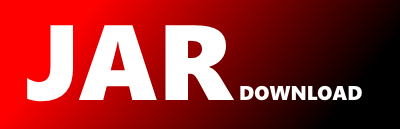
org.lwjgl.openxr.FBSpatialEntityStorage Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_FB_spatial_entity_storage extension.
*
* This extension enables spatial entities to be stored and persisted across sessions. If the {@link FBSpatialEntity#XR_SPACE_COMPONENT_TYPE_STORABLE_FB SPACE_COMPONENT_TYPE_STORABLE_FB} component has been enabled on the spatial entity, application developers may save, load, and erase persisted {@code XrSpace} entities.
*
* In order to enable the functionality of this extension, you must pass the name of the extension into {@link XR10#xrCreateInstance CreateInstance} via the {@link XrInstanceCreateInfo}{@code ::enabledExtensionNames} parameter as indicated in the extension section.
*/
public class FBSpatialEntityStorage {
/** The extension specification version. */
public static final int XR_FB_spatial_entity_storage_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_FB_SPATIAL_ENTITY_STORAGE_EXTENSION_NAME = "XR_FB_spatial_entity_storage";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SPACE_SAVE_INFO_FB TYPE_SPACE_SAVE_INFO_FB}
* - {@link #XR_TYPE_SPACE_ERASE_INFO_FB TYPE_SPACE_ERASE_INFO_FB}
* - {@link #XR_TYPE_EVENT_DATA_SPACE_SAVE_COMPLETE_FB TYPE_EVENT_DATA_SPACE_SAVE_COMPLETE_FB}
* - {@link #XR_TYPE_EVENT_DATA_SPACE_ERASE_COMPLETE_FB TYPE_EVENT_DATA_SPACE_ERASE_COMPLETE_FB}
*
*/
public static final int
XR_TYPE_SPACE_SAVE_INFO_FB = 1000158000,
XR_TYPE_SPACE_ERASE_INFO_FB = 1000158001,
XR_TYPE_EVENT_DATA_SPACE_SAVE_COMPLETE_FB = 1000158106,
XR_TYPE_EVENT_DATA_SPACE_ERASE_COMPLETE_FB = 1000158107;
/**
* XrSpacePersistenceModeFB - Persistence mode
*
* Description
*
* The {@code XrSpacePersistenceModeFB} enumeration specifies the persistence mode for the save operation.
*
* Enumerant Descriptions
*
*
* - {@link #XR_SPACE_PERSISTENCE_MODE_INVALID_FB SPACE_PERSISTENCE_MODE_INVALID_FB} — Invalid storage persistence
* - {@link #XR_SPACE_PERSISTENCE_MODE_INDEFINITE_FB SPACE_PERSISTENCE_MODE_INDEFINITE_FB} — Store {@code XrSpace} indefinitely, or until erased
*
*
* See Also
*
* {@link XrSpaceSaveInfoFB}
*/
public static final int
XR_SPACE_PERSISTENCE_MODE_INVALID_FB = 0,
XR_SPACE_PERSISTENCE_MODE_INDEFINITE_FB = 1;
protected FBSpatialEntityStorage() {
throw new UnsupportedOperationException();
}
// --- [ xrSaveSpaceFB ] ---
/** Unsafe version of: {@link #xrSaveSpaceFB SaveSpaceFB} */
public static int nxrSaveSpaceFB(XrSession session, long info, long requestId) {
long __functionAddress = session.getCapabilities().xrSaveSpaceFB;
if (CHECKS) {
check(__functionAddress);
XrSpaceSaveInfoFB.validate(info);
}
return callPPPI(session.address(), info, requestId, __functionAddress);
}
/**
* Saves a spatial entity to persistent storage.
