger) {
if (CHECKS) {
check(messenger, 1);
}
return nxrCreateDebugUtilsMessengerEXT(instance, createInfo.address(), memAddress(messenger));
}
// --- [ xrDestroyDebugUtilsMessengerEXT ] ---
/**
* Destroys a debug messenger.
*
* C Specification
*
*
* XrResult xrDestroyDebugUtilsMessengerEXT(
* XrDebugUtilsMessengerEXT messenger);
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTDebugUtils XR_EXT_debug_utils} extension must be enabled prior to calling {@link #xrDestroyDebugUtilsMessengerEXT DestroyDebugUtilsMessengerEXT}
* - {@code messenger} must be a valid {@code XrDebugUtilsMessengerEXT} handle
*
*
* Thread Safety
*
*
* - Access to {@code messenger} must be externally synchronized
* - Access to the {@code XrInstance} used to create {@code messenger}, and all of its child handles must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* The application must ensure that {@link #xrDestroyDebugUtilsMessengerEXT DestroyDebugUtilsMessengerEXT} is not executed in parallel with any OpenXR function that is also called with the {@code instance} or child of {@code instance} that it was created with.
*
* See Also
*
* {@link #xrCreateDebugUtilsMessengerEXT CreateDebugUtilsMessengerEXT}
*
* @param messenger the {@code XrDebugUtilsMessengerEXT} object to destroy. {@code messenger} is an externally synchronized object and must not be used on more than one thread at a time. This means that {@link #xrDestroyDebugUtilsMessengerEXT DestroyDebugUtilsMessengerEXT} must not be called when a callback is active.
*/
@NativeType("XrResult")
public static int xrDestroyDebugUtilsMessengerEXT(XrDebugUtilsMessengerEXT messenger) {
long __functionAddress = messenger.getCapabilities().xrDestroyDebugUtilsMessengerEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPI(messenger.address(), __functionAddress);
}
// --- [ xrSubmitDebugUtilsMessageEXT ] ---
/** Unsafe version of: {@link #xrSubmitDebugUtilsMessageEXT SubmitDebugUtilsMessageEXT} */
public static int nxrSubmitDebugUtilsMessageEXT(XrInstance instance, long messageSeverity, long messageTypes, long callbackData) {
long __functionAddress = instance.getCapabilities().xrSubmitDebugUtilsMessageEXT;
if (CHECKS) {
check(__functionAddress);
XrDebugUtilsMessengerCallbackDataEXT.validate(callbackData);
}
return callPJJPI(instance.address(), messageSeverity, messageTypes, callbackData, __functionAddress);
}
/**
* Submits debug utils message.
*
* C Specification
*
*
* XrResult xrSubmitDebugUtilsMessageEXT(
* XrInstance instance,
* XrDebugUtilsMessageSeverityFlagsEXT messageSeverity,
* XrDebugUtilsMessageTypeFlagsEXT messageTypes,
* const XrDebugUtilsMessengerCallbackDataEXT* callbackData);
*
* Valid Usage
*
*
* - For each structure in {@link XrDebugUtilsMessengerCallbackDataEXT}{@code ::objects}, the value of {@link XrDebugUtilsObjectNameInfoEXT}{@code ::objectType} must not be {@link XR10#XR_OBJECT_TYPE_UNKNOWN OBJECT_TYPE_UNKNOWN}
*
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTDebugUtils XR_EXT_debug_utils} extension must be enabled prior to calling {@link #xrSubmitDebugUtilsMessageEXT SubmitDebugUtilsMessageEXT}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code messageSeverity} must be a valid combination of {@code XrDebugUtilsMessageSeverityFlagBitsEXT} values
* - {@code messageSeverity} must not be 0
* - {@code messageTypes} must be a valid combination of {@code XrDebugUtilsMessageTypeFlagBitsEXT} values
* - {@code messageTypes} must not be 0
* - {@code callbackData} must be a pointer to a valid {@link XrDebugUtilsMessengerCallbackDataEXT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
*
*
*
* The application can also produce a debug message, and submit it into the OpenXR messaging system.
*
* The call will propagate through the layers and generate callback(s) as indicated by the message’s flags. The parameters are passed on to the callback in addition to the userData value that was defined at the time the messenger was created.
*
* See Also
*
* {@link XrDebugUtilsMessengerCallbackDataEXT}
*
* @param instance the debug stream’s {@code XrInstance}.
* @param messageSeverity a single bit value of {@code XrDebugUtilsMessageSeverityFlagsEXT} severity of this event/message.
* @param messageTypes an {@code XrDebugUtilsMessageTypeFlagsEXT} bitmask of {@code XrDebugUtilsMessageTypeFlagBitsEXT} specifying which types of event to identify this message with.
* @param callbackData contains all the callback related data in the {@link XrDebugUtilsMessengerCallbackDataEXT} structure.
*/
@NativeType("XrResult")
public static int xrSubmitDebugUtilsMessageEXT(XrInstance instance, @NativeType("XrDebugUtilsMessageSeverityFlagsEXT") long messageSeverity, @NativeType("XrDebugUtilsMessageTypeFlagsEXT") long messageTypes, @NativeType("XrDebugUtilsMessengerCallbackDataEXT const *") XrDebugUtilsMessengerCallbackDataEXT callbackData) {
return nxrSubmitDebugUtilsMessageEXT(instance, messageSeverity, messageTypes, callbackData.address());
}
// --- [ xrSessionBeginDebugUtilsLabelRegionEXT ] ---
/** Unsafe version of: {@link #xrSessionBeginDebugUtilsLabelRegionEXT SessionBeginDebugUtilsLabelRegionEXT} */
public static int nxrSessionBeginDebugUtilsLabelRegionEXT(XrSession session, long labelInfo) {
long __functionAddress = session.getCapabilities().xrSessionBeginDebugUtilsLabelRegionEXT;
if (CHECKS) {
check(__functionAddress);
XrDebugUtilsLabelEXT.validate(labelInfo);
}
return callPPI(session.address(), labelInfo, __functionAddress);
}
/**
* Session begin debug utils label region.
