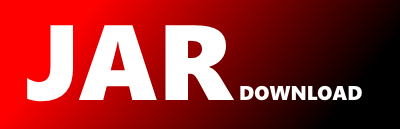
org.lwjgl.openxr.METAColocationDiscovery Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_META_colocation_discovery extension.
*
* Colocation discovery is a capability available through the {@link METAColocationDiscovery XR_META_colocation_discovery} extension that allows apps to discover physically colocated devices running the same app.
*
* In the context of this extension, "the same application" means "bytewise identical Android package name" when running on an Android-based platform.
*/
public class METAColocationDiscovery {
/** The extension specification version. */
public static final int XR_META_colocation_discovery_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_META_COLOCATION_DISCOVERY_EXTENSION_NAME = "XR_META_colocation_discovery";
/** XR_MAX_COLOCATION_DISCOVERY_BUFFER_SIZE_META */
public static final int XR_MAX_COLOCATION_DISCOVERY_BUFFER_SIZE_META = 1024;
/**
* Extends {@code XrResult}.
*
* Enum values:
*
*
* - {@link #XR_ERROR_COLOCATION_DISCOVERY_NETWORK_FAILED_META ERROR_COLOCATION_DISCOVERY_NETWORK_FAILED_META}
* - {@link #XR_ERROR_COLOCATION_DISCOVERY_NO_DISCOVERY_METHOD_META ERROR_COLOCATION_DISCOVERY_NO_DISCOVERY_METHOD_META}
* - {@link #XR_COLOCATION_DISCOVERY_ALREADY_ADVERTISING_META COLOCATION_DISCOVERY_ALREADY_ADVERTISING_META}
* - {@link #XR_COLOCATION_DISCOVERY_ALREADY_DISCOVERING_META COLOCATION_DISCOVERY_ALREADY_DISCOVERING_META}
*
*/
public static final int
XR_ERROR_COLOCATION_DISCOVERY_NETWORK_FAILED_META = -1000571001,
XR_ERROR_COLOCATION_DISCOVERY_NO_DISCOVERY_METHOD_META = -1000571002,
XR_COLOCATION_DISCOVERY_ALREADY_ADVERTISING_META = 1000571003,
XR_COLOCATION_DISCOVERY_ALREADY_DISCOVERING_META = 1000571004;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_COLOCATION_DISCOVERY_START_INFO_META TYPE_COLOCATION_DISCOVERY_START_INFO_META}
* - {@link #XR_TYPE_COLOCATION_DISCOVERY_STOP_INFO_META TYPE_COLOCATION_DISCOVERY_STOP_INFO_META}
* - {@link #XR_TYPE_COLOCATION_ADVERTISEMENT_START_INFO_META TYPE_COLOCATION_ADVERTISEMENT_START_INFO_META}
* - {@link #XR_TYPE_COLOCATION_ADVERTISEMENT_STOP_INFO_META TYPE_COLOCATION_ADVERTISEMENT_STOP_INFO_META}
* - {@link #XR_TYPE_EVENT_DATA_START_COLOCATION_ADVERTISEMENT_COMPLETE_META TYPE_EVENT_DATA_START_COLOCATION_ADVERTISEMENT_COMPLETE_META}
* - {@link #XR_TYPE_EVENT_DATA_STOP_COLOCATION_ADVERTISEMENT_COMPLETE_META TYPE_EVENT_DATA_STOP_COLOCATION_ADVERTISEMENT_COMPLETE_META}
* - {@link #XR_TYPE_EVENT_DATA_COLOCATION_ADVERTISEMENT_COMPLETE_META TYPE_EVENT_DATA_COLOCATION_ADVERTISEMENT_COMPLETE_META}
* - {@link #XR_TYPE_EVENT_DATA_START_COLOCATION_DISCOVERY_COMPLETE_META TYPE_EVENT_DATA_START_COLOCATION_DISCOVERY_COMPLETE_META}
* - {@link #XR_TYPE_EVENT_DATA_COLOCATION_DISCOVERY_RESULT_META TYPE_EVENT_DATA_COLOCATION_DISCOVERY_RESULT_META}
* - {@link #XR_TYPE_EVENT_DATA_COLOCATION_DISCOVERY_COMPLETE_META TYPE_EVENT_DATA_COLOCATION_DISCOVERY_COMPLETE_META}
* - {@link #XR_TYPE_EVENT_DATA_STOP_COLOCATION_DISCOVERY_COMPLETE_META TYPE_EVENT_DATA_STOP_COLOCATION_DISCOVERY_COMPLETE_META}
