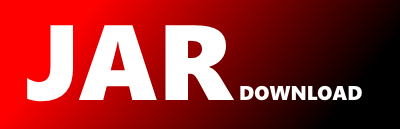
org.lwjgl.openxr.KHRVulkanEnable2 Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
import org.lwjgl.vulkan.*;
/**
* The XR_KHR_vulkan_enable2 extension.
*
* This extension enables the use of the Vulkan graphics API in an OpenXR runtime. Without this extension, the OpenXR runtime may not be able to use any Vulkan swapchain images.
*
* This extension provides the mechanisms necessary for an application to generate a valid {@link XrGraphicsBindingVulkan2KHR} structure in order to create a Vulkan-based {@code XrSession}.
*
* This extension also provides mechanisms for the application to interact with images acquired by calling {@link XR10#xrEnumerateSwapchainImages EnumerateSwapchainImages}.
*
* In order to expose the structures, types, and functions of this extension, you must define {@link XR10#XR_USE_GRAPHICS_API_VULKAN USE_GRAPHICS_API_VULKAN} before including the OpenXR platform header {@code openxr_platform.h}, in all portions of your library or application that include it.
*
* Note
*
* This extension is intended as an alternative to {@link KHRVulkanEnable XR_KHR_vulkan_enable}, and does not depend on it.
*
*/
public class KHRVulkanEnable2 {
/** The extension specification version. */
public static final int XR_KHR_vulkan_enable2_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_KHR_VULKAN_ENABLE2_EXTENSION_NAME = "XR_KHR_vulkan_enable2";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_VULKAN_INSTANCE_CREATE_INFO_KHR TYPE_VULKAN_INSTANCE_CREATE_INFO_KHR}
* - {@link #XR_TYPE_VULKAN_DEVICE_CREATE_INFO_KHR TYPE_VULKAN_DEVICE_CREATE_INFO_KHR}
* - {@link #XR_TYPE_VULKAN_GRAPHICS_DEVICE_GET_INFO_KHR TYPE_VULKAN_GRAPHICS_DEVICE_GET_INFO_KHR}
* - {@link #XR_TYPE_GRAPHICS_BINDING_VULKAN2_KHR TYPE_GRAPHICS_BINDING_VULKAN2_KHR}
* - {@link #XR_TYPE_SWAPCHAIN_IMAGE_VULKAN2_KHR TYPE_SWAPCHAIN_IMAGE_VULKAN2_KHR}
* - {@link #XR_TYPE_GRAPHICS_REQUIREMENTS_VULKAN2_KHR TYPE_GRAPHICS_REQUIREMENTS_VULKAN2_KHR}
*
*/
public static final int
XR_TYPE_VULKAN_INSTANCE_CREATE_INFO_KHR = 1000090000,
XR_TYPE_VULKAN_DEVICE_CREATE_INFO_KHR = 1000090001,
XR_TYPE_VULKAN_GRAPHICS_DEVICE_GET_INFO_KHR = 1000090003,
XR_TYPE_GRAPHICS_BINDING_VULKAN2_KHR = 1000090000,
XR_TYPE_SWAPCHAIN_IMAGE_VULKAN2_KHR = 1000090001,
XR_TYPE_GRAPHICS_REQUIREMENTS_VULKAN2_KHR = 1000090002;
protected KHRVulkanEnable2() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateVulkanInstanceKHR ] ---
/** Unsafe version of: {@link #xrCreateVulkanInstanceKHR CreateVulkanInstanceKHR} */
public static int nxrCreateVulkanInstanceKHR(XrInstance instance, long createInfo, long vulkanInstance, long vulkanResult) {
long __functionAddress = instance.getCapabilities().xrCreateVulkanInstanceKHR;
if (CHECKS) {
check(__functionAddress);
XrVulkanInstanceCreateInfoKHR.validate(createInfo);
}
return callPPPPI(instance.address(), createInfo, vulkanInstance, vulkanResult, __functionAddress);
}
/**
* Create an OpenXR compatible VkInstance.
