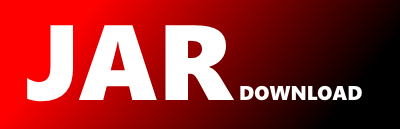
org.lwjgl.openxr.KHRWin32ConvertPerformanceCounterTime Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
import org.lwjgl.system.windows.*;
/**
* The XR_KHR_win32_convert_performance_counter_time extension.
*
* This extension provides two functions for converting between the Windows performance counter (QPC) time stamps and {@code XrTime}. The {@link #xrConvertWin32PerformanceCounterToTimeKHR ConvertWin32PerformanceCounterToTimeKHR} function converts from Windows performance counter time stamps to {@code XrTime}, while the {@link #xrConvertTimeToWin32PerformanceCounterKHR ConvertTimeToWin32PerformanceCounterKHR} function converts {@code XrTime} to Windows performance counter time stamps. The primary use case for this functionality is to be able to synchronize events between the local system and the OpenXR system.
*/
public class KHRWin32ConvertPerformanceCounterTime {
/** The extension specification version. */
public static final int XR_KHR_win32_convert_performance_counter_time_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_KHR_WIN32_CONVERT_PERFORMANCE_COUNTER_TIME_EXTENSION_NAME = "XR_KHR_win32_convert_performance_counter_time";
protected KHRWin32ConvertPerformanceCounterTime() {
throw new UnsupportedOperationException();
}
// --- [ xrConvertWin32PerformanceCounterToTimeKHR ] ---
/** Unsafe version of: {@link #xrConvertWin32PerformanceCounterToTimeKHR ConvertWin32PerformanceCounterToTimeKHR} */
public static int nxrConvertWin32PerformanceCounterToTimeKHR(XrInstance instance, long performanceCounter, long time) {
long __functionAddress = instance.getCapabilities().xrConvertWin32PerformanceCounterToTimeKHR;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(instance.address(), performanceCounter, time, __functionAddress);
}
/**
* Convert Win32 {@code QueryPerformanceCounter} time to XrTime.
*
* C Specification
*
* To convert from a Windows performance counter time stamp to {@code XrTime}, call:
*
*
* XrResult xrConvertWin32PerformanceCounterToTimeKHR(
* XrInstance instance,
* const LARGE_INTEGER* performanceCounter,
* XrTime* time);
*
* Description
*
* The {@link #xrConvertWin32PerformanceCounterToTimeKHR ConvertWin32PerformanceCounterToTimeKHR} function converts a time stamp obtained by the {@code QueryPerformanceCounter} Windows function to the equivalent {@code XrTime}.
*
* If the output {@code time} cannot represent the input {@code performanceCounter}, the runtime must return {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}.
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRWin32ConvertPerformanceCounterTime XR_KHR_win32_convert_performance_counter_time} extension must be enabled prior to calling {@link #xrConvertWin32PerformanceCounterToTimeKHR ConvertWin32PerformanceCounterToTimeKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code performanceCounter} must be a pointer to a valid {@code LARGE_INTEGER} value
* - {@code time} must be a pointer to an {@code XrTime} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
*
*
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param performanceCounter a time returned by {@code QueryPerformanceCounter}.
* @param time the resulting {@code XrTime} that is equivalent to the {@code performanceCounter}.
*/
@NativeType("XrResult")
public static int xrConvertWin32PerformanceCounterToTimeKHR(XrInstance instance, @NativeType("LARGE_INTEGER const *") LARGE_INTEGER performanceCounter, @NativeType("XrTime *") LongBuffer time) {
if (CHECKS) {
check(time, 1);
}
return nxrConvertWin32PerformanceCounterToTimeKHR(instance, performanceCounter.address(), memAddress(time));
}
// --- [ xrConvertTimeToWin32PerformanceCounterKHR ] ---
/** Unsafe version of: {@link #xrConvertTimeToWin32PerformanceCounterKHR ConvertTimeToWin32PerformanceCounterKHR} */
public static int nxrConvertTimeToWin32PerformanceCounterKHR(XrInstance instance, long time, long performanceCounter) {
long __functionAddress = instance.getCapabilities().xrConvertTimeToWin32PerformanceCounterKHR;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(instance.address(), time, performanceCounter, __functionAddress);
}
/**
* Convert XrTime to Win32 {@code QueryPerformanceCounter} time.
*
* C Specification
*
* To convert from {@code XrTime} to a Windows performance counter time stamp, call:
*
*
* XrResult xrConvertTimeToWin32PerformanceCounterKHR(
* XrInstance instance,
* XrTime time,
* LARGE_INTEGER* performanceCounter);
*
* Description
*
* The {@link #xrConvertTimeToWin32PerformanceCounterKHR ConvertTimeToWin32PerformanceCounterKHR} function converts an {@code XrTime} to time as if generated by the {@code QueryPerformanceCounter} Windows function.
*
* If the output {@code performanceCounter} cannot represent the input {@code time}, the runtime must return {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}.
*
* Valid Usage (Implicit)
*
*
* - The {@link KHRWin32ConvertPerformanceCounterTime XR_KHR_win32_convert_performance_counter_time} extension must be enabled prior to calling {@link #xrConvertTimeToWin32PerformanceCounterKHR ConvertTimeToWin32PerformanceCounterKHR}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code performanceCounter} must be a pointer to a {@code LARGE_INTEGER} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
*
*
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param time an {@code XrTime}.
* @param performanceCounter the resulting Windows performance counter time stamp that is equivalent to the {@code time}.
*/
@NativeType("XrResult")
public static int xrConvertTimeToWin32PerformanceCounterKHR(XrInstance instance, @NativeType("XrTime") long time, @NativeType("LARGE_INTEGER *") LARGE_INTEGER performanceCounter) {
return nxrConvertTimeToWin32PerformanceCounterKHR(instance, time, performanceCounter.address());
}
}