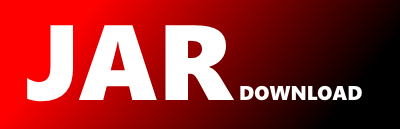
org.lwjgl.openxr.METAPerformanceMetrics Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_META_performance_metrics extension.
*
* This extension provides APIs to enumerate and query performance metrics counters of the current XR device and XR application. Developers can perform performance analysis and do targeted optimization to the XR application using the performance metrics counters being collected. The application should not change its behavior based on the counter reads.
*
* The performance metrics counters are organized into predefined {@code XrPath} values, under the root path pathname:/perfmetrics_meta. An application can query the available counters through {@link #xrEnumeratePerformanceMetricsCounterPathsMETA EnumeratePerformanceMetricsCounterPathsMETA}. Here is a list of the performance metrics counter paths that may be provided on Meta devices:
*
*
* - pathname:/perfmetrics_meta/app/cpu_frametime
* - pathname:/perfmetrics_meta/app/gpu_frametime
* - pathname:/perfmetrics_meta/app/motion_to_photon_latency
* - pathname:/perfmetrics_meta/compositor/cpu_frametime
* - pathname:/perfmetrics_meta/compositor/gpu_frametime
* - pathname:/perfmetrics_meta/compositor/dropped_frame_count
* - pathname:/perfmetrics_meta/compositor/spacewarp_mode
* - pathname:/perfmetrics_meta/device/cpu_utilization_average
* - pathname:/perfmetrics_meta/device/cpu_utilization_worst
* - pathname:/perfmetrics_meta/device/gpu_utilization
* - pathname:/perfmetrics_meta/device/cpu0_utilization through pathname:/perfmetrics_meta/device/cpuX_utilization
*
*
* After a session is created, an application can use {@link #xrSetPerformanceMetricsStateMETA SetPerformanceMetricsStateMETA} to enable the performance metrics system for that session. An application can use {@link #xrQueryPerformanceMetricsCounterMETA QueryPerformanceMetricsCounterMETA} to query a performance metrics counter on a session that has the performance metrics system enabled, or use {@link #xrGetPerformanceMetricsStateMETA GetPerformanceMetricsStateMETA} to query if the performance metrics system is enabled.
*
* Note: the measurement intervals of individual performance metrics counters are defined by the OpenXR runtime. The application must not make assumptions or change its behavior at runtime by measuring them.
*
* In order to enable the functionality of this extension, the application must pass the name of the extension into {@link XR10#xrCreateInstance CreateInstance} via the {@link XrInstanceCreateInfo}{@code ::enabledExtensionNames} parameter as indicated in the extension section.
*/
public class METAPerformanceMetrics {
/** The extension specification version. */
public static final int XR_META_performance_metrics_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_META_PERFORMANCE_METRICS_EXTENSION_NAME = "XR_META_performance_metrics";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_PERFORMANCE_METRICS_STATE_META TYPE_PERFORMANCE_METRICS_STATE_META}
* - {@link #XR_TYPE_PERFORMANCE_METRICS_COUNTER_META TYPE_PERFORMANCE_METRICS_COUNTER_META}
*
*/
public static final int
XR_TYPE_PERFORMANCE_METRICS_STATE_META = 1000232001,
XR_TYPE_PERFORMANCE_METRICS_COUNTER_META = 1000232002;
/**
* XrPerformanceMetricsCounterFlagBitsMETA - XrPerformanceMetricsCounterFlagBitsMETA
*
* Flag Descriptions
*
*
* - {@link #XR_PERFORMANCE_METRICS_COUNTER_ANY_VALUE_VALID_BIT_META PERFORMANCE_METRICS_COUNTER_ANY_VALUE_VALID_BIT_META} — Indicates any of the values in XrPerformanceMetricsCounterMETA is valid.
