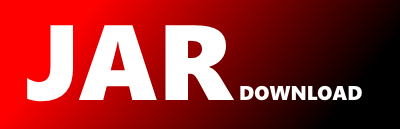
org.lwjgl.openxr.KHROpenGLEnable Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The XR_KHR_opengl_enable extension.
*
* This extension enables the use of the OpenGL graphics API in an OpenXR runtime. Without this extension, the OpenXR runtime may not be able to provide any OpenGL swapchain images.
*
* This extension provides the mechanisms necessary for an application to generate a valid stext:XrGraphicsBindingOpenGL*KHR structure in order to create an OpenGL-based {@code XrSession}. Note that during this process the application is responsible for creating an OpenGL context to be used for rendering. The runtime however will provide the OpenGL textures to render into in the form of a swapchain.
*
* This extension provides mechanisms for the application to interact with images acquired by calling {@link XR10#xrEnumerateSwapchainImages EnumerateSwapchainImages}.
*
* In order to expose the structures, types, and functions of this extension, the application must define {@link XR10#XR_USE_GRAPHICS_API_OPENGL USE_GRAPHICS_API_OPENGL}, as well as an appropriate window system define supported by this extension, before including the OpenXR platform header {@code openxr_platform.h}, in all portions of the library or application that include it. The window system defines currently supported by this extension are:
*
*
* - {@link XR10#XR_USE_PLATFORM_WIN32 USE_PLATFORM_WIN32}
* - {@link XR10#XR_USE_PLATFORM_XLIB USE_PLATFORM_XLIB}
* - {@link XR10#XR_USE_PLATFORM_XCB USE_PLATFORM_XCB}
* - {@link XR10#XR_USE_PLATFORM_WAYLAND USE_PLATFORM_WAYLAND}
*
*
* Note that a runtime implementation of this extension is only required to support the structs introduced by this extension which belong to the platform it is running on.
*
* Note that the OpenGL context given to the call {@link XR10#xrCreateSession CreateSession} must not be bound in another thread when calling the functions: {@link XR10#xrCreateSession CreateSession}, {@link XR10#xrDestroySession DestroySession}, {@link XR10#xrBeginFrame BeginFrame}, {@link XR10#xrEndFrame EndFrame}, {@link XR10#xrCreateSwapchain CreateSwapchain}, {@link XR10#xrDestroySwapchain DestroySwapchain}, {@link XR10#xrEnumerateSwapchainImages EnumerateSwapchainImages}, {@link XR10#xrAcquireSwapchainImage AcquireSwapchainImage}, {@link XR10#xrWaitSwapchainImage WaitSwapchainImage} and {@link XR10#xrReleaseSwapchainImage ReleaseSwapchainImage}. It may be bound in the thread calling those functions. The runtime must not access the context from any other function. In particular the application must be able to call {@link XR10#xrWaitFrame WaitFrame} from a different thread than the rendering thread.