*
* C Specification
*
* The {@link #xrSaveSpaceFB SaveSpaceFB} function is defined as:
*
*
* XrResult xrSaveSpaceFB(
* XrSession session,
* const XrSpaceSaveInfoFB* info,
* XrAsyncRequestIdFB* requestId);
*
* Description
*
* The {@link #xrSaveSpaceFB SaveSpaceFB} function persists the spatial entity at the specified location with the specified mode. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if {@link XrSpaceSaveInfoFB}{@code ::space} is {@link XR10#XR_NULL_HANDLE NULL_HANDLE} or otherwise invalid. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if {@link XrSpaceSaveInfoFB}{@code ::location} or {@link XrSpaceSaveInfoFB}{@code ::persistenceMode} is invalid. This operation is asynchronous and the runtime must post an {@link XrEventDataSpaceSaveCompleteFB} event when the operation completes successfully or encounters an error. If this function returns a failure code, no event is posted.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBSpatialEntityStorage XR_FB_spatial_entity_storage} extension must be enabled prior to calling {@link #xrSaveSpaceFB SaveSpaceFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrSpaceSaveInfoFB} structure
* - {@code requestId} must be a pointer to an {@code XrAsyncRequestIdFB} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link FBSpatialEntity#XR_ERROR_SPACE_COMPONENT_NOT_ENABLED_FB ERROR_SPACE_COMPONENT_NOT_ENABLED_FB}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrSpaceSaveInfoFB}, {@link #xrEraseSpaceFB EraseSpaceFB}
*
* @param session a handle to an {@code XrSession}.
* @param info contains the parameters for the save operation.
* @param requestId an output parameter, and the variable it points to will be populated with the ID of this asynchronous request.
*/
@NativeType("XrResult")
public static int xrSaveSpaceFB(XrSession session, @NativeType("XrSpaceSaveInfoFB const *") XrSpaceSaveInfoFB info, @NativeType("XrAsyncRequestIdFB *") LongBuffer requestId) {
if (CHECKS) {
check(requestId, 1);
}
return nxrSaveSpaceFB(session, info.address(), memAddress(requestId));
}
// --- [ xrEraseSpaceFB ] ---
/** Unsafe version of: {@link #xrEraseSpaceFB EraseSpaceFB} */
public static int nxrEraseSpaceFB(XrSession session, long info, long requestId) {
long __functionAddress = session.getCapabilities().xrEraseSpaceFB;
if (CHECKS) {
check(__functionAddress);
XrSpaceEraseInfoFB.validate(info);
}
return callPPPI(session.address(), info, requestId, __functionAddress);
}
/**
* Erases a spatial entity from persistent storage.
*
* C Specification
*
* The {@link #xrEraseSpaceFB EraseSpaceFB} function is defined as:
*
*
* XrResult xrEraseSpaceFB(
* XrSession session,
* const XrSpaceEraseInfoFB* info,
* XrAsyncRequestIdFB* requestId);
*
* Description
*
* The {@link #xrEraseSpaceFB EraseSpaceFB} function erases a spatial entity from storage at the specified location. The {@code XrSpace} remains valid in the current session until the application destroys it or the session ends. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if {@link XrSpaceEraseInfoFB}{@code ::space} is {@link XR10#XR_NULL_HANDLE NULL_HANDLE} or otherwise invalid. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if {@link XrSpaceEraseInfoFB}{@code ::location} is invalid. This operation is asynchronous and the runtime must post an {@link XrEventDataSpaceEraseCompleteFB} event when the operation completes successfully or encounters an error. If this function returns a failure code, no event is posted.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBSpatialEntityStorage XR_FB_spatial_entity_storage} extension must be enabled prior to calling {@link #xrEraseSpaceFB EraseSpaceFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrSpaceEraseInfoFB} structure
* - {@code requestId} must be a pointer to an {@code XrAsyncRequestIdFB} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link FBSpatialEntity#XR_ERROR_SPACE_COMPONENT_NOT_ENABLED_FB ERROR_SPACE_COMPONENT_NOT_ENABLED_FB}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrSpaceEraseInfoFB}, {@link #xrSaveSpaceFB SaveSpaceFB}
*
* @param session a handle to an {@code XrSession}.
* @param info contains the parameters for the erase operation.
* @param requestId an output parameter, and the variable it points to will be populated with the ID of this asynchronous request.
*/
@NativeType("XrResult")
public static int xrEraseSpaceFB(XrSession session, @NativeType("XrSpaceEraseInfoFB const *") XrSpaceEraseInfoFB info, @NativeType("XrAsyncRequestIdFB *") LongBuffer requestId) {
if (CHECKS) {
check(requestId, 1);
}
return nxrEraseSpaceFB(session, info.address(), memAddress(requestId));
}
}