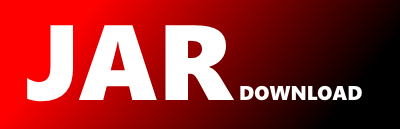
org.lwjgl.openxr.HTCPassthrough Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_HTC_passthrough extension.
*
* This extension enables an application to show the passthrough image to see the surrounding environment from the VR headset. The application is allowed to configure the passthrough image with the different appearances according to the demand of the application.
*
* The passthrough configurations that runtime provides to applications contain:
*
*
* - Decide the passthrough layer shown over or under the frame submitted by the application.
* - Specify the passthrough form with full of the entire screen or projection onto the mesh specified by the application.
* - Set the alpha blending level for the composition of the passthrough layer.
*
*/
public class HTCPassthrough {
/** The extension specification version. */
public static final int XR_HTC_passthrough_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_HTC_PASSTHROUGH_EXTENSION_NAME = "XR_HTC_passthrough";
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_PASSTHROUGH_HTC = 1000317000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_PASSTHROUGH_CREATE_INFO_HTC TYPE_PASSTHROUGH_CREATE_INFO_HTC}
* - {@link #XR_TYPE_PASSTHROUGH_COLOR_HTC TYPE_PASSTHROUGH_COLOR_HTC}
* - {@link #XR_TYPE_PASSTHROUGH_MESH_TRANSFORM_INFO_HTC TYPE_PASSTHROUGH_MESH_TRANSFORM_INFO_HTC}
* - {@link #XR_TYPE_COMPOSITION_LAYER_PASSTHROUGH_HTC TYPE_COMPOSITION_LAYER_PASSTHROUGH_HTC}
*
*/
public static final int
XR_TYPE_PASSTHROUGH_CREATE_INFO_HTC = 1000317001,
XR_TYPE_PASSTHROUGH_COLOR_HTC = 1000317002,
XR_TYPE_PASSTHROUGH_MESH_TRANSFORM_INFO_HTC = 1000317003,
XR_TYPE_COMPOSITION_LAYER_PASSTHROUGH_HTC = 1000317004;
/**
* XrPassthroughFormHTC - Describes the form of passthrough.
*
* Enumerant Descriptions
*
*
* - {@link #XR_PASSTHROUGH_FORM_PLANAR_HTC PASSTHROUGH_FORM_PLANAR_HTC} — Presents the passthrough with full of the entire screen.
* - {@link #XR_PASSTHROUGH_FORM_PROJECTED_HTC PASSTHROUGH_FORM_PROJECTED_HTC} — Presents the passthrough projecting onto a custom mesh.
*
*
* See Also
*
* {@link XrPassthroughCreateInfoHTC}
*/
public static final int
XR_PASSTHROUGH_FORM_PLANAR_HTC = 0,
XR_PASSTHROUGH_FORM_PROJECTED_HTC = 1;
protected HTCPassthrough() {
throw new UnsupportedOperationException();
}
// --- [ xrCreatePassthroughHTC ] ---
/** Unsafe version of: {@link #xrCreatePassthroughHTC CreatePassthroughHTC} */
public static int nxrCreatePassthroughHTC(XrSession session, long createInfo, long passthrough) {
long __functionAddress = session.getCapabilities().xrCreatePassthroughHTC;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, passthrough, __functionAddress);
}
/**
* Create a passthrough handle.
*
* C Specification
*
* The {@link #xrCreatePassthroughHTC CreatePassthroughHTC} function is defined as:
*
*
* XrResult xrCreatePassthroughHTC(
* XrSession session,
* const XrPassthroughCreateInfoHTC* createInfo,
* XrPassthroughHTC* passthrough);
*
* Description
*
* Creates an {@code XrPassthroughHTC} handle.
*
* If the function successfully returned, the output {@code passthrough} must be a valid handle.
*
* Valid Usage (Implicit)
*
*
* - The {@link HTCPassthrough XR_HTC_passthrough} extension must be enabled prior to calling {@link #xrCreatePassthroughHTC CreatePassthroughHTC}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrPassthroughCreateInfoHTC} structure
* - {@code passthrough} must be a pointer to an {@code XrPassthroughHTC} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrPassthroughCreateInfoHTC}
*
* @param session an {@code XrSession} in which the passthrough will be active.
* @param createInfo a pointer to an {@link XrPassthroughCreateInfoHTC} structure containing information about how to create the passthrough.
* @param passthrough a pointer to a handle in which the created {@code XrPassthroughHTC} is returned.
*/
@NativeType("XrResult")
public static int xrCreatePassthroughHTC(XrSession session, @NativeType("XrPassthroughCreateInfoHTC const *") XrPassthroughCreateInfoHTC createInfo, @NativeType("XrPassthroughHTC *") PointerBuffer passthrough) {
if (CHECKS) {
check(passthrough, 1);
}
return nxrCreatePassthroughHTC(session, createInfo.address(), memAddress(passthrough));
}
// --- [ xrDestroyPassthroughHTC ] ---
/**
* Destroy a passthrough handle.
*
* C Specification
*
* The {@link #xrDestroyPassthroughHTC DestroyPassthroughHTC} function is defined as:
*
*
* XrResult xrDestroyPassthroughHTC(
* XrPassthroughHTC passthrough);
*
* Description
*
* The {@link #xrDestroyPassthroughHTC DestroyPassthroughHTC} function releases the passthrough and the underlying resources.
*
* Valid Usage (Implicit)
*
*
* - The {@link HTCPassthrough XR_HTC_passthrough} extension must be enabled prior to calling {@link #xrDestroyPassthroughHTC DestroyPassthroughHTC}
* - {@code passthrough} must be a valid {@code XrPassthroughHTC} handle
*
*
* Thread Safety
*
*
* - Access to {@code passthrough}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* @param passthrough the {@code XrPassthroughHTC} to be destroyed.
*/
@NativeType("XrResult")
public static int xrDestroyPassthroughHTC(XrPassthroughHTC passthrough) {
long __functionAddress = passthrough.getCapabilities().xrDestroyPassthroughHTC;
if (CHECKS) {
check(__functionAddress);
}
return callPI(passthrough.address(), __functionAddress);
}
}