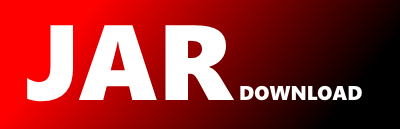
org.lwjgl.openxr.FBTriangleMesh Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_FB_triangle_mesh extension.
*
* Meshes may be useful in XR applications when representing parts of the environment. In particular, application may provide the surfaces of real-world objects tagged manually to the runtime, or obtain automatically detected environment contents.
*
* This extension allows:
*
*
* - An application to create a triangle mesh and specify the mesh data.
* - An application to update mesh contents if a mesh is mutable.
*
*
* In order to enable the functionality of this extension, the application must pass the name of the extension into {@link XR10#xrCreateInstance CreateInstance} via the {@link XrInstanceCreateInfo}{@code ::enabledExtensionNames} parameter as indicated in the extension section.
*/
public class FBTriangleMesh {
/** The extension specification version. */
public static final int XR_FB_triangle_mesh_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_FB_TRIANGLE_MESH_EXTENSION_NAME = "XR_FB_triangle_mesh";
/** Extends {@code XrStructureType}. */
public static final int XR_TYPE_TRIANGLE_MESH_CREATE_INFO_FB = 1000117001;
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_TRIANGLE_MESH_FB = 1000117000;
/**
* XrTriangleMeshFlagBitsFB - XrTriangleMeshFlagBitsFB
*
* Flag Descriptions
*
*
* - {@link #XR_TRIANGLE_MESH_MUTABLE_BIT_FB TRIANGLE_MESH_MUTABLE_BIT_FB} — The triangle mesh is mutable (can be modified after it is created).
*
*/
public static final int XR_TRIANGLE_MESH_MUTABLE_BIT_FB = 0x1;
/**
* XrWindingOrderFB - Triangle winding order
*
* Enumerant Descriptions
*
*
* - {@link #XR_WINDING_ORDER_UNKNOWN_FB WINDING_ORDER_UNKNOWN_FB} — Winding order is unknown and the runtime cannot make any assumptions on the triangle orientation
* - {@link #XR_WINDING_ORDER_CW_FB WINDING_ORDER_CW_FB} — Clockwise winding order
* - {@link #XR_WINDING_ORDER_CCW_FB WINDING_ORDER_CCW_FB} — Counter-clockwise winding order
*
*
* See Also
*
* {@link XrTriangleMeshCreateInfoFB}
*/
public static final int
XR_WINDING_ORDER_UNKNOWN_FB = 0,
XR_WINDING_ORDER_CW_FB = 1,
XR_WINDING_ORDER_CCW_FB = 2;
protected FBTriangleMesh() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateTriangleMeshFB ] ---
/** Unsafe version of: {@link #xrCreateTriangleMeshFB CreateTriangleMeshFB} */
public static int nxrCreateTriangleMeshFB(XrSession session, long createInfo, long outTriangleMesh) {
long __functionAddress = session.getCapabilities().xrCreateTriangleMeshFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, outTriangleMesh, __functionAddress);
}
/**
* Create a triangle mesh.
*
* C Specification
*
* The {@link #xrCreateTriangleMeshFB CreateTriangleMeshFB} function is defined as:
*
*
* XrResult xrCreateTriangleMeshFB(
* XrSession session,
* const XrTriangleMeshCreateInfoFB* createInfo,
* XrTriangleMeshFB* outTriangleMesh);
*
* Description
*
* This creates an {@code XrTriangleMeshFB} handle. The returned triangle mesh handle may be subsequently used in API calls.
*
* When the mesh is mutable (the {@link #XR_TRIANGLE_MESH_MUTABLE_BIT_FB TRIANGLE_MESH_MUTABLE_BIT_FB} bit is set in {@link XrTriangleMeshCreateInfoFB}{@code ::flags}), the created triangle mesh starts in the Undefined Topology state.
*
* Immutable meshes have no state machine; they may be considered to be in state Ready with no valid edges leaving that state.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBTriangleMesh XR_FB_triangle_mesh} extension must be enabled prior to calling {@link #xrCreateTriangleMeshFB CreateTriangleMeshFB}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrTriangleMeshCreateInfoFB} structure
* - {@code outTriangleMesh} must be a pointer to an {@code XrTriangleMeshFB} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link FBPassthrough#XR_ERROR_INSUFFICIENT_RESOURCES_PASSTHROUGH_FB ERROR_INSUFFICIENT_RESOURCES_PASSTHROUGH_FB}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrTriangleMeshCreateInfoFB}
*
* @param session the {@code XrSession} to which the mesh will belong.
