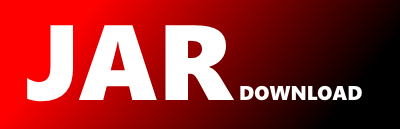
org.lwjgl.openxr.FBRenderModel Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_FB_render_model extension.
*
* This extension allows applications to request GLTF models for certain connected devices supported by the runtime. Paths that correspond to these devices will be provided through the extension and can be used to get information about the models as well as loading them.
*/
public class FBRenderModel {
/** The extension specification version. */
public static final int XR_FB_render_model_SPEC_VERSION = 4;
/** The extension name. */
public static final String XR_FB_RENDER_MODEL_EXTENSION_NAME = "XR_FB_render_model";
/** XR_MAX_RENDER_MODEL_NAME_SIZE_FB */
public static final int XR_MAX_RENDER_MODEL_NAME_SIZE_FB = 64;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_RENDER_MODEL_PATH_INFO_FB TYPE_RENDER_MODEL_PATH_INFO_FB}
* - {@link #XR_TYPE_RENDER_MODEL_PROPERTIES_FB TYPE_RENDER_MODEL_PROPERTIES_FB}
* - {@link #XR_TYPE_RENDER_MODEL_BUFFER_FB TYPE_RENDER_MODEL_BUFFER_FB}
* - {@link #XR_TYPE_RENDER_MODEL_LOAD_INFO_FB TYPE_RENDER_MODEL_LOAD_INFO_FB}
* - {@link #XR_TYPE_SYSTEM_RENDER_MODEL_PROPERTIES_FB TYPE_SYSTEM_RENDER_MODEL_PROPERTIES_FB}
* - {@link #XR_TYPE_RENDER_MODEL_CAPABILITIES_REQUEST_FB TYPE_RENDER_MODEL_CAPABILITIES_REQUEST_FB}
*
*/
public static final int
XR_TYPE_RENDER_MODEL_PATH_INFO_FB = 1000119000,
XR_TYPE_RENDER_MODEL_PROPERTIES_FB = 1000119001,
XR_TYPE_RENDER_MODEL_BUFFER_FB = 1000119002,
XR_TYPE_RENDER_MODEL_LOAD_INFO_FB = 1000119003,
XR_TYPE_SYSTEM_RENDER_MODEL_PROPERTIES_FB = 1000119004,
XR_TYPE_RENDER_MODEL_CAPABILITIES_REQUEST_FB = 1000119005;
/**
* Extends {@code XrResult}.
*
* Enum values:
*
*
* - {@link #XR_ERROR_RENDER_MODEL_KEY_INVALID_FB ERROR_RENDER_MODEL_KEY_INVALID_FB}
* - {@link #XR_RENDER_MODEL_UNAVAILABLE_FB RENDER_MODEL_UNAVAILABLE_FB}
*
*/
public static final int
XR_ERROR_RENDER_MODEL_KEY_INVALID_FB = -1000119000,
XR_RENDER_MODEL_UNAVAILABLE_FB = 1000119020;
/**
* XrRenderModelFlagBitsFB - XrRenderModelFlagBitsFB
*
* Flag Descriptions
*
*
* - {@link #XR_RENDER_MODEL_SUPPORTS_GLTF_2_0_SUBSET_1_BIT_FB RENDER_MODEL_SUPPORTS_GLTF_2_0_SUBSET_1_BIT_FB} — Minimal level of support. Can only contain a single mesh. Can only contain a single texture. Can not contain transparency. Assumes unlit rendering. Requires Extension KHR_texturebasisu.
* - {@link #XR_RENDER_MODEL_SUPPORTS_GLTF_2_0_SUBSET_2_BIT_FB RENDER_MODEL_SUPPORTS_GLTF_2_0_SUBSET_2_BIT_FB} — All of XR_RENDER_MODEL_SUPPORTS_GLTF_2_0_SUBSET_1_BIT_FB support plus: Multiple meshes. Multiple Textures. Texture Transparency.
*
*
* Render Model Support Levels: An application should request a model of a certain complexity via the {@link XrRenderModelCapabilitiesRequestFB} on the structure chain of {@link XrRenderModelPropertiesFB} passed into {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB}. The flags on the {@link XrRenderModelCapabilitiesRequestFB} are an acknowledgement of the application’s ability to render such a model. Multiple values of {@code XrRenderModelFlagBitsFB} can be set on this variable to indicate acceptance of different support levels. The flags parameter on the {@link XrRenderModelPropertiesFB} will indicate what capabilities the model in the runtime actually requires. It will be set to a single value of {@code XrRenderModelFlagBitsFB}.
