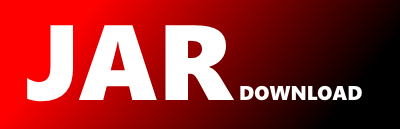
org.lwjgl.openxr.METAEnvironmentDepth Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_META_environment_depth extension.
*
* This extension allows the application to request depth maps of the real-world environment around the headset. The depth maps are generated by the runtime and shared with the application using an {@code XrEnvironmentDepthSwapchainMETA}.
*/
public class METAEnvironmentDepth {
/** The extension specification version. */
public static final int XR_META_environment_depth_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_META_ENVIRONMENT_DEPTH_EXTENSION_NAME = "XR_META_environment_depth";
/**
* Extends {@code XrObjectType}.
*
* Enum values:
*
*
* - {@link #XR_OBJECT_TYPE_ENVIRONMENT_DEPTH_PROVIDER_META OBJECT_TYPE_ENVIRONMENT_DEPTH_PROVIDER_META}
* - {@link #XR_OBJECT_TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_META OBJECT_TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_META}
*
*/
public static final int
XR_OBJECT_TYPE_ENVIRONMENT_DEPTH_PROVIDER_META = 1000291000,
XR_OBJECT_TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_META = 1000291001;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_ENVIRONMENT_DEPTH_PROVIDER_CREATE_INFO_META TYPE_ENVIRONMENT_DEPTH_PROVIDER_CREATE_INFO_META}
* - {@link #XR_TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_CREATE_INFO_META TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_CREATE_INFO_META}
* - {@link #XR_TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_STATE_META TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_STATE_META}
* - {@link #XR_TYPE_ENVIRONMENT_DEPTH_IMAGE_ACQUIRE_INFO_META TYPE_ENVIRONMENT_DEPTH_IMAGE_ACQUIRE_INFO_META}
* - {@link #XR_TYPE_ENVIRONMENT_DEPTH_IMAGE_VIEW_META TYPE_ENVIRONMENT_DEPTH_IMAGE_VIEW_META}
* - {@link #XR_TYPE_ENVIRONMENT_DEPTH_IMAGE_META TYPE_ENVIRONMENT_DEPTH_IMAGE_META}
* - {@link #XR_TYPE_ENVIRONMENT_DEPTH_HAND_REMOVAL_SET_INFO_META TYPE_ENVIRONMENT_DEPTH_HAND_REMOVAL_SET_INFO_META}
* - {@link #XR_TYPE_SYSTEM_ENVIRONMENT_DEPTH_PROPERTIES_META TYPE_SYSTEM_ENVIRONMENT_DEPTH_PROPERTIES_META}
*
*/
public static final int
XR_TYPE_ENVIRONMENT_DEPTH_PROVIDER_CREATE_INFO_META = 1000291000,
XR_TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_CREATE_INFO_META = 1000291001,
XR_TYPE_ENVIRONMENT_DEPTH_SWAPCHAIN_STATE_META = 1000291002,
XR_TYPE_ENVIRONMENT_DEPTH_IMAGE_ACQUIRE_INFO_META = 1000291003,
XR_TYPE_ENVIRONMENT_DEPTH_IMAGE_VIEW_META = 1000291004,
XR_TYPE_ENVIRONMENT_DEPTH_IMAGE_META = 1000291005,
XR_TYPE_ENVIRONMENT_DEPTH_HAND_REMOVAL_SET_INFO_META = 1000291006,
XR_TYPE_SYSTEM_ENVIRONMENT_DEPTH_PROPERTIES_META = 1000291007;
/** Extends {@code XrResult}. */
public static final int XR_ENVIRONMENT_DEPTH_NOT_AVAILABLE_META = 1000291000;
protected METAEnvironmentDepth() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateEnvironmentDepthProviderMETA ] ---
/** Unsafe version of: {@link #xrCreateEnvironmentDepthProviderMETA CreateEnvironmentDepthProviderMETA} */
public static int nxrCreateEnvironmentDepthProviderMETA(XrSession session, long createInfo, long environmentDepthProvider) {
long __functionAddress = session.getCapabilities().xrCreateEnvironmentDepthProviderMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, environmentDepthProvider, __functionAddress);
}
/**
* Creates and initializes a depth provider.