*
* C Specification
*
*
* XrResult xrSessionBeginDebugUtilsLabelRegionEXT(
* XrSession session,
* const XrDebugUtilsLabelEXT* labelInfo);
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTDebugUtils XR_EXT_debug_utils} extension must be enabled prior to calling {@link #xrSessionBeginDebugUtilsLabelRegionEXT SessionBeginDebugUtilsLabelRegionEXT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code labelInfo} must be a pointer to a valid {@link XrDebugUtilsLabelEXT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* The {@link #xrSessionBeginDebugUtilsLabelRegionEXT SessionBeginDebugUtilsLabelRegionEXT} function begins a label region within {@code session}.
*
* See Also
*
* {@link XrDebugUtilsLabelEXT}
*
* @param session the {@code XrSession} that a label region should be associated with.
* @param labelInfo the {@link XrDebugUtilsLabelEXT} containing the label information for the region that should be begun.
*/
@NativeType("XrResult")
public static int xrSessionBeginDebugUtilsLabelRegionEXT(XrSession session, @NativeType("XrDebugUtilsLabelEXT const *") XrDebugUtilsLabelEXT labelInfo) {
return nxrSessionBeginDebugUtilsLabelRegionEXT(session, labelInfo.address());
}
// --- [ xrSessionEndDebugUtilsLabelRegionEXT ] ---
/**
* Session end debug utils label region.
*
* C Specification
*
*
* XrResult xrSessionEndDebugUtilsLabelRegionEXT(
* XrSession session);
*
* Valid Usage
*
*
* - {@link #xrSessionEndDebugUtilsLabelRegionEXT SessionEndDebugUtilsLabelRegionEXT} must be called only after a matching {@link #xrSessionBeginDebugUtilsLabelRegionEXT SessionBeginDebugUtilsLabelRegionEXT}.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTDebugUtils XR_EXT_debug_utils} extension must be enabled prior to calling {@link #xrSessionEndDebugUtilsLabelRegionEXT SessionEndDebugUtilsLabelRegionEXT}
* - {@code session} must be a valid {@code XrSession} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* This function ends the last label region begun with the {@link #xrSessionBeginDebugUtilsLabelRegionEXT SessionBeginDebugUtilsLabelRegionEXT} function within the same {@code session}.
*
* @param session the {@code XrSession} that a label region should be associated with.
*/
@NativeType("XrResult")
public static int xrSessionEndDebugUtilsLabelRegionEXT(XrSession session) {
long __functionAddress = session.getCapabilities().xrSessionEndDebugUtilsLabelRegionEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPI(session.address(), __functionAddress);
}
// --- [ xrSessionInsertDebugUtilsLabelEXT ] ---
/** Unsafe version of: {@link #xrSessionInsertDebugUtilsLabelEXT SessionInsertDebugUtilsLabelEXT} */
public static int nxrSessionInsertDebugUtilsLabelEXT(XrSession session, long labelInfo) {
long __functionAddress = session.getCapabilities().xrSessionInsertDebugUtilsLabelEXT;
if (CHECKS) {
check(__functionAddress);
XrDebugUtilsLabelEXT.validate(labelInfo);
}
return callPPI(session.address(), labelInfo, __functionAddress);
}
/**
* Session insert debug utils label.
*
* C Specification
*
*
* XrResult xrSessionInsertDebugUtilsLabelEXT(
* XrSession session,
* const XrDebugUtilsLabelEXT* labelInfo);
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTDebugUtils XR_EXT_debug_utils} extension must be enabled prior to calling {@link #xrSessionInsertDebugUtilsLabelEXT SessionInsertDebugUtilsLabelEXT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code labelInfo} must be a pointer to a valid {@link XrDebugUtilsLabelEXT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* The {@link #xrSessionInsertDebugUtilsLabelEXT SessionInsertDebugUtilsLabelEXT} function inserts an individual label within {@code session}. The individual labels are useful for different reasons based on the type of debugging scenario. When used with something active like a profiler or debugger, it identifies a single point of time. When used with logging, the individual label identifies that a particular location has been passed at the point the log message is triggered. Because of this usage, individual labels only exist in a log until the next call to any of the label functions:
*
*
* - {@link #xrSessionBeginDebugUtilsLabelRegionEXT SessionBeginDebugUtilsLabelRegionEXT}
* - {@link #xrSessionEndDebugUtilsLabelRegionEXT SessionEndDebugUtilsLabelRegionEXT}
* - {@link #xrSessionInsertDebugUtilsLabelEXT SessionInsertDebugUtilsLabelEXT}
*
*
* See Also
*
* {@link XrDebugUtilsLabelEXT}, {@link #xrSessionBeginDebugUtilsLabelRegionEXT SessionBeginDebugUtilsLabelRegionEXT}, {@link #xrSessionEndDebugUtilsLabelRegionEXT SessionEndDebugUtilsLabelRegionEXT}
*
* @param session the {@code XrSession} that a label region should be associated with.
* @param labelInfo the {@link XrDebugUtilsLabelEXT} containing the label information for the region that should be begun.
*/
@NativeType("XrResult")
public static int xrSessionInsertDebugUtilsLabelEXT(XrSession session, @NativeType("XrDebugUtilsLabelEXT const *") XrDebugUtilsLabelEXT labelInfo) {
return nxrSessionInsertDebugUtilsLabelEXT(session, labelInfo.address());
}
}