* @param createInfo a pointer to an {@link XrTriangleMeshCreateInfoFB} structure containing parameters to be used to create the mesh.
* @param outTriangleMesh a pointer to a handle in which the created {@code XrTriangleMeshFB} is returned.
*/
@NativeType("XrResult")
public static int xrCreateTriangleMeshFB(XrSession session, @NativeType("XrTriangleMeshCreateInfoFB const *") XrTriangleMeshCreateInfoFB createInfo, @NativeType("XrTriangleMeshFB *") PointerBuffer outTriangleMesh) {
if (CHECKS) {
check(outTriangleMesh, 1);
}
return nxrCreateTriangleMeshFB(session, createInfo.address(), memAddress(outTriangleMesh));
}
// --- [ xrDestroyTriangleMeshFB ] ---
/**
* Destroy a triangle mesh.
*
* C Specification
*
* The {@link #xrDestroyTriangleMeshFB DestroyTriangleMeshFB} function is defined as:
*
*
* XrResult xrDestroyTriangleMeshFB(
* XrTriangleMeshFB mesh);
*
* Description
*
* {@code XrTriangleMeshFB} handles and their associated data are destroyed by {@link #xrDestroyTriangleMeshFB DestroyTriangleMeshFB}. The mesh buffers retrieved by {@link #xrTriangleMeshGetVertexBufferFB TriangleMeshGetVertexBufferFB} and {@link #xrTriangleMeshGetIndexBufferFB TriangleMeshGetIndexBufferFB} must not be accessed anymore after their parent mesh object has been destroyed.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBTriangleMesh XR_FB_triangle_mesh} extension must be enabled prior to calling {@link #xrDestroyTriangleMeshFB DestroyTriangleMeshFB}
* - {@code mesh} must be a valid {@code XrTriangleMeshFB} handle
*
*
* Thread Safety
*
*
* - Access to {@code mesh}, and any child handles, must be externally synchronized
* - Access to the buffers returned from calls to {@link #xrTriangleMeshGetVertexBufferFB TriangleMeshGetVertexBufferFB} and {@link #xrTriangleMeshGetIndexBufferFB TriangleMeshGetIndexBufferFB} on {@code mesh} must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* @param mesh the {@code XrTriangleMeshFB} to destroy.
*/
@NativeType("XrResult")
public static int xrDestroyTriangleMeshFB(XrTriangleMeshFB mesh) {
long __functionAddress = mesh.getCapabilities().xrDestroyTriangleMeshFB;
if (CHECKS) {
check(__functionAddress);
}
return callPI(mesh.address(), __functionAddress);
}
// --- [ xrTriangleMeshGetVertexBufferFB ] ---
/** Unsafe version of: {@link #xrTriangleMeshGetVertexBufferFB TriangleMeshGetVertexBufferFB} */
public static int nxrTriangleMeshGetVertexBufferFB(XrTriangleMeshFB mesh, long outVertexBuffer) {
long __functionAddress = mesh.getCapabilities().xrTriangleMeshGetVertexBufferFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(mesh.address(), outVertexBuffer, __functionAddress);
}
/**
* Obtain the vertex buffer of a triangle mesh.
*
* C Specification
*
* The {@link #xrTriangleMeshGetVertexBufferFB TriangleMeshGetVertexBufferFB} function is defined as:
*
*
* XrResult xrTriangleMeshGetVertexBufferFB(
* XrTriangleMeshFB mesh,
* XrVector3f** outVertexBuffer);
*
* Description
*
* Retrieves a pointer to the vertex buffer. The vertex buffer is structured as an array of {@link XrVector3f}. The size of the buffer is {@link XrTriangleMeshCreateInfoFB}{@code ::vertexCount} elements. The buffer location is guaranteed to remain constant over the lifecycle of the mesh object.
*
* A mesh must be mutable and in a specific state for the application to modify it through the retrieved vertex buffer.
