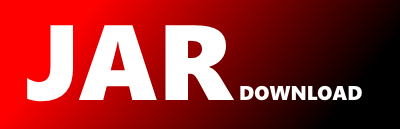
org.lwjgl.openxr.HTCAnchor Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_HTC_anchor extension.
*
* This extension allows an application to create a spatial anchor to track a point in the physical environment. The runtime adjusts the pose of the anchor over time to align it with the real world.
*/
public class HTCAnchor {
/** The extension specification version. */
public static final int XR_HTC_anchor_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_HTC_ANCHOR_EXTENSION_NAME = "XR_HTC_anchor";
/** XR_MAX_SPATIAL_ANCHOR_NAME_SIZE_HTC */
public static final int XR_MAX_SPATIAL_ANCHOR_NAME_SIZE_HTC = 256;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SYSTEM_ANCHOR_PROPERTIES_HTC TYPE_SYSTEM_ANCHOR_PROPERTIES_HTC}
* - {@link #XR_TYPE_SPATIAL_ANCHOR_CREATE_INFO_HTC TYPE_SPATIAL_ANCHOR_CREATE_INFO_HTC}
*
*/
public static final int
XR_TYPE_SYSTEM_ANCHOR_PROPERTIES_HTC = 1000319000,
XR_TYPE_SPATIAL_ANCHOR_CREATE_INFO_HTC = 1000319001;
/** Extends {@code XrResult}. */
public static final int XR_ERROR_NOT_AN_ANCHOR_HTC = -1000319000;
protected HTCAnchor() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateSpatialAnchorHTC ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorHTC CreateSpatialAnchorHTC} */
public static int nxrCreateSpatialAnchorHTC(XrSession session, long createInfo, long anchor) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorHTC;
if (CHECKS) {
check(__functionAddress);
XrSpatialAnchorCreateInfoHTC.validate(createInfo);
}
return callPPPI(session.address(), createInfo, anchor, __functionAddress);
}
/**
* Creates a spatial anchor.
*
* C Specification
*
* The {@link #xrCreateSpatialAnchorHTC CreateSpatialAnchorHTC} function is defined as:
*
*
* XrResult xrCreateSpatialAnchorHTC(
* XrSession session,
* const XrSpatialAnchorCreateInfoHTC* createInfo,
* XrSpace* anchor);
*
* Description
*
* The {@link #xrCreateSpatialAnchorHTC CreateSpatialAnchorHTC} function creates a spatial anchor with specified base space and pose in the space. The anchor is represented by an {@code XrSpace} and its pose can be tracked via {@link XR10#xrLocateSpace LocateSpace}. Once the anchor is no longer needed, call {@link XR10#xrDestroySpace DestroySpace} to erase the anchor.
*
* Valid Usage (Implicit)
*
*
* - The {@link HTCAnchor XR_HTC_anchor} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorHTC CreateSpatialAnchorHTC}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrSpatialAnchorCreateInfoHTC} structure
* - {@code anchor} must be a pointer to an {@code XrSpace} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
* - {@link XR10#XR_ERROR_NAME_INVALID ERROR_NAME_INVALID}
*
*
*
* See Also
*
* {@link XrSpatialAnchorCreateInfoHTC}
*
* @param session the {@code XrSession} to create the anchor in.
* @param createInfo the {@link XrSpatialAnchorCreateInfoHTC} used to specify the anchor.
* @param anchor the returned {@code XrSpace} handle.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorHTC(XrSession session, @NativeType("XrSpatialAnchorCreateInfoHTC const *") XrSpatialAnchorCreateInfoHTC createInfo, @NativeType("XrSpace *") PointerBuffer anchor) {
if (CHECKS) {
check(anchor, 1);
}
return nxrCreateSpatialAnchorHTC(session, createInfo.address(), memAddress(anchor));
}
// --- [ xrGetSpatialAnchorNameHTC ] ---
/** Unsafe version of: {@link #xrGetSpatialAnchorNameHTC GetSpatialAnchorNameHTC} */
public static int nxrGetSpatialAnchorNameHTC(XrSpace anchor, long name) {
long __functionAddress = anchor.getCapabilities().xrGetSpatialAnchorNameHTC;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(anchor.address(), name, __functionAddress);
}
/**
* Get name of a spatial anchor.
*
* C Specification
*
* The {@link #xrGetSpatialAnchorNameHTC GetSpatialAnchorNameHTC} function is defined as:
*
*
* XrResult xrGetSpatialAnchorNameHTC(
* XrSpace anchor,
* XrSpatialAnchorNameHTC* name);
*
* Description
*
* The {@link #xrGetSpatialAnchorNameHTC GetSpatialAnchorNameHTC} function gets the name of an anchor. If the provided {@code anchor} is a valid space handle but was not created with {@link #xrCreateSpatialAnchorHTC CreateSpatialAnchorHTC}, the runtime must return {@link #XR_ERROR_NOT_AN_ANCHOR_HTC ERROR_NOT_AN_ANCHOR_HTC}.
*
* Valid Usage (Implicit)
*
*
* - The {@link HTCAnchor XR_HTC_anchor} extension must be enabled prior to calling {@link #xrGetSpatialAnchorNameHTC GetSpatialAnchorNameHTC}
* - {@code anchor} must be a valid {@code XrSpace} handle
* - {@code name} must be a pointer to an {@link XrSpatialAnchorNameHTC} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link #XR_ERROR_NOT_AN_ANCHOR_HTC ERROR_NOT_AN_ANCHOR_HTC}
*
*
*
* See Also
*
* {@link XrSpatialAnchorNameHTC}
*
* @param anchor the {@code XrSpace} created by {@link #xrCreateSpatialAnchorHTC CreateSpatialAnchorHTC}.
* @param name a pointer to output {@link XrSpatialAnchorNameHTC}.
*/
@NativeType("XrResult")
public static int xrGetSpatialAnchorNameHTC(XrSpace anchor, @NativeType("XrSpatialAnchorNameHTC *") XrSpatialAnchorNameHTC name) {
return nxrGetSpatialAnchorNameHTC(anchor, name.address());
}
}