* - {@link #XR_PERFORMANCE_METRICS_COUNTER_UINT_VALUE_VALID_BIT_META PERFORMANCE_METRICS_COUNTER_UINT_VALUE_VALID_BIT_META} — Indicates the uintValue in XrPerformanceMetricsCounterMETA is valid.
* - {@link #XR_PERFORMANCE_METRICS_COUNTER_FLOAT_VALUE_VALID_BIT_META PERFORMANCE_METRICS_COUNTER_FLOAT_VALUE_VALID_BIT_META} — Indicates the floatValue in XrPerformanceMetricsCounterMETA is valid.
*
*/
public static final int
XR_PERFORMANCE_METRICS_COUNTER_ANY_VALUE_VALID_BIT_META = 0x1,
XR_PERFORMANCE_METRICS_COUNTER_UINT_VALUE_VALID_BIT_META = 0x2,
XR_PERFORMANCE_METRICS_COUNTER_FLOAT_VALUE_VALID_BIT_META = 0x4;
/**
* XrPerformanceMetricsCounterUnitMETA - XrPerformanceMetricsCounterUnitMETA
*
* Description
*
*
* Enum Description
*
* {@link #XR_PERFORMANCE_METRICS_COUNTER_UNIT_GENERIC_META PERFORMANCE_METRICS_COUNTER_UNIT_GENERIC_META} the performance counter unit is generic (unspecified).
* {@link #XR_PERFORMANCE_METRICS_COUNTER_UNIT_PERCENTAGE_META PERFORMANCE_METRICS_COUNTER_UNIT_PERCENTAGE_META} the performance counter unit is percentage (%).
* {@link #XR_PERFORMANCE_METRICS_COUNTER_UNIT_MILLISECONDS_META PERFORMANCE_METRICS_COUNTER_UNIT_MILLISECONDS_META} the performance counter unit is millisecond.
* {@link #XR_PERFORMANCE_METRICS_COUNTER_UNIT_BYTES_META PERFORMANCE_METRICS_COUNTER_UNIT_BYTES_META} the performance counter unit is byte.
* {@link #XR_PERFORMANCE_METRICS_COUNTER_UNIT_HERTZ_META PERFORMANCE_METRICS_COUNTER_UNIT_HERTZ_META} the performance counter unit is hertz (Hz).
*
*
*
* See Also
*
* {@link XrPerformanceMetricsCounterMETA}
*/
public static final int
XR_PERFORMANCE_METRICS_COUNTER_UNIT_GENERIC_META = 0,
XR_PERFORMANCE_METRICS_COUNTER_UNIT_PERCENTAGE_META = 1,
XR_PERFORMANCE_METRICS_COUNTER_UNIT_MILLISECONDS_META = 2,
XR_PERFORMANCE_METRICS_COUNTER_UNIT_BYTES_META = 3,
XR_PERFORMANCE_METRICS_COUNTER_UNIT_HERTZ_META = 4;
protected METAPerformanceMetrics() {
throw new UnsupportedOperationException();
}
// --- [ xrEnumeratePerformanceMetricsCounterPathsMETA ] ---
/**
* Unsafe version of: {@link #xrEnumeratePerformanceMetricsCounterPathsMETA EnumeratePerformanceMetricsCounterPathsMETA}
*
* @param counterPathCapacityInput the capacity of the {@code counterPaths} array, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrEnumeratePerformanceMetricsCounterPathsMETA(XrInstance instance, int counterPathCapacityInput, long counterPathCountOutput, long counterPaths) {
long __functionAddress = instance.getCapabilities().xrEnumeratePerformanceMetricsCounterPathsMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(instance.address(), counterPathCapacityInput, counterPathCountOutput, counterPaths, __functionAddress);
}
/**
* Enumerate all performance metrics counter paths that supported by the runtime.