*
*
* - A mutable triangle mesh must be in state Defining Topology, Updating Mesh, or Updating Vertices to modify the contents of the vertex buffer retrieved by this function.
* - A mutable triangle mesh must be in state Defining Topology or Updating Mesh to modify the count of elements in the vertex buffer retrieved by this function. The new count is passed as a parameter to {@link #xrTriangleMeshEndUpdateFB TriangleMeshEndUpdateFB}.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link FBTriangleMesh XR_FB_triangle_mesh} extension must be enabled prior to calling {@link #xrTriangleMeshGetVertexBufferFB TriangleMeshGetVertexBufferFB}
* - {@code mesh} must be a valid {@code XrTriangleMeshFB} handle
* - {@code outVertexBuffer} must be a pointer to a pointer to an {@link XrVector3f} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrVector3f}, {@link #xrTriangleMeshGetIndexBufferFB TriangleMeshGetIndexBufferFB}
*
* @param mesh the {@code XrTriangleMeshFB} to get the vertex buffer for.
* @param outVertexBuffer a pointer to return the vertex buffer into.
*/
@NativeType("XrResult")
public static int xrTriangleMeshGetVertexBufferFB(XrTriangleMeshFB mesh, @NativeType("XrVector3f **") PointerBuffer outVertexBuffer) {
if (CHECKS) {
check(outVertexBuffer, 1);
}
return nxrTriangleMeshGetVertexBufferFB(mesh, memAddress(outVertexBuffer));
}
// --- [ xrTriangleMeshGetIndexBufferFB ] ---
/** Unsafe version of: {@link #xrTriangleMeshGetIndexBufferFB TriangleMeshGetIndexBufferFB} */
public static int nxrTriangleMeshGetIndexBufferFB(XrTriangleMeshFB mesh, long outIndexBuffer) {
long __functionAddress = mesh.getCapabilities().xrTriangleMeshGetIndexBufferFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(mesh.address(), outIndexBuffer, __functionAddress);
}
/**
* Obtain the index buffer of a triangle mesh.
*
* C Specification
*
* The {@link #xrTriangleMeshGetIndexBufferFB TriangleMeshGetIndexBufferFB} function is defined as:
*
*
* XrResult xrTriangleMeshGetIndexBufferFB(
* XrTriangleMeshFB mesh,
* uint32_t** outIndexBuffer);
*
* Description
*
* Retrieves a pointer to the index buffer that defines the topology of the triangle mesh. Each triplet of consecutive elements points to three vertices in the vertex buffer and thus form a triangle. The size of the index buffer is 3 * {@link XrTriangleMeshCreateInfoFB}::triangleCount
elements. The buffer location is guaranteed to remain constant over the lifecycle of the mesh object.
*
* A triangle mesh must be mutable and in state Defining Topology or Updating Mesh for the application to modify the contents and/or triangle count in the index buffer retrieved by this function.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBTriangleMesh XR_FB_triangle_mesh} extension must be enabled prior to calling {@link #xrTriangleMeshGetIndexBufferFB TriangleMeshGetIndexBufferFB}
* - {@code mesh} must be a valid {@code XrTriangleMeshFB} handle
* - {@code outIndexBuffer} must be a pointer to a pointer to a {@code uint32_t} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link #xrTriangleMeshGetVertexBufferFB TriangleMeshGetVertexBufferFB}
*
* @param mesh the {@code XrTriangleMeshFB} to get the index buffer for.
* @param outIndexBuffer a pointer to return the index buffer into.
*/
@NativeType("XrResult")
public static int xrTriangleMeshGetIndexBufferFB(XrTriangleMeshFB mesh, @NativeType("uint32_t **") PointerBuffer outIndexBuffer) {
if (CHECKS) {
check(outIndexBuffer, 1);
}
return nxrTriangleMeshGetIndexBufferFB(mesh, memAddress(outIndexBuffer));
}
// --- [ xrTriangleMeshBeginUpdateFB ] ---
/**
* Begin an update of the mesh data.
