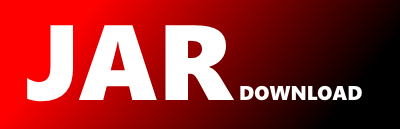
org.lwjgl.openxr.FBDisplayRefreshRate Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_FB_display_refresh_rate extension.
*
* On platforms which support dynamically adjusting the display refresh rate, application developers may request a specific display refresh rate in order to improve the overall user experience, examples include:
*
*
* - A video application may choose a display refresh rate which better matches the video content playback rate in order to achieve smoother video frames.
* - An application which can support a higher frame rate may choose to render at the higher rate to improve the overall perceptual quality, for example, lower latency and less flicker.
*
*
* This extension allows:
*
*
* - An application to identify what display refresh rates the session supports and the current display refresh rate.
* - An application to request a display refresh rate to indicate its preference to the runtime.
* - An application to receive notification of changes to the display refresh rate which are delivered via events.
*
*
* In order to enable the functionality of this extension, the application must pass the name of the extension into {@link XR10#xrCreateInstance CreateInstance} via the {@link XrInstanceCreateInfo}{@code ::enabledExtensionNames} parameter as indicated in the extension section.
*/
public class FBDisplayRefreshRate {
/** The extension specification version. */
public static final int XR_FB_display_refresh_rate_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_FB_DISPLAY_REFRESH_RATE_EXTENSION_NAME = "XR_FB_display_refresh_rate";
/** Extends {@code XrStructureType}. */
public static final int XR_TYPE_EVENT_DATA_DISPLAY_REFRESH_RATE_CHANGED_FB = 1000101000;
/** Extends {@code XrResult}. */
public static final int XR_ERROR_DISPLAY_REFRESH_RATE_UNSUPPORTED_FB = -1000101000;
protected FBDisplayRefreshRate() {
throw new UnsupportedOperationException();
}
// --- [ xrEnumerateDisplayRefreshRatesFB ] ---
/**
* Unsafe version of: {@link #xrEnumerateDisplayRefreshRatesFB EnumerateDisplayRefreshRatesFB}
*
* @param displayRefreshRateCapacityInput the capacity of the {@code displayRefreshRates}, or 0 to retrieve the required capacity.
*/
public static int nxrEnumerateDisplayRefreshRatesFB(XrSession session, int displayRefreshRateCapacityInput, long displayRefreshRateCountOutput, long displayRefreshRates) {
long __functionAddress = session.getCapabilities().xrEnumerateDisplayRefreshRatesFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), displayRefreshRateCapacityInput, displayRefreshRateCountOutput, displayRefreshRates, __functionAddress);
}
/**
* Enumerates display refresh rates.
*
* C Specification
*
* The {@link #xrEnumerateDisplayRefreshRatesFB EnumerateDisplayRefreshRatesFB} function is defined as:
*
*
* XrResult xrEnumerateDisplayRefreshRatesFB(
* XrSession session,
* uint32_t displayRefreshRateCapacityInput,
* uint32_t* displayRefreshRateCountOutput,
* float* displayRefreshRates);
*
* Description
*
* {@link #xrEnumerateDisplayRefreshRatesFB EnumerateDisplayRefreshRatesFB} enumerates the display refresh rates supported by the current session. Display refresh rates must be in order from lowest to highest supported display refresh rates. Runtimes must always return identical buffer contents from this enumeration for the lifetime of the session.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBDisplayRefreshRate XR_FB_display_refresh_rate} extension must be enabled prior to calling {@link #xrEnumerateDisplayRefreshRatesFB EnumerateDisplayRefreshRatesFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code displayRefreshRateCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code displayRefreshRateCapacityInput} is not 0, {@code displayRefreshRates} must be a pointer to an array of {@code displayRefreshRateCapacityInput} {@code float} values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
*
*
*
* See Also
*
* {@link #xrGetDisplayRefreshRateFB GetDisplayRefreshRateFB}, {@link #xrRequestDisplayRefreshRateFB RequestDisplayRefreshRateFB}
*
* @param session the session that enumerates the supported display refresh rates.
* @param displayRefreshRateCountOutput a pointer to the count of {@code float} {@code displayRefreshRates} written, or a pointer to the required capacity in the case that {@code displayRefreshRateCapacityInput} is insufficient.
* @param displayRefreshRates a pointer to an array of {@code float} display refresh rates, but can be {@code NULL} if {@code displayRefreshRateCapacityInput} is 0.
