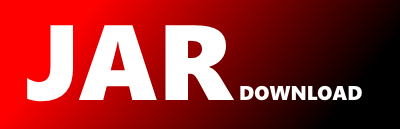
org.lwjgl.openxr.EXTPlaneDetection Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_EXT_plane_detection extension.
*
* This extension enables applications to detect planes in the scene.
*/
public class EXTPlaneDetection {
/** The extension specification version. */
public static final int XR_EXT_plane_detection_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_EXT_PLANE_DETECTION_EXTENSION_NAME = "XR_EXT_plane_detection";
/**
* Extends {@code XrResult}.
*
* Enum values:
*
*
* - {@link #XR_ERROR_SPACE_NOT_LOCATABLE_EXT ERROR_SPACE_NOT_LOCATABLE_EXT}
* - {@link #XR_ERROR_PLANE_DETECTION_PERMISSION_DENIED_EXT ERROR_PLANE_DETECTION_PERMISSION_DENIED_EXT}
*
*/
public static final int
XR_ERROR_SPACE_NOT_LOCATABLE_EXT = -1000429000,
XR_ERROR_PLANE_DETECTION_PERMISSION_DENIED_EXT = -1000429001;
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_PLANE_DETECTOR_EXT = 1000429000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_PLANE_DETECTOR_CREATE_INFO_EXT TYPE_PLANE_DETECTOR_CREATE_INFO_EXT}
* - {@link #XR_TYPE_PLANE_DETECTOR_BEGIN_INFO_EXT TYPE_PLANE_DETECTOR_BEGIN_INFO_EXT}
* - {@link #XR_TYPE_PLANE_DETECTOR_GET_INFO_EXT TYPE_PLANE_DETECTOR_GET_INFO_EXT}
* - {@link #XR_TYPE_PLANE_DETECTOR_LOCATIONS_EXT TYPE_PLANE_DETECTOR_LOCATIONS_EXT}
* - {@link #XR_TYPE_PLANE_DETECTOR_LOCATION_EXT TYPE_PLANE_DETECTOR_LOCATION_EXT}
* - {@link #XR_TYPE_PLANE_DETECTOR_POLYGON_BUFFER_EXT TYPE_PLANE_DETECTOR_POLYGON_BUFFER_EXT}
* - {@link #XR_TYPE_SYSTEM_PLANE_DETECTION_PROPERTIES_EXT TYPE_SYSTEM_PLANE_DETECTION_PROPERTIES_EXT}
*
*/
public static final int
XR_TYPE_PLANE_DETECTOR_CREATE_INFO_EXT = 1000429001,
XR_TYPE_PLANE_DETECTOR_BEGIN_INFO_EXT = 1000429002,
XR_TYPE_PLANE_DETECTOR_GET_INFO_EXT = 1000429003,
XR_TYPE_PLANE_DETECTOR_LOCATIONS_EXT = 1000429004,
XR_TYPE_PLANE_DETECTOR_LOCATION_EXT = 1000429005,
XR_TYPE_PLANE_DETECTOR_POLYGON_BUFFER_EXT = 1000429006,
XR_TYPE_SYSTEM_PLANE_DETECTION_PROPERTIES_EXT = 1000429007;
/**
* XrPlaneDetectionCapabilityFlagBitsEXT - Feature Flag Bits
*
* Description
*
* The flag bits have the following meanings:
*
* Flag Descriptions
*
*
* - {@link #XR_PLANE_DETECTION_CAPABILITY_PLANE_DETECTION_BIT_EXT PLANE_DETECTION_CAPABILITY_PLANE_DETECTION_BIT_EXT} — plane detection is supported
* - {@link #XR_PLANE_DETECTION_CAPABILITY_PLANE_HOLES_BIT_EXT PLANE_DETECTION_CAPABILITY_PLANE_HOLES_BIT_EXT} — polygon buffers for holes in planes can be generated
* - {@link #XR_PLANE_DETECTION_CAPABILITY_SEMANTIC_CEILING_BIT_EXT PLANE_DETECTION_CAPABILITY_SEMANTIC_CEILING_BIT_EXT} — plane detection supports ceiling semantic classification
* - {@link #XR_PLANE_DETECTION_CAPABILITY_SEMANTIC_FLOOR_BIT_EXT PLANE_DETECTION_CAPABILITY_SEMANTIC_FLOOR_BIT_EXT} — plane detection supports floor semantic classification
* - {@link #XR_PLANE_DETECTION_CAPABILITY_SEMANTIC_WALL_BIT_EXT PLANE_DETECTION_CAPABILITY_SEMANTIC_WALL_BIT_EXT} — plane detection supports wall semantic classification
* - {@link #XR_PLANE_DETECTION_CAPABILITY_SEMANTIC_PLATFORM_BIT_EXT PLANE_DETECTION_CAPABILITY_SEMANTIC_PLATFORM_BIT_EXT} — plane detection supports platform semantic classification (for example table tops)
* - {@link #XR_PLANE_DETECTION_CAPABILITY_ORIENTATION_BIT_EXT PLANE_DETECTION_CAPABILITY_ORIENTATION_BIT_EXT} — plane detection supports plane orientation classification. If not supported planes are always classified as ARBITRARY.