* - {@link #XR_TYPE_SYSTEM_COLOCATION_DISCOVERY_PROPERTIES_META TYPE_SYSTEM_COLOCATION_DISCOVERY_PROPERTIES_META}
*
*/
public static final int
XR_TYPE_COLOCATION_DISCOVERY_START_INFO_META = 1000571010,
XR_TYPE_COLOCATION_DISCOVERY_STOP_INFO_META = 1000571011,
XR_TYPE_COLOCATION_ADVERTISEMENT_START_INFO_META = 1000571012,
XR_TYPE_COLOCATION_ADVERTISEMENT_STOP_INFO_META = 1000571013,
XR_TYPE_EVENT_DATA_START_COLOCATION_ADVERTISEMENT_COMPLETE_META = 1000571020,
XR_TYPE_EVENT_DATA_STOP_COLOCATION_ADVERTISEMENT_COMPLETE_META = 1000571021,
XR_TYPE_EVENT_DATA_COLOCATION_ADVERTISEMENT_COMPLETE_META = 1000571022,
XR_TYPE_EVENT_DATA_START_COLOCATION_DISCOVERY_COMPLETE_META = 1000571023,
XR_TYPE_EVENT_DATA_COLOCATION_DISCOVERY_RESULT_META = 1000571024,
XR_TYPE_EVENT_DATA_COLOCATION_DISCOVERY_COMPLETE_META = 1000571025,
XR_TYPE_EVENT_DATA_STOP_COLOCATION_DISCOVERY_COMPLETE_META = 1000571026,
XR_TYPE_SYSTEM_COLOCATION_DISCOVERY_PROPERTIES_META = 1000571030;
protected METAColocationDiscovery() {
throw new UnsupportedOperationException();
}
// --- [ xrStartColocationDiscoveryMETA ] ---
/** Unsafe version of: {@link #xrStartColocationDiscoveryMETA StartColocationDiscoveryMETA} */
public static int nxrStartColocationDiscoveryMETA(XrSession session, long info, long discoveryRequestId) {
long __functionAddress = session.getCapabilities().xrStartColocationDiscoveryMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), info, discoveryRequestId, __functionAddress);
}
/**
* Start colocation discovery.
*
* C Specification
*
* The {@link #xrStartColocationDiscoveryMETA StartColocationDiscoveryMETA} function is defined as:
*
*
* XrResult xrStartColocationDiscoveryMETA(
* XrSession session,
* const XrColocationDiscoveryStartInfoMETA* info,
* XrAsyncRequestIdFB* discoveryRequestId);
*
* Description
*
* The application can call {@link #xrStartColocationDiscoveryMETA StartColocationDiscoveryMETA} to start discovering physically colocated devices.
*
* If the system does not support colocation advertisement and discovery, the runtime must return {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED} from {@link #xrStartColocationDiscoveryMETA StartColocationDiscoveryMETA}. In this case, the runtime must return {@link XR10#XR_FALSE FALSE} for {@link XrSystemColocationDiscoveryPropertiesMETA}{@code ::supportsColocationDiscovery} when the function {@link XR10#xrGetSystemProperties GetSystemProperties} is called, so that the application knows to not use this functionality.
*
* This is an asynchronous operation. Completion results are conveyed in the event {@link XrEventDataStartColocationDiscoveryCompleteMETA}.
*
* If the asynchronous operation is scheduled successfully, the runtime must return {@link XR10#XR_SUCCESS SUCCESS}.
*
* If and only if the runtime returns {@link XR10#XR_SUCCESS SUCCESS}, the runtime must queue a single {@link XrEventDataStartColocationDiscoveryCompleteMETA} event identified with a {@code discoveryRequestId} matching the {@code discoveryRequestId} value output by this function, referred to as the "corresponding completion event."