*
* C Specification
*
* The {@link #xrCreateEnvironmentDepthProviderMETA CreateEnvironmentDepthProviderMETA} function is defined as:
*
*
* XrResult xrCreateEnvironmentDepthProviderMETA(
* XrSession session,
* const XrEnvironmentDepthProviderCreateInfoMETA* createInfo,
* XrEnvironmentDepthProviderMETA* environmentDepthProvider);
*
* Description
*
* The {@link #xrCreateEnvironmentDepthProviderMETA CreateEnvironmentDepthProviderMETA} function creates a depth provider instance.
*
* Creating the depth provider may allocate resources, but should not incur any per-frame compute costs until the provider has been started.
*
*
* - Runtimes must create the provider in a stopped state.
* - Runtimes may limit the number of depth providers per {@code XrInstance}. If {@link #xrCreateEnvironmentDepthProviderMETA CreateEnvironmentDepthProviderMETA} fails due to reaching this limit, the runtime must return {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}.
* - Runtimes must support at least 1 provider per {@code XrInstance}.
* - Runtimes may return {@link FBPassthrough#XR_ERROR_NOT_PERMITTED_PASSTHROUGH_FB ERROR_NOT_PERMITTED_PASSTHROUGH_FB} if the app permissions have not been granted to the calling app.
* - Applications can call {@link #xrStartEnvironmentDepthProviderMETA StartEnvironmentDepthProviderMETA} to start the generation of depth maps.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrCreateEnvironmentDepthProviderMETA CreateEnvironmentDepthProviderMETA}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrEnvironmentDepthProviderCreateInfoMETA} structure
* - {@code environmentDepthProvider} must be a pointer to an {@code XrEnvironmentDepthProviderMETA} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link FBPassthrough#XR_ERROR_NOT_PERMITTED_PASSTHROUGH_FB ERROR_NOT_PERMITTED_PASSTHROUGH_FB}
*
*
*
* See Also
*
* {@link XrEnvironmentDepthProviderCreateInfoMETA}
*
* @param session the {@code XrSession}.
* @param createInfo a pointer to an {@link XrEnvironmentDepthProviderCreateInfoMETA} containing creation options for the depth provider.
* @param environmentDepthProvider the returned {@code XrEnvironmentDepthProviderMETA} handle for the created depth provider.
*/
@NativeType("XrResult")
public static int xrCreateEnvironmentDepthProviderMETA(XrSession session, @NativeType("XrEnvironmentDepthProviderCreateInfoMETA const *") XrEnvironmentDepthProviderCreateInfoMETA createInfo, @NativeType("XrEnvironmentDepthProviderMETA *") PointerBuffer environmentDepthProvider) {
if (CHECKS) {
check(environmentDepthProvider, 1);
}
return nxrCreateEnvironmentDepthProviderMETA(session, createInfo.address(), memAddress(environmentDepthProvider));
}
// --- [ xrDestroyEnvironmentDepthProviderMETA ] ---
/**
* Destroys the depth provider and frees all memory and resources.
*
* C Specification
*
* The {@link #xrDestroyEnvironmentDepthProviderMETA DestroyEnvironmentDepthProviderMETA} function is defined as:
*
*
* XrResult xrDestroyEnvironmentDepthProviderMETA(
* XrEnvironmentDepthProviderMETA environmentDepthProvider);
*
* Description
*
* The {@link #xrDestroyEnvironmentDepthProviderMETA DestroyEnvironmentDepthProviderMETA} function destroys the depth provider. After this call the runtime may free all related memory and resources.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrDestroyEnvironmentDepthProviderMETA DestroyEnvironmentDepthProviderMETA}
* - {@code environmentDepthProvider} must be a valid {@code XrEnvironmentDepthProviderMETA} handle
*
*
* Thread Safety
*
*
* - Access to {@code environmentDepthProvider}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* @param environmentDepthProvider an {@code XrEnvironmentDepthProviderMETA} handle for the depth provider.