*
* C Specification
*
*
* XrResult xrCreateVulkanInstanceKHR(
* XrInstance instance,
* const XrVulkanInstanceCreateInfoKHR* createInfo,
* VkInstance* vulkanInstance,
* VkResult* vulkanResult);
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRVulkanEnable2 XR_KHR_vulkan_enable2} extension must be enabled prior to calling {@link #xrCreateVulkanInstanceKHR CreateVulkanInstanceKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code createInfo} must be a pointer to a valid {@link XrVulkanInstanceCreateInfoKHR} structure
* - {@code vulkanInstance} must be a pointer to a {@code VkInstance} value
* - {@code vulkanResult} must be a pointer to a {@code VkResult} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* See Also
*
* {@link XrVulkanInstanceCreateInfoKHR}, {@link #xrCreateVulkanDeviceKHR CreateVulkanDeviceKHR}
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param createInfo extensible input struct of type {@link XrVulkanInstanceCreateInfoKHR}
* @param vulkanInstance points to a {@code VkInstance} handle to populate with the new Vulkan instance.
* @param vulkanResult points to a {@code VkResult} to populate with the result of the {@code vkCreateInstance} operation as returned by {@link XrVulkanInstanceCreateInfoKHR}{@code ::pfnGetInstanceProcAddr}.
*/
@NativeType("XrResult")
public static int xrCreateVulkanInstanceKHR(XrInstance instance, @NativeType("XrVulkanInstanceCreateInfoKHR const *") XrVulkanInstanceCreateInfoKHR createInfo, @NativeType("VkInstance *") PointerBuffer vulkanInstance, @NativeType("VkResult *") IntBuffer vulkanResult) {
if (CHECKS) {
check(vulkanInstance, 1);
check(vulkanResult, 1);
}
return nxrCreateVulkanInstanceKHR(instance, createInfo.address(), memAddress(vulkanInstance), memAddress(vulkanResult));
}
// --- [ xrCreateVulkanDeviceKHR ] ---
/** Unsafe version of: {@link #xrCreateVulkanDeviceKHR CreateVulkanDeviceKHR} */
public static int nxrCreateVulkanDeviceKHR(XrInstance instance, long createInfo, long vulkanDevice, long vulkanResult) {
long __functionAddress = instance.getCapabilities().xrCreateVulkanDeviceKHR;
if (CHECKS) {
check(__functionAddress);
XrVulkanDeviceCreateInfoKHR.validate(createInfo);
}
return callPPPPI(instance.address(), createInfo, vulkanDevice, vulkanResult, __functionAddress);
}
/**
* Create an OpenXR compatible VkDevice.
*
* C Specification
*
*
* XrResult xrCreateVulkanDeviceKHR(
* XrInstance instance,
* const XrVulkanDeviceCreateInfoKHR* createInfo,
* VkDevice* vulkanDevice,
* VkResult* vulkanResult);
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRVulkanEnable2 XR_KHR_vulkan_enable2} extension must be enabled prior to calling {@link #xrCreateVulkanDeviceKHR CreateVulkanDeviceKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code createInfo} must be a pointer to a valid {@link XrVulkanDeviceCreateInfoKHR} structure
* - {@code vulkanDevice} must be a pointer to a {@code VkDevice} value
* - {@code vulkanResult} must be a pointer to a {@code VkResult} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* See Also
*
* {@link XrVulkanDeviceCreateInfoKHR}, {@link #xrCreateVulkanInstanceKHR CreateVulkanInstanceKHR}
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param createInfo extensible input struct of type {@code XrCreateVulkanDeviceCreateInfoKHR}
* @param vulkanDevice points to a {@code VkDevice} handle to populate with the new Vulkan device.
* @param vulkanResult points to a {@code VkResult} to populate with the result of the {@code vkCreateDevice} operation as returned by {@link XrVulkanInstanceCreateInfoKHR}{@code ::pfnGetInstanceProcAddr}.