*
*/
public static final int
XR_PLANE_DETECTION_CAPABILITY_PLANE_DETECTION_BIT_EXT = 0x1,
XR_PLANE_DETECTION_CAPABILITY_PLANE_HOLES_BIT_EXT = 0x2,
XR_PLANE_DETECTION_CAPABILITY_SEMANTIC_CEILING_BIT_EXT = 0x4,
XR_PLANE_DETECTION_CAPABILITY_SEMANTIC_FLOOR_BIT_EXT = 0x8,
XR_PLANE_DETECTION_CAPABILITY_SEMANTIC_WALL_BIT_EXT = 0x10,
XR_PLANE_DETECTION_CAPABILITY_SEMANTIC_PLATFORM_BIT_EXT = 0x20,
XR_PLANE_DETECTION_CAPABILITY_ORIENTATION_BIT_EXT = 0x40;
/**
* XrPlaneDetectorFlagBitsEXT - Plane Detector flags
*
* Description
*
* The flag bits have the following meanings:
*
* Flag Descriptions
*
*
* - {@link #XR_PLANE_DETECTOR_ENABLE_CONTOUR_BIT_EXT PLANE_DETECTOR_ENABLE_CONTOUR_BIT_EXT} — populate the plane contour information
*
*/
public static final int XR_PLANE_DETECTOR_ENABLE_CONTOUR_BIT_EXT = 0x1;
/**
* XrPlaneDetectorOrientationEXT - Orientation of the detected plane (upward, downward…)
*
* Description
*
* The enums have the following meanings:
*
*
* Enum Description
*
* {@link #XR_PLANE_DETECTOR_ORIENTATION_HORIZONTAL_UPWARD_EXT PLANE_DETECTOR_ORIENTATION_HORIZONTAL_UPWARD_EXT} The detected plane is horizontal and faces upward (e.g. floor).
* {@link #XR_PLANE_DETECTOR_ORIENTATION_HORIZONTAL_DOWNWARD_EXT PLANE_DETECTOR_ORIENTATION_HORIZONTAL_DOWNWARD_EXT} The detected plane is horizontal and faces downward (e.g. ceiling).
* {@link #XR_PLANE_DETECTOR_ORIENTATION_VERTICAL_EXT PLANE_DETECTOR_ORIENTATION_VERTICAL_EXT} The detected plane is vertical (e.g. wall).
* {@link #XR_PLANE_DETECTOR_ORIENTATION_ARBITRARY_EXT PLANE_DETECTOR_ORIENTATION_ARBITRARY_EXT} The detected plane has an arbitrary, non-vertical and non-horizontal orientation.
*
*
*
* See Also
*
* {@link XrPlaneDetectorBeginInfoEXT}, {@link XrPlaneDetectorLocationEXT}, {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}
*/
public static final int
XR_PLANE_DETECTOR_ORIENTATION_HORIZONTAL_UPWARD_EXT = 0,
XR_PLANE_DETECTOR_ORIENTATION_HORIZONTAL_DOWNWARD_EXT = 1,
XR_PLANE_DETECTOR_ORIENTATION_VERTICAL_EXT = 2,
XR_PLANE_DETECTOR_ORIENTATION_ARBITRARY_EXT = 3;
/**
* XrPlaneDetectorSemanticTypeEXT - Type of the detected plane (upward, downward…)
*
* Description
*
* The enums have the following meanings:
*
*
* Enum Description
*
* {@link #XR_PLANE_DETECTOR_SEMANTIC_TYPE_UNDEFINED_EXT PLANE_DETECTOR_SEMANTIC_TYPE_UNDEFINED_EXT} The runtime was unable to classify this plane.