*/
@NativeType("XrResult")
public static int xrDestroyEnvironmentDepthProviderMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider) {
long __functionAddress = environmentDepthProvider.getCapabilities().xrDestroyEnvironmentDepthProviderMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPI(environmentDepthProvider.address(), __functionAddress);
}
// --- [ xrStartEnvironmentDepthProviderMETA ] ---
/**
* Starts the generation of depth maps.
*
* C Specification
*
* The {@link #xrStartEnvironmentDepthProviderMETA StartEnvironmentDepthProviderMETA} function is defined as:
*
*
* XrResult xrStartEnvironmentDepthProviderMETA(
* XrEnvironmentDepthProviderMETA environmentDepthProvider);
*
* Description
*
* The {@link #xrStartEnvironmentDepthProviderMETA StartEnvironmentDepthProviderMETA} function starts the asynchronous generation of depth maps.
*
* Starting the depth provider may use CPU and GPU resources.
*
* Runtimes must return {@link FBPassthrough#XR_ERROR_UNEXPECTED_STATE_PASSTHROUGH_FB ERROR_UNEXPECTED_STATE_PASSTHROUGH_FB} if {@link #xrStartEnvironmentDepthProviderMETA StartEnvironmentDepthProviderMETA} is called on an already started {@code XrEnvironmentDepthProviderMETA}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrStartEnvironmentDepthProviderMETA StartEnvironmentDepthProviderMETA}
* - {@code environmentDepthProvider} must be a valid {@code XrEnvironmentDepthProviderMETA} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link FBPassthrough#XR_ERROR_UNEXPECTED_STATE_PASSTHROUGH_FB ERROR_UNEXPECTED_STATE_PASSTHROUGH_FB}
*
*
*
* @param environmentDepthProvider an {@code XrEnvironmentDepthProviderMETA} handle for the depth provider.
*/
@NativeType("XrResult")
public static int xrStartEnvironmentDepthProviderMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider) {
long __functionAddress = environmentDepthProvider.getCapabilities().xrStartEnvironmentDepthProviderMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPI(environmentDepthProvider.address(), __functionAddress);
}
// --- [ xrStopEnvironmentDepthProviderMETA ] ---
/**
* Stops the depth feature.
*
* C Specification
*
* The {@link #xrStopEnvironmentDepthProviderMETA StopEnvironmentDepthProviderMETA} function is defined as:
*
*
* XrResult xrStopEnvironmentDepthProviderMETA(
* XrEnvironmentDepthProviderMETA environmentDepthProvider);
*
* Description
*
* The {@link #xrStopEnvironmentDepthProviderMETA StopEnvironmentDepthProviderMETA} function stops the generation of depth maps. This stops all per frame computation of environment depth for the application.
*
* Runtimes must return {@link FBPassthrough#XR_ERROR_UNEXPECTED_STATE_PASSTHROUGH_FB ERROR_UNEXPECTED_STATE_PASSTHROUGH_FB} if {@link #xrStopEnvironmentDepthProviderMETA StopEnvironmentDepthProviderMETA} is called on an already stopped {@code XrEnvironmentDepthProviderMETA}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrStopEnvironmentDepthProviderMETA StopEnvironmentDepthProviderMETA}
* - {@code environmentDepthProvider} must be a valid {@code XrEnvironmentDepthProviderMETA} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link FBPassthrough#XR_ERROR_UNEXPECTED_STATE_PASSTHROUGH_FB ERROR_UNEXPECTED_STATE_PASSTHROUGH_FB}
*
*
*
* @param environmentDepthProvider an {@code XrEnvironmentDepthProviderMETA} handle for the depth provider.