*
* C Specification
*
* The {@link #xrTriangleMeshBeginUpdateFB TriangleMeshBeginUpdateFB} function is defined as:
*
*
* XrResult xrTriangleMeshBeginUpdateFB(
* XrTriangleMeshFB mesh);
*
* Description
*
* Begins updating the mesh buffer data. The application must call this function before it makes any modifications to the buffers retrieved by {@link #xrTriangleMeshGetVertexBufferFB TriangleMeshGetVertexBufferFB} and {@link #xrTriangleMeshGetIndexBufferFB TriangleMeshGetIndexBufferFB}. If only the vertex buffer contents need to be updated, and the mesh is in state Ready, {@link #xrTriangleMeshBeginVertexBufferUpdateFB TriangleMeshBeginVertexBufferUpdateFB} may be used instead. To commit the modifications, the application must call {@link #xrTriangleMeshEndUpdateFB TriangleMeshEndUpdateFB}.
*
* The triangle mesh {@code mesh} must be mutable. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if the mesh is immutable.
*
* The triangle mesh {@code mesh} must be in state Undefined Topology or Ready.
*
*
* - If the triangle mesh is in state Undefined Topology before this call, a successful call moves it to state Defining Topology.
* - If the triangle mesh is in state Ready before this call, a successful call moves it to state Updating Mesh.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link FBTriangleMesh XR_FB_triangle_mesh} extension must be enabled prior to calling {@link #xrTriangleMeshBeginUpdateFB TriangleMeshBeginUpdateFB}
* - {@code mesh} must be a valid {@code XrTriangleMeshFB} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID}
*
*
*
* See Also
*
* {@link #xrTriangleMeshEndUpdateFB TriangleMeshEndUpdateFB}
*
* @param mesh the {@code XrTriangleMeshFB} to update.
*/
@NativeType("XrResult")
public static int xrTriangleMeshBeginUpdateFB(XrTriangleMeshFB mesh) {
long __functionAddress = mesh.getCapabilities().xrTriangleMeshBeginUpdateFB;
if (CHECKS) {
check(__functionAddress);
}
return callPI(mesh.address(), __functionAddress);
}
// --- [ xrTriangleMeshEndUpdateFB ] ---
/**
* End an update of the mesh data.
*
* C Specification
*
* The {@link #xrTriangleMeshEndUpdateFB TriangleMeshEndUpdateFB} function is defined as:
*
*
* XrResult xrTriangleMeshEndUpdateFB(
* XrTriangleMeshFB mesh,
* uint32_t vertexCount,
* uint32_t triangleCount);
*
* Description
*
* Signals to the runtime that the application has finished initially populating or updating the mesh buffers. {@code vertexCount} and {@code triangleCount} specify the actual number of primitives that make up the mesh after the update. They must be larger than zero but smaller or equal to the maximum counts defined at create time. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if an invalid count is passed.
*
* The triangle mesh {@code mesh} must be mutable. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if the mesh is immutable.
*
* The triangle mesh {@code mesh} must be in state Defining Topology or Updating Mesh.
*
* A successful call moves {@code mesh} to state Ready.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBTriangleMesh XR_FB_triangle_mesh} extension must be enabled prior to calling {@link #xrTriangleMeshEndUpdateFB TriangleMeshEndUpdateFB}
* - {@code mesh} must be a valid {@code XrTriangleMeshFB} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID}
*
*
*
* See Also
*
* {@link #xrTriangleMeshBeginUpdateFB TriangleMeshBeginUpdateFB}
*
* @param mesh the {@code XrTriangleMeshFB} to update.
* @param vertexCount the vertex count after the update.
* @param triangleCount the triangle count after the update.
*/
@NativeType("XrResult")
public static int xrTriangleMeshEndUpdateFB(XrTriangleMeshFB mesh, @NativeType("uint32_t") int vertexCount, @NativeType("uint32_t") int triangleCount) {
long __functionAddress = mesh.getCapabilities().xrTriangleMeshEndUpdateFB;
if (CHECKS) {
check(__functionAddress);
}
return callPI(mesh.address(), vertexCount, triangleCount, __functionAddress);
}
// --- [ xrTriangleMeshBeginVertexBufferUpdateFB ] ---
/** Unsafe version of: {@link #xrTriangleMeshBeginVertexBufferUpdateFB TriangleMeshBeginVertexBufferUpdateFB} */
public static int nxrTriangleMeshBeginVertexBufferUpdateFB(XrTriangleMeshFB mesh, long outVertexCount) {
long __functionAddress = mesh.getCapabilities().xrTriangleMeshBeginVertexBufferUpdateFB;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(mesh.address(), outVertexCount, __functionAddress);
}
/**
* Begin an update of the vertex data.
