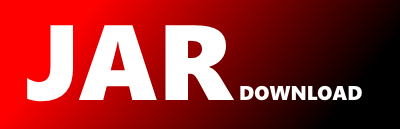
org.lwjgl.openxr.KHRLoaderInit Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The XR_KHR_loader_init extension.
*
* On some platforms, before loading can occur the loader must be initialized with platform-specific parameters.
*
* Unlike other extensions, the presence of this extension is signaled by a successful call to {@link XR10#xrGetInstanceProcAddr GetInstanceProcAddr} to retrieve the function pointer for {@link #xrInitializeLoaderKHR InitializeLoaderKHR} using {@link XR10#XR_NULL_HANDLE NULL_HANDLE} as the {@code instance} parameter.
*
* If this extension is supported, its use may be required on some platforms and the use of the {@link #xrInitializeLoaderKHR InitializeLoaderKHR} function must precede other OpenXR calls except {@link XR10#xrGetInstanceProcAddr GetInstanceProcAddr}.
*
* This function exists as part of the loader library that the application is using and the loader must pass calls to {@link #xrInitializeLoaderKHR InitializeLoaderKHR} to the active runtime, and all enabled API layers that expose a {@link #xrInitializeLoaderKHR InitializeLoaderKHR} function exposed either through their manifest, or through their implementation of {@link XR10#xrGetInstanceProcAddr GetInstanceProcAddr}.
*
* If the {@link #xrInitializeLoaderKHR InitializeLoaderKHR} function is discovered through the manifest, {@link #xrInitializeLoaderKHR InitializeLoaderKHR} will be called before {@code xrNegotiateLoaderRuntimeInterface} or {@code xrNegotiateLoaderApiLayerInterface} has been called on the runtime or layer respectively.
*/
public class KHRLoaderInit {
/** The extension specification version. */
public static final int XR_KHR_loader_init_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_KHR_LOADER_INIT_EXTENSION_NAME = "XR_KHR_loader_init";
protected KHRLoaderInit() {
throw new UnsupportedOperationException();
}
// --- [ xrInitializeLoaderKHR ] ---
/** Unsafe version of: {@link #xrInitializeLoaderKHR InitializeLoaderKHR} */
public static int nxrInitializeLoaderKHR(long loaderInitInfo) {
long __functionAddress = XR.getGlobalCommands().xrInitializeLoaderKHR;
if (CHECKS) {
check(__functionAddress);
}
return callPI(loaderInitInfo, __functionAddress);
}
/**
* Initializes loader.
*
* C Specification
*
* To initialize an OpenXR loader with platform or implementation-specific parameters, call:
*
*
* XrResult xrInitializeLoaderKHR(
* const XrLoaderInitInfoBaseHeaderKHR* loaderInitInfo);
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRLoaderInit XR_KHR_loader_init} extension must be enabled prior to calling {@link #xrInitializeLoaderKHR InitializeLoaderKHR}
* - {@code loaderInitInfo} must be a pointer to a valid {@link XrLoaderInitInfoBaseHeaderKHR}-based structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
*
*
*
* See Also
*
* {@link XrLoaderInitInfoBaseHeaderKHR}
*
* @param loaderInitInfo a pointer to an {@link XrLoaderInitInfoBaseHeaderKHR} structure, which is a polymorphic type defined by other platform- or implementation-specific extensions.
*/
@NativeType("XrResult")
public static int xrInitializeLoaderKHR(@NativeType("XrLoaderInitInfoBaseHeaderKHR const *") XrLoaderInitInfoBaseHeaderKHR loaderInitInfo) {
return nxrInitializeLoaderKHR(loaderInitInfo.address());
}
}