*/
public static final int
XR_RENDER_MODEL_SUPPORTS_GLTF_2_0_SUBSET_1_BIT_FB = 0x1,
XR_RENDER_MODEL_SUPPORTS_GLTF_2_0_SUBSET_2_BIT_FB = 0x2;
/** API Constants */
public static final long XR_NULL_RENDER_MODEL_KEY_FB = 0x0L;
protected FBRenderModel() {
throw new UnsupportedOperationException();
}
// --- [ xrEnumerateRenderModelPathsFB ] ---
/**
* Unsafe version of: {@link #xrEnumerateRenderModelPathsFB EnumerateRenderModelPathsFB}
*
* @param pathCapacityInput the capacity of the {@code paths}, or 0 to retrieve the required capacity.
*/
public static int nxrEnumerateRenderModelPathsFB(XrSession session, int pathCapacityInput, long pathCountOutput, long paths) {
long __functionAddress = session.getCapabilities().xrEnumerateRenderModelPathsFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), pathCapacityInput, pathCountOutput, paths, __functionAddress);
}
/**
* Enumerate supported render model paths.
*
* C Specification
*
* The {@link #xrEnumerateRenderModelPathsFB EnumerateRenderModelPathsFB} function is defined as:
*
*
* XrResult xrEnumerateRenderModelPathsFB(
* XrSession session,
* uint32_t pathCapacityInput,
* uint32_t* pathCountOutput,
* XrRenderModelPathInfoFB* paths);
*
* Description
*
* The application must call {@link #xrEnumerateRenderModelPathsFB EnumerateRenderModelPathsFB} to enumerate the valid render model paths that are supported by the runtime before calling {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB}. The paths returned may be used later in {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB}.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBRenderModel XR_FB_render_model} extension must be enabled prior to calling {@link #xrEnumerateRenderModelPathsFB EnumerateRenderModelPathsFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code pathCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code pathCapacityInput} is not 0, {@code paths} must be a pointer to an array of {@code pathCapacityInput} {@link XrRenderModelPathInfoFB} structures
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
*
*
*
* See Also
*
* {@link XrRenderModelPathInfoFB}
*
* @param session the specified {@code XrSession}.
* @param pathCountOutput a pointer to the count of {@code float} {@code paths} written, or a pointer to the required capacity in the case that {@code pathCapacityInput} is insufficient.
* @param paths a pointer to an application-allocated array that will be filled with {@link XrRenderModelPathInfoFB} values that are supported by the runtime, but can be {@code NULL} if {@code pathCapacityInput} is 0
*/
@NativeType("XrResult")
public static int xrEnumerateRenderModelPathsFB(XrSession session, @NativeType("uint32_t *") IntBuffer pathCountOutput, @NativeType("XrRenderModelPathInfoFB *") XrRenderModelPathInfoFB.@Nullable Buffer paths) {
if (CHECKS) {
check(pathCountOutput, 1);
}
return nxrEnumerateRenderModelPathsFB(session, remainingSafe(paths), memAddress(pathCountOutput), memAddressSafe(paths));
}
// --- [ xrGetRenderModelPropertiesFB ] ---
/** Unsafe version of: {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB} */
public static int nxrGetRenderModelPropertiesFB(XrSession session, long path, long properties) {
long __functionAddress = session.getCapabilities().xrGetRenderModelPropertiesFB;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(session.address(), path, properties, __functionAddress);
}
/**
* Get information for a render model.
*
* C Specification
*
* The {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB} function is defined as:
*
*
* XrResult xrGetRenderModelPropertiesFB(
* XrSession session,
* XrPath path,
* XrRenderModelPropertiesFB* properties);
*
* Description
*
* {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB} is used for getting information for a render model using a path retrieved from {@link #xrEnumerateRenderModelPathsFB EnumerateRenderModelPathsFB}. The information returned will be for the connected device that corresponds to the path given. For example, using pathname:/model_fb/controller/left will return information for the left controller that is currently connected and will change if a different device that also represents a left controller is connected.
*
* The runtime must return {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID} if {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB} is called with render model paths before calling {@link #xrEnumerateRenderModelPathsFB EnumerateRenderModelPathsFB}. The runtime must return {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID} if a path not given by {@link #xrEnumerateRenderModelPathsFB EnumerateRenderModelPathsFB} is used.