*/
@NativeType("XrResult")
public static int xrStopEnvironmentDepthProviderMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider) {
long __functionAddress = environmentDepthProvider.getCapabilities().xrStopEnvironmentDepthProviderMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPI(environmentDepthProvider.address(), __functionAddress);
}
// --- [ xrCreateEnvironmentDepthSwapchainMETA ] ---
/** Unsafe version of: {@link #xrCreateEnvironmentDepthSwapchainMETA CreateEnvironmentDepthSwapchainMETA} */
public static int nxrCreateEnvironmentDepthSwapchainMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider, long createInfo, long swapchain) {
long __functionAddress = environmentDepthProvider.getCapabilities().xrCreateEnvironmentDepthSwapchainMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(environmentDepthProvider.address(), createInfo, swapchain, __functionAddress);
}
/**
* Returns a readable depth swapchain.
*
* C Specification
*
* The {@link #xrCreateEnvironmentDepthSwapchainMETA CreateEnvironmentDepthSwapchainMETA} function is defined as:
*
*
* XrResult xrCreateEnvironmentDepthSwapchainMETA(
* XrEnvironmentDepthProviderMETA environmentDepthProvider,
* const XrEnvironmentDepthSwapchainCreateInfoMETA* createInfo,
* XrEnvironmentDepthSwapchainMETA* swapchain);
*
* Description
*
* The {@link #xrCreateEnvironmentDepthSwapchainMETA CreateEnvironmentDepthSwapchainMETA} function creates a readable swapchain, which is used for accessing the depth data.
*
* The runtime decides on the resolution and length of the swapchain. Additional information about the swapchain can be accessed by calling {@link #xrGetEnvironmentDepthSwapchainStateMETA GetEnvironmentDepthSwapchainStateMETA}.
*
* Runtimes must create a swapchain with array textures of length 2, which map to a left-eye and right-eye view. View index 0 must represent the left eye and view index 1 must represent the right eye. This is the same convention as for {@link XR10#XR_VIEW_CONFIGURATION_TYPE_PRIMARY_STEREO VIEW_CONFIGURATION_TYPE_PRIMARY_STEREO} in {@code XrViewConfigurationType}. Runtimes must create the swapchain with the following image formats depending on the graphics API associated with the session:
*
*
* - OpenGL: {@code GL_DEPTH_COMPONENT16}
* - Vulkan: {@code VK_FORMAT_D16_UNORM}
* - Direct3D: {@code DXGI_FORMAT_D16_UNORM}
*
*
* Runtimes must only allow maximum one swapchain to exist per depth provider at any given time, and must return {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED} if {@link #xrCreateEnvironmentDepthSwapchainMETA CreateEnvironmentDepthSwapchainMETA} is called to create more. Applications should destroy the swapchain when no longer needed. Applications must be able to handle different swapchain lengths and resolutions.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrCreateEnvironmentDepthSwapchainMETA CreateEnvironmentDepthSwapchainMETA}
* - {@code environmentDepthProvider} must be a valid {@code XrEnvironmentDepthProviderMETA} handle
* - {@code createInfo} must be a pointer to a valid {@link XrEnvironmentDepthSwapchainCreateInfoMETA} structure
* - {@code swapchain} must be a pointer to an {@code XrEnvironmentDepthSwapchainMETA} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* See Also
*
* {@link XrEnvironmentDepthSwapchainCreateInfoMETA}
*
* @param environmentDepthProvider an {@code XrEnvironmentDepthProviderMETA} handle for the depth provider.
* @param createInfo a pointer to an {@link XrEnvironmentDepthSwapchainCreateInfoMETA} containing creation options for the swapchain.
* @param swapchain the returned {@code XrEnvironmentDepthSwapchainMETA} handle for the created swapchain.
*/
@NativeType("XrResult")
public static int xrCreateEnvironmentDepthSwapchainMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider, @NativeType("XrEnvironmentDepthSwapchainCreateInfoMETA const *") XrEnvironmentDepthSwapchainCreateInfoMETA createInfo, @NativeType("XrEnvironmentDepthSwapchainMETA *") PointerBuffer swapchain) {
if (CHECKS) {
check(swapchain, 1);
}
return nxrCreateEnvironmentDepthSwapchainMETA(environmentDepthProvider, createInfo.address(), memAddress(swapchain));
}
// --- [ xrDestroyEnvironmentDepthSwapchainMETA ] ---
/**
* Destroys a readable depth swapchain.
