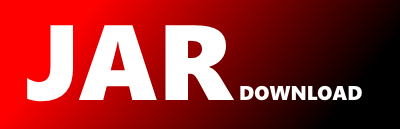
org.lwjgl.openxr.FBEyeTrackingSocial Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_FB_eye_tracking_social extension.
*
* This extension enables applications to obtain position and orientation of the user’s eyes. It enables applications to render eyes in XR experiences.
*
* This extension is intended to drive animation of avatar eyes. So, for that purpose, the runtimes may filter the poses in ways that are suitable for avatar eye interaction but detrimental to other use cases. This extension should not be used for other eye tracking purposes. For interaction, {@link EXTEyeGazeInteraction XR_EXT_eye_gaze_interaction} should be used.
*
* Eye tracking data is sensitive personal information and is closely linked to personal privacy and integrity. It is strongly recommended that applications that store or transfer eye tracking data always ask the user for active and specific acceptance to do so.
*
* If a runtime supports a permission system to control application access to the eye tracker, then the runtime must set the {@code isValid} field to {@link XR10#XR_FALSE FALSE} on the supplied {@link XrEyeGazeFB} structure until the application has been allowed access to the eye tracker. When the application access has been allowed, the runtime may set {@code isValid} on the supplied {@link XrEyeGazeFB} structure to {@link XR10#XR_TRUE TRUE}.
*/
public class FBEyeTrackingSocial {
/** The extension specification version. */
public static final int XR_FB_eye_tracking_social_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_FB_EYE_TRACKING_SOCIAL_EXTENSION_NAME = "XR_FB_eye_tracking_social";
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_EYE_TRACKER_FB = 1000202000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_EYE_TRACKER_CREATE_INFO_FB TYPE_EYE_TRACKER_CREATE_INFO_FB}
* - {@link #XR_TYPE_EYE_GAZES_INFO_FB TYPE_EYE_GAZES_INFO_FB}
* - {@link #XR_TYPE_EYE_GAZES_FB TYPE_EYE_GAZES_FB}
* - {@link #XR_TYPE_SYSTEM_EYE_TRACKING_PROPERTIES_FB TYPE_SYSTEM_EYE_TRACKING_PROPERTIES_FB}
*
*/
public static final int
XR_TYPE_EYE_TRACKER_CREATE_INFO_FB = 1000202001,
XR_TYPE_EYE_GAZES_INFO_FB = 1000202002,
XR_TYPE_EYE_GAZES_FB = 1000202003,
XR_TYPE_SYSTEM_EYE_TRACKING_PROPERTIES_FB = 1000202004;
/**
* XrEyePositionFB - Enumerates eyes.
*
* Enumerant Descriptions
*
*
* - {@link #XR_EYE_POSITION_LEFT_FB EYE_POSITION_LEFT_FB} — Specifies the position of the left eye.
* - {@link #XR_EYE_POSITION_RIGHT_FB EYE_POSITION_RIGHT_FB} — Specifies the position of the right eye.
*
*
* See Also
*
* {@link XrEyeGazesFB}
*
* Enum values:
*
*
* - {@link #XR_EYE_POSITION_COUNT_FB EYE_POSITION_COUNT_FB}
*
*/
public static final int
XR_EYE_POSITION_LEFT_FB = 0,
XR_EYE_POSITION_RIGHT_FB = 1,
XR_EYE_POSITION_COUNT_FB = 2;
protected FBEyeTrackingSocial() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateEyeTrackerFB ] ---
/** Unsafe version of: {@link #xrCreateEyeTrackerFB CreateEyeTrackerFB} */
public static int nxrCreateEyeTrackerFB(XrSession session, long createInfo, long eyeTracker) {
long __functionAddress = session.getCapabilities().xrCreateEyeTrackerFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, eyeTracker, __functionAddress);
}
/**
* Create an eye gaze handle.
*
* C Specification
*
* An application creates an {@code XrEyeTrackerFB} handle using {@link #xrCreateEyeTrackerFB CreateEyeTrackerFB} function.
*
*
* XrResult xrCreateEyeTrackerFB(
* XrSession session,
* const XrEyeTrackerCreateInfoFB* createInfo,
* XrEyeTrackerFB* eyeTracker);
*
* Description
*
* If the system does not support eye tracking, the runtime must return {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED} from {@link #xrCreateEyeTrackerFB CreateEyeTrackerFB}. In this case, the runtime must return {@link XR10#XR_FALSE FALSE} for {@link XrSystemEyeTrackingPropertiesFB}{@code ::supportsEyeTracking} when the function {@link XR10#xrGetSystemProperties GetSystemProperties} is called, so that the application can avoid creating an eye tracker.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBEyeTrackingSocial XR_FB_eye_tracking_social} extension must be enabled prior to calling {@link #xrCreateEyeTrackerFB CreateEyeTrackerFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrEyeTrackerCreateInfoFB} structure
* - {@code eyeTracker} must be a pointer to an {@code XrEyeTrackerFB} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrEyeTrackerCreateInfoFB}
*
* @param session an {@code XrSession} in which the eye tracker will be active.