*/
@NativeType("XrResult")
public static int xrCreateVulkanDeviceKHR(XrInstance instance, @NativeType("XrVulkanDeviceCreateInfoKHR const *") XrVulkanDeviceCreateInfoKHR createInfo, @NativeType("VkDevice *") PointerBuffer vulkanDevice, @NativeType("VkResult *") IntBuffer vulkanResult) {
if (CHECKS) {
check(vulkanDevice, 1);
check(vulkanResult, 1);
}
return nxrCreateVulkanDeviceKHR(instance, createInfo.address(), memAddress(vulkanDevice), memAddress(vulkanResult));
}
// --- [ xrGetVulkanGraphicsDevice2KHR ] ---
/** Unsafe version of: {@link #xrGetVulkanGraphicsDevice2KHR GetVulkanGraphicsDevice2KHR} */
public static int nxrGetVulkanGraphicsDevice2KHR(XrInstance instance, long getInfo, long vulkanPhysicalDevice) {
long __functionAddress = instance.getCapabilities().xrGetVulkanGraphicsDevice2KHR;
if (CHECKS) {
check(__functionAddress);
XrVulkanGraphicsDeviceGetInfoKHR.validate(getInfo);
}
return callPPPI(instance.address(), getInfo, vulkanPhysicalDevice, __functionAddress);
}
/**
* Retrieve the Vulkan physical device associated with an OpenXR instance and system.
*
* C Specification
*
*
* XrResult xrGetVulkanGraphicsDevice2KHR(
* XrInstance instance,
* const XrVulkanGraphicsDeviceGetInfoKHR* getInfo,
* VkPhysicalDevice* vulkanPhysicalDevice);
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRVulkanEnable2 XR_KHR_vulkan_enable2} extension must be enabled prior to calling {@link #xrGetVulkanGraphicsDevice2KHR GetVulkanGraphicsDevice2KHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code getInfo} must be a pointer to a valid {@link XrVulkanGraphicsDeviceGetInfoKHR} structure
* - {@code vulkanPhysicalDevice} must be a pointer to a {@code VkPhysicalDevice} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* See Also
*
* {@link XrVulkanGraphicsDeviceGetInfoKHR}
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param getInfo extensible input struct of type {@link XrVulkanGraphicsDeviceGetInfoKHR}
* @param vulkanPhysicalDevice a pointer to a {@code VkPhysicalDevice} handle to populate.
*/
@NativeType("XrResult")
public static int xrGetVulkanGraphicsDevice2KHR(XrInstance instance, @NativeType("XrVulkanGraphicsDeviceGetInfoKHR const *") XrVulkanGraphicsDeviceGetInfoKHR getInfo, @NativeType("VkPhysicalDevice *") PointerBuffer vulkanPhysicalDevice) {
if (CHECKS) {
check(vulkanPhysicalDevice, 1);
}
return nxrGetVulkanGraphicsDevice2KHR(instance, getInfo.address(), memAddress(vulkanPhysicalDevice));
}
// --- [ xrGetVulkanGraphicsRequirements2KHR ] ---
/** Unsafe version of: {@link #xrGetVulkanGraphicsRequirements2KHR GetVulkanGraphicsRequirements2KHR} */
public static int nxrGetVulkanGraphicsRequirements2KHR(XrInstance instance, long systemId, long graphicsRequirements) {
long __functionAddress = instance.getCapabilities().xrGetVulkanGraphicsRequirements2KHR;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(instance.address(), systemId, graphicsRequirements, __functionAddress);
}
/**
* See {@link KHRVulkanEnable#xrGetVulkanGraphicsRequirementsKHR GetVulkanGraphicsRequirementsKHR}.
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param systemId an {@code XrSystemId} handle for the system which will be used to create a session.
* @param graphicsRequirements the {@link XrGraphicsRequirementsVulkanKHR} output structure.
*/
@NativeType("XrResult")
public static int xrGetVulkanGraphicsRequirements2KHR(XrInstance instance, @NativeType("XrSystemId") long systemId, @NativeType("XrGraphicsRequirementsVulkanKHR *") XrGraphicsRequirementsVulkanKHR graphicsRequirements) {
return nxrGetVulkanGraphicsRequirements2KHR(instance, systemId, graphicsRequirements.address());
}
}