*
* C Specification
*
* The {@link #xrDestroyEnvironmentDepthSwapchainMETA DestroyEnvironmentDepthSwapchainMETA} function is defined as:
*
*
* XrResult xrDestroyEnvironmentDepthSwapchainMETA(
* XrEnvironmentDepthSwapchainMETA swapchain);
*
* Description
*
* The {@link #xrDestroyEnvironmentDepthSwapchainMETA DestroyEnvironmentDepthSwapchainMETA} function destroys a readable environment depth swapchain.
*
* All submitted graphics API commands that refer to {@code swapchain} must have completed execution. Runtimes may continue to utilize swapchain images after {@link #xrDestroyEnvironmentDepthSwapchainMETA DestroyEnvironmentDepthSwapchainMETA} is called.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrDestroyEnvironmentDepthSwapchainMETA DestroyEnvironmentDepthSwapchainMETA}
* - {@code swapchain} must be a valid {@code XrEnvironmentDepthSwapchainMETA} handle
*
*
* Thread Safety
*
*
* - Access to {@code swapchain}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* @param swapchain the {@code XrEnvironmentDepthSwapchainMETA} to be destroyed.
*/
@NativeType("XrResult")
public static int xrDestroyEnvironmentDepthSwapchainMETA(XrEnvironmentDepthSwapchainMETA swapchain) {
long __functionAddress = swapchain.getCapabilities().xrDestroyEnvironmentDepthSwapchainMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPI(swapchain.address(), __functionAddress);
}
// --- [ xrEnumerateEnvironmentDepthSwapchainImagesMETA ] ---
/**
* Unsafe version of: {@link #xrEnumerateEnvironmentDepthSwapchainImagesMETA EnumerateEnvironmentDepthSwapchainImagesMETA}
*
* @param imageCapacityInput the capacity of the images array, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrEnumerateEnvironmentDepthSwapchainImagesMETA(XrEnvironmentDepthSwapchainMETA swapchain, int imageCapacityInput, long imageCountOutput, long images) {
long __functionAddress = swapchain.getCapabilities().xrEnumerateEnvironmentDepthSwapchainImagesMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(swapchain.address(), imageCapacityInput, imageCountOutput, images, __functionAddress);
}
/**
* Gets images from a readable depth swapchain.
*
* C Specification
*
* The {@link #xrEnumerateEnvironmentDepthSwapchainImagesMETA EnumerateEnvironmentDepthSwapchainImagesMETA} function is defined as:
*
*
* XrResult xrEnumerateEnvironmentDepthSwapchainImagesMETA(
* XrEnvironmentDepthSwapchainMETA swapchain,
* uint32_t imageCapacityInput,
* uint32_t* imageCountOutput,
* XrSwapchainImageBaseHeader* images);
*
* Description
*
* {@link #xrEnumerateEnvironmentDepthSwapchainImagesMETA EnumerateEnvironmentDepthSwapchainImagesMETA} fills an array of graphics API-specific stext:XrSwapchainImage* structures derived from {@link XrSwapchainImageBaseHeader}. The resources must be constant and valid for the lifetime of the {@code XrEnvironmentDepthSwapchainMETA}. This function behaves analogously to {@link XR10#xrEnumerateSwapchainImages EnumerateSwapchainImages}.
*
* Runtimes must always return identical buffer contents from this enumeration for the lifetime of the swapchain.
*
* Note: {@code images} is a pointer to an array of structures of graphics API-specific type, not an array of structure pointers.
*
* The pointer submitted as {@code images} will be treated as an array of the expected graphics API-specific type based on the graphics API used at session creation time. If the type member of any array element accessed in this way does not match the expected value, the runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrEnumerateEnvironmentDepthSwapchainImagesMETA EnumerateEnvironmentDepthSwapchainImagesMETA}
* - {@code swapchain} must be a valid {@code XrEnvironmentDepthSwapchainMETA} handle
* - {@code imageCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code imageCapacityInput} is not 0, {@code images} must be a pointer to an array of {@code imageCapacityInput} {@link XrSwapchainImageBaseHeader}-based structures. See also: {@link XrSwapchainImageOpenGLKHR}, {@link XrSwapchainImageVulkanKHR}
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
*
*
*
* See Also
*
* {@link XrSwapchainImageBaseHeader}
*
* @param swapchain the {@code XrEnvironmentDepthSwapchainMETA} to get images from.
