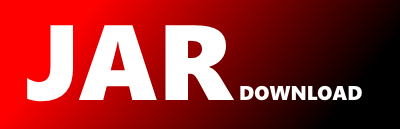
org.lwjgl.openxr.FBHapticPcm Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The XR_FB_haptic_pcm extension.
*
* This extension enables applications to trigger haptic effects using Pulse Code Modulation (PCM) buffers.
*/
public class FBHapticPcm {
/** The extension specification version. */
public static final int XR_FB_haptic_pcm_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_FB_HAPTIC_PCM_EXTENSION_NAME = "XR_FB_haptic_pcm";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_HAPTIC_PCM_VIBRATION_FB TYPE_HAPTIC_PCM_VIBRATION_FB}
* - {@link #XR_TYPE_DEVICE_PCM_SAMPLE_RATE_STATE_FB TYPE_DEVICE_PCM_SAMPLE_RATE_STATE_FB}
* - {@link #XR_TYPE_DEVICE_PCM_SAMPLE_RATE_GET_INFO_FB TYPE_DEVICE_PCM_SAMPLE_RATE_GET_INFO_FB}
*
*/
public static final int
XR_TYPE_HAPTIC_PCM_VIBRATION_FB = 1000209001,
XR_TYPE_DEVICE_PCM_SAMPLE_RATE_STATE_FB = 1000209002,
XR_TYPE_DEVICE_PCM_SAMPLE_RATE_GET_INFO_FB = 1000209002;
/** API Constants */
public static final int XR_MAX_HAPTIC_PCM_BUFFER_SIZE_FB = 0xFA0;
protected FBHapticPcm() {
throw new UnsupportedOperationException();
}
// --- [ xrGetDeviceSampleRateFB ] ---
/** Unsafe version of: {@link #xrGetDeviceSampleRateFB GetDeviceSampleRateFB} */
public static int nxrGetDeviceSampleRateFB(XrSession session, long hapticActionInfo, long deviceSampleRate) {
long __functionAddress = session.getCapabilities().xrGetDeviceSampleRateFB;
if (CHECKS) {
check(__functionAddress);
XrHapticActionInfo.validate(hapticActionInfo);
}
return callPPPI(session.address(), hapticActionInfo, deviceSampleRate, __functionAddress);
}
/**
* Get device sample rate.
*
* C Specification
*
*
* XrResult xrGetDeviceSampleRateFB(
* XrSession session,
* const XrHapticActionInfo* hapticActionInfo,
* XrDevicePcmSampleRateGetInfoFB* deviceSampleRate);
*
* Description
*
* The runtime must use the {@code hapticActionInfo} to get the sample rate of the currently bound device on which haptics is triggered and populate the {@code deviceSampleRate} structure. The device is determined by the {@link XrHapticActionInfo}{@code ::action} and {@link XrHapticActionInfo}{@code ::subactionPath}. If the {@code hapticActionInfo} is bound to more than one device, then runtime should assume that the all these bound devices have the same {@code deviceSampleRate} and the runtime should return the sampleRate for any of those bound devices. If the device is invalid, the runtime must populate the {@code deviceSampleRate} of {@link XrDevicePcmSampleRateStateFB} as 0. A device can be invalid if the runtime does not find any device (which can play haptics) connected to the headset, or if the device does not support PCM haptic effect.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBHapticPcm XR_FB_haptic_pcm} extension must be enabled prior to calling {@link #xrGetDeviceSampleRateFB GetDeviceSampleRateFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code hapticActionInfo} must be a pointer to a valid {@link XrHapticActionInfo} structure
* - {@code deviceSampleRate} must be a pointer to an {@link XrDevicePcmSampleRateGetInfoFB} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
* - {@link XR10#XR_ERROR_ACTION_TYPE_MISMATCH ERROR_ACTION_TYPE_MISMATCH}
* - {@link XR10#XR_ERROR_ACTIONSET_NOT_ATTACHED ERROR_ACTIONSET_NOT_ATTACHED}
*
*
*
* See Also
*
* {@link XrDevicePcmSampleRateGetInfoFB}, {@link XrDevicePcmSampleRateStateFB}, {@link XrHapticActionInfo}
*
* @param session the specified {@code XrSession}.
* @param hapticActionInfo the {@link XrHapticActionInfo} used to provide action and subaction paths
* @param deviceSampleRate a pointer to {@link XrDevicePcmSampleRateStateFB} which is populated by the runtime.
*/
@NativeType("XrResult")
public static int xrGetDeviceSampleRateFB(XrSession session, @NativeType("XrHapticActionInfo const *") XrHapticActionInfo hapticActionInfo, @NativeType("XrDevicePcmSampleRateGetInfoFB *") XrDevicePcmSampleRateGetInfoFB deviceSampleRate) {
return nxrGetDeviceSampleRateFB(session, hapticActionInfo.address(), deviceSampleRate.address());
}
}