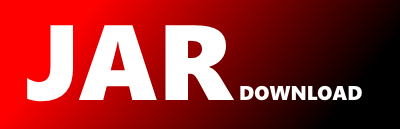
org.lwjgl.openxr.FBFoveation Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_FB_foveation extension.
*
* Foveation in the context of XR is a rendering technique that allows the area of an image near the focal point or fovea of the eye to be displayed at higher resolution than areas in the periphery. This trades some visual fidelity in the periphery, where it is less noticeable for the user, for improved rendering performance, most notably regarding the fragment shader, as fewer pixels or subpixels in the periphery need to be shaded and processed. On platforms which support foveation patterns and features tailored towards the optical properties, performance profiles, and hardware support of specific HMDs, application developers may request and use available foveation profiles from the runtime. Foveation profiles refer to a set of properties describing how, when, and where foveation will be applied.
*
* This extension allows:
*
*
* - An application to create swapchains that can support foveation for its graphics API.
* - An application to request foveation profiles supported by the runtime and apply them to foveation-supported swapchains.
*
*
* In order to enable the functionality of this extension, you must pass the name of the extension into {@link XR10#xrCreateInstance CreateInstance} via the {@link XrInstanceCreateInfo} {@code enabledExtensionNames} parameter as indicated in the extension section.
*/
public class FBFoveation {
/** The extension specification version. */
public static final int XR_FB_foveation_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_FB_FOVEATION_EXTENSION_NAME = "XR_FB_foveation";
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_FOVEATION_PROFILE_FB = 1000114000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_FOVEATION_PROFILE_CREATE_INFO_FB TYPE_FOVEATION_PROFILE_CREATE_INFO_FB}
* - {@link #XR_TYPE_SWAPCHAIN_CREATE_INFO_FOVEATION_FB TYPE_SWAPCHAIN_CREATE_INFO_FOVEATION_FB}
* - {@link #XR_TYPE_SWAPCHAIN_STATE_FOVEATION_FB TYPE_SWAPCHAIN_STATE_FOVEATION_FB}
*
*/
public static final int
XR_TYPE_FOVEATION_PROFILE_CREATE_INFO_FB = 1000114000,
XR_TYPE_SWAPCHAIN_CREATE_INFO_FOVEATION_FB = 1000114001,
XR_TYPE_SWAPCHAIN_STATE_FOVEATION_FB = 1000114002;
/**
* XrSwapchainCreateFoveationFlagBitsFB - XrSwapchainCreateFoveationFlagBitsFB
*
* Flag Descriptions
*
*
* - {@link #XR_SWAPCHAIN_CREATE_FOVEATION_SCALED_BIN_BIT_FB SWAPCHAIN_CREATE_FOVEATION_SCALED_BIN_BIT_FB} — Explicitly create the swapchain with scaled bin foveation support. The application must ensure that the swapchain is using the OpenGL graphics API and that the QCOM_texture_foveated extension is supported and enabled.
* - {@link #XR_SWAPCHAIN_CREATE_FOVEATION_FRAGMENT_DENSITY_MAP_BIT_FB SWAPCHAIN_CREATE_FOVEATION_FRAGMENT_DENSITY_MAP_BIT_FB} — Explicitly create the swapchain with fragment density map foveation support. The application must ensure that the swapchain is using the Vulkan graphics API and that the VK_EXT_fragment_density_map extension is supported and enabled.
*
*/
public static final int
XR_SWAPCHAIN_CREATE_FOVEATION_SCALED_BIN_BIT_FB = 0x1,
XR_SWAPCHAIN_CREATE_FOVEATION_FRAGMENT_DENSITY_MAP_BIT_FB = 0x2;
protected FBFoveation() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateFoveationProfileFB ] ---
/** Unsafe version of: {@link #xrCreateFoveationProfileFB CreateFoveationProfileFB} */
public static int nxrCreateFoveationProfileFB(XrSession session, long createInfo, long profile) {
long __functionAddress = session.getCapabilities().xrCreateFoveationProfileFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, profile, __functionAddress);
}
/**
* Create a foveation profile.
*
* C Specification
*
* The {@link #xrCreateFoveationProfileFB CreateFoveationProfileFB} function is defined as:
*
*
* XrResult xrCreateFoveationProfileFB(
* XrSession session,
* const XrFoveationProfileCreateInfoFB* createInfo,
* XrFoveationProfileFB* profile);
*
* Description
*
* Creates an {@code XrFoveationProfileFB} handle. The returned foveation profile handle may be subsequently used in API calls.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBFoveation XR_FB_foveation} extension must be enabled prior to calling {@link #xrCreateFoveationProfileFB CreateFoveationProfileFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrFoveationProfileCreateInfoFB} structure
* - {@code profile} must be a pointer to an {@code XrFoveationProfileFB} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* See Also
*
* {@link XrFoveationProfileCreateInfoFB}
*
* @param session the {@code XrSession} that created the swapchains to which this foveation profile will be applied.
* @param createInfo a pointer to an {@link XrFoveationProfileCreateInfoFB} structure containing parameters to be used to create the foveation profile.
* @param profile a pointer to a handle in which the created {@code XrFoveationProfileFB} is returned.
*/
@NativeType("XrResult")
public static int xrCreateFoveationProfileFB(XrSession session, @NativeType("XrFoveationProfileCreateInfoFB const *") XrFoveationProfileCreateInfoFB createInfo, @NativeType("XrFoveationProfileFB *") PointerBuffer profile) {
if (CHECKS) {
check(profile, 1);
}
return nxrCreateFoveationProfileFB(session, createInfo.address(), memAddress(profile));
}
// --- [ xrDestroyFoveationProfileFB ] ---
/**
* Destroy a foveation profile.
*
* C Specification
*
* The {@link #xrDestroyFoveationProfileFB DestroyFoveationProfileFB} function is defined as:
*
*
* XrResult xrDestroyFoveationProfileFB(
* XrFoveationProfileFB profile);
*
* Description
*
* {@code XrFoveationProfileFB} handles are destroyed using {@link #xrDestroyFoveationProfileFB DestroyFoveationProfileFB}. A {@code XrFoveationProfileFB} may be safely destroyed after being applied to a swapchain state using {@link FBSwapchainUpdateState#xrUpdateSwapchainFB UpdateSwapchainFB} without affecting the foveation parameters of the swapchain. The application is responsible for ensuring that it has no calls using profile in progress when the foveation profile is destroyed.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBFoveation XR_FB_foveation} extension must be enabled prior to calling {@link #xrDestroyFoveationProfileFB DestroyFoveationProfileFB}
* - {@code profile} must be a valid {@code XrFoveationProfileFB} handle
*
*
* Thread Safety
*
*
* - Access to {@code profile}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* @param profile the {@code XrFoveationProfileFB} to destroy.
*/
@NativeType("XrResult")
public static int xrDestroyFoveationProfileFB(XrFoveationProfileFB profile) {
long __functionAddress = profile.getCapabilities().xrDestroyFoveationProfileFB;
if (CHECKS) {
check(__functionAddress);
}
return callPI(profile.address(), __functionAddress);
}
}