* {@link #XR_PLANE_DETECTOR_SEMANTIC_TYPE_CEILING_EXT PLANE_DETECTOR_SEMANTIC_TYPE_CEILING_EXT} The detected plane is a ceiling.
* {@link #XR_PLANE_DETECTOR_SEMANTIC_TYPE_FLOOR_EXT PLANE_DETECTOR_SEMANTIC_TYPE_FLOOR_EXT} The detected plane is a floor.
* {@link #XR_PLANE_DETECTOR_SEMANTIC_TYPE_WALL_EXT PLANE_DETECTOR_SEMANTIC_TYPE_WALL_EXT} The detected plane is a wall.
* {@link #XR_PLANE_DETECTOR_SEMANTIC_TYPE_PLATFORM_EXT PLANE_DETECTOR_SEMANTIC_TYPE_PLATFORM_EXT} The detected plane is a platform, like a table.
*
*
*
* See Also
*
* {@link XrPlaneDetectorBeginInfoEXT}, {@link XrPlaneDetectorLocationEXT}, {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}
*/
public static final int
XR_PLANE_DETECTOR_SEMANTIC_TYPE_UNDEFINED_EXT = 0,
XR_PLANE_DETECTOR_SEMANTIC_TYPE_CEILING_EXT = 1,
XR_PLANE_DETECTOR_SEMANTIC_TYPE_FLOOR_EXT = 2,
XR_PLANE_DETECTOR_SEMANTIC_TYPE_WALL_EXT = 3,
XR_PLANE_DETECTOR_SEMANTIC_TYPE_PLATFORM_EXT = 4;
/**
* XrPlaneDetectionStateEXT - Plane Detection State
*
* Enumerant Descriptions
*
*
* - {@link #XR_PLANE_DETECTION_STATE_NONE_EXT PLANE_DETECTION_STATE_NONE_EXT} - The plane detector is not actively looking for planes; call {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} to start detection.
* - {@link #XR_PLANE_DETECTION_STATE_PENDING_EXT PLANE_DETECTION_STATE_PENDING_EXT} - This plane detector is currently looking for planes but not yet ready with results; call {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT} again, or call {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} to restart with new filter parameters.
* - {@link #XR_PLANE_DETECTION_STATE_DONE_EXT PLANE_DETECTION_STATE_DONE_EXT} - This plane detector has finished and results may now be retrieved. The results are valid until {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} or {@link #xrDestroyPlaneDetectorEXT DestroyPlaneDetectorEXT} are called.
* - {@link #XR_PLANE_DETECTION_STATE_ERROR_EXT PLANE_DETECTION_STATE_ERROR_EXT} - An error occurred. The query may be tried again.
* - {@link #XR_PLANE_DETECTION_STATE_FATAL_EXT PLANE_DETECTION_STATE_FATAL_EXT} - An error occurred. The query must not be tried again.
*
*
* See Also
*
* {@link #xrGetPlaneDetectionStateEXT GetPlaneDetectionStateEXT}, {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}
*/
public static final int
XR_PLANE_DETECTION_STATE_NONE_EXT = 0,
XR_PLANE_DETECTION_STATE_PENDING_EXT = 1,
XR_PLANE_DETECTION_STATE_DONE_EXT = 2,
XR_PLANE_DETECTION_STATE_ERROR_EXT = 3,
XR_PLANE_DETECTION_STATE_FATAL_EXT = 4;
protected EXTPlaneDetection() {
throw new UnsupportedOperationException();
}
// --- [ xrCreatePlaneDetectorEXT ] ---
/** Unsafe version of: {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT} */
public static int nxrCreatePlaneDetectorEXT(XrSession session, long createInfo, long planeDetector) {
long __functionAddress = session.getCapabilities().xrCreatePlaneDetectorEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, planeDetector, __functionAddress);
}
/**
* Create a plane detection handle.