* @param createInfo the {@link XrEyeTrackerCreateInfoFB} used to specify the eye tracker.
* @param eyeTracker the returned {@code XrEyeTrackerFB} handle.
*/
@NativeType("XrResult")
public static int xrCreateEyeTrackerFB(XrSession session, @NativeType("XrEyeTrackerCreateInfoFB const *") XrEyeTrackerCreateInfoFB createInfo, @NativeType("XrEyeTrackerFB *") PointerBuffer eyeTracker) {
if (CHECKS) {
check(eyeTracker, 1);
}
return nxrCreateEyeTrackerFB(session, createInfo.address(), memAddress(eyeTracker));
}
// --- [ xrDestroyEyeTrackerFB ] ---
/**
* Destroy an eye gaze handle.
*
* C Specification
*
* {@link #xrDestroyEyeTrackerFB DestroyEyeTrackerFB} function releases the {@code eyeTracker} and the underlying resources when the eye tracking experience is over.
*
*
* XrResult xrDestroyEyeTrackerFB(
* XrEyeTrackerFB eyeTracker);
*
* Valid Usage (Implicit)
*
*
* - The {@link FBEyeTrackingSocial XR_FB_eye_tracking_social} extension must be enabled prior to calling {@link #xrDestroyEyeTrackerFB DestroyEyeTrackerFB}
* - {@code eyeTracker} must be a valid {@code XrEyeTrackerFB} handle
*
*
* Thread Safety
*
*
* - Access to {@code eyeTracker}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* @param eyeTracker an {@code XrEyeTrackerFB} previously created by {@link #xrCreateEyeTrackerFB CreateEyeTrackerFB}.
*/
@NativeType("XrResult")
public static int xrDestroyEyeTrackerFB(XrEyeTrackerFB eyeTracker) {
long __functionAddress = eyeTracker.getCapabilities().xrDestroyEyeTrackerFB;
if (CHECKS) {
check(__functionAddress);
}
return callPI(eyeTracker.address(), __functionAddress);
}
// --- [ xrGetEyeGazesFB ] ---
/** Unsafe version of: {@link #xrGetEyeGazesFB GetEyeGazesFB} */
public static int nxrGetEyeGazesFB(XrEyeTrackerFB eyeTracker, long gazeInfo, long eyeGazes) {
long __functionAddress = eyeTracker.getCapabilities().xrGetEyeGazesFB;
if (CHECKS) {
check(__functionAddress);
XrEyeGazesInfoFB.validate(gazeInfo);
}
return callPPPI(eyeTracker.address(), gazeInfo, eyeGazes, __functionAddress);
}
/**
* Locate eye gaze directions.
*
* C Specification
*
* The {@link #xrGetEyeGazesFB GetEyeGazesFB} function is defined as:
*
*
* XrResult xrGetEyeGazesFB(
* XrEyeTrackerFB eyeTracker,
* const XrEyeGazesInfoFB* gazeInfo,
* XrEyeGazesFB* eyeGazes);
*
* Description
*
* The {@link #xrGetEyeGazesFB GetEyeGazesFB} function obtains pose for a user’s eyes at a specific time and within a specific coordinate system.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBEyeTrackingSocial XR_FB_eye_tracking_social} extension must be enabled prior to calling {@link #xrGetEyeGazesFB GetEyeGazesFB}
* - {@code eyeTracker} must be a valid {@code XrEyeTrackerFB} handle
* - {@code gazeInfo} must be a pointer to a valid {@link XrEyeGazesInfoFB} structure
* - {@code eyeGazes} must be a pointer to an {@link XrEyeGazesFB} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
*
*
*
* See Also
*
* {@link XrEyeGazesFB}, {@link XrEyeGazesInfoFB}
*
* @param eyeTracker an {@code XrEyeTrackerFB} previously created by {@link #xrCreateEyeTrackerFB CreateEyeTrackerFB}.
* @param gazeInfo the information to get eye gaze.
* @param eyeGazes a pointer to {@link XrEyeGazesFB} receiving the returned eye poses and confidence.
*/
@NativeType("XrResult")
public static int xrGetEyeGazesFB(XrEyeTrackerFB eyeTracker, @NativeType("XrEyeGazesInfoFB const *") XrEyeGazesInfoFB gazeInfo, @NativeType("XrEyeGazesFB *") XrEyeGazesFB eyeGazes) {
return nxrGetEyeGazesFB(eyeTracker, gazeInfo.address(), eyeGazes.address());
}
}