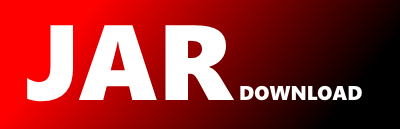
org.lwjgl.openxr.EXTThermalQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwjgl-openxr Show documentation
Show all versions of lwjgl-openxr Show documentation
A royalty-free, open standard that provides high-performance access to Augmented Reality (AR) and Virtual Reality (VR)—collectively known as XR—platforms and devices.
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_EXT_thermal_query extension.
*
* This extension provides an API to query a domain’s current thermal warning level and current thermal trend.
*/
public class EXTThermalQuery {
/** The extension specification version. */
public static final int XR_EXT_thermal_query_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_EXT_THERMAL_QUERY_EXTENSION_NAME = "XR_EXT_thermal_query";
protected EXTThermalQuery() {
throw new UnsupportedOperationException();
}
// --- [ xrThermalGetTemperatureTrendEXT ] ---
/** Unsafe version of: {@link #xrThermalGetTemperatureTrendEXT ThermalGetTemperatureTrendEXT} */
public static int nxrThermalGetTemperatureTrendEXT(XrSession session, int domain, long notificationLevel, long tempHeadroom, long tempSlope) {
long __functionAddress = session.getCapabilities().xrThermalGetTemperatureTrendEXT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPPI(session.address(), domain, notificationLevel, tempHeadroom, tempSlope, __functionAddress);
}
/**
* xrThermalGetTemperatureTrendEXT.
*
* C Specification
*
*
* XrResult xrThermalGetTemperatureTrendEXT(
* XrSession session,
* XrPerfSettingsDomainEXT domain,
* XrPerfSettingsNotificationLevelEXT* notificationLevel,
* float* tempHeadroom,
* float* tempSlope);
*
* Allows to query the current temperature warning level of a domain, the remaining headroom and the trend.
*
* Valid Usage (Implicit)
*
*
* - The {@link EXTThermalQuery XR_EXT_thermal_query} extension must be enabled prior to calling {@link #xrThermalGetTemperatureTrendEXT ThermalGetTemperatureTrendEXT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code domain} must be a valid {@code XrPerfSettingsDomainEXT} value
* - {@code notificationLevel} must be a pointer to an {@code XrPerfSettingsNotificationLevelEXT} value
* - {@code tempHeadroom} must be a pointer to a {@code float} value
* - {@code tempSlope} must be a pointer to a {@code float} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* @param session a valid {@code XrSession} handle.
* @param domain : the processing domain
* @param notificationLevel : the current warning level
* @param tempHeadroom : temperature headroom in degrees Celsius, expressing how far the most-critical temperature of the domain is from its thermal throttling threshold temperature.
* @param tempSlope : the current trend in degrees Celsius per second of the most critical temperature of the domain.
*/
@NativeType("XrResult")
public static int xrThermalGetTemperatureTrendEXT(XrSession session, @NativeType("XrPerfSettingsDomainEXT") int domain, @NativeType("XrPerfSettingsNotificationLevelEXT *") IntBuffer notificationLevel, @NativeType("float *") FloatBuffer tempHeadroom, @NativeType("float *") FloatBuffer tempSlope) {
if (CHECKS) {
check(notificationLevel, 1);
check(tempHeadroom, 1);
check(tempSlope, 1);
}
return nxrThermalGetTemperatureTrendEXT(session, domain, memAddress(notificationLevel), memAddress(tempHeadroom), memAddress(tempSlope));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy