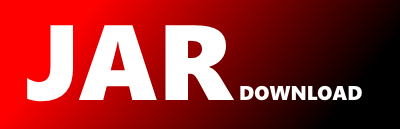
org.lwjgl.openxr.FBSpatialEntityQuery Maven / Gradle / Ivy
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_FB_spatial_entity_query extension.
*
* This extension enables an application to discover persistent spatial entities in the area and restore them. Using the query system, the application can load persistent spatial entities from storage. The query system consists of a set of filters to define the spatial entity search query and an operation that needs to be performed on the search results.
*
* In order to enable the functionality of this extension, you must pass the name of the extension into {@link XR10#xrCreateInstance CreateInstance} via the {@link XrInstanceCreateInfo}{@code ::enabledExtensionNames} parameter as indicated in the extension section.
*/
public class FBSpatialEntityQuery {
/** The extension specification version. */
public static final int XR_FB_spatial_entity_query_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_FB_SPATIAL_ENTITY_QUERY_EXTENSION_NAME = "XR_FB_spatial_entity_query";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SPACE_QUERY_INFO_FB TYPE_SPACE_QUERY_INFO_FB}
* - {@link #XR_TYPE_SPACE_QUERY_RESULTS_FB TYPE_SPACE_QUERY_RESULTS_FB}
* - {@link #XR_TYPE_SPACE_STORAGE_LOCATION_FILTER_INFO_FB TYPE_SPACE_STORAGE_LOCATION_FILTER_INFO_FB}
* - {@link #XR_TYPE_SPACE_UUID_FILTER_INFO_FB TYPE_SPACE_UUID_FILTER_INFO_FB}
* - {@link #XR_TYPE_SPACE_COMPONENT_FILTER_INFO_FB TYPE_SPACE_COMPONENT_FILTER_INFO_FB}
* - {@link #XR_TYPE_EVENT_DATA_SPACE_QUERY_RESULTS_AVAILABLE_FB TYPE_EVENT_DATA_SPACE_QUERY_RESULTS_AVAILABLE_FB}
* - {@link #XR_TYPE_EVENT_DATA_SPACE_QUERY_COMPLETE_FB TYPE_EVENT_DATA_SPACE_QUERY_COMPLETE_FB}
*
*/
public static final int
XR_TYPE_SPACE_QUERY_INFO_FB = 1000156001,
XR_TYPE_SPACE_QUERY_RESULTS_FB = 1000156002,
XR_TYPE_SPACE_STORAGE_LOCATION_FILTER_INFO_FB = 1000156003,
XR_TYPE_SPACE_UUID_FILTER_INFO_FB = 1000156054,
XR_TYPE_SPACE_COMPONENT_FILTER_INFO_FB = 1000156052,
XR_TYPE_EVENT_DATA_SPACE_QUERY_RESULTS_AVAILABLE_FB = 1000156103,
XR_TYPE_EVENT_DATA_SPACE_QUERY_COMPLETE_FB = 1000156104;
/**
* XrSpaceQueryActionFB - Type of query being performed
*
* Description
*
* Specify the type of query being performed.
*
* Enumerant Descriptions
*
*
* - {@link #XR_SPACE_QUERY_ACTION_LOAD_FB SPACE_QUERY_ACTION_LOAD_FB} — Tells the query to perform a load operation on any {@code XrSpace} returned by the query.
*
*
* See Also
*
* {@link XrSpaceQueryInfoFB}
*/
public static final int XR_SPACE_QUERY_ACTION_LOAD_FB = 0;
/**
* XrSpaceStorageLocationFB - Storage location to persist spatial entities
*
* Description
*
* The {@code XrSpaceStorageLocationFB} enumeration contains the storage locations used to store, erase, and query spatial entities.
*
* Enumerant Descriptions
*
*
* - {@link #XR_SPACE_STORAGE_LOCATION_INVALID_FB SPACE_STORAGE_LOCATION_INVALID_FB} — Invalid storage location
* - {@link #XR_SPACE_STORAGE_LOCATION_LOCAL_FB SPACE_STORAGE_LOCATION_LOCAL_FB} — Local device storage
* - {@link #XR_SPACE_STORAGE_LOCATION_CLOUD_FB SPACE_STORAGE_LOCATION_CLOUD_FB} — Cloud storage
*
*
* See Also
*
* {@link XrEventDataSpaceEraseCompleteFB}, {@link XrEventDataSpaceSaveCompleteFB}, {@link XrSpaceEraseInfoFB}, {@link XrSpaceListSaveInfoFB}, {@link XrSpaceSaveInfoFB}, {@link XrSpaceStorageLocationFilterInfoFB}
*/
public static final int
XR_SPACE_STORAGE_LOCATION_INVALID_FB = 0,
XR_SPACE_STORAGE_LOCATION_LOCAL_FB = 1,
XR_SPACE_STORAGE_LOCATION_CLOUD_FB = 2;
protected FBSpatialEntityQuery() {
throw new UnsupportedOperationException();
}
// --- [ xrQuerySpacesFB ] ---
/** Unsafe version of: {@link #xrQuerySpacesFB QuerySpacesFB} */
public static int nxrQuerySpacesFB(XrSession session, long info, long requestId) {
long __functionAddress = session.getCapabilities().xrQuerySpacesFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), info, requestId, __functionAddress);
}
/**
* Queries for spatial entities.
*
* C Specification
*
* The {@link #xrQuerySpacesFB QuerySpacesFB} function is defined as:
*
*
* XrResult xrQuerySpacesFB(
* XrSession session,
* const XrSpaceQueryInfoBaseHeaderFB* info,
* XrAsyncRequestIdFB* requestId);
*
* Description
*
* The {@link #xrQuerySpacesFB QuerySpacesFB} function enables an application to find and retrieve spatial entities from storage. Cast an {@link XrSpaceQueryInfoFB} pointer to a {@link XrSpaceQueryInfoBaseHeaderFB} pointer to pass as {@code info}. The application should keep the returned {@code requestId} for the duration of the request as it is used to refer to the request when calling {@link #xrRetrieveSpaceQueryResultsFB RetrieveSpaceQueryResultsFB} and is used to map completion events to the request. This operation is asynchronous and the runtime must post an {@link XrEventDataSpaceQueryCompleteFB} event when the operation completes successfully or encounters an error. If this function returns a failure code, no event is posted. The runtime must post an {@link XrEventDataSpaceQueryResultsAvailableFB} before {@link XrEventDataSpaceQueryCompleteFB} if any results are found. Once an {@link XrEventDataSpaceQueryResultsAvailableFB} event has been posted, the application may call {@link #xrRetrieveSpaceQueryResultsFB RetrieveSpaceQueryResultsFB} to retrieve the available results.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBSpatialEntityQuery XR_FB_spatial_entity_query} extension must be enabled prior to calling {@link #xrQuerySpacesFB QuerySpacesFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrSpaceQueryInfoBaseHeaderFB}-based structure. See also: {@link XrSpaceQueryInfoFB}
* - {@code requestId} must be a pointer to an {@code XrAsyncRequestIdFB} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrSpaceQueryInfoBaseHeaderFB}
*
* @param session a handle to an {@code XrSession}.
* @param info a pointer to the {@link XrSpaceQueryInfoBaseHeaderFB} structure.
* @param requestId an output parameter, and the variable it points to will be populated with the ID of this asynchronous request.
*/
@NativeType("XrResult")
public static int xrQuerySpacesFB(XrSession session, @NativeType("XrSpaceQueryInfoBaseHeaderFB const *") XrSpaceQueryInfoBaseHeaderFB info, @NativeType("XrAsyncRequestIdFB *") LongBuffer requestId) {
if (CHECKS) {
check(requestId, 1);
}
return nxrQuerySpacesFB(session, info.address(), memAddress(requestId));
}
// --- [ xrRetrieveSpaceQueryResultsFB ] ---
/** Unsafe version of: {@link #xrRetrieveSpaceQueryResultsFB RetrieveSpaceQueryResultsFB} */
public static int nxrRetrieveSpaceQueryResultsFB(XrSession session, long requestId, long results) {
long __functionAddress = session.getCapabilities().xrRetrieveSpaceQueryResultsFB;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(session.address(), requestId, results, __functionAddress);
}
/**
* Copies query results into an output buffer.
*
* C Specification
*
* The {@link #xrRetrieveSpaceQueryResultsFB RetrieveSpaceQueryResultsFB} function is defined as:
*
*
* XrResult xrRetrieveSpaceQueryResultsFB(
* XrSession session,
* XrAsyncRequestIdFB requestId,
* XrSpaceQueryResultsFB* results);
*
* Description
*
* Allows an application to retrieve all available results for a specified query. Call this function once to get the number of results found and then once more to copy the results into a buffer provided by the application. The number of results will not change between the two calls used to retrieve results. This function must only retrieve each query result once. After the application has used this function to retrieve a query result, the runtime frees its copy. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if {@code requestId} refers to a request that is not yet complete, a request for which results have already been retrieved, or if {@code requestId} does not refer to a known request.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBSpatialEntityQuery XR_FB_spatial_entity_query} extension must be enabled prior to calling {@link #xrRetrieveSpaceQueryResultsFB RetrieveSpaceQueryResultsFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code results} must be a pointer to an {@link XrSpaceQueryResultsFB} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrSpaceQueryResultsFB}
*
* @param session the {@code XrSession} for which the in-progress query is valid.
* @param requestId the {@code XrAsyncRequestIdFB} to enumerate results for.
* @param results a pointer to an {@link XrSpaceQueryResultsFB} to populate with results.
*/
@NativeType("XrResult")
public static int xrRetrieveSpaceQueryResultsFB(XrSession session, @NativeType("XrAsyncRequestIdFB") long requestId, @NativeType("XrSpaceQueryResultsFB *") XrSpaceQueryResultsFB results) {
return nxrRetrieveSpaceQueryResultsFB(session, requestId, results.address());
}
}