*
* C Specification
*
* The {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT} function is defined as:
*
*
* XrResult xrCreatePlaneDetectorEXT(
* XrSession session,
* const XrPlaneDetectorCreateInfoEXT* createInfo,
* XrPlaneDetectorEXT* planeDetector);
*
* Description
*
* An application creates an {@code XrPlaneDetectorEXT} handle using {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT} function.
*
* If the system does not support plane detection, the runtime must return {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED} from {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT}.
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTPlaneDetection XR_EXT_plane_detection} extension must be enabled prior to calling {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrPlaneDetectorCreateInfoEXT} structure
* - {@code planeDetector} must be a pointer to an {@code XrPlaneDetectorEXT} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link #XR_ERROR_PLANE_DETECTION_PERMISSION_DENIED_EXT ERROR_PLANE_DETECTION_PERMISSION_DENIED_EXT}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrPlaneDetectorCreateInfoEXT}, {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT}, {@link #xrDestroyPlaneDetectorEXT DestroyPlaneDetectorEXT}
*
* @param session an {@code XrSession} in which the plane detection will be active.
* @param createInfo the {@link XrPlaneDetectorCreateInfoEXT} used to specify the plane detection.
* @param planeDetector the returned {@code XrPlaneDetectorEXT} handle.
*/
@NativeType("XrResult")
public static int xrCreatePlaneDetectorEXT(XrSession session, @NativeType("XrPlaneDetectorCreateInfoEXT const *") XrPlaneDetectorCreateInfoEXT createInfo, @NativeType("XrPlaneDetectorEXT *") PointerBuffer planeDetector) {
if (CHECKS) {
check(planeDetector, 1);
}
return nxrCreatePlaneDetectorEXT(session, createInfo.address(), memAddress(planeDetector));
}
// --- [ xrDestroyPlaneDetectorEXT ] ---
/**
* Destroy a plane detection handle.
*
* C Specification
*
* The {@link #xrDestroyPlaneDetectorEXT DestroyPlaneDetectorEXT} function is defined as:
*
*
* XrResult xrDestroyPlaneDetectorEXT(
* XrPlaneDetectorEXT planeDetector);
*
* Description
*
* {@link #xrDestroyPlaneDetectorEXT DestroyPlaneDetectorEXT} function releases the {@code planeDetector} and the underlying resources when finished with plane detection experiences.
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTPlaneDetection XR_EXT_plane_detection} extension must be enabled prior to calling {@link #xrDestroyPlaneDetectorEXT DestroyPlaneDetectorEXT}
* - {@code planeDetector} must be a valid {@code XrPlaneDetectorEXT} handle
*
*
* Thread Safety
*
*
* - Access to {@code planeDetector}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* See Also
*
* {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT}
*
* @param planeDetector an {@code XrPlaneDetectorEXT} previously created by {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT}.
*/
@NativeType("XrResult")
public static int xrDestroyPlaneDetectorEXT(XrPlaneDetectorEXT planeDetector) {
long __functionAddress = planeDetector.getCapabilities().xrDestroyPlaneDetectorEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPI(planeDetector.address(), __functionAddress);
}
// --- [ xrBeginPlaneDetectionEXT ] ---
/** Unsafe version of: {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} */
public static int nxrBeginPlaneDetectionEXT(XrPlaneDetectorEXT planeDetector, long beginInfo) {
long __functionAddress = planeDetector.getCapabilities().xrBeginPlaneDetectionEXT;
if (CHECKS) {
check(__functionAddress);
XrPlaneDetectorBeginInfoEXT.validate(beginInfo);
}
return callPPI(planeDetector.address(), beginInfo, __functionAddress);
}
/**
* Detect planes.
*
* C Specification
*
* The {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} function is defined as:
*
*
* XrResult xrBeginPlaneDetectionEXT(
* XrPlaneDetectorEXT planeDetector,
* const XrPlaneDetectorBeginInfoEXT* beginInfo);
*
* Description
*
* The {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} function begins the detection of planes in the scene. Detecting planes in a scene is an asynchronous operation. {@link #xrGetPlaneDetectionStateEXT GetPlaneDetectionStateEXT} can be used to determine if the query has finished. Once it has finished the results may be retrieved via {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}. If a detection has already been started on a plane detector handle, calling {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} again on the same handle will cancel the operation in progress and start a new detection with the new filter parameters.
