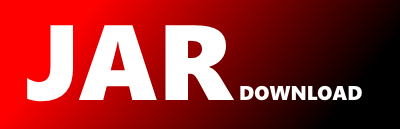
org.lwjgl.openxr.FBSpatialEntityUser Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_FB_spatial_entity_user extension.
*
* This extension enables creation and management of user objects which can be used by the application to reference a user other than the current user.
*
* In order to enable the functionality of this extension, you must pass the name of the extension into {@link XR10#xrCreateInstance CreateInstance} via the {@link XrInstanceCreateInfo}{@code ::enabledExtensionNames} parameter as indicated in the extension section.
*/
public class FBSpatialEntityUser {
/** The extension specification version. */
public static final int XR_FB_spatial_entity_user_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_FB_SPATIAL_ENTITY_USER_EXTENSION_NAME = "XR_FB_spatial_entity_user";
/** Extends {@code XrStructureType}. */
public static final int XR_TYPE_SPACE_USER_CREATE_INFO_FB = 1000241001;
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_SPACE_USER_FB = 1000241000;
protected FBSpatialEntityUser() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateSpaceUserFB ] ---
/** Unsafe version of: {@link #xrCreateSpaceUserFB CreateSpaceUserFB} */
public static int nxrCreateSpaceUserFB(XrSession session, long info, long user) {
long __functionAddress = session.getCapabilities().xrCreateSpaceUserFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), info, user, __functionAddress);
}
/**
* Creates a user other than the current user.
*
* C Specification
*
* The {@link #xrCreateSpaceUserFB CreateSpaceUserFB} function is defined as:
*
*
* XrResult xrCreateSpaceUserFB(
* XrSession session,
* const XrSpaceUserCreateInfoFB* info,
* XrSpaceUserFB* user);
*
* Description
*
* The application can use this function to create a user handle with which it can then interact, such as sharing {@code XrSpace} objects.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBSpatialEntityUser XR_FB_spatial_entity_user} extension must be enabled prior to calling {@link #xrCreateSpaceUserFB CreateSpaceUserFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrSpaceUserCreateInfoFB} structure
* - {@code user} must be a pointer to an {@code XrSpaceUserFB} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* See Also
*
* {@link XrSpaceUserCreateInfoFB}
*
* @param session a handle to an {@code XrSession}.
* @param info a pointer to an {@link XrSpaceUserCreateInfoFB} structure containing information to create the user handle.
* @param user the output parameter that points to the handle of the user being created.
*/
@NativeType("XrResult")
public static int xrCreateSpaceUserFB(XrSession session, @NativeType("XrSpaceUserCreateInfoFB const *") XrSpaceUserCreateInfoFB info, @NativeType("XrSpaceUserFB *") PointerBuffer user) {
if (CHECKS) {
check(user, 1);
}
return nxrCreateSpaceUserFB(session, info.address(), memAddress(user));
}
// --- [ xrGetSpaceUserIdFB ] ---
/** Unsafe version of: {@link #xrGetSpaceUserIdFB GetSpaceUserIdFB} */
public static int nxrGetSpaceUserIdFB(XrSpaceUserFB user, long userId) {
long __functionAddress = user.getCapabilities().xrGetSpaceUserIdFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(user.address(), userId, __functionAddress);
}
/**
* Gets the user ID for a given user handle.
*
* C Specification
*
* The {@link #xrGetSpaceUserIdFB GetSpaceUserIdFB} function is defined as:
*
*
* XrResult xrGetSpaceUserIdFB(
* XrSpaceUserFB user,
* XrSpaceUserIdFB* userId);
*
* Description
*
* The application can use this function to retrieve the user ID of a given user handle.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBSpatialEntityUser XR_FB_spatial_entity_user} extension must be enabled prior to calling {@link #xrGetSpaceUserIdFB GetSpaceUserIdFB}
* - {@code user} must be a valid {@code XrSpaceUserFB} handle
* - {@code userId} must be a pointer to an {@code XrSpaceUserIdFB} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* @param user a handle to an {@code XrSpaceUserFB}.
* @param userId the output parameter that points to the user ID of the user.
*/
@NativeType("XrResult")
public static int xrGetSpaceUserIdFB(XrSpaceUserFB user, @NativeType("XrSpaceUserIdFB *") LongBuffer userId) {
if (CHECKS) {
check(userId, 1);
}
return nxrGetSpaceUserIdFB(user, memAddress(userId));
}
// --- [ xrDestroySpaceUserFB ] ---
/**
* Destroys a user handle.
*
* C Specification
*
* The {@link #xrDestroySpaceUserFB DestroySpaceUserFB} function is defined as:
*
*
* XrResult xrDestroySpaceUserFB(
* XrSpaceUserFB user);
*
* Description
*
* The application should use this function to release resources tied to a given {@code XrSpaceUserFB} once the application no longer needs to reference the user.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBSpatialEntityUser XR_FB_spatial_entity_user} extension must be enabled prior to calling {@link #xrDestroySpaceUserFB DestroySpaceUserFB}
* - {@code user} must be a valid {@code XrSpaceUserFB} handle
*
*
* Thread Safety
*
*
* - Access to {@code user}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* @param user a handle to the user object to be destroyed.
*/
@NativeType("XrResult")
public static int xrDestroySpaceUserFB(XrSpaceUserFB user) {
long __functionAddress = user.getCapabilities().xrDestroySpaceUserFB;
if (CHECKS) {
check(__functionAddress);
}
return callPI(user.address(), __functionAddress);
}
}