*
* (This implies that if the runtime returns anything other than {@link XR10#XR_SUCCESS SUCCESS}, the runtime must not queue any {@link XrEventDataStartColocationDiscoveryCompleteMETA} events with {@code discoveryRequestId} field matching the {@code discoveryRequestId} populated by this function.)
*
* If the asynchronous operation is successful, in the corresponding completion event, the runtime must set the {@link XrEventDataStartColocationDiscoveryCompleteMETA}{@code ::result} field to {@link XR10#XR_SUCCESS SUCCESS}. The runtime may subsequently queue zero or more {@link XrEventDataColocationDiscoveryResultMETA} events asynchronously as the runtime discovers nearby advertisements. Once the application or runtime stops the colocation discovery, the runtime must queue a single {@link XrEventDataColocationDiscoveryCompleteMETA} event. All {@link XrEventDataColocationDiscoveryResultMETA} and {@link XrEventDataColocationDiscoveryCompleteMETA} events will identified with {@code discoveryRequestId} matching the value populated in {@code discoveryRequestId} by {@link #xrStartColocationDiscoveryMETA StartColocationDiscoveryMETA}.
*
* If the asynchronous operation is scheduled but not successful, in the corresponding completion event, the runtime must set the {@link XrEventDataStartColocationDiscoveryCompleteMETA}{@code ::result} field to an appropriate error code instead of {@link XR10#XR_SUCCESS SUCCESS}.
*
* If the application already has an active colocation discovery, in the corresponding completion event, the runtime must set the {@link XrEventDataStartColocationDiscoveryCompleteMETA}{@code ::result} field to {@link #XR_COLOCATION_DISCOVERY_ALREADY_DISCOVERING_META COLOCATION_DISCOVERY_ALREADY_DISCOVERING_META}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAColocationDiscovery XR_META_colocation_discovery} extension must be enabled prior to calling {@link #xrStartColocationDiscoveryMETA StartColocationDiscoveryMETA}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrColocationDiscoveryStartInfoMETA} structure
* - {@code discoveryRequestId} must be a pointer to an {@code XrAsyncRequestIdFB} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_RUNTIME_UNAVAILABLE ERROR_RUNTIME_UNAVAILABLE}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link XR10#XR_ERROR_EXTENSION_NOT_PRESENT ERROR_EXTENSION_NOT_PRESENT}
*
*
*
* See Also
*
* {@link XrColocationDiscoveryStartInfoMETA}, {@link XrEventDataStartColocationDiscoveryCompleteMETA}
*
* @param session an {@code XrSession} in which the colocation discovery will be active.
* @param info a pointer to {@link XrColocationDiscoveryStartInfoMETA} structure to specify the discovery request information.
* @param discoveryRequestId an output parameter, and the variable it points to will be populated with the ID of this asynchronous request. Note that this ID is used for associating additional events with this original call, in addition to the typical completion event.
*/
@NativeType("XrResult")
public static int xrStartColocationDiscoveryMETA(XrSession session, @NativeType("XrColocationDiscoveryStartInfoMETA const *") XrColocationDiscoveryStartInfoMETA info, @NativeType("XrAsyncRequestIdFB *") LongBuffer discoveryRequestId) {
if (CHECKS) {
check(discoveryRequestId, 1);
}
return nxrStartColocationDiscoveryMETA(session, info.address(), memAddress(discoveryRequestId));
}
// --- [ xrStopColocationDiscoveryMETA ] ---
/** Unsafe version of: {@link #xrStopColocationDiscoveryMETA StopColocationDiscoveryMETA} */
public static int nxrStopColocationDiscoveryMETA(XrSession session, long info, long requestId) {
long __functionAddress = session.getCapabilities().xrStopColocationDiscoveryMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), info, requestId, __functionAddress);
}
/**
* Stop ongoing discovery process.
*
* C Specification
*
* The {@link #xrStopColocationDiscoveryMETA StopColocationDiscoveryMETA} function is defined as:
*
*
* XrResult xrStopColocationDiscoveryMETA(
* XrSession session,
* const XrColocationDiscoveryStopInfoMETA* info,
* XrAsyncRequestIdFB* requestId);
*
* Description
*
* The application can call {@link #xrStopColocationDiscoveryMETA StopColocationDiscoveryMETA} to stop an ongoing discovery process that was started by {@link #xrStartColocationDiscoveryMETA StartColocationDiscoveryMETA}.