*
* The bounding volume is resolved and fixed relative to LOCAL space at the time of the call to {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} using {@link XrPlaneDetectorBeginInfoEXT}{@code ::baseSpace}, {@link XrPlaneDetectorBeginInfoEXT}{@code ::time}, {@link XrPlaneDetectorBeginInfoEXT}{@code ::boundingBoxPose} and {@link XrPlaneDetectorBeginInfoEXT}{@code ::boundingBoxExtent}. The runtime must resolve the location defined by {@link XrPlaneDetectorBeginInfoEXT}{@code ::baseSpace} at the time of the call. The {@link XrPlaneDetectorBeginInfoEXT}{@code ::boundingBoxPose} is the pose of the center of the box defined by {@link XrPlaneDetectorBeginInfoEXT}{@code ::boundingBoxExtent}.
*
* The runtime must return {@link #XR_ERROR_SPACE_NOT_LOCATABLE_EXT ERROR_SPACE_NOT_LOCATABLE_EXT} if the {@link XrPlaneDetectorBeginInfoEXT}{@code ::baseSpace} is not locatable at the time of the call.
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTPlaneDetection XR_EXT_plane_detection} extension must be enabled prior to calling {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT}
* - {@code planeDetector} must be a valid {@code XrPlaneDetectorEXT} handle
* - {@code beginInfo} must be a pointer to a valid {@link XrPlaneDetectorBeginInfoEXT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
* - {@link #XR_ERROR_SPACE_NOT_LOCATABLE_EXT ERROR_SPACE_NOT_LOCATABLE_EXT}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
*
*
*
* See Also
*
* {@link XrPlaneDetectorBeginInfoEXT}, {@link XrPlaneDetectorGetInfoEXT}, {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}
*
* @param planeDetector an {@code XrPlaneDetectorEXT} previously created by {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT}.
* @param beginInfo a pointer to {@link XrPlaneDetectorBeginInfoEXT} containing plane detection parameters.
*/
@NativeType("XrResult")
public static int xrBeginPlaneDetectionEXT(XrPlaneDetectorEXT planeDetector, @NativeType("XrPlaneDetectorBeginInfoEXT const *") XrPlaneDetectorBeginInfoEXT beginInfo) {
return nxrBeginPlaneDetectionEXT(planeDetector, beginInfo.address());
}
// --- [ xrGetPlaneDetectionStateEXT ] ---
/** Unsafe version of: {@link #xrGetPlaneDetectionStateEXT GetPlaneDetectionStateEXT} */
public static int nxrGetPlaneDetectionStateEXT(XrPlaneDetectorEXT planeDetector, long state) {
long __functionAddress = planeDetector.getCapabilities().xrGetPlaneDetectionStateEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(planeDetector.address(), state, __functionAddress);
}
/**
* Get the state of the plane detection pass.
*
* C Specification
*
* The {@link #xrGetPlaneDetectionStateEXT GetPlaneDetectionStateEXT} function is defined as:
*
*
* XrResult xrGetPlaneDetectionStateEXT(
* XrPlaneDetectorEXT planeDetector,
* XrPlaneDetectionStateEXT* state);
*
* Description
*
* The {@link #xrGetPlaneDetectionStateEXT GetPlaneDetectionStateEXT} function retrieves the state of the plane query and must be called before calling {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}.
*
* If the plane detection has not yet finished {@code state} must be {@link #XR_PLANE_DETECTION_STATE_PENDING_EXT PLANE_DETECTION_STATE_PENDING_EXT}. If the plane detection has finished {@code state} must be {@link #XR_PLANE_DETECTION_STATE_DONE_EXT PLANE_DETECTION_STATE_DONE_EXT}. If no plane detection was previously started {@link #XR_PLANE_DETECTION_STATE_NONE_EXT PLANE_DETECTION_STATE_NONE_EXT} must be returned. For all three states the function must return {@link XR10#XR_SUCCESS SUCCESS}.
*
* When a query error occurs the function must return {@link XR10#XR_SUCCESS SUCCESS} and the appropriate error state value must be set.