*/
public class KHROpenGLEnable {
/** The extension specification version. */
public static final int XR_KHR_opengl_enable_SPEC_VERSION = 10;
/** The extension name. */
public static final String XR_KHR_OPENGL_ENABLE_EXTENSION_NAME = "XR_KHR_opengl_enable";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_GRAPHICS_BINDING_OPENGL_WIN32_KHR TYPE_GRAPHICS_BINDING_OPENGL_WIN32_KHR}
* - {@link #XR_TYPE_GRAPHICS_BINDING_OPENGL_XLIB_KHR TYPE_GRAPHICS_BINDING_OPENGL_XLIB_KHR}
* - {@link #XR_TYPE_GRAPHICS_BINDING_OPENGL_XCB_KHR TYPE_GRAPHICS_BINDING_OPENGL_XCB_KHR}
* - {@link #XR_TYPE_GRAPHICS_BINDING_OPENGL_WAYLAND_KHR TYPE_GRAPHICS_BINDING_OPENGL_WAYLAND_KHR}
* - {@link #XR_TYPE_SWAPCHAIN_IMAGE_OPENGL_KHR TYPE_SWAPCHAIN_IMAGE_OPENGL_KHR}
* - {@link #XR_TYPE_GRAPHICS_REQUIREMENTS_OPENGL_KHR TYPE_GRAPHICS_REQUIREMENTS_OPENGL_KHR}
*
*/
public static final int
XR_TYPE_GRAPHICS_BINDING_OPENGL_WIN32_KHR = 1000023000,
XR_TYPE_GRAPHICS_BINDING_OPENGL_XLIB_KHR = 1000023001,
XR_TYPE_GRAPHICS_BINDING_OPENGL_XCB_KHR = 1000023002,
XR_TYPE_GRAPHICS_BINDING_OPENGL_WAYLAND_KHR = 1000023003,
XR_TYPE_SWAPCHAIN_IMAGE_OPENGL_KHR = 1000023004,
XR_TYPE_GRAPHICS_REQUIREMENTS_OPENGL_KHR = 1000023005;
protected KHROpenGLEnable() {
throw new UnsupportedOperationException();
}
// --- [ xrGetOpenGLGraphicsRequirementsKHR ] ---
/** Unsafe version of: {@link #xrGetOpenGLGraphicsRequirementsKHR GetOpenGLGraphicsRequirementsKHR} */
public static int nxrGetOpenGLGraphicsRequirementsKHR(XrInstance instance, long systemId, long graphicsRequirements) {
long __functionAddress = instance.getCapabilities().xrGetOpenGLGraphicsRequirementsKHR;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(instance.address(), systemId, graphicsRequirements, __functionAddress);
}
/**
* Retrieve the OpenGL version requirements for an instance and system.
*
* C Specification
*
* To query OpenGL API version requirements for an instance and system, call:
*
*
* XrResult xrGetOpenGLGraphicsRequirementsKHR(
* XrInstance instance,
* XrSystemId systemId,
* XrGraphicsRequirementsOpenGLKHR* graphicsRequirements);
*
* Description
*
* The {@link #xrGetOpenGLGraphicsRequirementsKHR GetOpenGLGraphicsRequirementsKHR} function identifies to the application the minimum OpenGL version requirement and the highest known tested OpenGL version. The runtime must return {@link XR10#XR_ERROR_GRAPHICS_REQUIREMENTS_CALL_MISSING ERROR_GRAPHICS_REQUIREMENTS_CALL_MISSING} ({@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} may be returned due to legacy behavior) on calls to {@link XR10#xrCreateSession CreateSession} if {@link #xrGetOpenGLGraphicsRequirementsKHR GetOpenGLGraphicsRequirementsKHR} has not been called for the same {@code instance} and {@code systemId}.
*
* Valid Usage (Implicit)
*
*
* - The {@link KHROpenGLEnable XR_KHR_opengl_enable} extension must be enabled prior to calling {@link #xrGetOpenGLGraphicsRequirementsKHR GetOpenGLGraphicsRequirementsKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code graphicsRequirements} must be a pointer to an {@link XrGraphicsRequirementsOpenGLKHR} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* See Also
*
* {@link XrGraphicsRequirementsOpenGLKHR}
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param systemId an {@code XrSystemId} handle for the system which will be used to create a session.
* @param graphicsRequirements the {@link XrGraphicsRequirementsOpenGLKHR} output structure.
*/
@NativeType("XrResult")
public static int xrGetOpenGLGraphicsRequirementsKHR(XrInstance instance, @NativeType("XrSystemId") long systemId, @NativeType("XrGraphicsRequirementsOpenGLKHR *") XrGraphicsRequirementsOpenGLKHR graphicsRequirements) {
return nxrGetOpenGLGraphicsRequirementsKHR(instance, systemId, graphicsRequirements.address());
}
}