*
* C Specification
*
* The {@link #xrEnumeratePerformanceMetricsCounterPathsMETA EnumeratePerformanceMetricsCounterPathsMETA} function enumerates all performance metrics counter paths that supported by the runtime, it is defined as:
*
*
* XrResult xrEnumeratePerformanceMetricsCounterPathsMETA(
* XrInstance instance,
* uint32_t counterPathCapacityInput,
* uint32_t* counterPathCountOutput,
* XrPath* counterPaths);
*
* Valid Usage (Implicit)
*
*
* - The {@link METAPerformanceMetrics XR_META_performance_metrics} extension must be enabled prior to calling {@link #xrEnumeratePerformanceMetricsCounterPathsMETA EnumeratePerformanceMetricsCounterPathsMETA}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code counterPathCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code counterPathCapacityInput} is not 0, {@code counterPaths} must be a pointer to an array of {@code counterPathCapacityInput} {@code XrPath} values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
*
*
*
* @param instance an {@code XrInstance} handle previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param counterPathCountOutput filled in by the runtime with the count of {@code counterPaths} written or the required capacity in the case that {@code counterPathCapacityInput} is insufficient.
* @param counterPaths an array of {@code XrPath} filled in by the runtime which contains all the available performance metrics counters, but can be {@code NULL} if {@code counterPathCapacityInput} is 0.
*/
@NativeType("XrResult")
public static int xrEnumeratePerformanceMetricsCounterPathsMETA(XrInstance instance, @NativeType("uint32_t *") IntBuffer counterPathCountOutput, @NativeType("XrPath *") @Nullable LongBuffer counterPaths) {
if (CHECKS) {
check(counterPathCountOutput, 1);
}
return nxrEnumeratePerformanceMetricsCounterPathsMETA(instance, remainingSafe(counterPaths), memAddress(counterPathCountOutput), memAddressSafe(counterPaths));
}
// --- [ xrSetPerformanceMetricsStateMETA ] ---
/** Unsafe version of: {@link #xrSetPerformanceMetricsStateMETA SetPerformanceMetricsStateMETA} */
public static int nxrSetPerformanceMetricsStateMETA(XrSession session, long state) {
long __functionAddress = session.getCapabilities().xrSetPerformanceMetricsStateMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(session.address(), state, __functionAddress);
}
/**
* Enable/disable performance metrics.
*
* C Specification
*
* The {@link #xrSetPerformanceMetricsStateMETA SetPerformanceMetricsStateMETA} function is defined as:
*
*
* XrResult xrSetPerformanceMetricsStateMETA(
* XrSession session,
* const XrPerformanceMetricsStateMETA* state);
*
* Description
*
* The {@link #xrSetPerformanceMetricsStateMETA SetPerformanceMetricsStateMETA} function enables or disables the performance metrics system.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAPerformanceMetrics XR_META_performance_metrics} extension must be enabled prior to calling {@link #xrSetPerformanceMetricsStateMETA SetPerformanceMetricsStateMETA}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code state} must be a pointer to a valid {@link XrPerformanceMetricsStateMETA} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link XrPerformanceMetricsStateMETA}, {@link #xrGetPerformanceMetricsStateMETA GetPerformanceMetricsStateMETA}
*
* @param session an {@code XrSession} handle previously created with {@link XR10#xrCreateSession CreateSession}.
* @param state a pointer to an {@link XrPerformanceMetricsStateMETA} structure.
*/
@NativeType("XrResult")
public static int xrSetPerformanceMetricsStateMETA(XrSession session, @NativeType("XrPerformanceMetricsStateMETA const *") XrPerformanceMetricsStateMETA state) {
return nxrSetPerformanceMetricsStateMETA(session, state.address());
}
// --- [ xrGetPerformanceMetricsStateMETA ] ---
/** Unsafe version of: {@link #xrGetPerformanceMetricsStateMETA GetPerformanceMetricsStateMETA} */
public static int nxrGetPerformanceMetricsStateMETA(XrSession session, long state) {
long __functionAddress = session.getCapabilities().xrGetPerformanceMetricsStateMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(session.address(), state, __functionAddress);
}
/**
* Get current state of performance metrics.