* @param imageCountOutput a pointer to the count of images written, or a pointer to the required capacity in the case that {@code imageCapacityInput} is insufficient.
* @param images a pointer to an array of graphics API-specific XrSwapchainImage structures, all of the same type, based on {@link XrSwapchainImageBaseHeader}. It can be {@code NULL} if {@code imageCapacityInput} is 0.
*/
@NativeType("XrResult")
public static int xrEnumerateEnvironmentDepthSwapchainImagesMETA(XrEnvironmentDepthSwapchainMETA swapchain, @NativeType("uint32_t *") IntBuffer imageCountOutput, @NativeType("XrSwapchainImageBaseHeader *") XrSwapchainImageBaseHeader.@Nullable Buffer images) {
if (CHECKS) {
check(imageCountOutput, 1);
}
return nxrEnumerateEnvironmentDepthSwapchainImagesMETA(swapchain, remainingSafe(images), memAddress(imageCountOutput), memAddressSafe(images));
}
// --- [ xrGetEnvironmentDepthSwapchainStateMETA ] ---
/** Unsafe version of: {@link #xrGetEnvironmentDepthSwapchainStateMETA GetEnvironmentDepthSwapchainStateMETA} */
public static int nxrGetEnvironmentDepthSwapchainStateMETA(XrEnvironmentDepthSwapchainMETA swapchain, long state) {
long __functionAddress = swapchain.getCapabilities().xrGetEnvironmentDepthSwapchainStateMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(swapchain.address(), state, __functionAddress);
}
/**
* Returns the state of the readable depth swapchain.
*
* C Specification
*
* The {@link #xrGetEnvironmentDepthSwapchainStateMETA GetEnvironmentDepthSwapchainStateMETA} function is defined as:
*
*
* XrResult xrGetEnvironmentDepthSwapchainStateMETA(
* XrEnvironmentDepthSwapchainMETA swapchain,
* XrEnvironmentDepthSwapchainStateMETA* state);
*
* Description
*
* {@link #xrGetEnvironmentDepthSwapchainStateMETA GetEnvironmentDepthSwapchainStateMETA} retrieves information about the {@code XrEnvironmentDepthSwapchainMETA}. This information is constant throughout the lifetime of the {@code XrEnvironmentDepthSwapchainMETA}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrGetEnvironmentDepthSwapchainStateMETA GetEnvironmentDepthSwapchainStateMETA}
* - {@code swapchain} must be a valid {@code XrEnvironmentDepthSwapchainMETA} handle
* - {@code state} must be a pointer to an {@link XrEnvironmentDepthSwapchainStateMETA} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link XrEnvironmentDepthSwapchainStateMETA}
*
* @param swapchain an {@code XrEnvironmentDepthSwapchainMETA} handle.
* @param state a pointer to an {@link XrEnvironmentDepthSwapchainStateMETA}.
*/
@NativeType("XrResult")
public static int xrGetEnvironmentDepthSwapchainStateMETA(XrEnvironmentDepthSwapchainMETA swapchain, @NativeType("XrEnvironmentDepthSwapchainStateMETA *") XrEnvironmentDepthSwapchainStateMETA state) {
return nxrGetEnvironmentDepthSwapchainStateMETA(swapchain, state.address());
}
// --- [ xrAcquireEnvironmentDepthImageMETA ] ---
/** Unsafe version of: {@link #xrAcquireEnvironmentDepthImageMETA AcquireEnvironmentDepthImageMETA} */
public static int nxrAcquireEnvironmentDepthImageMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider, long acquireInfo, long environmentDepthImage) {
long __functionAddress = environmentDepthProvider.getCapabilities().xrAcquireEnvironmentDepthImageMETA;
if (CHECKS) {
check(__functionAddress);
XrEnvironmentDepthImageAcquireInfoMETA.validate(acquireInfo);
}
return callPPPI(environmentDepthProvider.address(), acquireInfo, environmentDepthImage, __functionAddress);
}
/**
* Returns an image index in a readable depth swapchain and associated metadata.