*
* If {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB} returns a success code of {@link #XR_RENDER_MODEL_UNAVAILABLE_FB RENDER_MODEL_UNAVAILABLE_FB} and has a {@link XrRenderModelPropertiesFB}{@code ::modelKey} of {@link #XR_NULL_RENDER_MODEL_KEY_FB NULL_RENDER_MODEL_KEY_FB}, this indicates that the model for the device is unavailable. The application may keep calling {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB} because the model may become available later when a device is connected.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBRenderModel XR_FB_render_model} extension must be enabled prior to calling {@link #xrGetRenderModelPropertiesFB GetRenderModelPropertiesFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code properties} must be a pointer to an {@link XrRenderModelPropertiesFB} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
* - {@link #XR_RENDER_MODEL_UNAVAILABLE_FB RENDER_MODEL_UNAVAILABLE_FB}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
* - {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID}
*
*
*
* See Also
*
* {@link XrRenderModelPropertiesFB}
*
* @param session the specified {@code XrSession}.
* @param path the path of the render model to get the properties for.
* @param properties a pointer to the {@link XrRenderModelPropertiesFB} to write the render model information to.
*/
@NativeType("XrResult")
public static int xrGetRenderModelPropertiesFB(XrSession session, @NativeType("XrPath") long path, @NativeType("XrRenderModelPropertiesFB *") XrRenderModelPropertiesFB properties) {
return nxrGetRenderModelPropertiesFB(session, path, properties.address());
}
// --- [ xrLoadRenderModelFB ] ---
/** Unsafe version of: {@link #xrLoadRenderModelFB LoadRenderModelFB} */
public static int nxrLoadRenderModelFB(XrSession session, long info, long buffer) {
long __functionAddress = session.getCapabilities().xrLoadRenderModelFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), info, buffer, __functionAddress);
}
/**
* Load binary data for a render model.
*
* C Specification
*
* The {@link #xrLoadRenderModelFB LoadRenderModelFB} function is defined as:
*
*
* XrResult xrLoadRenderModelFB(
* XrSession session,
* const XrRenderModelLoadInfoFB* info,
* XrRenderModelBufferFB* buffer);
*
* Description
*
* {@link #xrLoadRenderModelFB LoadRenderModelFB} is used to load the GLTF model data using a valid {@link XrRenderModelLoadInfoFB}{@code ::modelKey}. {@link #xrLoadRenderModelFB LoadRenderModelFB} loads the model as a byte buffer containing the GLTF in the binary format (GLB). The GLB data must conform to the glTF 2.0 format defined at https://registry.khronos.org/glTF/specs/2.0/glTF-2.0.html. The GLB may contain texture data in a format that requires the use of the {@code KHR_texture_basisu} GLTF extension defined at https://github.com/KhronosGroup/glTF/tree/main/extensions/2.0/Khronos/KHR_texture_basisu. Therefore, the application should ensure it can handle this extension.
*
* If the device for the requested model is disconnected or does not match the {@link XrRenderModelLoadInfoFB}{@code ::modelKey} provided, {@link #xrLoadRenderModelFB LoadRenderModelFB} must return {@link #XR_RENDER_MODEL_UNAVAILABLE_FB RENDER_MODEL_UNAVAILABLE_FB} as well as an {@link XrRenderModelBufferFB}{@code ::bufferCountOutput} value of 0 indicating that the model was not available.
*
* The {@link #xrLoadRenderModelFB LoadRenderModelFB} function may be slow, therefore applications should call it from a non-time sensitive thread.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBRenderModel XR_FB_render_model} extension must be enabled prior to calling {@link #xrLoadRenderModelFB LoadRenderModelFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrRenderModelLoadInfoFB} structure
* - {@code buffer} must be a pointer to an {@link XrRenderModelBufferFB} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
* - {@link #XR_RENDER_MODEL_UNAVAILABLE_FB RENDER_MODEL_UNAVAILABLE_FB}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link #XR_ERROR_RENDER_MODEL_KEY_INVALID_FB ERROR_RENDER_MODEL_KEY_INVALID_FB}
*
*
*
* See Also
*
* {@link XrRenderModelBufferFB}, {@link XrRenderModelLoadInfoFB}
*
* @param session the specified {@code XrSession}.
* @param info a pointer to the {@link XrRenderModelLoadInfoFB} structure.
* @param buffer a pointer to the {@link XrRenderModelBufferFB} structure to write the binary data into.
*/
@NativeType("XrResult")
public static int xrLoadRenderModelFB(XrSession session, @NativeType("XrRenderModelLoadInfoFB const *") XrRenderModelLoadInfoFB info, @NativeType("XrRenderModelBufferFB *") XrRenderModelBufferFB buffer) {
return nxrLoadRenderModelFB(session, info.address(), buffer.address());
}
}