*
* If the system does not support colocation advertisement and discovery, the runtime must return {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED} from {@link #xrStopColocationDiscoveryMETA StopColocationDiscoveryMETA}. In this case, the runtime must return {@link XR10#XR_FALSE FALSE} for {@link XrSystemColocationDiscoveryPropertiesMETA}{@code ::supportsColocationDiscovery} when the function {@link XR10#xrGetSystemProperties GetSystemProperties} is called, so that the application knows to not use this functionality.
*
* This is an asynchronous operation. Completion results are conveyed in the event {@link XrEventDataStopColocationDiscoveryCompleteMETA}.
*
* If the asynchronous operation is scheduled successfully, the runtime must return {@link XR10#XR_SUCCESS SUCCESS}.
*
* If and only if the runtime returns {@link XR10#XR_SUCCESS SUCCESS}, the runtime must queue a single {@link XrEventDataStopColocationDiscoveryCompleteMETA} event identified with a {@code requestId} matching the {@code requestId} value output by this function, referred to as the "corresponding completion event."
*
* (This implies that if the runtime returns anything other than {@link XR10#XR_SUCCESS SUCCESS}, the runtime must not queue any {@link XrEventDataStopColocationDiscoveryCompleteMETA} events with {@code requestId} field matching the {@code requestId} populated by this function.)
*
* If the asynchronous operation is successful, in the corresponding completion event, the runtime must set the {@link XrEventDataStopColocationDiscoveryCompleteMETA}{@code ::result} field to {@link XR10#XR_SUCCESS SUCCESS}.
*
* If the asynchronous operation is scheduled but not successful, in the corresponding completion event, the runtime must set the {@link XrEventDataStopColocationDiscoveryCompleteMETA}{@code ::result} field to an appropriate error code instead of {@link XR10#XR_SUCCESS SUCCESS}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAColocationDiscovery XR_META_colocation_discovery} extension must be enabled prior to calling {@link #xrStopColocationDiscoveryMETA StopColocationDiscoveryMETA}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrColocationDiscoveryStopInfoMETA} structure
* - {@code requestId} must be a pointer to an {@code XrAsyncRequestIdFB} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_RUNTIME_UNAVAILABLE ERROR_RUNTIME_UNAVAILABLE}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link XR10#XR_ERROR_EXTENSION_NOT_PRESENT ERROR_EXTENSION_NOT_PRESENT}
*
*
*
* See Also
*
* {@link XrColocationDiscoveryStopInfoMETA}, {@link #xrStartColocationDiscoveryMETA StartColocationDiscoveryMETA}
*
* @param session an {@code XrSession} in which the colocation discovery will be active.
* @param info a pointer to {@link XrColocationDiscoveryStopInfoMETA} structure to specify the stop discovery request information.
* @param requestId an output parameter, and the variable it points to will be populated with the ID of this asynchronous request.
*/
@NativeType("XrResult")
public static int xrStopColocationDiscoveryMETA(XrSession session, @NativeType("XrColocationDiscoveryStopInfoMETA const *") XrColocationDiscoveryStopInfoMETA info, @NativeType("XrAsyncRequestIdFB *") LongBuffer requestId) {
if (CHECKS) {
check(requestId, 1);
}
return nxrStopColocationDiscoveryMETA(session, info.address(), memAddress(requestId));
}
// --- [ xrStartColocationAdvertisementMETA ] ---
/** Unsafe version of: {@link #xrStartColocationAdvertisementMETA StartColocationAdvertisementMETA} */
public static int nxrStartColocationAdvertisementMETA(XrSession session, long info, long advertisementRequestId) {
long __functionAddress = session.getCapabilities().xrStartColocationAdvertisementMETA;
if (CHECKS) {
check(__functionAddress);
XrColocationAdvertisementStartInfoMETA.validate(info);
}
return callPPPI(session.address(), info, advertisementRequestId, __functionAddress);
}
/**
* Start colocation visibility.
