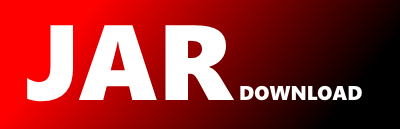
org.lwjgl.openxr.KHRVulkanEnable Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
import org.lwjgl.vulkan.*;
/**
* The XR_KHR_vulkan_enable extension.
*
* This extension enables the use of the Vulkan graphics API in an OpenXR runtime. Without this extension, the OpenXR runtime may not be able to use any Vulkan swapchain images.
*
* This extension provides the mechanisms necessary for an application to generate a valid {@link XrGraphicsBindingVulkanKHR} structure in order to create a Vulkan-based {@code XrSession}. Note that during this process the application is responsible for creating all the required Vulkan objects.
*
* This extension also provides mechanisms for the application to interact with images acquired by calling {@link XR10#xrEnumerateSwapchainImages EnumerateSwapchainImages}.
*
* In order to expose the structures, types, and functions of this extension, you must define {@link XR10#XR_USE_GRAPHICS_API_VULKAN USE_GRAPHICS_API_VULKAN} before including the OpenXR platform header {@code openxr_platform.h}, in all portions of your library or application that include it.
*/
public class KHRVulkanEnable {
/** The extension specification version. */
public static final int XR_KHR_vulkan_enable_SPEC_VERSION = 8;
/** The extension name. */
public static final String XR_KHR_VULKAN_ENABLE_EXTENSION_NAME = "XR_KHR_vulkan_enable";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_GRAPHICS_BINDING_VULKAN_KHR TYPE_GRAPHICS_BINDING_VULKAN_KHR}
* - {@link #XR_TYPE_SWAPCHAIN_IMAGE_VULKAN_KHR TYPE_SWAPCHAIN_IMAGE_VULKAN_KHR}
* - {@link #XR_TYPE_GRAPHICS_REQUIREMENTS_VULKAN_KHR TYPE_GRAPHICS_REQUIREMENTS_VULKAN_KHR}
*
*/
public static final int
XR_TYPE_GRAPHICS_BINDING_VULKAN_KHR = 1000025000,
XR_TYPE_SWAPCHAIN_IMAGE_VULKAN_KHR = 1000025001,
XR_TYPE_GRAPHICS_REQUIREMENTS_VULKAN_KHR = 1000025002;
protected KHRVulkanEnable() {
throw new UnsupportedOperationException();
}
// --- [ xrGetVulkanInstanceExtensionsKHR ] ---
/**
* Unsafe version of: {@link #xrGetVulkanInstanceExtensionsKHR GetVulkanInstanceExtensionsKHR}
*
* @param bufferCapacityInput the capacity of the {@code buffer}, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrGetVulkanInstanceExtensionsKHR(XrInstance instance, long systemId, int bufferCapacityInput, long bufferCountOutput, long buffer) {
long __functionAddress = instance.getCapabilities().xrGetVulkanInstanceExtensionsKHR;
if (CHECKS) {
check(__functionAddress);
}
return callPJPPI(instance.address(), systemId, bufferCapacityInput, bufferCountOutput, buffer, __functionAddress);
}
/**
* Get list of required Vulkan instance extensions for an OpenXR instance and system.
*
* C Specification
*
*
* XrResult xrGetVulkanInstanceExtensionsKHR(
* XrInstance instance,
* XrSystemId systemId,
* uint32_t bufferCapacityInput,
* uint32_t* bufferCountOutput,
* char* buffer);
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRVulkanEnable XR_KHR_vulkan_enable} extension must be enabled prior to calling {@link #xrGetVulkanInstanceExtensionsKHR GetVulkanInstanceExtensionsKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code bufferCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code bufferCapacityInput} is not 0, {@code buffer} must be a pointer to an array of {@code bufferCapacityInput} char values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* See Also
*
* {@link #xrGetVulkanDeviceExtensionsKHR GetVulkanDeviceExtensionsKHR}
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param systemId an {@code XrSystemId} handle for the system which will be used to create a session.
* @param bufferCountOutput a pointer to the count of characters written (including terminating {@code \0}), or a pointer to the required capacity in the case that {@code bufferCapacityInput} is insufficient.