*/
@NativeType("XrResult")
public static int xrEnumerateDisplayRefreshRatesFB(XrSession session, @NativeType("uint32_t *") IntBuffer displayRefreshRateCountOutput, @NativeType("float *") @Nullable FloatBuffer displayRefreshRates) {
if (CHECKS) {
check(displayRefreshRateCountOutput, 1);
}
return nxrEnumerateDisplayRefreshRatesFB(session, remainingSafe(displayRefreshRates), memAddress(displayRefreshRateCountOutput), memAddressSafe(displayRefreshRates));
}
// --- [ xrGetDisplayRefreshRateFB ] ---
/** Unsafe version of: {@link #xrGetDisplayRefreshRateFB GetDisplayRefreshRateFB} */
public static int nxrGetDisplayRefreshRateFB(XrSession session, long displayRefreshRate) {
long __functionAddress = session.getCapabilities().xrGetDisplayRefreshRateFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(session.address(), displayRefreshRate, __functionAddress);
}
/**
* Get the current display refresh rate.
*
* C Specification
*
* The {@link #xrGetDisplayRefreshRateFB GetDisplayRefreshRateFB} function is defined as:
*
*
* XrResult xrGetDisplayRefreshRateFB(
* XrSession session,
* float* displayRefreshRate);
*
* Description
*
* {@link #xrGetDisplayRefreshRateFB GetDisplayRefreshRateFB} retrieves the current display refresh rate.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBDisplayRefreshRate XR_FB_display_refresh_rate} extension must be enabled prior to calling {@link #xrGetDisplayRefreshRateFB GetDisplayRefreshRateFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code displayRefreshRate} must be a pointer to a {@code float} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link #xrEnumerateDisplayRefreshRatesFB EnumerateDisplayRefreshRatesFB}, {@link #xrRequestDisplayRefreshRateFB RequestDisplayRefreshRateFB}
*
* @param session the {@code XrSession} to query.
* @param displayRefreshRate a pointer to a float into which the current display refresh rate will be placed.
*/
@NativeType("XrResult")
public static int xrGetDisplayRefreshRateFB(XrSession session, @NativeType("float *") FloatBuffer displayRefreshRate) {
if (CHECKS) {
check(displayRefreshRate, 1);
}
return nxrGetDisplayRefreshRateFB(session, memAddress(displayRefreshRate));
}
// --- [ xrRequestDisplayRefreshRateFB ] ---
/**
* Request a display refresh rate.
*
* C Specification
*
* The {@link #xrRequestDisplayRefreshRateFB RequestDisplayRefreshRateFB} function is defined as:
*
*
* XrResult xrRequestDisplayRefreshRateFB(
* XrSession session,
* float displayRefreshRate);
*
* Description
*
* {@link #xrRequestDisplayRefreshRateFB RequestDisplayRefreshRateFB} provides a mechanism for an application to request the system to dynamically change the display refresh rate to the application preferred value. The runtime must return {@link #XR_ERROR_DISPLAY_REFRESH_RATE_UNSUPPORTED_FB ERROR_DISPLAY_REFRESH_RATE_UNSUPPORTED_FB} if {@code displayRefreshRate} is not either {@code 0.0f} or one of the values enumerated by {@link #xrEnumerateDisplayRefreshRatesFB EnumerateDisplayRefreshRatesFB}. A display refresh rate of {@code 0.0f} indicates the application has no preference.
*
* Note that this is only a request and does not guarantee the system will switch to the requested display refresh rate.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBDisplayRefreshRate XR_FB_display_refresh_rate} extension must be enabled prior to calling {@link #xrRequestDisplayRefreshRateFB RequestDisplayRefreshRateFB}
* - {@code session} must be a valid {@code XrSession} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link #XR_ERROR_DISPLAY_REFRESH_RATE_UNSUPPORTED_FB ERROR_DISPLAY_REFRESH_RATE_UNSUPPORTED_FB}
*
*
*
* See Also
*
* {@link #xrEnumerateDisplayRefreshRatesFB EnumerateDisplayRefreshRatesFB}, {@link #xrGetDisplayRefreshRateFB GetDisplayRefreshRateFB}
*
* @param session a valid {@code XrSession} handle.
* @param displayRefreshRate {@code 0.0f} or a supported display refresh rate. Supported display refresh rates are indicated by {@link #xrEnumerateDisplayRefreshRatesFB EnumerateDisplayRefreshRatesFB}.
*/
@NativeType("XrResult")
public static int xrRequestDisplayRefreshRateFB(XrSession session, float displayRefreshRate) {
long __functionAddress = session.getCapabilities().xrRequestDisplayRefreshRateFB;
if (CHECKS) {
check(__functionAddress);
}
return callPI(session.address(), displayRefreshRate, __functionAddress);
}
}