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTPlaneDetection XR_EXT_plane_detection} extension must be enabled prior to calling {@link #xrGetPlaneDetectionStateEXT GetPlaneDetectionStateEXT}
* - {@code planeDetector} must be a valid {@code XrPlaneDetectorEXT} handle
* - {@code state} must be a pointer to an {@code XrPlaneDetectionStateEXT} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT}
*
* @param planeDetector an {@code XrPlaneDetectorEXT} previously created by {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT}.
* @param state a pointer to {@code XrPlaneDetectionStateEXT}.
*/
@NativeType("XrResult")
public static int xrGetPlaneDetectionStateEXT(XrPlaneDetectorEXT planeDetector, @NativeType("XrPlaneDetectionStateEXT *") IntBuffer state) {
if (CHECKS) {
check(state, 1);
}
return nxrGetPlaneDetectionStateEXT(planeDetector, memAddress(state));
}
// --- [ xrGetPlaneDetectionsEXT ] ---
/** Unsafe version of: {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT} */
public static int nxrGetPlaneDetectionsEXT(XrPlaneDetectorEXT planeDetector, long info, long locations) {
long __functionAddress = planeDetector.getCapabilities().xrGetPlaneDetectionsEXT;
if (CHECKS) {
check(__functionAddress);
XrPlaneDetectorGetInfoEXT.validate(info);
}
return callPPPI(planeDetector.address(), info, locations, __functionAddress);
}
/**
* Get the detected planes.
*
* C Specification
*
* The {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT} function is defined as:
*
*
* XrResult xrGetPlaneDetectionsEXT(
* XrPlaneDetectorEXT planeDetector,
* const XrPlaneDetectorGetInfoEXT* info,
* XrPlaneDetectorLocationsEXT* locations);
*
* Description
*
* {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT} must return {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID} if the detector state reported by {@link #xrGetPlaneDetectionStateEXT GetPlaneDetectionStateEXT} is not {@link #XR_PLANE_DETECTION_STATE_DONE_EXT PLANE_DETECTION_STATE_DONE_EXT} for the current query started by {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT}.
*
* If the {@link XrPlaneDetectorGetInfoEXT}::baseSpace is not locatable {@link #XR_ERROR_SPACE_NOT_LOCATABLE_EXT ERROR_SPACE_NOT_LOCATABLE_EXT} must be returned.
*
* Once {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} is called again, the previous results for that handle are no longer available. The application should cache them before calling {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT} again if it needs access to that data while waiting for updated detection results.
*
* Upon the completion of a detection cycle ({@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT}, {@link #xrGetPlaneDetectionStateEXT GetPlaneDetectionStateEXT} to {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}) the runtime must keep a snapshot of the plane data and no data may be modified. Calling {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT} multiple times with the same baseSpace and time must return the same plane pose data.
*
* The current snapshot, if any, must be discarded upon calling {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT}.
*
* If the {@link XrEventDataReferenceSpaceChangePending} is queued and the changeTime elapsed while the application is holding cached data the application may use the event data to adjusted poses accordingly.
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTPlaneDetection XR_EXT_plane_detection} extension must be enabled prior to calling {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}
* - {@code planeDetector} must be a valid {@code XrPlaneDetectorEXT} handle
* - {@code info} must be a pointer to a valid {@link XrPlaneDetectorGetInfoEXT} structure
* - {@code locations} must be a pointer to an {@link XrPlaneDetectorLocationsEXT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
* - {@link #XR_ERROR_SPACE_NOT_LOCATABLE_EXT ERROR_SPACE_NOT_LOCATABLE_EXT}
* - {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID}
*
*
*
* See Also
*
* {@link XrPlaneDetectorGetInfoEXT}, {@link XrPlaneDetectorLocationsEXT}, {@link #xrBeginPlaneDetectionEXT BeginPlaneDetectionEXT}
*
* @param planeDetector an {@code XrPlaneDetectorEXT} previously created by {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT}.
* @param info a pointer to {@link XrPlaneDetectorGetInfoEXT}.
* @param locations a pointer to {@link XrPlaneDetectorLocationsEXT} receiving the returned plane locations.