* @param buffer a pointer to an array of characters, but can be {@code NULL} if {@code bufferCapacityInput} is 0. The format of the output is a single space (ASCII {@code 0x20}) delimited string of extension names.
*/
@NativeType("XrResult")
public static int xrGetVulkanInstanceExtensionsKHR(XrInstance instance, @NativeType("XrSystemId") long systemId, @NativeType("uint32_t *") IntBuffer bufferCountOutput, @NativeType("char *") @Nullable ByteBuffer buffer) {
if (CHECKS) {
check(bufferCountOutput, 1);
}
return nxrGetVulkanInstanceExtensionsKHR(instance, systemId, remainingSafe(buffer), memAddress(bufferCountOutput), memAddressSafe(buffer));
}
// --- [ xrGetVulkanDeviceExtensionsKHR ] ---
/**
* Unsafe version of: {@link #xrGetVulkanDeviceExtensionsKHR GetVulkanDeviceExtensionsKHR}
*
* @param bufferCapacityInput the capacity of the {@code buffer}, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrGetVulkanDeviceExtensionsKHR(XrInstance instance, long systemId, int bufferCapacityInput, long bufferCountOutput, long buffer) {
long __functionAddress = instance.getCapabilities().xrGetVulkanDeviceExtensionsKHR;
if (CHECKS) {
check(__functionAddress);
}
return callPJPPI(instance.address(), systemId, bufferCapacityInput, bufferCountOutput, buffer, __functionAddress);
}
/**
* Get list of required Vulkan device extensions for an OpenXR instance and system.
*
* C Specification
*
*
* XrResult xrGetVulkanDeviceExtensionsKHR(
* XrInstance instance,
* XrSystemId systemId,
* uint32_t bufferCapacityInput,
* uint32_t* bufferCountOutput,
* char* buffer);
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRVulkanEnable XR_KHR_vulkan_enable} extension must be enabled prior to calling {@link #xrGetVulkanDeviceExtensionsKHR GetVulkanDeviceExtensionsKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code bufferCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code bufferCapacityInput} is not 0, {@code buffer} must be a pointer to an array of {@code bufferCapacityInput} char values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* See Also
*
* {@link #xrGetVulkanInstanceExtensionsKHR GetVulkanInstanceExtensionsKHR}
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param systemId an {@code XrSystemId} handle for the system which will be used to create a session.
* @param bufferCountOutput a pointer to the count of characters written (including terminating {@code \0}), or a pointer to the required capacity in the case that {@code bufferCapacityInput} is insufficient.
* @param buffer a pointer to an array of characters, but can be {@code NULL} if {@code bufferCapacityInput} is 0. The format of the output is a single space (ASCII {@code 0x20}) delimited string of extension names.
*/
@NativeType("XrResult")
public static int xrGetVulkanDeviceExtensionsKHR(XrInstance instance, @NativeType("XrSystemId") long systemId, @NativeType("uint32_t *") IntBuffer bufferCountOutput, @NativeType("char *") @Nullable ByteBuffer buffer) {
if (CHECKS) {
check(bufferCountOutput, 1);
}
return nxrGetVulkanDeviceExtensionsKHR(instance, systemId, remainingSafe(buffer), memAddress(bufferCountOutput), memAddressSafe(buffer));
}
// --- [ xrGetVulkanGraphicsDeviceKHR ] ---
/** Unsafe version of: {@link #xrGetVulkanGraphicsDeviceKHR GetVulkanGraphicsDeviceKHR} */
public static int nxrGetVulkanGraphicsDeviceKHR(XrInstance instance, long systemId, VkInstance vkInstance, long vkPhysicalDevice) {
long __functionAddress = instance.getCapabilities().xrGetVulkanGraphicsDeviceKHR;
if (CHECKS) {
check(__functionAddress);
}
return callPJPPI(instance.address(), systemId, vkInstance.address(), vkPhysicalDevice, __functionAddress);
}
/**
* Retrieve the Vulkan physical device associated with an OpenXR instance and system.