*
* C Specification
*
* The {@link #xrTriangleMeshBeginVertexBufferUpdateFB TriangleMeshBeginVertexBufferUpdateFB} function is defined as:
*
*
* XrResult xrTriangleMeshBeginVertexBufferUpdateFB(
* XrTriangleMeshFB mesh,
* uint32_t* outVertexCount);
*
* Description
*
* Begins an update of the vertex positions of a mutable triangle mesh. The vertex count returned through {@code outVertexCount} is defined by the last call to {@link #xrTriangleMeshEndUpdateFB TriangleMeshEndUpdateFB}. Once the modification is done, call {@link #xrTriangleMeshEndVertexBufferUpdateFB TriangleMeshEndVertexBufferUpdateFB} to commit the changes and move to state Ready.
*
* The triangle mesh {@code mesh} must be mutable. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if the mesh is immutable.
*
* The triangle mesh {@code mesh} must be in state Ready.
*
* A successful call moves {@code mesh} to state Updating Vertices.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBTriangleMesh XR_FB_triangle_mesh} extension must be enabled prior to calling {@link #xrTriangleMeshBeginVertexBufferUpdateFB TriangleMeshBeginVertexBufferUpdateFB}
* - {@code mesh} must be a valid {@code XrTriangleMeshFB} handle
* - {@code outVertexCount} must be a pointer to a {@code uint32_t} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID}
*
*
*
* See Also
*
* {@link #xrTriangleMeshBeginUpdateFB TriangleMeshBeginUpdateFB}, {@link #xrTriangleMeshEndVertexBufferUpdateFB TriangleMeshEndVertexBufferUpdateFB}
*
* @param mesh the {@code XrTriangleMeshFB} to update.
* @param outVertexCount a pointer to a value to populate with the current vertex count. The updated data must have the exact same number of vertices.
*/
@NativeType("XrResult")
public static int xrTriangleMeshBeginVertexBufferUpdateFB(XrTriangleMeshFB mesh, @NativeType("uint32_t *") IntBuffer outVertexCount) {
if (CHECKS) {
check(outVertexCount, 1);
}
return nxrTriangleMeshBeginVertexBufferUpdateFB(mesh, memAddress(outVertexCount));
}
// --- [ xrTriangleMeshEndVertexBufferUpdateFB ] ---
/**
* End an update of the vertex data.
*
* C Specification
*
* The {@link #xrTriangleMeshEndVertexBufferUpdateFB TriangleMeshEndVertexBufferUpdateFB} function is defined as:
*
*
* XrResult xrTriangleMeshEndVertexBufferUpdateFB(
* XrTriangleMeshFB mesh);
*
* Description
*
* Signals to the runtime that the application has finished updating the vertex buffer data following a call to {@link #xrTriangleMeshBeginVertexBufferUpdateFB TriangleMeshBeginVertexBufferUpdateFB}.
*
* The triangle mesh {@code mesh} must be mutable. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if the mesh is immutable.
*
* The triangle mesh {@code mesh} must be in state Updating Vertices.
*
* A successful call moves {@code mesh} to state Ready.
*
* Valid Usage (Implicit)
*
*
* - The {@link FBTriangleMesh XR_FB_triangle_mesh} extension must be enabled prior to calling {@link #xrTriangleMeshEndVertexBufferUpdateFB TriangleMeshEndVertexBufferUpdateFB}
* - {@code mesh} must be a valid {@code XrTriangleMeshFB} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID}
*
*
*
* See Also
*
* {@link #xrTriangleMeshBeginVertexBufferUpdateFB TriangleMeshBeginVertexBufferUpdateFB}
*
* @param mesh the {@code XrTriangleMeshFB} to update.
*/
@NativeType("XrResult")
public static int xrTriangleMeshEndVertexBufferUpdateFB(XrTriangleMeshFB mesh) {
long __functionAddress = mesh.getCapabilities().xrTriangleMeshEndVertexBufferUpdateFB;
if (CHECKS) {
check(__functionAddress);
}
return callPI(mesh.address(), __functionAddress);
}
}