*
* C Specification
*
* The {@link #xrAcquireEnvironmentDepthImageMETA AcquireEnvironmentDepthImageMETA} function is defined as:
*
*
* XrResult xrAcquireEnvironmentDepthImageMETA(
* XrEnvironmentDepthProviderMETA environmentDepthProvider,
* const XrEnvironmentDepthImageAcquireInfoMETA* acquireInfo,
* XrEnvironmentDepthImageMETA* environmentDepthImage);
*
* Description
*
* Acquires the latest available swapchain image that has been generated by the depth provider and ensures it is ready to be accessed by the application. The application may access and queue GPU operations using the acquired image until the next {@link XR10#xrEndFrame EndFrame} call, when the image is released and the depth provider may write new depth data into it after completion of all work queued before the {@link XR10#xrEndFrame EndFrame} call.
*
* The returned {@link XrEnvironmentDepthImageMETA} contains the swapchain index into the array enumerated by {@link #xrEnumerateEnvironmentDepthSwapchainImagesMETA EnumerateEnvironmentDepthSwapchainImagesMETA}. It also contains other information such as the field of view and pose that are necessary to interpret the depth data.
*
* There must be no more than one call to {@link #xrAcquireEnvironmentDepthImageMETA AcquireEnvironmentDepthImageMETA} between any pair of corresponding {@link XR10#xrBeginFrame BeginFrame} and {@link XR10#xrEndFrame EndFrame} calls in a session.
*
*
* - The runtime may block if previously acquired swapchain images are still being used by the graphics API.
* - The runtime must return {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID} if {@link #xrAcquireEnvironmentDepthImageMETA AcquireEnvironmentDepthImageMETA} is called before {@link XR10#xrBeginFrame BeginFrame} or after {@link XR10#xrEndFrame EndFrame}.
* - The runtime must return {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID} if {@link #xrAcquireEnvironmentDepthImageMETA AcquireEnvironmentDepthImageMETA} is called on a stopped {@code XrEnvironmentDepthProviderMETA}.
* - The runtime must return {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED} if {@link #xrAcquireEnvironmentDepthImageMETA AcquireEnvironmentDepthImageMETA} is called more than once per frame - i.e. in a running session, after a call to {@link XR10#xrBeginFrame BeginFrame} that has not had an associated {@link XR10#xrEndFrame EndFrame}.
* - Runtimes must return {@link #XR_ENVIRONMENT_DEPTH_NOT_AVAILABLE_META ENVIRONMENT_DEPTH_NOT_AVAILABLE_META} if no depth frame is available yet (i.e. the provider was recently started and did not yet have time to compute depth). Note that this is a success code. In this case the output parameters must be unchanged.
* - The application must not utilize the swapchain image in calls to the graphics API after {@link XR10#xrEndFrame EndFrame} has been called.
* - A runtime may use the graphics API specific contexts provided to OpenXR. In particular:
*
*
* - For OpenGL, a runtime may use the OpenGL context specified in the call to {@link XR10#xrCreateSession CreateSession}, which needs external synchronization.
* - For Vulkan, a runtime may use the {@code VkQueue} specified in the {@link XrGraphicsBindingVulkan2KHR}, which needs external synchronization.