*
* C Specification
*
* To identify what graphics device needs to be used for an instance and system, call:
*
*
* XrResult xrGetVulkanGraphicsDeviceKHR(
* XrInstance instance,
* XrSystemId systemId,
* VkInstance vkInstance,
* VkPhysicalDevice* vkPhysicalDevice);
*
* Description
*
* {@link #xrGetVulkanGraphicsDeviceKHR GetVulkanGraphicsDeviceKHR} function identifies to the application what graphics device (Vulkan {@code VkPhysicalDevice}) needs to be used. {@link #xrGetVulkanGraphicsDeviceKHR GetVulkanGraphicsDeviceKHR} must be called prior to calling {@link XR10#xrCreateSession CreateSession}, and the {@code VkPhysicalDevice} that {@link #xrGetVulkanGraphicsDeviceKHR GetVulkanGraphicsDeviceKHR} returns should be passed to {@link XR10#xrCreateSession CreateSession} in the {@link XrGraphicsBindingVulkanKHR}.
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRVulkanEnable XR_KHR_vulkan_enable} extension must be enabled prior to calling {@link #xrGetVulkanGraphicsDeviceKHR GetVulkanGraphicsDeviceKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code vkInstance} must be a valid {@code VkInstance} value
* - {@code vkPhysicalDevice} must be a pointer to a {@code VkPhysicalDevice} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param systemId an {@code XrSystemId} handle for the system which will be used to create a session.
* @param vkInstance a valid Vulkan {@code VkInstance}.
* @param vkPhysicalDevice a pointer to a {@code VkPhysicalDevice} value to populate.
*/
@NativeType("XrResult")
public static int xrGetVulkanGraphicsDeviceKHR(XrInstance instance, @NativeType("XrSystemId") long systemId, VkInstance vkInstance, @NativeType("VkPhysicalDevice *") PointerBuffer vkPhysicalDevice) {
if (CHECKS) {
check(vkPhysicalDevice, 1);
}
return nxrGetVulkanGraphicsDeviceKHR(instance, systemId, vkInstance, memAddress(vkPhysicalDevice));
}
// --- [ xrGetVulkanGraphicsRequirementsKHR ] ---
/** Unsafe version of: {@link #xrGetVulkanGraphicsRequirementsKHR GetVulkanGraphicsRequirementsKHR} */
public static int nxrGetVulkanGraphicsRequirementsKHR(XrInstance instance, long systemId, long graphicsRequirements) {
long __functionAddress = instance.getCapabilities().xrGetVulkanGraphicsRequirementsKHR;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(instance.address(), systemId, graphicsRequirements, __functionAddress);
}
/**
* Retrieve the Vulkan version requirements for an instance and system.
*
* C Specification
*
* To query Vulkan API version requirements, call:
*
*
* XrResult xrGetVulkanGraphicsRequirementsKHR(
* XrInstance instance,
* XrSystemId systemId,
* XrGraphicsRequirementsVulkanKHR* graphicsRequirements);
*
* Description
*
* The {@link #xrGetVulkanGraphicsRequirementsKHR GetVulkanGraphicsRequirementsKHR} function identifies to the application the minimum Vulkan version requirement and the highest known tested Vulkan version. The runtime must return {@link XR10#XR_ERROR_GRAPHICS_REQUIREMENTS_CALL_MISSING ERROR_GRAPHICS_REQUIREMENTS_CALL_MISSING} ({@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} may be returned due to legacy behavior) on calls to {@link XR10#xrCreateSession CreateSession} if {@link #xrGetVulkanGraphicsRequirementsKHR GetVulkanGraphicsRequirementsKHR} has not been called for the same {@code instance} and {@code systemId}.
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRVulkanEnable XR_KHR_vulkan_enable} extension must be enabled prior to calling {@link #xrGetVulkanGraphicsRequirementsKHR GetVulkanGraphicsRequirementsKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code graphicsRequirements} must be a pointer to an {@link XrGraphicsRequirementsVulkanKHR} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* See Also
*
* {@link XrGraphicsRequirementsVulkanKHR}
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param systemId an {@code XrSystemId} handle for the system which will be used to create a session.
* @param graphicsRequirements the {@link XrGraphicsRequirementsVulkanKHR} output structure.
*/
@NativeType("XrResult")
public static int xrGetVulkanGraphicsRequirementsKHR(XrInstance instance, @NativeType("XrSystemId") long systemId, @NativeType("XrGraphicsRequirementsVulkanKHR *") XrGraphicsRequirementsVulkanKHR graphicsRequirements) {
return nxrGetVulkanGraphicsRequirementsKHR(instance, systemId, graphicsRequirements.address());
}
}