*
* C Specification
*
* The {@link #xrStartColocationAdvertisementMETA StartColocationAdvertisementMETA} function is defined as:
*
*
* XrResult xrStartColocationAdvertisementMETA(
* XrSession session,
* const XrColocationAdvertisementStartInfoMETA* info,
* XrAsyncRequestIdFB* advertisementRequestId);
*
* Description
*
* The {@link #xrStartColocationAdvertisementMETA StartColocationAdvertisementMETA} function requests that the current device become discoverable by other physically colocated devices running the same application.
*
* If the system does not support colocation advertisement and discovery, the runtime must return {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED} from {@link #xrStartColocationAdvertisementMETA StartColocationAdvertisementMETA}. In this case, the runtime must return {@link XR10#XR_FALSE FALSE} for {@link XrSystemColocationDiscoveryPropertiesMETA}{@code ::supportsColocationDiscovery} when the function {@link XR10#xrGetSystemProperties GetSystemProperties} is called, so that the application knows to not use this functionality.
*
* This is an asynchronous operation. Completion results are conveyed in the event {@link XrEventDataStartColocationAdvertisementCompleteMETA}.
*
* If the asynchronous operation is scheduled successfully, the runtime must return {@link XR10#XR_SUCCESS SUCCESS}.
*
* If and only if the runtime returns {@link XR10#XR_SUCCESS SUCCESS}, the runtime must queue a single {@link XrEventDataStartColocationAdvertisementCompleteMETA} event identified with a {@code advertisementRequestId} matching the {@code advertisementRequestId} value output by this function, referred to as the "corresponding completion event."
*
* (This implies that if the runtime returns anything other than {@link XR10#XR_SUCCESS SUCCESS}, the runtime must not queue any {@link XrEventDataStartColocationAdvertisementCompleteMETA} events with {@code advertisementRequestId} field matching the {@code advertisementRequestId} populated by this function.)
*
* If the asynchronous operation is successful, in the corresponding completion event, the runtime must set the {@link XrEventDataStartColocationAdvertisementCompleteMETA}{@code ::result} field to {@link XR10#XR_SUCCESS SUCCESS}.
*
* If the asynchronous operation is scheduled but not successful, in the corresponding completion event, the runtime must set the {@link XrEventDataStartColocationAdvertisementCompleteMETA}{@code ::result} field to an appropriate error code instead of {@link XR10#XR_SUCCESS SUCCESS}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAColocationDiscovery XR_META_colocation_discovery} extension must be enabled prior to calling {@link #xrStartColocationAdvertisementMETA StartColocationAdvertisementMETA}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrColocationAdvertisementStartInfoMETA} structure
* - {@code advertisementRequestId} must be a pointer to an {@code XrAsyncRequestIdFB} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_RUNTIME_UNAVAILABLE ERROR_RUNTIME_UNAVAILABLE}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link XR10#XR_ERROR_EXTENSION_NOT_PRESENT ERROR_EXTENSION_NOT_PRESENT}
*
*
*
* See Also
*
* {@link XrColocationAdvertisementStartInfoMETA}, {@link XrEventDataStartColocationAdvertisementCompleteMETA}
*
* @param session an {@code XrSession} in which the colocation discovery will be active.
* @param info a pointer to {@link XrColocationAdvertisementStartInfoMETA} structure to specify the visibility configuration.
* @param advertisementRequestId an output parameter, and the variable it points to will be populated with the ID of this asynchronous request. Note that this ID is used for associating additional events with this original call, in addition to the typical completion event.
*/
@NativeType("XrResult")
public static int xrStartColocationAdvertisementMETA(XrSession session, @NativeType("XrColocationAdvertisementStartInfoMETA const *") XrColocationAdvertisementStartInfoMETA info, @NativeType("XrAsyncRequestIdFB *") LongBuffer advertisementRequestId) {
if (CHECKS) {
check(advertisementRequestId, 1);
}
return nxrStartColocationAdvertisementMETA(session, info.address(), memAddress(advertisementRequestId));
}
// --- [ xrStopColocationAdvertisementMETA ] ---
/** Unsafe version of: {@link #xrStopColocationAdvertisementMETA StopColocationAdvertisementMETA} */
public static int nxrStopColocationAdvertisementMETA(XrSession session, long info, long requestId) {
long __functionAddress = session.getCapabilities().xrStopColocationAdvertisementMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), info, requestId, __functionAddress);
}
/**
* Stop colocation advertisement.