*
*
*
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrAcquireEnvironmentDepthImageMETA AcquireEnvironmentDepthImageMETA}
* - {@code environmentDepthProvider} must be a valid {@code XrEnvironmentDepthProviderMETA} handle
* - {@code acquireInfo} must be a pointer to a valid {@link XrEnvironmentDepthImageAcquireInfoMETA} structure
* - {@code environmentDepthImage} must be a pointer to an {@link XrEnvironmentDepthImageMETA} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
* - {@link #XR_ENVIRONMENT_DEPTH_NOT_AVAILABLE_META ENVIRONMENT_DEPTH_NOT_AVAILABLE_META}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
* - {@link XR10#XR_ERROR_CALL_ORDER_INVALID ERROR_CALL_ORDER_INVALID}
*
*
*
* See Also
*
* {@link XrEnvironmentDepthImageAcquireInfoMETA}, {@link XrEnvironmentDepthImageMETA}
*
* @param environmentDepthProvider an {@code XrEnvironmentDepthProviderMETA} handle for the depth provider.
* @param acquireInfo an {@link XrEnvironmentDepthImageAcquireInfoMETA} containing parameters for populating a depth swapchain image.
* @param environmentDepthImage the returned {@link XrEnvironmentDepthImageMETA} containing information about the acquired depth image.
*/
@NativeType("XrResult")
public static int xrAcquireEnvironmentDepthImageMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider, @NativeType("XrEnvironmentDepthImageAcquireInfoMETA const *") XrEnvironmentDepthImageAcquireInfoMETA acquireInfo, @NativeType("XrEnvironmentDepthImageMETA *") XrEnvironmentDepthImageMETA environmentDepthImage) {
return nxrAcquireEnvironmentDepthImageMETA(environmentDepthProvider, acquireInfo.address(), environmentDepthImage.address());
}
// --- [ xrSetEnvironmentDepthHandRemovalMETA ] ---
/** Unsafe version of: {@link #xrSetEnvironmentDepthHandRemovalMETA SetEnvironmentDepthHandRemovalMETA} */
public static int nxrSetEnvironmentDepthHandRemovalMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider, long setInfo) {
long __functionAddress = environmentDepthProvider.getCapabilities().xrSetEnvironmentDepthHandRemovalMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(environmentDepthProvider.address(), setInfo, __functionAddress);
}
/**
* Enables/disables hand removal from the depth map.
*
* C Specification
*
* The {@link #xrSetEnvironmentDepthHandRemovalMETA SetEnvironmentDepthHandRemovalMETA} function is defined as:
*
*
* XrResult xrSetEnvironmentDepthHandRemovalMETA(
* XrEnvironmentDepthProviderMETA environmentDepthProvider,
* const XrEnvironmentDepthHandRemovalSetInfoMETA* setInfo);
*
* Description
*
* The {@link #xrSetEnvironmentDepthHandRemovalMETA SetEnvironmentDepthHandRemovalMETA} function sets hand removal options.
*
* Runtimes should enable or disable the removal of the hand depths from the depth map. If enabled, the corresponding depth pixels should be replaced with the estimated background depth behind the hands. Runtimes must return {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED} if and only if {@link XrSystemEnvironmentDepthPropertiesMETA}{@code ::supportsHandRemoval} is {@link XR10#XR_FALSE FALSE}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAEnvironmentDepth XR_META_environment_depth} extension must be enabled prior to calling {@link #xrSetEnvironmentDepthHandRemovalMETA SetEnvironmentDepthHandRemovalMETA}
* - {@code environmentDepthProvider} must be a valid {@code XrEnvironmentDepthProviderMETA} handle
* - {@code setInfo} must be a pointer to a valid {@link XrEnvironmentDepthHandRemovalSetInfoMETA} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrEnvironmentDepthHandRemovalSetInfoMETA}
*
* @param environmentDepthProvider an {@code XrEnvironmentDepthProviderMETA} handle for the depth provider.
* @param setInfo a pointer to an {@link XrEnvironmentDepthHandRemovalSetInfoMETA} containing options for the hand removal.
*/
@NativeType("XrResult")
public static int xrSetEnvironmentDepthHandRemovalMETA(XrEnvironmentDepthProviderMETA environmentDepthProvider, @NativeType("XrEnvironmentDepthHandRemovalSetInfoMETA const *") XrEnvironmentDepthHandRemovalSetInfoMETA setInfo) {
return nxrSetEnvironmentDepthHandRemovalMETA(environmentDepthProvider, setInfo.address());
}
}