*
* C Specification
*
* The {@link #xrGetPerformanceMetricsStateMETA GetPerformanceMetricsStateMETA} function is defined as:
*
*
* XrResult xrGetPerformanceMetricsStateMETA(
* XrSession session,
* XrPerformanceMetricsStateMETA* state);
*
* Description
*
* The {@link #xrGetPerformanceMetricsStateMETA GetPerformanceMetricsStateMETA} function gets the current state of the performance metrics system.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAPerformanceMetrics XR_META_performance_metrics} extension must be enabled prior to calling {@link #xrGetPerformanceMetricsStateMETA GetPerformanceMetricsStateMETA}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code state} must be a pointer to an {@link XrPerformanceMetricsStateMETA} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link XrPerformanceMetricsStateMETA}, {@link #xrSetPerformanceMetricsStateMETA SetPerformanceMetricsStateMETA}
*
* @param session an {@code XrSession} handle previously created with {@link XR10#xrCreateSession CreateSession}.
* @param state a pointer to an {@link XrPerformanceMetricsStateMETA} structure.
*/
@NativeType("XrResult")
public static int xrGetPerformanceMetricsStateMETA(XrSession session, @NativeType("XrPerformanceMetricsStateMETA *") XrPerformanceMetricsStateMETA state) {
return nxrGetPerformanceMetricsStateMETA(session, state.address());
}
// --- [ xrQueryPerformanceMetricsCounterMETA ] ---
/** Unsafe version of: {@link #xrQueryPerformanceMetricsCounterMETA QueryPerformanceMetricsCounterMETA} */
public static int nxrQueryPerformanceMetricsCounterMETA(XrSession session, long counterPath, long counter) {
long __functionAddress = session.getCapabilities().xrQueryPerformanceMetricsCounterMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(session.address(), counterPath, counter, __functionAddress);
}
/**
* Query performance metrics counter.
*
* C Specification
*
* The {@link #xrQueryPerformanceMetricsCounterMETA QueryPerformanceMetricsCounterMETA} function is defined as:
*
*
* XrResult xrQueryPerformanceMetricsCounterMETA(
* XrSession session,
* XrPath counterPath,
* XrPerformanceMetricsCounterMETA* counter);
*
* Description
*
* The {@link #xrQueryPerformanceMetricsCounterMETA QueryPerformanceMetricsCounterMETA} function queries a performance metrics counter.
*
* The application should enable the performance metrics system (by calling {@link #xrSetPerformanceMetricsStateMETA SetPerformanceMetricsStateMETA}) before querying metrics using {@link #xrQueryPerformanceMetricsCounterMETA QueryPerformanceMetricsCounterMETA}. If the performance metrics system has not been enabled before calling {@link #xrQueryPerformanceMetricsCounterMETA QueryPerformanceMetricsCounterMETA}, the runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}.
*
* If {@code counterPath} is not in the list returned by {@link #xrEnumeratePerformanceMetricsCounterPathsMETA EnumeratePerformanceMetricsCounterPathsMETA}, the runtime must return {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METAPerformanceMetrics XR_META_performance_metrics} extension must be enabled prior to calling {@link #xrQueryPerformanceMetricsCounterMETA QueryPerformanceMetricsCounterMETA}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code counter} must be a pointer to an {@link XrPerformanceMetricsCounterMETA} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
*
*
*
* See Also
*
* {@link XrPerformanceMetricsCounterMETA}
*
* @param session an {@code XrSession} handle previously created with {@link XR10#xrCreateSession CreateSession}.
* @param counterPath a valid performance metrics counter path.
* @param counter a pointer to an {@link XrPerformanceMetricsCounterMETA} structure.
*/
@NativeType("XrResult")
public static int xrQueryPerformanceMetricsCounterMETA(XrSession session, @NativeType("XrPath") long counterPath, @NativeType("XrPerformanceMetricsCounterMETA *") XrPerformanceMetricsCounterMETA counter) {
return nxrQueryPerformanceMetricsCounterMETA(session, counterPath, counter.address());
}
}