*/
@NativeType("XrResult")
public static int xrGetPlaneDetectionsEXT(XrPlaneDetectorEXT planeDetector, @NativeType("XrPlaneDetectorGetInfoEXT const *") XrPlaneDetectorGetInfoEXT info, @NativeType("XrPlaneDetectorLocationsEXT *") XrPlaneDetectorLocationsEXT locations) {
return nxrGetPlaneDetectionsEXT(planeDetector, info.address(), locations.address());
}
// --- [ xrGetPlanePolygonBufferEXT ] ---
/** Unsafe version of: {@link #xrGetPlanePolygonBufferEXT GetPlanePolygonBufferEXT} */
public static int nxrGetPlanePolygonBufferEXT(XrPlaneDetectorEXT planeDetector, long planeId, int polygonBufferIndex, long polygonBuffer) {
long __functionAddress = planeDetector.getCapabilities().xrGetPlanePolygonBufferEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(planeDetector.address(), planeId, polygonBufferIndex, polygonBuffer, __functionAddress);
}
/**
* Gets the plane.
*
* C Specification
*
* The {@link #xrGetPlanePolygonBufferEXT GetPlanePolygonBufferEXT} function is defined as:
*
*
* XrResult xrGetPlanePolygonBufferEXT(
* XrPlaneDetectorEXT planeDetector,
* uint64_t planeId,
* uint32_t polygonBufferIndex,
* XrPlaneDetectorPolygonBufferEXT* polygonBuffer);
*
* Description
*
* The {@link #xrGetPlanePolygonBufferEXT GetPlanePolygonBufferEXT} function retrieves the plane’s polygon buffer for the given {@code planeId} and {@code polygonBufferIndex}. Calling {@link #xrGetPlanePolygonBufferEXT GetPlanePolygonBufferEXT} with {@code polygonBufferIndex} equal to 0 must return the outside contour, if available. Calls with non-zero indices less than {@link XrPlaneDetectorLocationEXT}{@code ::polygonBufferCount} must return polygons corresponding to holes in the plane. This feature may not be supported by all runtimes, check the {@link XrSystemPlaneDetectionPropertiesEXT}{@code ::supportedFeatures} for support.
*
* Outside contour polygon vertices must be ordered in counter clockwise order. Vertices of holes must be ordered in clockwise order. The right-hand rule is used to determine the direction of the normal of this plane. The polygon contour data is relative to the pose of the plane and coplanar with it.
*
* This function only retrieves polygons, which means that it needs to be converted to a regular mesh to be rendered.
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTPlaneDetection XR_EXT_plane_detection} extension must be enabled prior to calling {@link #xrGetPlanePolygonBufferEXT GetPlanePolygonBufferEXT}
* - {@code planeDetector} must be a valid {@code XrPlaneDetectorEXT} handle
* - {@code polygonBuffer} must be a pointer to an {@link XrPlaneDetectorPolygonBufferEXT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link XrPlaneDetectorPolygonBufferEXT}, {@link #xrGetPlaneDetectionsEXT GetPlaneDetectionsEXT}
*
* @param planeDetector an {@code XrPlaneDetectorEXT} previously created by {@link #xrCreatePlaneDetectorEXT CreatePlaneDetectorEXT}.
* @param planeId the {@link XrPlaneDetectorLocationEXT}{@code ::planeId}.
* @param polygonBufferIndex the index of the polygon contour buffer to retrieve. This must be a number from 0 to {@link XrPlaneDetectorLocationEXT}:polygonBufferCount -1. Index 0 retrieves the outside contour, larger indexes retrieve holes in the plane.
* @param polygonBuffer a pointer to {@link XrPlaneDetectorPolygonBufferEXT} receiving the returned plane polygon buffer.
*/
@NativeType("XrResult")
public static int xrGetPlanePolygonBufferEXT(XrPlaneDetectorEXT planeDetector, @NativeType("uint64_t") long planeId, @NativeType("uint32_t") int polygonBufferIndex, @NativeType("XrPlaneDetectorPolygonBufferEXT *") XrPlaneDetectorPolygonBufferEXT polygonBuffer) {
return nxrGetPlanePolygonBufferEXT(planeDetector, planeId, polygonBufferIndex, polygonBuffer.address());
}
}