*
* C Specification
*
* The {@link #xrStopColocationAdvertisementMETA StopColocationAdvertisementMETA} function is defined as:
*
*
* XrResult xrStopColocationAdvertisementMETA(
* XrSession session,
* const XrColocationAdvertisementStopInfoMETA* info,
* XrAsyncRequestIdFB* requestId);
*
* Description
*
* The application can use the {@link #xrStopColocationAdvertisementMETA StopColocationAdvertisementMETA} function to disable the ability for other physically colocated devices running the same application to discover the current device.
*
* If the system does not support colocation advertisement and discovery, the runtime must return {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED} from {@link #xrStopColocationAdvertisementMETA StopColocationAdvertisementMETA}. In this case, the runtime must return {@link XR10#XR_FALSE FALSE} for {@link XrSystemColocationDiscoveryPropertiesMETA}{@code ::supportsColocationDiscovery} when the function {@link XR10#xrGetSystemProperties GetSystemProperties} is called, so that the application knows to not use this functionality.
*
* This is an asynchronous operation. Completion results are conveyed in the event {@link XrEventDataStopColocationAdvertisementCompleteMETA}.
*
* If the asynchronous operation is scheduled successfully, the runtime must return {@link XR10#XR_SUCCESS SUCCESS}.
*
* If and only if the runtime returns {@link XR10#XR_SUCCESS SUCCESS}, the runtime must queue a single {@link XrEventDataStopColocationAdvertisementCompleteMETA} event identified with a {@code requestId} matching the {@code requestId} value output by this function, referred to as the "corresponding completion event."
*
* (This implies that if the runtime returns anything other than {@link XR10#XR_SUCCESS SUCCESS}, the runtime must not queue any {@link XrEventDataStopColocationAdvertisementCompleteMETA} events with {@code requestId} field matching the {@code requestId} populated by this function.)
*
* If the asynchronous operation is successful, in the corresponding completion event, the runtime must set the {@link XrEventDataStopColocationAdvertisementCompleteMETA}{@code ::result} field to {@link XR10#XR_SUCCESS SUCCESS}.
*
* If the asynchronous operation is scheduled but not successful, in the corresponding completion event, the runtime must set the {@link XrEventDataStopColocationAdvertisementCompleteMETA}{@code ::result} field to an appropriate error code instead of {@link XR10#XR_SUCCESS SUCCESS}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAColocationDiscovery XR_META_colocation_discovery} extension must be enabled prior to calling {@link #xrStopColocationAdvertisementMETA StopColocationAdvertisementMETA}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrColocationAdvertisementStopInfoMETA} structure
* - {@code requestId} must be a pointer to an {@code XrAsyncRequestIdFB} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_RUNTIME_UNAVAILABLE ERROR_RUNTIME_UNAVAILABLE}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
* - {@link XR10#XR_ERROR_EXTENSION_NOT_PRESENT ERROR_EXTENSION_NOT_PRESENT}
*
*
*
* See Also
*
* {@link XrColocationAdvertisementStopInfoMETA}, {@link XrEventDataStopColocationAdvertisementCompleteMETA}, {@link #xrStartColocationAdvertisementMETA StartColocationAdvertisementMETA}
*
* @param session an {@code XrSession} in which the colocation discovery will be active.
* @param info a pointer to {@link XrColocationAdvertisementStopInfoMETA} structure to specify the advertisement configuration.
* @param requestId an output parameter, and the variable it points to will be populated with the ID of this asynchronous request.
*/
@NativeType("XrResult")
public static int xrStopColocationAdvertisementMETA(XrSession session, @NativeType("XrColocationAdvertisementStopInfoMETA const *") XrColocationAdvertisementStopInfoMETA info, @NativeType("XrAsyncRequestIdFB *") LongBuffer requestId) {
if (CHECKS) {
check(requestId, 1);
}
return nxrStopColocationAdvertisementMETA(session, info.address(), memAddress(requestId));
}
}